This content originally appeared on DEV Community and was authored by David Morales
I’m going to show you how to create a project with Rails 6.1 and its default Webpacker version (4.5), and configure Tailwind CSS with its dependencies and JIT mode enabled.
You’ll end up with a project that compiles the final CSS automatically, and refreshes the browser for you as well, after every change.
Create a Rails application:
rails new tailwind-jit-rails
Install the Tailwind CSS dependencies. Open the terminal and run:
yarn add @fullhuman/postcss-purgecss@^4 postcss@^8 postcss-loader@^4 autoprefixer@^10 tailwindcss@^2
Create the file app/javascript/stylesheets/application.scss
(you will have to create the stylesheets
directory).
Now open application.scss
and add the Tailwind CSS directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
Remember that this is an SCSS file, so you can add here the SCSS code you need later.
Open app/javascript/packs/application.js
and add this import to include the new application.scss
:
import "stylesheets/application.scss"
Put that line below the last import
(channels).
Open postcss.config.js
and add this require
at the beginning of the plugins
array, just before the first require
:
require('tailwindcss')
Open app/views/layouts/application.html.erb
and add this before stylesheet_link_tag
:
<%= stylesheet_pack_tag 'application', 'data-turbolinks-track': 'reload' %>
So it will look like this:
<%= stylesheet_pack_tag 'application', 'data-turbolinks-track': 'reload' %>
<%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %>
<%= javascript_pack_tag 'application', 'data-turbolinks-track': 'reload' %>
Now you can create the Tailwind CSS configuration file in the terminal:
npx tailwindcss init
Enable JIT mode and configure the purge
option so that it looks like this:
mode: 'jit',
purge: [
'./app/views/**/*.html.erb',
'./app/helpers/**/*.rb',
'./app/javascript/**/*.js',
],
To prevent Webpacker from generating a rather annoying warning message, open babel.config.js
and add this at the bottom, just before the closing ].filter(Boolean)
:
['@babel/plugin-proposal-private-methods', { loose: true }],
['@babel/plugin-proposal-private-property-in-object', { loose: true }]
Remember to add a comma to the previous element. It will look like this:
[
'@babel/plugin-transform-regenerator',
{
async: false
}
],
['@babel/plugin-proposal-private-methods', { loose: true }],
['@babel/plugin-proposal-private-property-in-object', { loose: true }]
Finally, to get Webpacker 5 to work with PostCSS 8 we need to add a small hack. Open config/webpack/environment.js
and add this before module.exports = environment
:
// https://github.com/rails/webpacker/issues/2784#issuecomment-737003955
function hotfixPostcssLoaderConfig (subloader) {
const subloaderName = subloader.loader
if (subloaderName === 'postcss-loader') {
if (subloader.options.postcssOptions) {
console.log(
'\x1b[31m%s\x1b[0m',
'Remove postcssOptions workaround in config/webpack/environment.js'
)
} else {
subloader.options.postcssOptions = subloader.options.config;
delete subloader.options.config;
}
}
}
environment.loaders.keys().forEach(loaderName => {
const loader = environment.loaders.get(loaderName);
loader.use.forEach(hotfixPostcssLoaderConfig);
});
That’s it!
If you want to test if it works, create a test controller:
rails g controller Home index
Add the root
in config/routes.rb
:
root 'home#index'
Open app/views/home/index.html.erb
and create an H1
like this:
<h1 class="font-bold text-4xl text-center text-red-500">Hello World!</h1>
Now open the Webpacker server in a terminal tab:
bin/webpack-dev-server
And the Rails server in another tab:
bin/rails s
Open your browser and load localhost:3000
You should see a big, red Hello World!
Try making changes, and you’ll see how fast it compiles and refreshes the browser.
As an additional note, for the Tailwind CSS IntelliSense extension to work in html.erb
files you must open the preferences and look for the files.associations
option. Click the Add Item button and add *.html.erb
as item, and html
as value.
Enjoy!
This content originally appeared on DEV Community and was authored by David Morales
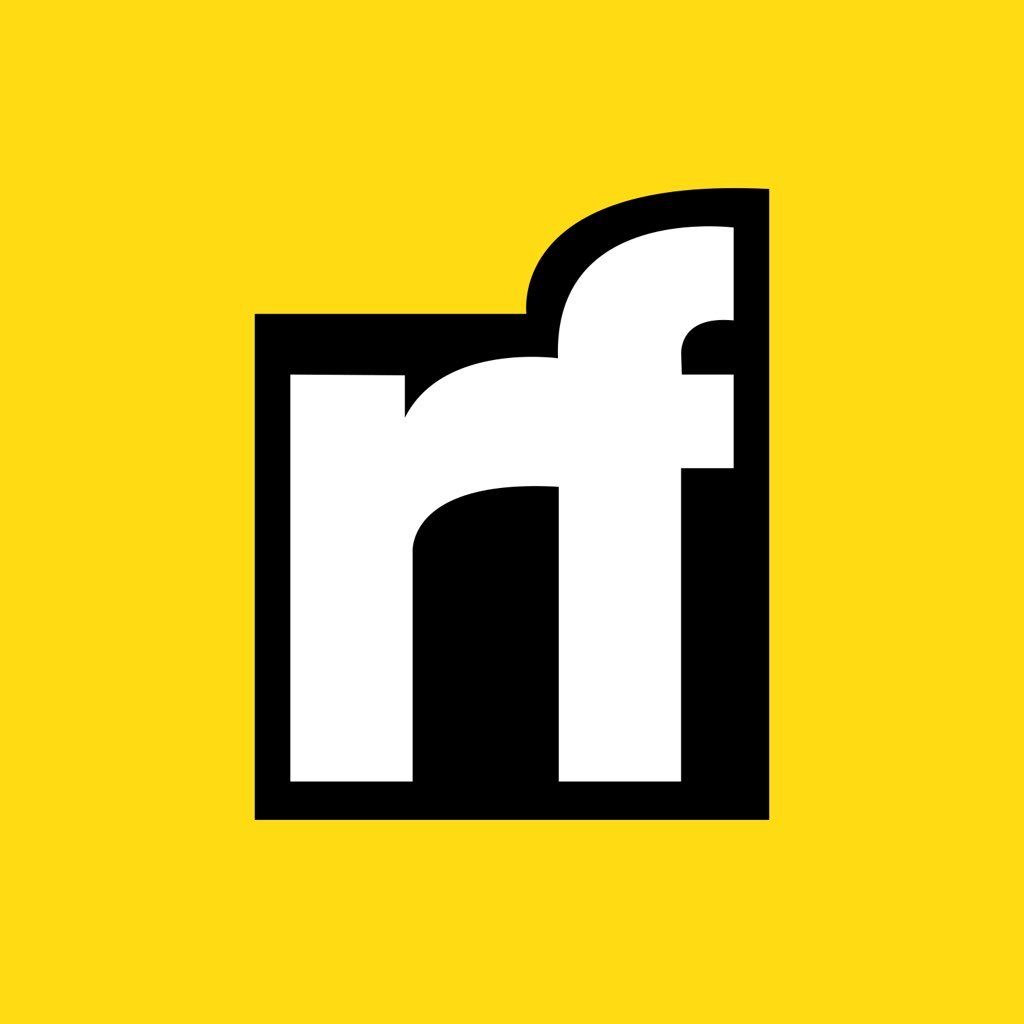
David Morales | Sciencx (2021-08-05T15:39:14+00:00) Setting Up Rails 6.1 + Tailwind CSS 2.2 with JIT. Retrieved from https://www.scien.cx/2021/08/05/setting-up-rails-6-1-tailwind-css-2-2-with-jit/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.