This content originally appeared on Level Up Coding - Medium and was authored by Austin Rhoads
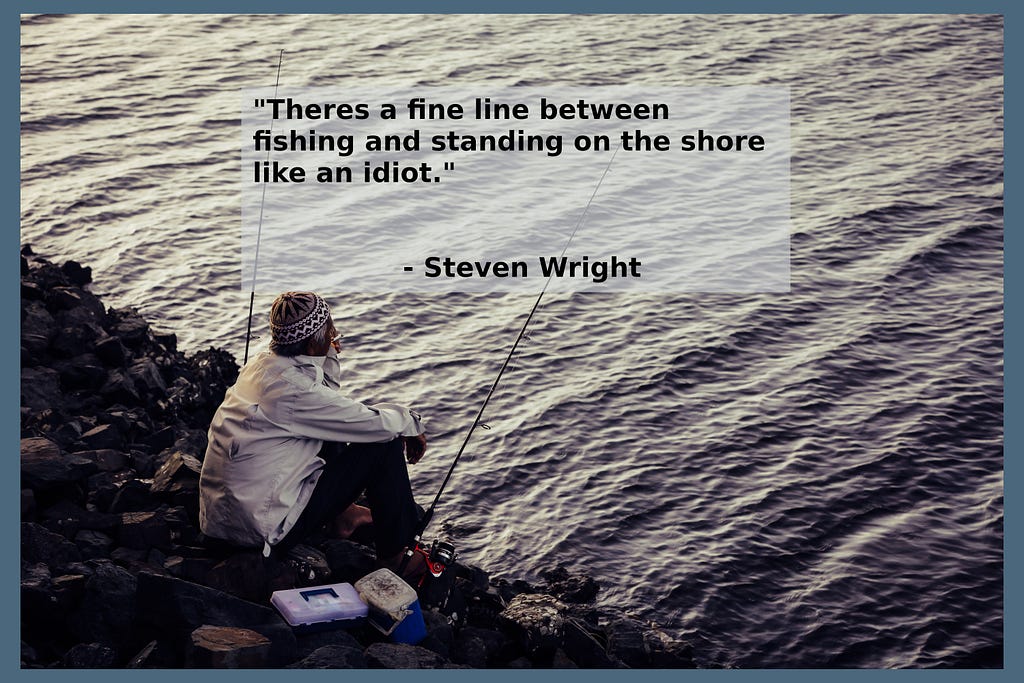
When I decided to make a React fishing app I knew it was crucial to allow users to save locations because one of the main differences between standing on the shore like an idiot and catching fish is knowing where to fish.
Whether you want to drop a pin on that new fishing spot where you caught a monster trout, provide directions to a local business or display search results for a realty website, adding a map to your application can be very powerful.
What To Expect From This Article
This is the first part in a series I am doing on building a high functioning dynamic map using GoogleMapReact in your React application. Assuming you are familiar with React by the end of this series you should be able to create your own map component and give it customized functionality based on your own needs. By the end of this article you should have a map up and running and be able to populate it with custom markers.
GoogleMapReact gives you access to the functionality of the Google Maps API but also allows you to render custom React components inside the map. In fact it will render any component onto the map that has lat and lng properties. Which is super awesome.
Google API
To get started you’ll need to create a Google API project. You can do so here. Once you’ve created your project enable the Maps JavaScript API and get an API key. There are many resources for how to do this and the Google documentation for this can be found here. Once you have your API key, hang on to it we’ll need it later.
The NPM Package
There are many different packages with similar names so make sure you’re using the google-map-react (NOT react-google-maps or google-maps-react). The correct package can be found here.
Install the package in your react application by running:
npm i google-map-react
Getting Up and Running
Next we are going to create our own map component to render in our app. We’ll import GoogleMapReact inside our MapExample component. Also, there are a few things required initially so the map will display and function correctly.
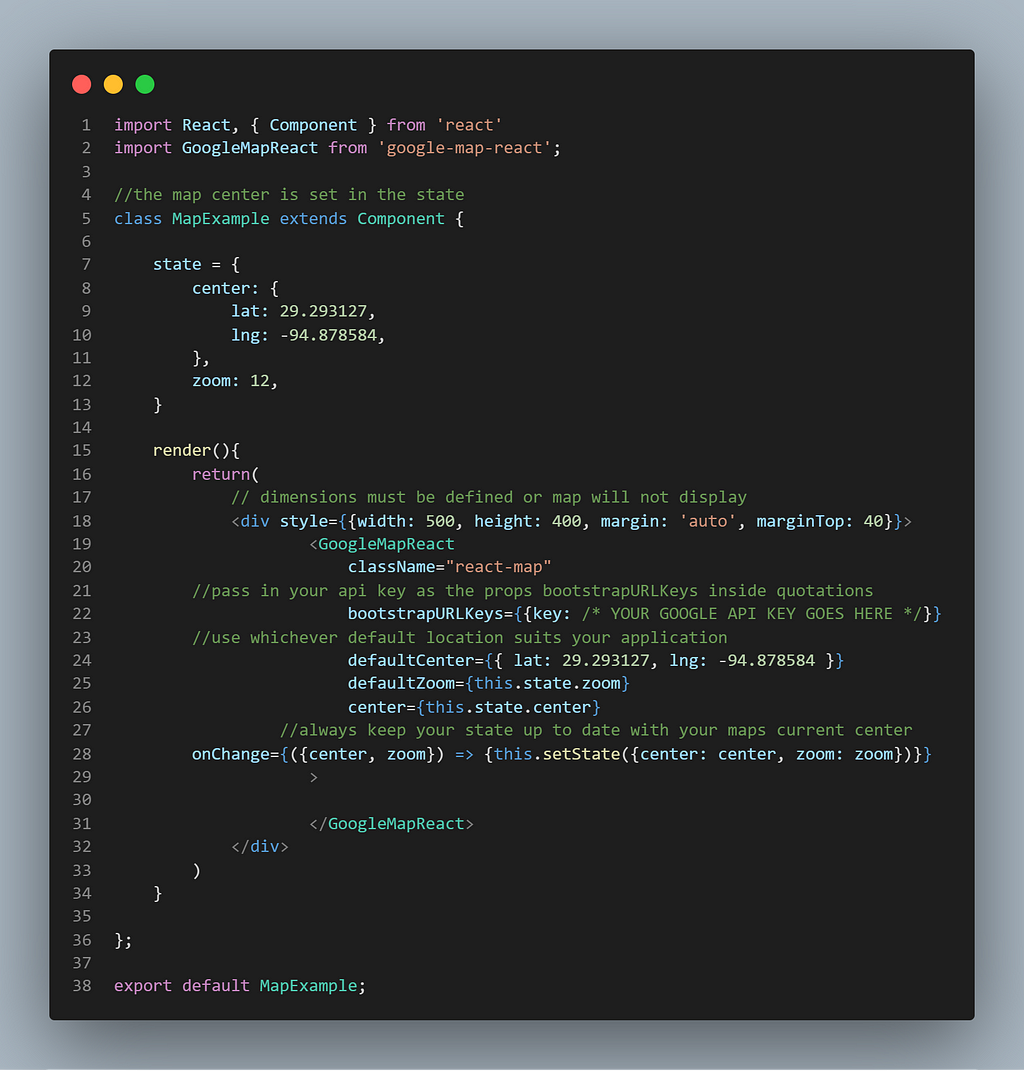
Set Inline Dimensions
Set the width and height of the MapExample component. As a requirement of all Google Maps, the imported map will fill the dimensions of it’s parent component but if there are no dimensions set, it will collapse down to zero. I set the dimensions with inline CSS to ensure it always loads with set dimensions.
Default Map Props
Before it loads, Google Maps looks for it’s defaultCenter, center and defaultZoom properties. defaultCenter and center should return an object that has lat and lng properties, and defaultZoom is an integer. I start with 12. The higher the zoom integer the more zoomed in it is.
In the MapExample I have the default lat and lng set to the spot where I caught all of these speckled trout. :)
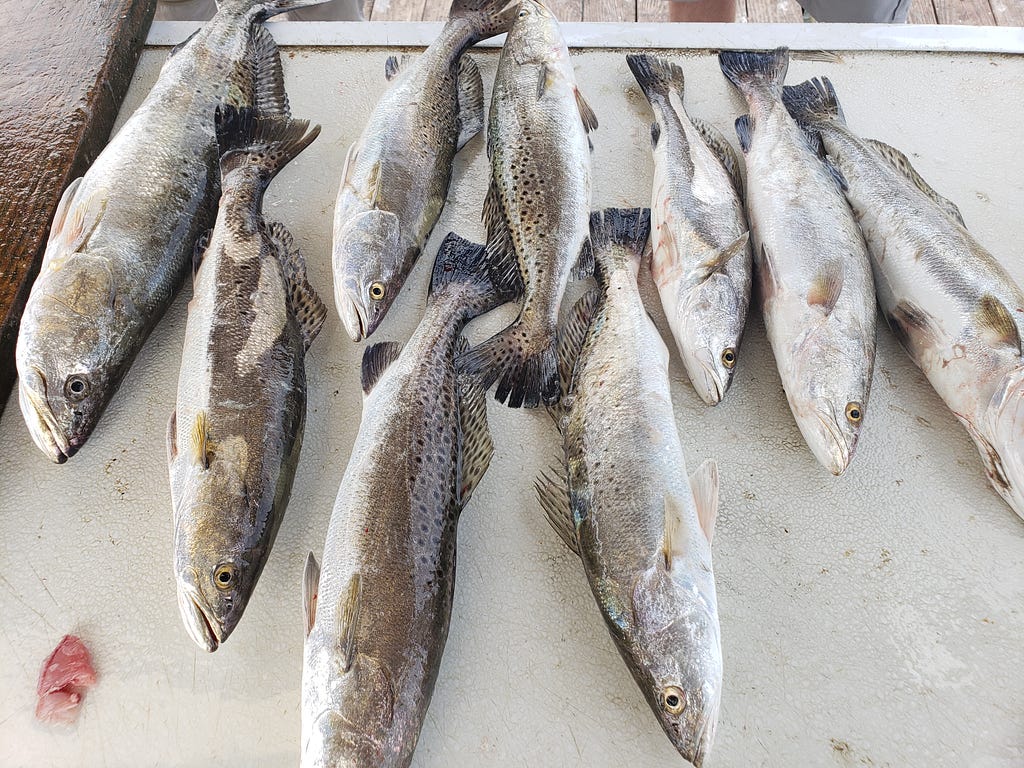
The center is set to this.state.center which for now is the same as the defaultCenter. You do not want the defaultCenter set to the state because Google Maps doesn’t like it when you change the default props and as you use the map you will change the state’s center property continuously.
Google API Key
In the GoogleMapReact object pass in your API key in the bootstrapURLKeys. Pass in an object that has a key property set to your API Key in quotations.
OnChange State Update
We want our state to always reflect the current position of the map center, which can be changed by dragging the map with the grabber tool. Luckily, built into the onChange prop is access to current center and zoom properties. Within this event handler we can update the state to wherever the map is currently pointing every time it changes.
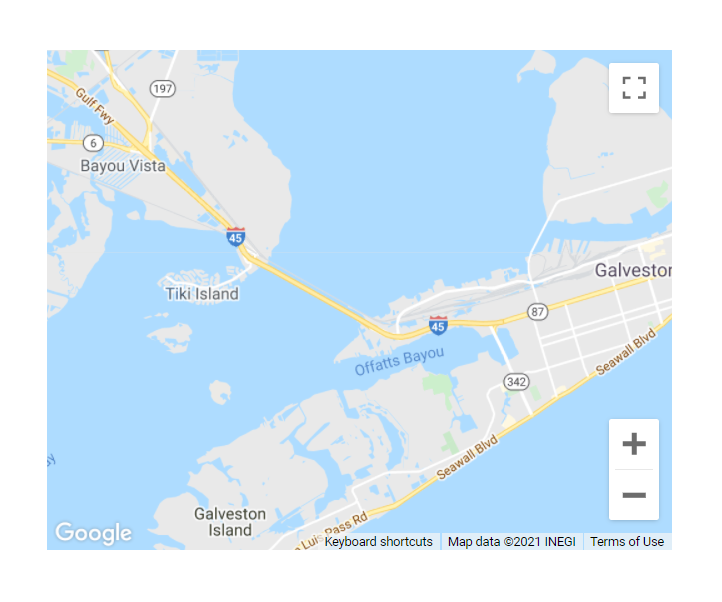
Now that we have our map up and running there are some basic functions you might want for your application.
Getting the User’s Location
Let’s go ahead and put a button in the MapExample component that when clicked grabs the user’s location and re-centers the map to that location.
First we grab the user’s location in the App component. Then we pass it down through Props to the MapExample component. I also save it to localStor
age for reasons which I’ll get into later.
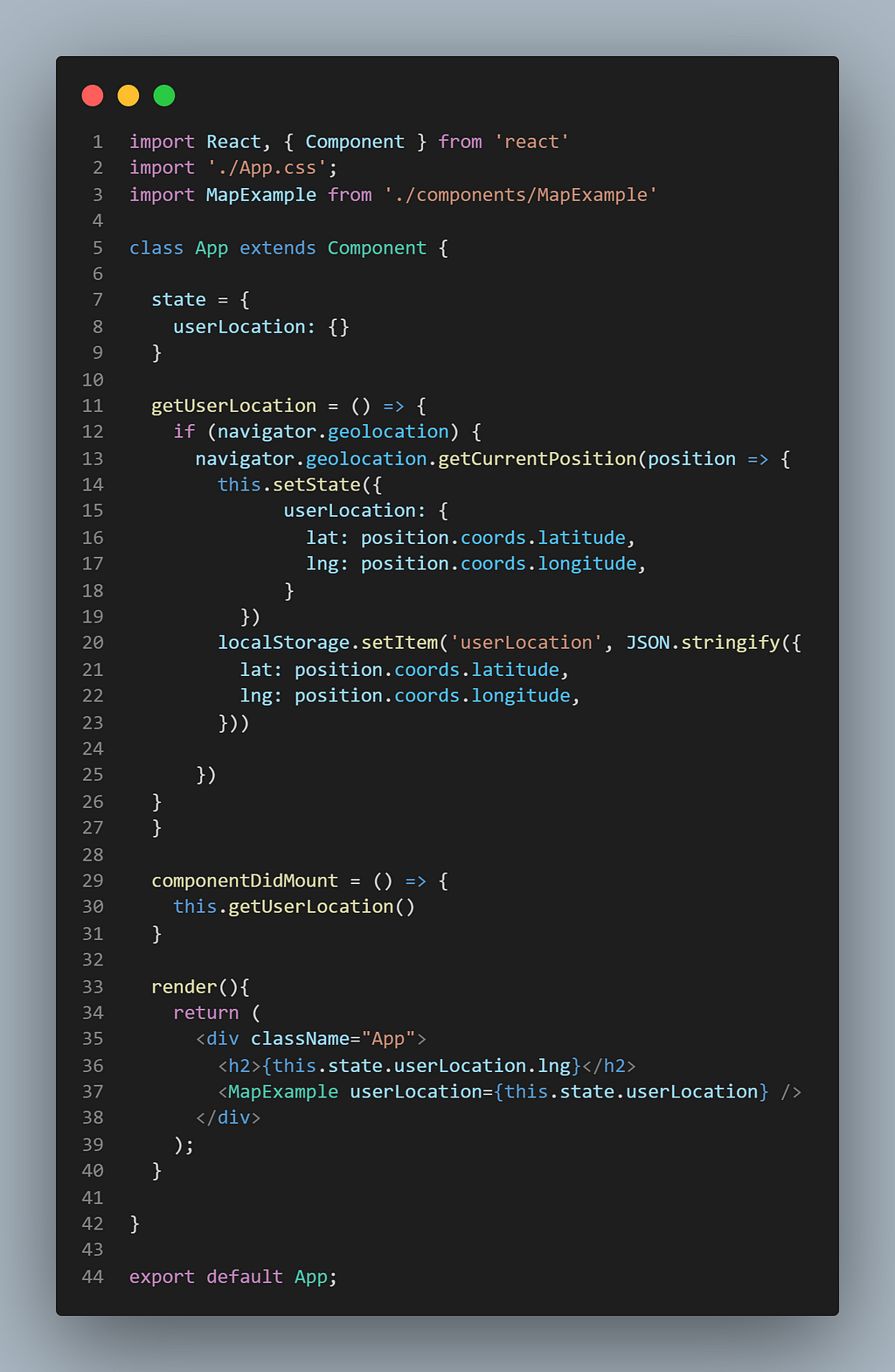
Inside the MapExample component we make a button which calls the centerMyLocation function:
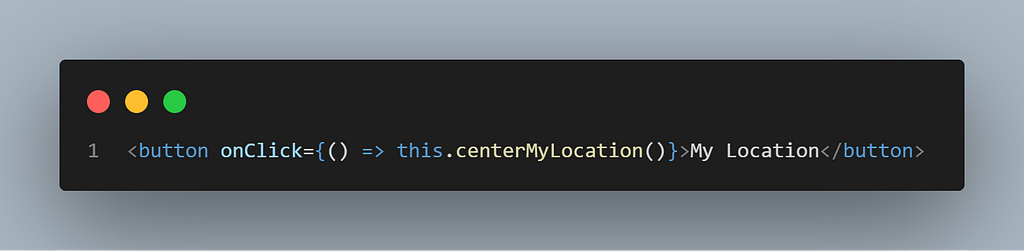
This function inside the MapExample component then centers the map over the user’s location by updating the state.
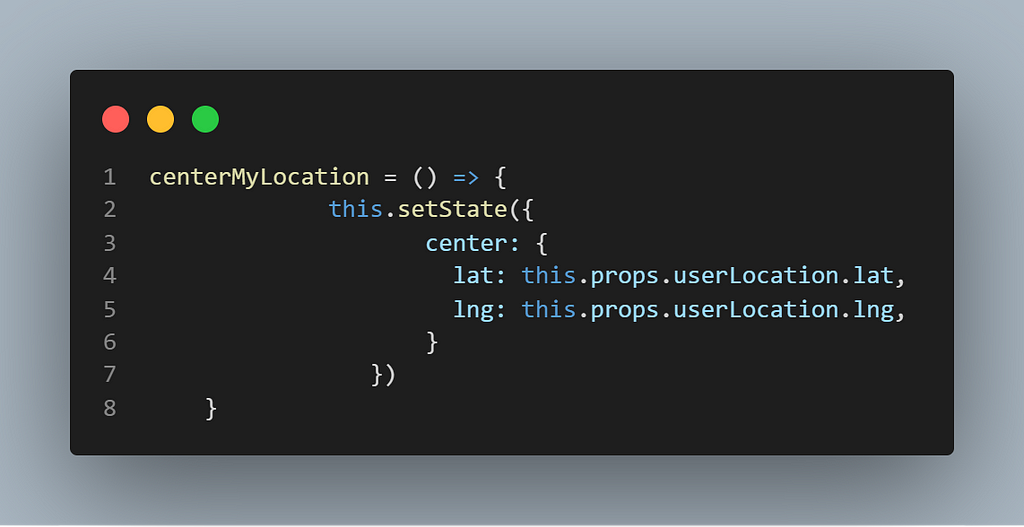
Placing Markers
As I said before, one of the coolest things about GoogleMapReact is that it will render any component onto the map that has lat and lng props. You can also create that component with functions that suit your applications specific needs. So let’s make some markers!
My Location Marker
First we’ll create a simple MyLocationMarker component that automatically displays the user’s location when the map loads. I’ve provided some inline CSS that will make the div a blue dot.
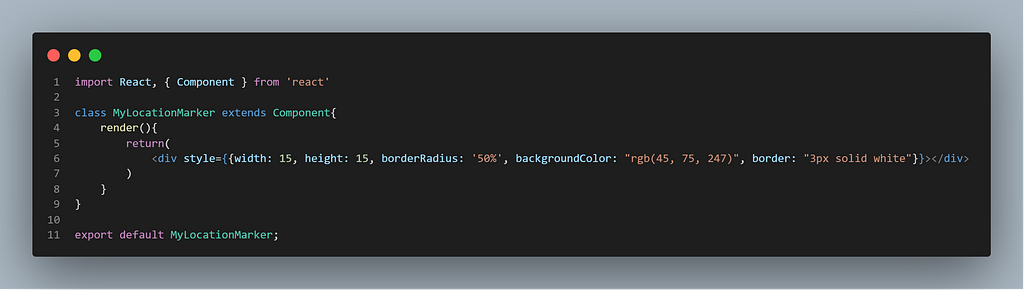
From here all we have to do is import the marker to the MapExample and pass in the user’s lat and lng as props.
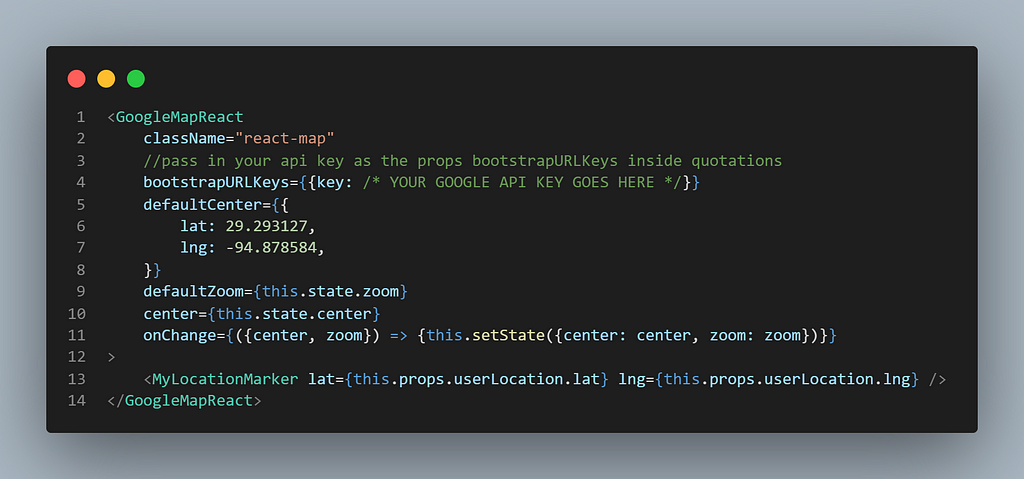
And Boom! We have our user’s location…
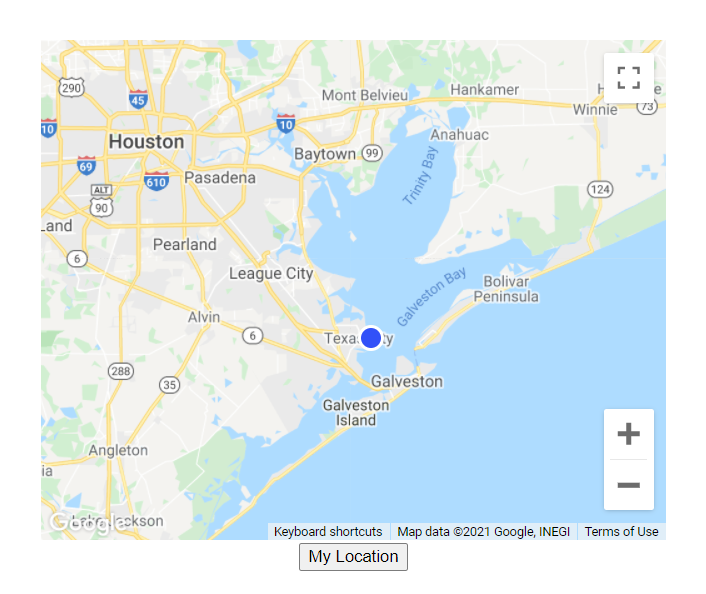
To get the map to automatically center on the user’s location when it loads there are several options. For this example I simply save the user’s location to localStorage and retrieve that in the MapExample component. Then I set it to the state with a logical OR operator.
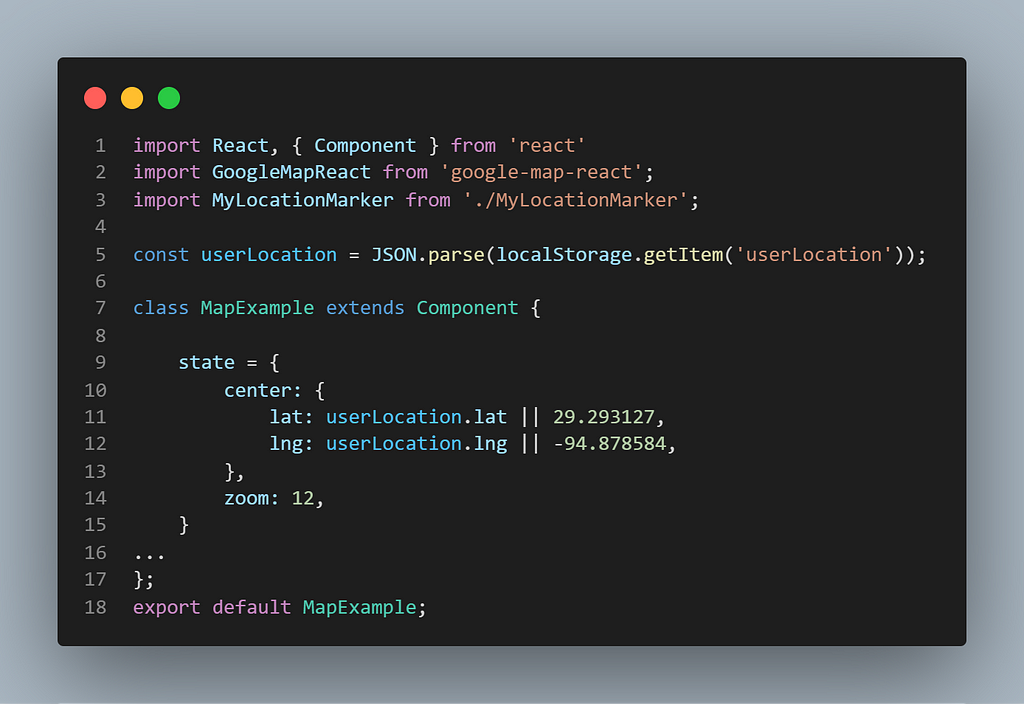
Re-Cap
So far in the first part of the series we’ve learned how to:
- Import google-map-react into a component.
- Set the default props.
- Access map properties from the onChange event handler.
- Get the user’s Location.
- Place a marker component set to the user’s location inside the map.
I hope this helps you feel more oriented towards using google-map-react. In the next part of the series I’ll go over how to create more functional marker components with info bubbles. Happy coding!
How to use google-maps-react: Part One was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Austin Rhoads
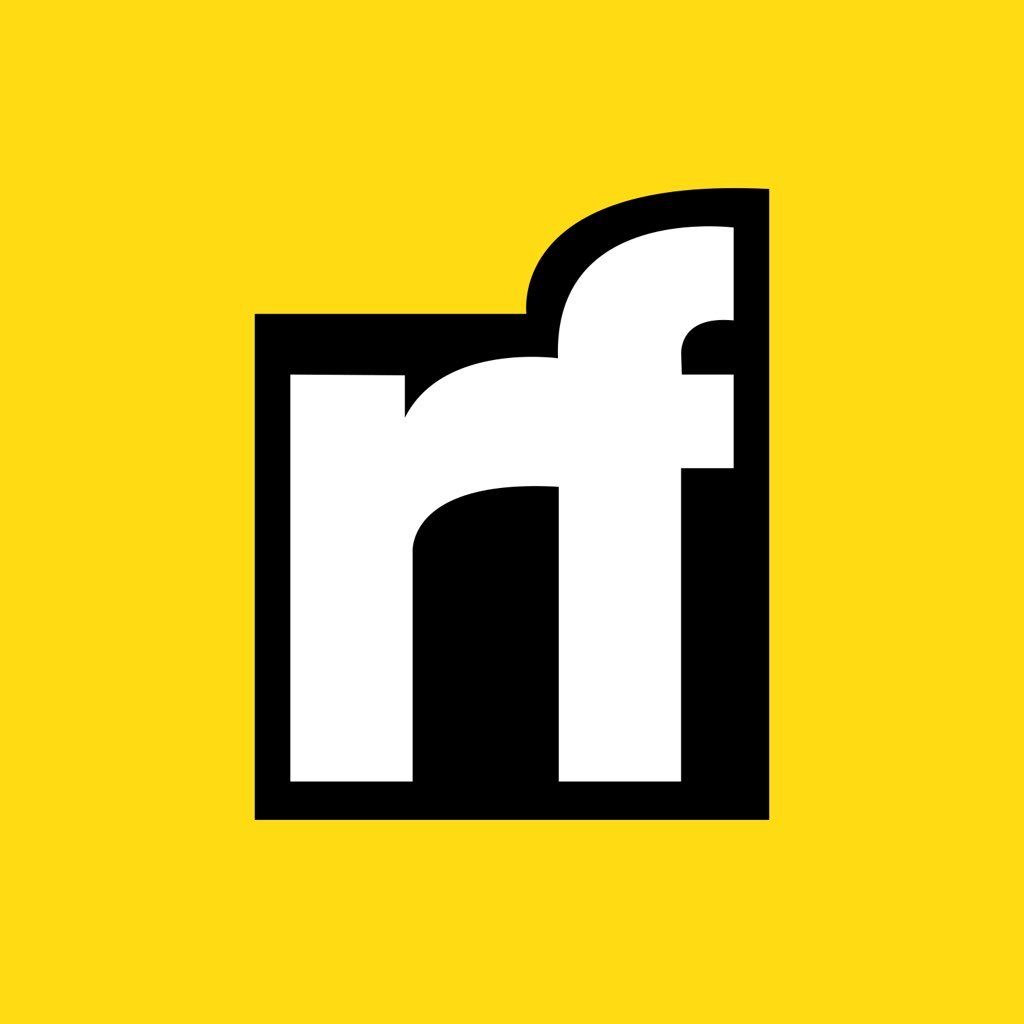
Austin Rhoads | Sciencx (2021-09-03T14:12:29+00:00) How to use google-maps-react: Part One. Retrieved from https://www.scien.cx/2021/09/03/how-to-use-google-maps-react-part-one/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.