This content originally appeared on Level Up Coding - Medium and was authored by Tara Prasad Routray
Use web workers to do parallel programming and perform multiple operations simultaneously.
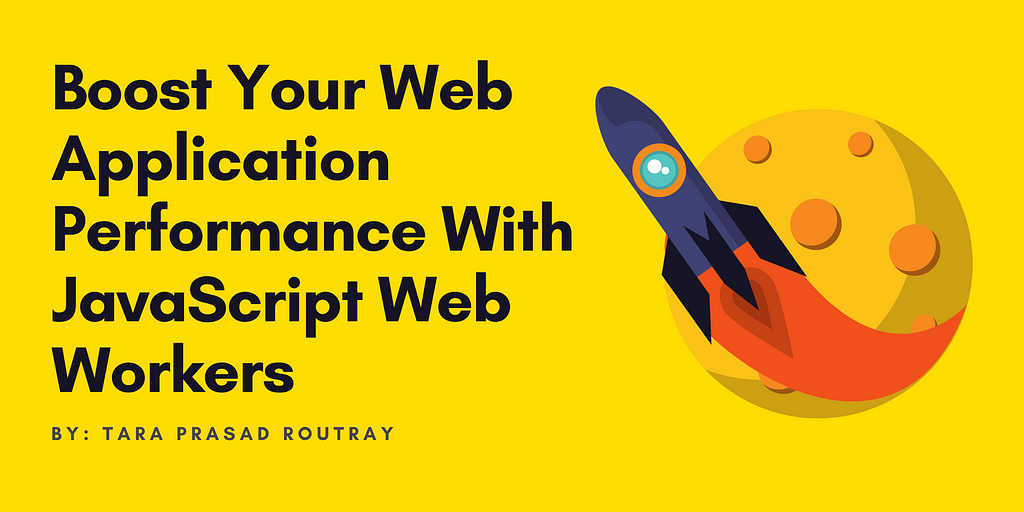
Through this article, I will give you a walkthrough of web workers, and how you can integrate them into your web applications.
Introduction To Web Workers
JavaScript web workers allow developers to perform parallel programming to help applications gain the power to run multiple computations at the same time.
These web workers can run scripts in the background threads, which are completely separate from the main thread. Because of this, a lot of workload gets reduced from the main thread. The major benefit of this workload separation is, you can execute CPU intensive operations within an isolated thread which will not interrupt or affect the reactivity and usability of the main thread. When the background thread completes its task, it instantly notifies the main thread about the results through an event, which is managed through the vanilla JavaScript event handling.
But, these web workers have some restrictions, such as not having access to the DOM and not having access to the web worker’s parent page (the page that created it). Keeping this in mind, you can now continue to learn how to create web workers.
Verify Web Worker Support
To have more controlled error handling and backwards compatibility, it is recommended to wrap your web worker’s code inside the following check. It would help to detect whether your browser supports web workers or not.
if (window.Worker){
// Your web worker's code goes here
}
Nowadays, almost all browsers support web workers.
Creating a Web Worker
You can create a web worker by making a new instance of Worker. You must provide the path of the script, which you want the worker to execute, as its argument.
const worker = new Worker('./worker-script.js');
In the above code, the variable worker becomes a Worker instance, that will execute the script on worker-script.js.
This web worker can now communicate with the main thread and other web workers with the help of messages. Messages can consist of anything that can be serialized and unserialized. The functions or HTML elements cannot be sent in a message. But, the messages consisting of numbers, strings, plain objects, and plain arrays can be sent.
Creating web workers will spawn real OS-level threads that will consume system resources. If not used wisely, this can affect the performance of the user’s whole computer, not just the web browser.
Sending a Message From Main Thread To Worker Thread
Once you have created the web worker, you can fire it up using the postMessage() method.
worker.postMessage(message);
Refer to the following code snippet to learn how you can implement the above into your project.
You can capture this message in the web worker by implementing an onmessage() event handler. Refer to the following code snippet to learn how you can handle the message.
Sending a Message From Worker Thread To Main Thread
You can send a message from a web worker by calling the postMessage() method inside the worker script. Refer to the following code snippet to learn how you can send a response or message back to the main thread.
Back in the main thread, you can bind the onmessage() method to the worker object to listen to the message sent back from the web worker. Refer to the following code snippet to learn how you can listen to the worker’s response.
When a message gets transmitted between the main thread and web worker, it is only copied or transferred (moved), not shared.
Terminating a Web Worker
You can terminate a web worker from the main thread directly or from the worker thread. From the main thread, you can terminate by calling the method terminate() of the web worker’s API.
worker.terminate();
You can also terminate a web worker from the worker thread itself using its close() method.
close();
When the terminate() is triggered, the web worker gets terminated instantly without any opportunity of completing the ongoing or pending operations. Also, it gets no time to clean up. Thus, terminating a web worker suddenly can lead to memory leaks. Similarly, When the close() is triggered inside a web worker, any queued tasks present in the event loop gets rejected and the web worker’s scope gets closed.
It is recommended to use web workers responsibly, and stop them when they have completed executing their tasks. This helps to free up system resources for other applications on the client’s computer.
Kudos! You’ve learned the basics of how to create a web worker, how to efficiently send messages between two threads, and how to respond to those messages. I would be glad to hear how you are using these web workers in your own projects. Let me know in the comment section.
If you enjoyed reading this post and have learnt something new, then please give a clap, share it with your friends, and follow me to get updates for my upcoming posts. You can connect with me on LinkedIn.
Boost Your Web Application Performance With JavaScript Web Workers was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Tara Prasad Routray
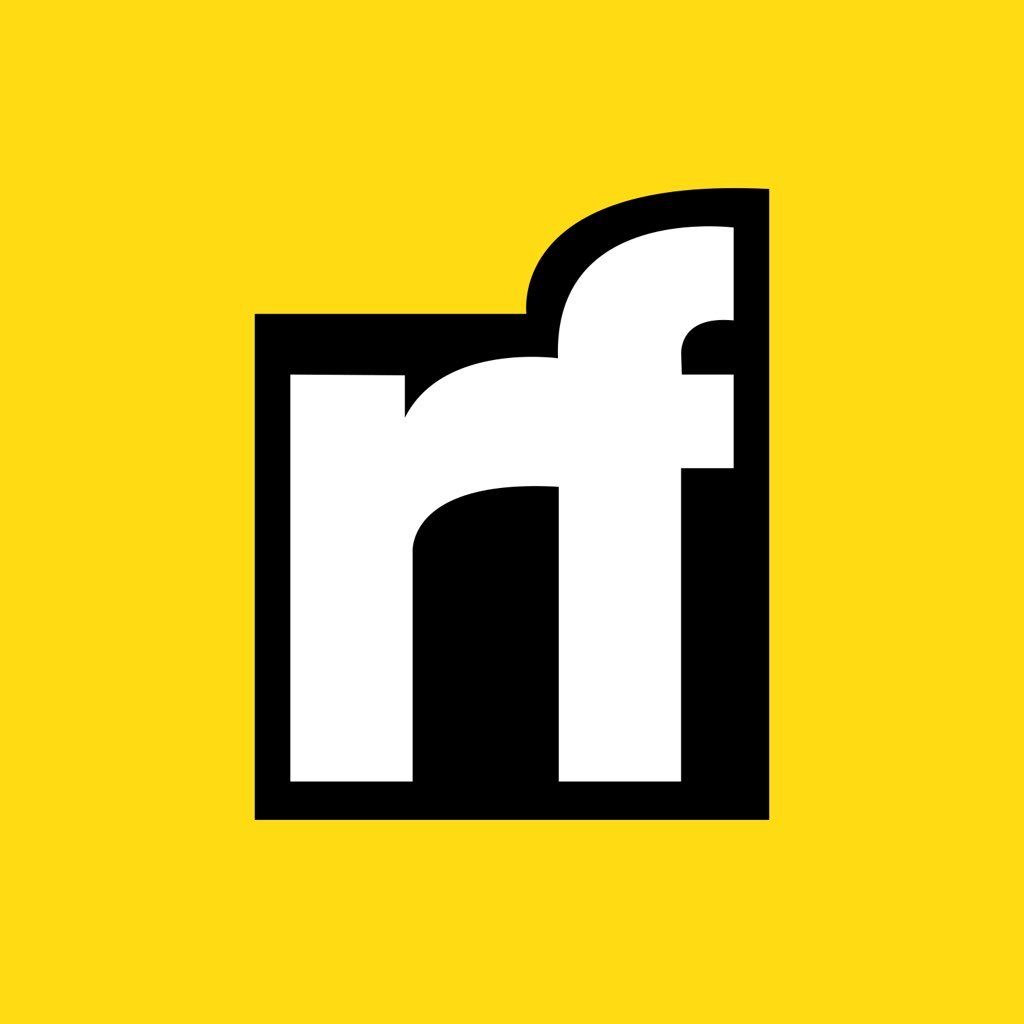
Tara Prasad Routray | Sciencx (2021-10-26T14:05:39+00:00) Boost Your Web Application Performance With JavaScript Web Workers. Retrieved from https://www.scien.cx/2021/10/26/boost-your-web-application-performance-with-javascript-web-workers/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.