This content originally appeared on DEV Community and was authored by Melvin Carvalho
I've decided to download my dev.to posts so that I can store them in git. That means that if the site ever goes down, I have a copy of my blog content. The API is documented here.
The following endpoint will give a list of articles: https://dev.to/api/articles?username=melvincarvalho
Replace melvincarvalho
with your own username
So I wrote a JavaScript script that will pull out my articles. It supports pagination, each page will contain 30 articles by default.
#!/usr/bin/env node
// requires
const argv = require('minimist')(process.argv.slice(2))
const $ = require('child_process').execSync
const fs = require('fs')
// data
globalThis.data = {
user: 'melvincarvalho',
api: 'https://dev.to/api/articles/',
datadir: '.'
}
// init
data.user = argv._[0] || data.user
data.api = argv.api || data.api
data.datadir = argv.datadir || data.datadir
console.log('data', data)
// main
const useruri = `${data.api}?username=${data.user}`
const cmd = `curl ${useruri}`
console.log('cmd', cmd)
const json = JSON.parse($(cmd).toString())
const output = JSON.stringify(json, null, 2)
const outfile = `${data.datadir}/posts.json`
console.log('output', output)
fs.writeFileSync(outfile, output)
First we initialize the endpoint and username. Then we run some curl to get the result, and finally we format it and write it to a file.
The JSON output can be seen here and the current script is here
Now that I have a list of articles, it should be possible to download the markdown from individual articles too. I will hopefully cover that in a future post.
This content originally appeared on DEV Community and was authored by Melvin Carvalho
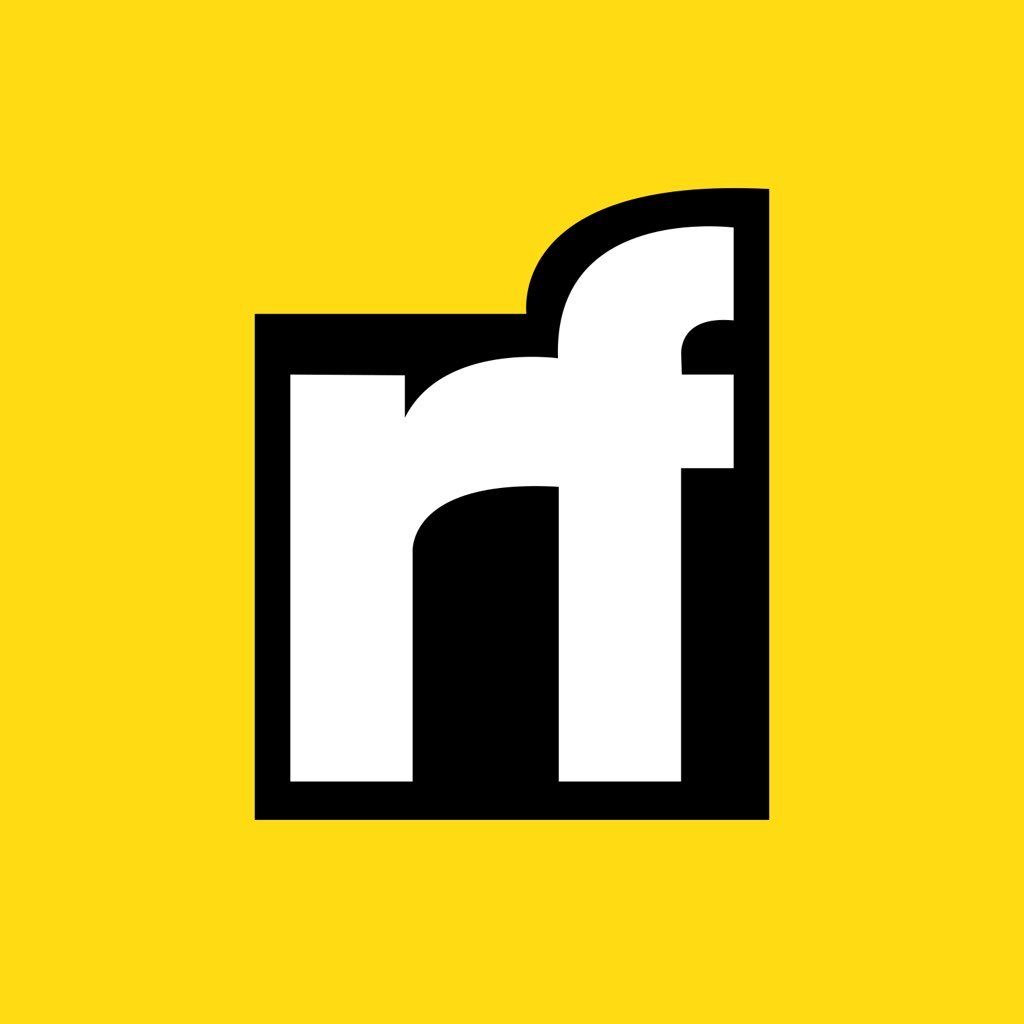
Melvin Carvalho | Sciencx (2021-11-27T11:43:17+00:00) How to get dev.to posts from the API. Retrieved from https://www.scien.cx/2021/11/27/how-to-get-dev-to-posts-from-the-api/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.