This content originally appeared on DEV Community and was authored by Haider Ali
Most of these JavaScript Hacks uses techniques from ECMAScript6(ES2015) onwards, though the latest version is ECMAScript11(ES2020).
Note : All the below tricks were tested using Google Chrome’s Console.
1. Declare and Initialize Arrays
You can use default values such as “”, null, or 0. to initialize an array of a particular size. These values can be used to initialize an array of a particular size with default values like “”, null or 0.
const array = Array(5).fill('');
// Output
(5) ["", "", "", "", ""]
const matrix = Array(5).fill(0).map(()=>Array(5).fill(0));
// Output
(5) [Array(5), Array(5), Array(5), Array(5), Array(5)]
0: (5) [0, 0, 0, 0, 0]
1: (5) [0, 0, 0, 0, 0]
2: (5) [0, 0, 0, 0, 0]
3: (5) [0, 0, 0, 0, 0]
4: (5) [0, 0, 0, 0, 0]
length: 5
2. Find out the sum, minimum and maximum value
To quickly find basic math operations, we should use reduce.
const array = [5,4,7,8,9,2];
Sum
array.reduce((a,b) => a+b);
// Output: 35
Max
array.reduce((a,b) => a>b?a:b);
// Output: 9
Min
array.reduce((a,b) => a<b?a:b);
// Output: 2
3. Sorting an array of strings, numbers or objects
There are inbuilt methods to sort strings, such as reverse() and sort(). But what about numbers and arrays of objects? Let’s look at sorting hacks that can be used for both Numbers and Objects in Increasing or Decreasing order.
Sort String Array
const stringArr = ["Joe", "Kapil", "Steve", "Musk"]
stringArr.sort()
// Output
(4) ["Joe", "Kapil", "Musk", "Steve"]
stringArr.reverse()
// Output
(4) ["Steve", "Musk", "Kapil", "Joe"]
__S.12__
Sort Number Array
const array = [40.100, 1, 5, 25, 10,];
array.sort(a,b). => a–b
// Output
(6) [1, 5, 10, 25, 40, 100]
array.sort((a.b) => (b-a);
// Output
(6) [100-40, 25, 10, 5, 1,]
__S.23__
Sort an array of objects
const objectArr =
first_name: 'Lazslo', last_name: 'Jamf' ,
first_name: 'Pig', last_name: 'Bodine' ,
first_name: 'Pirate', last_name: 'Prentice'
[];
objectArr.sort((a, b) => a.last_name.localeCompare(b.last_name));
// Output
(3) [{}, {}]
0: first_name: "Pig", last_name: "Bodine"
1: first_name: "Lazslo", last_name: "Jamf"
2: first_name: "Pirate", last_name: "Prentice"
Länge: 3
4. Do you ever need to filter out false values from an array?
You can easily omit false values such as 0, undefined null, false, or “”, by using the below trick
const array = [3,0, 6, 7, ''?, false]
array.filter(Boolean);
// Output
(3) [3, 6, 7, 7]
5. Use Logical Operators to deal with various conditions
You can use basic of logical operator OR to reduce nested If..else cases or switch cases.
function doSomething(arg1){function doSomething (arg1)
10;
// Set arg1-10 as the default, if it isn't already.
Return arg1
}
let foo = 10;
foo ===10 && doSomething()
// is the same as "foo == 10") then doSomething()
// Output: 10.
doSomething();
// is the same as "foo!= 5" then doSomething()
// Output: 10.
6. Get rid of duplicates
Perhaps you have used indexOf() in conjunction with for loop to return the first index found or the newer includes(), which returns boolean true/false of an array to remove duplicates. These are two faster approaches.
const array = [5,4,7.8,9,2,7.5,]
array.filter((item,idx,arr) => arr.indexOf(item) === idx);
//
const nonUnique = [...new Set(array)];
// Output: (5, 4, 7, 8, 9, 2, 2)
7. Create a Counter Object, or Map
Most of the time, the requirement to solve problem by creating counter object or map which tracks variables as keys with their frequency/occurrences as values.
let string = "kapilalipak"
={}; Const Table
for(let char of string) {For(let char string)
table[char]=table[char]+1 || 1;
}
// Output
k. 2, a. 3, p. 2, i. 2, l. 2
And
const countMap = New Map()
for (let i = 0; i < string.length; i++) {
if (countMap.has(string[i])) {
countMap.set(string[i], countMap.get(string[i]) + 1);
} else {or else
countMap.set(string[i], 1);
}
}
// Output
Map(5) "k” => 2, “a” => 3, “p” => 2, „i” => 2, „l” => 2
Read the Full official Article by Clicking Upper Canonical URL
Or
Read Full Article by HERE
This content originally appeared on DEV Community and was authored by Haider Ali
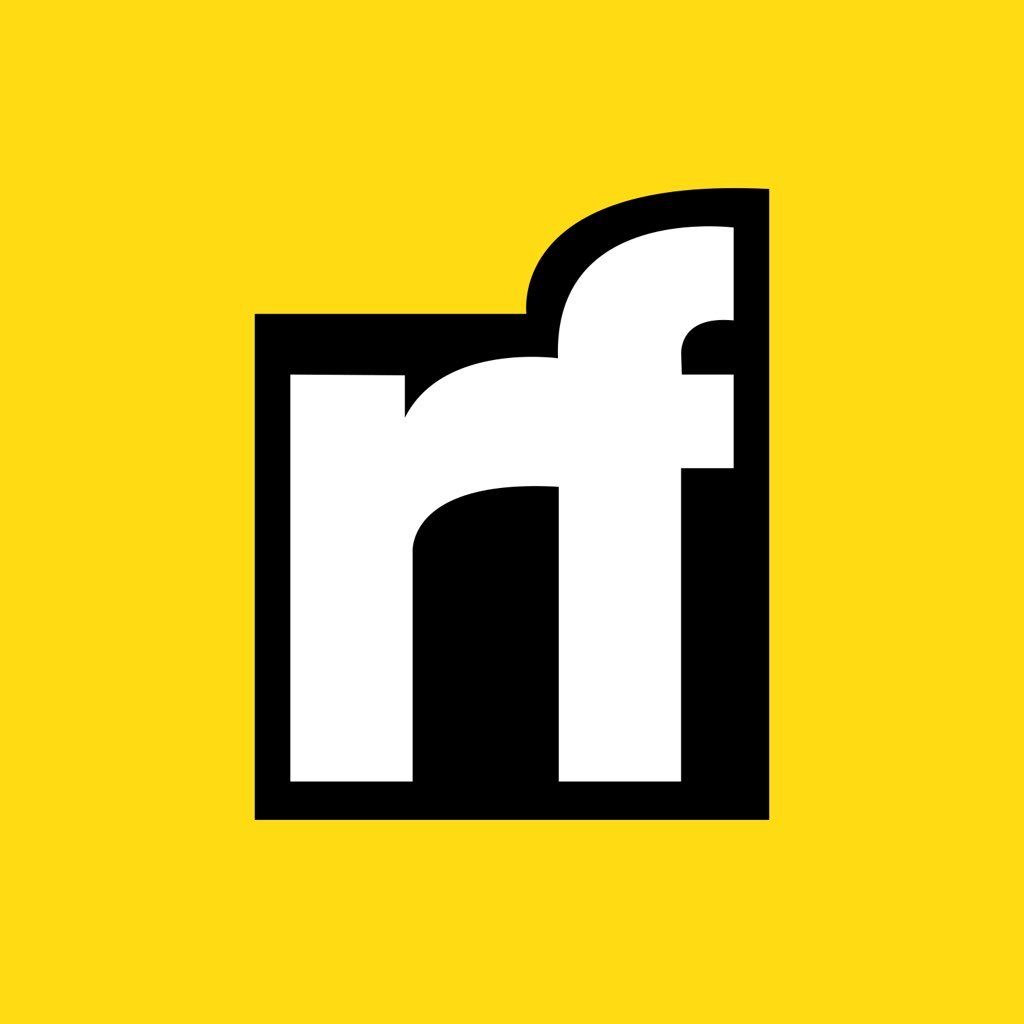
Haider Ali | Sciencx (2021-08-09T16:46:54+00:00) Top 20 JavaScript tips and tricks to increase your Speed and Efficiency. Retrieved from https://www.scien.cx/2021/08/09/top-20-javascript-tips-and-tricks-to-increase-your-speed-and-efficiency-6/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.