This content originally appeared on foobartel.com :: Stream and was authored by foobartel.com :: All Posts
There are a variety of use cases for when you might want to add an EventListener with addEventListener to an element to change its native behaviour. Sometimes event handlers need to get attached to single elements, other times adding to multiple elements at once can be neccessary.
Most of the time when I do need to add it to multiple elements, I can’t remember… This post serves as a little reminder for myself (and possibly you), in case I forget again, which is pretty surely going to happen. And that’s ok.
Adding an EventListener to a single element that is listening to the click event often looks like this.
document.querySelector('.button').addEventListener('click', event => {
// do something on click
});
Yet sometimes you want to add an EventListener to multiple elements. This can be helpful when you want to execute the same function on different elements, e.g. each with its own parameter, option, etc.
One way to add an Event Listener to multiple elements is by using the convenience of a for…of statement, which creates a loop to iterate over its passed elements.
let buttons = document.querySelectorAll('.button');
for(i of buttons) {
i.addEventListener('click', function() {
// do something on click
});
}
Another way to add an EventListener to multiple elements is by using a forEach() loop.
With querySelectorAll()
we’re selecting all elements with the .button
class, then we iterate over all of these elements with forEach()
and add the EventListener (for the ‘click’ event) to each of them. The code to do so looks like this:
document.querySelectorAll('.button').forEach(item => {
item.addEventListener('click', event => {
// do something on click
});
});
For situations where elements do not have the same class name, but require the same EventListener, it’s possible to create an array to select all elements.
[document.querySelector('.button'), document.querySelector('.other-element')].forEach(item => {
item.addEventListener('click', event => {
// do something on click
});
});
By now and with the above examples, you (and my future self) should have a good idea on the different possibilities of how to use addEventListener on multiple elements. With that, go build something and have a nice day!
Please reach out if you have any comments or further improvements to the above snippets.
This content originally appeared on foobartel.com :: Stream and was authored by foobartel.com :: All Posts
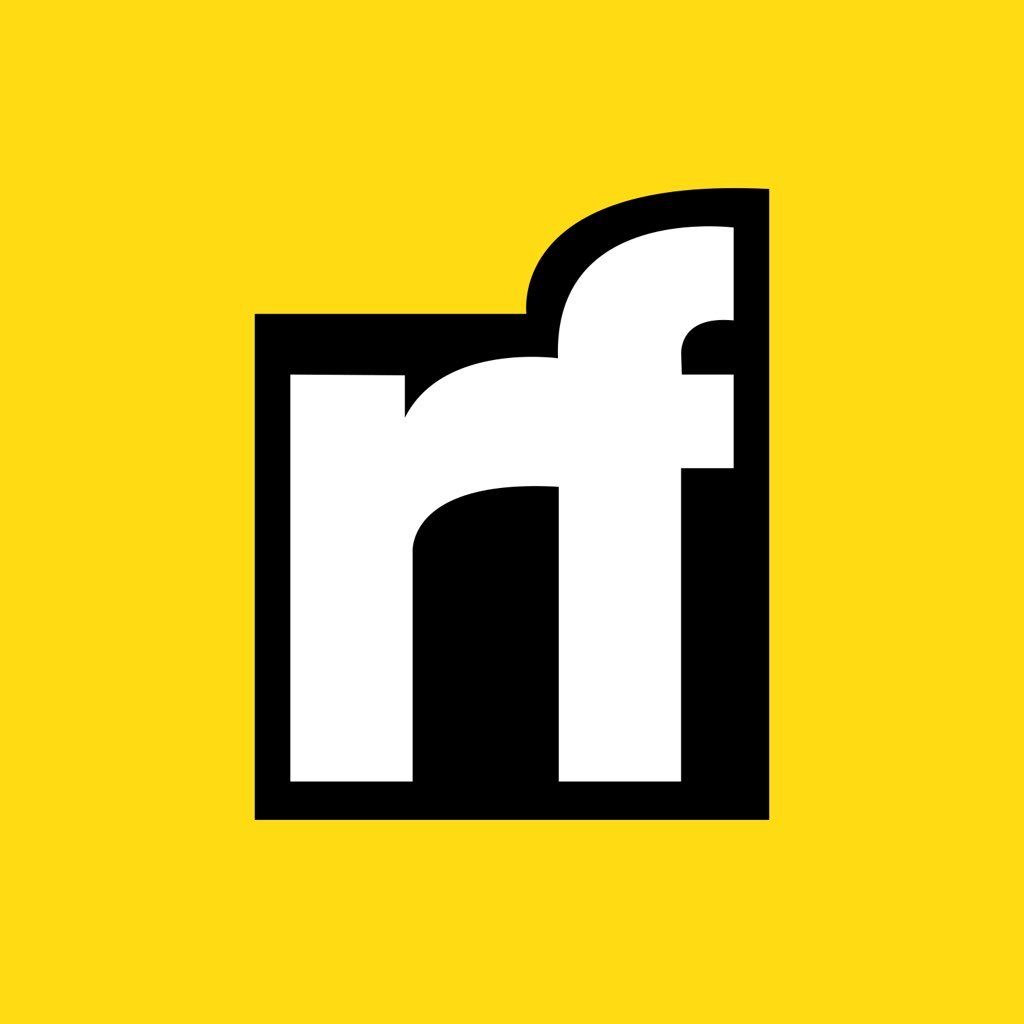
foobartel.com :: All Posts | Sciencx (2021-12-02T23:00:00+00:00) Adding a JavaScript Event Handler (with addEventListener) to Multiple Elements. Retrieved from https://www.scien.cx/2021/12/02/adding-a-javascript-event-handler-with-addeventlistener-to-multiple-elements/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.