This content originally appeared on Bits and Pieces - Medium and was authored by Simon Ugorji (Octagon)
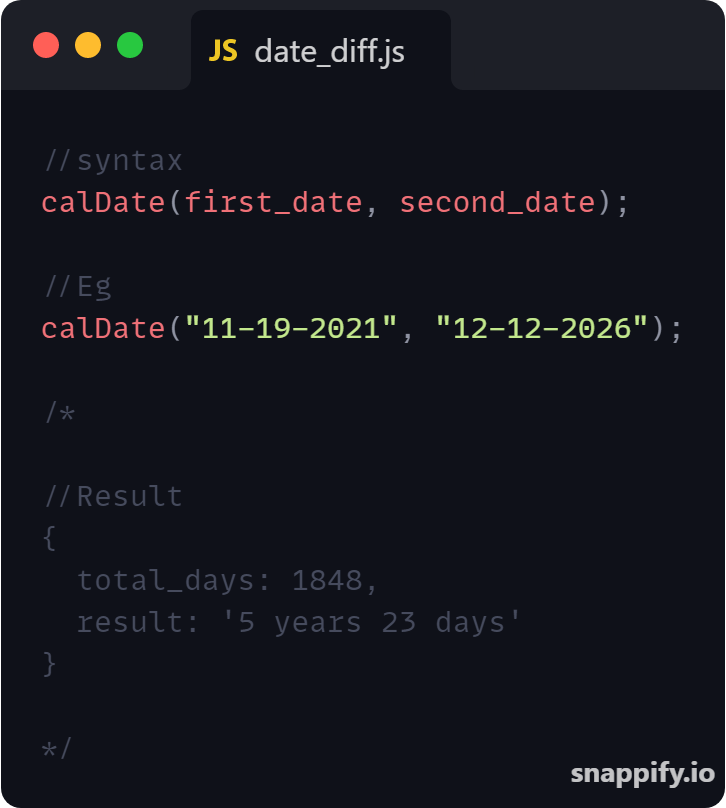
Let’s suppose that you have two dates in mind, and you wish to calculate the difference between them. There are many instances where you might need this functionality, such as calculating how many days it may take for a delivery to arrive, or maybe you want to build your own time calculator like this Amazing App.
Let’s demonstrate how you can create this functionality.
Let’s begin
We will call our Function calDate() short for ‘calculateDate’. Then we will accept two parameters which are date1 and date2 in the format Month-Day-Year:
We then initiate a new Date object and find the timestamps of both dates:
Next, we have to prevent negative values when we subtract a higher timestamp from a lower timestamp because this can ruin our result.
We will use a condition to check which timestamp is greater and then swap them, as we can see on line 17 below:
We have also passed the result of subtracting timestamp 2 from timestamp 1 to a new date object in order for JavaScript to convert the resulting timestamp to a date.
Next, we have to retrieve the days, months, and years from our result and then join them with a hyphen. This will enable us to convert it to an array that we will read from later.
If you observe the code above, you’ll notice that we added +1 to the getMonth() method.
This is because JavaScript counts months using a zero index, from 0–11, so 0 for January, 1 for February, up to 11 for December.
Now it’s time to read from our array
Our array was created in this format:
- At position 0 is the Day
- At position 1 is the Month
- At position 2 is the Year
We will subtract each member of our array from the default date which is (01–01 -1970). This will enable us to know the number of days, months, and years that have elapsed.
Let’s set up our custom text to prevent errors in counting such as; 1 years ago or 1 months ago.
Now if you search for how many days there are in 6 months, you would get the formula that we will use to get the total number of days between our two dates.
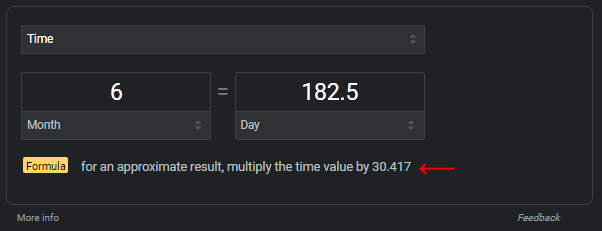
Let’s find the total number of days between the two dates by converting the years and months to days, then we will sum the result with the already existing days from our calculation.
Improving our custom text
We will check if the years that have passed (years_passed) is equal to 1, then we will show our custom text for years (ysrTxt) at the position 0 (year) ie (1 year). But if it is greater than 1, we will show our custom text at position 1 but if it is 0, we will not show any value.
Note: I used a Tenary Operator to simplify our logic.
Let’s round off the total days to the nearest integer because there is a 95% possibility that our result will end up as a float data type. We will use the method Math.round() to achieve this, and then we will return our result as an object.
Testing our code
I will use the dates below:
- date 1 = today (m-d-y) = 11–19–2021
- date 2 = future (m-d-y) = 12–12–2026
This is the output:

Now if you use an external App like time and date for the same calculation, you should get the same value.
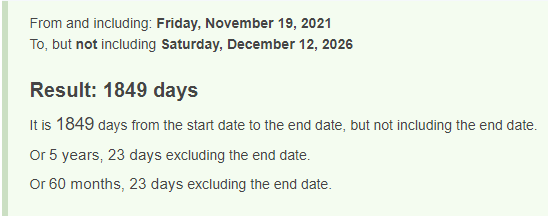
Conclusion
So that’s the basic setup for our ‘calculate difference between two dates’ function. From here you can choose to extend the script to show the total number of seconds, total number of weeks, total number of hours, total number of months, etc.
You can find the PHP version here.
Build Micro Frontends with components
Microfrontends are a great way to speed up and scale app development, with independent deployments, decoupled codebases, and autonomous teams.
Bit offers a great developer experience for building component-driven Micro frontends. Build components, collaborate, and compose applications that scale. Our GitHub has over 14.5k stars!
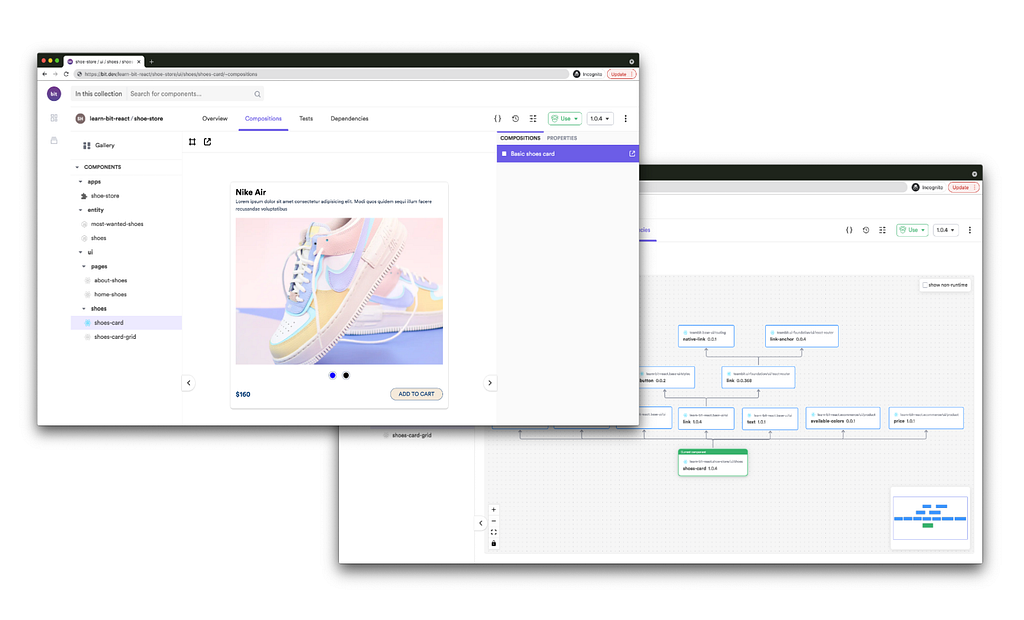
Learn more
- Building a React Component Library — The Right Way
- Microservices are Dead — Long Live Miniservices
- 7 Tools for Faster Frontend Development in 2022
Calculate the Difference Between Two Dates in JavaScript was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Simon Ugorji (Octagon)
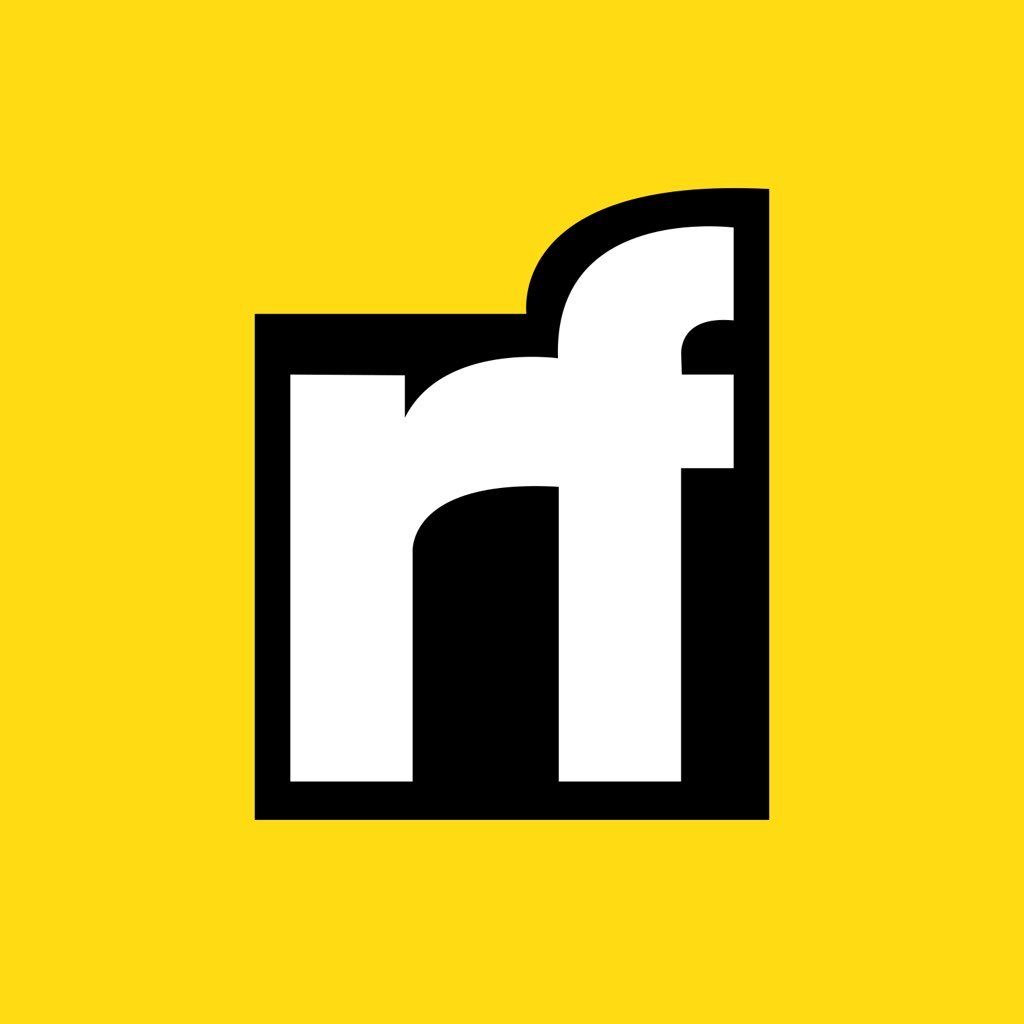
Simon Ugorji (Octagon) | Sciencx (2021-12-29T09:05:10+00:00) Calculate the Difference Between Two Dates in JavaScript. Retrieved from https://www.scien.cx/2021/12/29/calculate-the-difference-between-two-dates-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.