This content originally appeared on DEV Community and was authored by Nelson Adonis Hernandez
In this publication we will see the main methods to use with Azure Blob Storage
pip install azure-storage-blob
Blob Service Client
from os import getenv
from azure.storage.blob import BlobServiceClient
blob_service_client = BlobServiceClient.from_connection_string(
getenv("AZURE_STORAGE_CONNECTION_STRING"))
Methods for blobs (Files)
Upload Blob
def upload_blob(filename: str, container: str, data: BinaryIO):
try:
blob_client = blob_service_client.get_blob_client(
container=container, blob=filename)
blob_client.upload_blob(data)
print("success")
except Exception as e:
print(e.message)
Download Blob
def download_blob(filename: str, container: str):
try:
blob_client = blob_service_client.get_blob_client(
container=container, blob=filename)
print(blob_client.download_blob().readall())
except Exception as e:
print(e.message)
Delete Blob
def delete_blob(filename: str, container: str):
try:
blob_client = blob_service_client.get_blob_client(
container=container, blob=filename)
blob_client.delete_blob()
print("success")
except Exception as e:
print(e.message)
Methods for Containers (Folders)
Create Container
def create_container(container: str):
try:
blob_service_client.create_container(container)
print("success")
except Exception as e:
print(e.message)
Delete Container
def delete_container(container: str):
try:
blob_service_client.delete_container(container)
print("success")
except Exception as e:
print(e.message)
List Containers
def get_containers():
try:
containers = blob_service_client.list_containers()
print("success")
except Exception as e:
print(e.message)
Github Gist
https://gist.github.com/nelsoncode019/eade5071c80f659bfa7ce9a452345d85
This content originally appeared on DEV Community and was authored by Nelson Adonis Hernandez
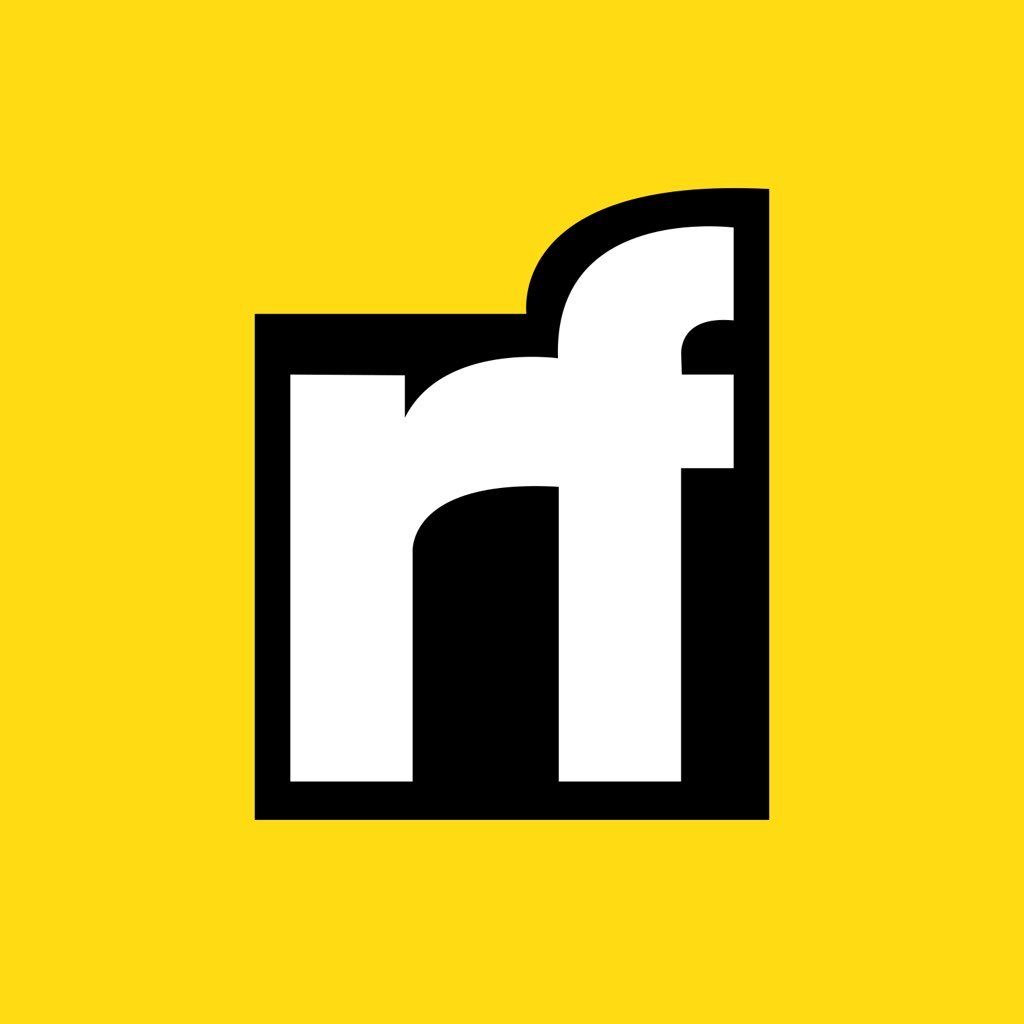
Nelson Adonis Hernandez | Sciencx (2021-12-06T19:31:43+00:00) How to use Azure Blob Storage with Python. Retrieved from https://www.scien.cx/2021/12/06/how-to-use-azure-blob-storage-with-python/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.