This content originally appeared on DEV Community and was authored by Carlo Gino Catapang
How to create a loading and error state placeholder for images?
TL;DR
Check the full code here
Long version
Creating a basic image
next/image
provides an easy way to create an image.
import Image from 'next/image'
<Image src="https://i.imgur.com/gf3TZMr.jpeg" alt="Some alt text" />
However, we still need to configure some properties to cater to our specific needs such as:
- A placeholder when loading
- An error placeholder when the image fails to load
The GIF below shows what a user will see for an image loaded using a slow internet connection.
It gives the impression that something is wrong with our app
How to handle the loading state?
Simply adding the placeholder
and blurDataURL
will do the trick.
<Image
src="https://i.imgur.com/gf3TZMr.jpeg"
placeholder="blur"
blurDataURL="/assets/image-placeholder.png"
/>
The code will yield the following result:
There's a brief delay before the placeholder is loaded
because even the placeholder image is needed to be fetch from the server.
If there's a need to make sure that there's an immediate placeholder to the image, you refer to this guide on how to create a dynamic image placeholder
The good thing is once the placeholder image is loaded, all other images that uses the same asset
will display the placeholder immediately.
What happens if there's an error when loading the image
One possibility is that the user will stare at the placeholder for eternity
Or this sadder version which shows the alt
and a lot of empty space.
It is not fun to see too much unnecessary empty space, is it?
How to display another image during an error state?
We can simply replace the value of src
with the path to error image
in the onError
callback when an error happens.
const [src, setSrc] = React.useState('https://i.imgur.com/gf3TZMr.jpeg');
<Image
{...props}
src={src}
placeholder="blur"
blurDataURL="/assets/image-placeholder.png"
onError={() => setSrc('/assets/image-error.png')}
/>
I believe it's much better.
Putting all the code together
To make the behavior easy to replicate, we can create a custom image component.
function CustomImage({alt, ...props}) {
const [src, setSrc] = React.useState(props.src);
return (
<Image
{...props}
src={src}
alt={alt} // To fix lint warning
onError={() => setSrc('/assets/image-error.png')}
placeholder="blur"
blurDataURL="/assets/image-placeholder.png"
/>
);
}
Conclusion
When a web application displays a lot of images,
it is a good idea to give an immediate feedback to the user of what is happening. One way to address this is to use an alternate image to show the current state of an image.
This content originally appeared on DEV Community and was authored by Carlo Gino Catapang
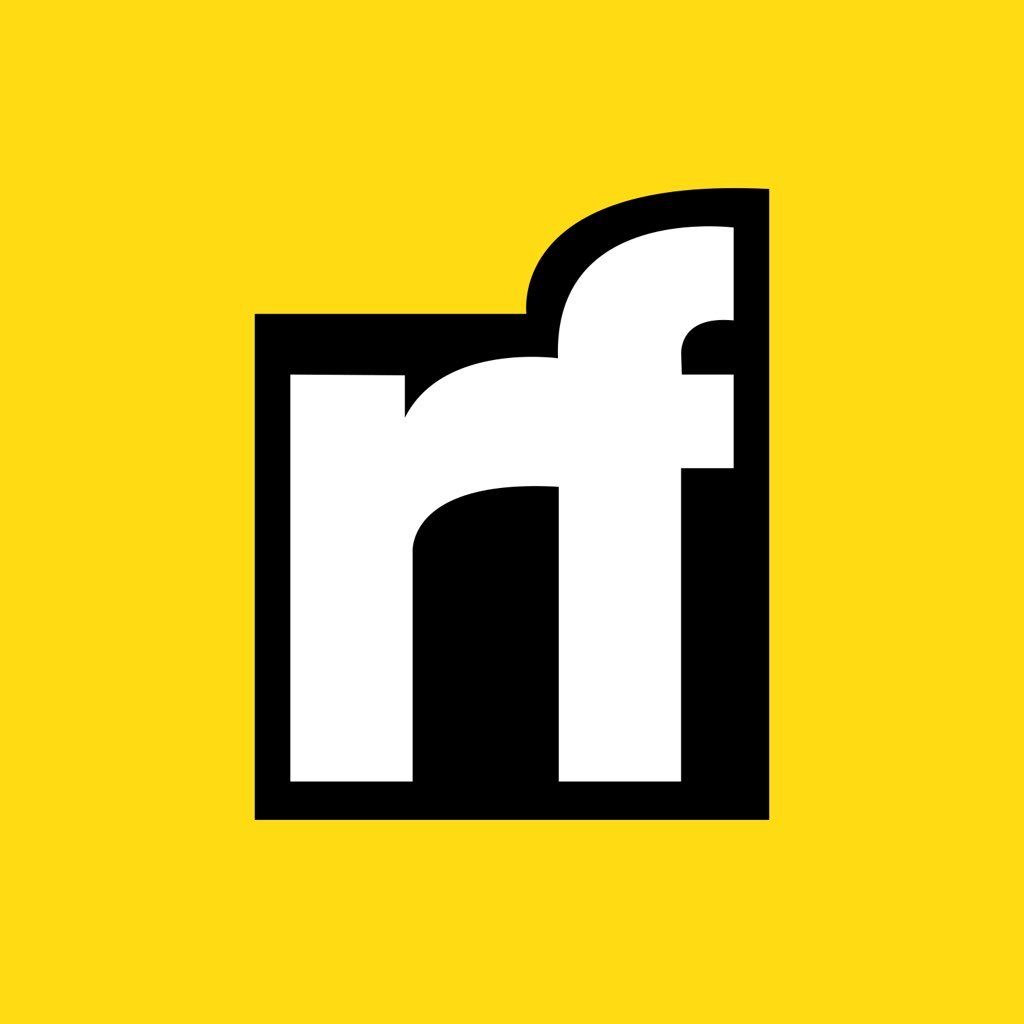
Carlo Gino Catapang | Sciencx (2021-11-17T10:16:58+00:00) Handling image loading and error state in Next.js. Retrieved from https://www.scien.cx/2021/11/17/handling-image-loading-and-error-state-in-next-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.