This content originally appeared on DEV Community and was authored by Bellotaiwo
Quick Summary: in this article, we are going to build a reusable react tree component
A react tree view component is a graphical user interface component that represents a hierarchical view of information, where each item can have several subitems. They are often seen in the sidebar, file manager applications.
Let's start by creating the tree data. The treeData
is an array of node
objects.
Each node object has the following fields.
- key
- label
- children: The children field is an array of a
node
object.
const treeData = [
{
key: "0",
label: "Documents",
children: [
{
key: "0-0",
label: "Document 1-1",
children: [
{
key: "0-1-1",
label: "Document-0-1.doc",
},
{
key: "0-1-2",
label: "Document-0-2.doc",
},
],
},
],
},
{
key: "1",
label: "Desktop",
children: [
{
key: "1-0",
label: "document1.doc",
},
{
key: "0-0",
label: "documennt-2.doc",
},
],
},
{
key: "2",
label: "Downloads",
children: [],
},
];
function App() {
return (
<div className="App">
<h1>React Tree View</h1>
<Tree treeData={treeData} />
</div>
);
}
We then proceed to create a Tree
component that takes the treeData
as a prop.
The Tree
component maps through the treeData
and returns a TreeNode
component that takes the tree node
as a prop.
function Tree({ treeData }) {
return (
<ul>
{treeData.map((node) => (
<TreeNode node={node} key={node.key} />
))}
</ul>
);
}
The TreeNode
component incorporates the following.
- A
showChildren
state is the state responsible for displaying theTree
component. - A
handleClick
is a click handler function that toggles theshowChildren
state.
The TreeNode
component displays the node label
The TreeNode
component also conditionally renders the Tree
component if the showChildren
state is true and passes the node children
to the Tree
component as a prop.
function TreeNode({ node }) {
const { children, label } = node;
const [showChildren, setShowChildren] = useState(false);
const handleClick = () => {
setShowChildren(!showChildren);
};
return (
<>
<div onClick={handleClick} style={{ marginBottom: "10px" }}>
<span>{label}</span>
</div>
<ul style={{ paddingLeft: "10px", borderLeft: "1px solid black" }}>
{showChildren && <Tree treeData={children} />}
</ul>
</>
);
}
This would be the result.
This content originally appeared on DEV Community and was authored by Bellotaiwo
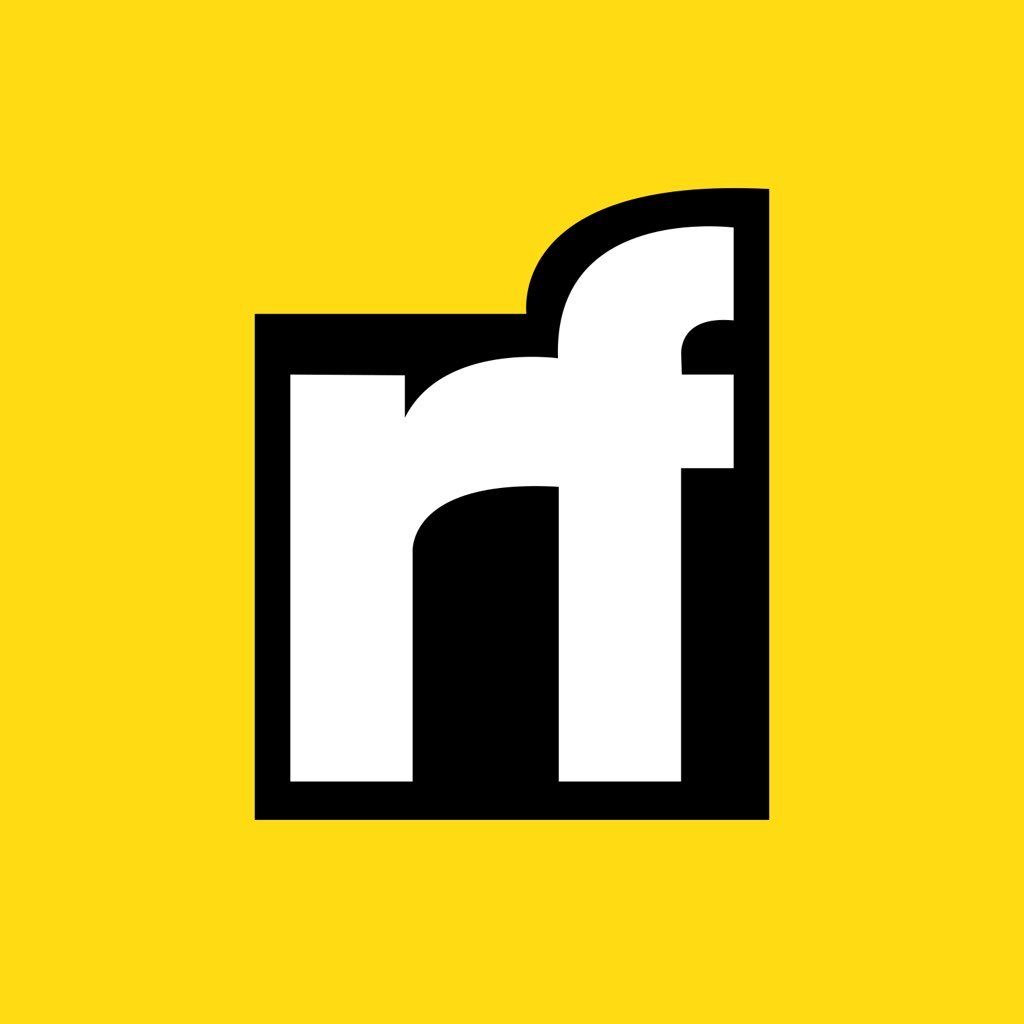
Bellotaiwo | Sciencx (2022-01-03T12:50:41+00:00) How to create a React tree view component.. Retrieved from https://www.scien.cx/2022/01/03/how-to-create-a-react-tree-view-component/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.