This content originally appeared on Level Up Coding - Medium and was authored by Jacques Sham
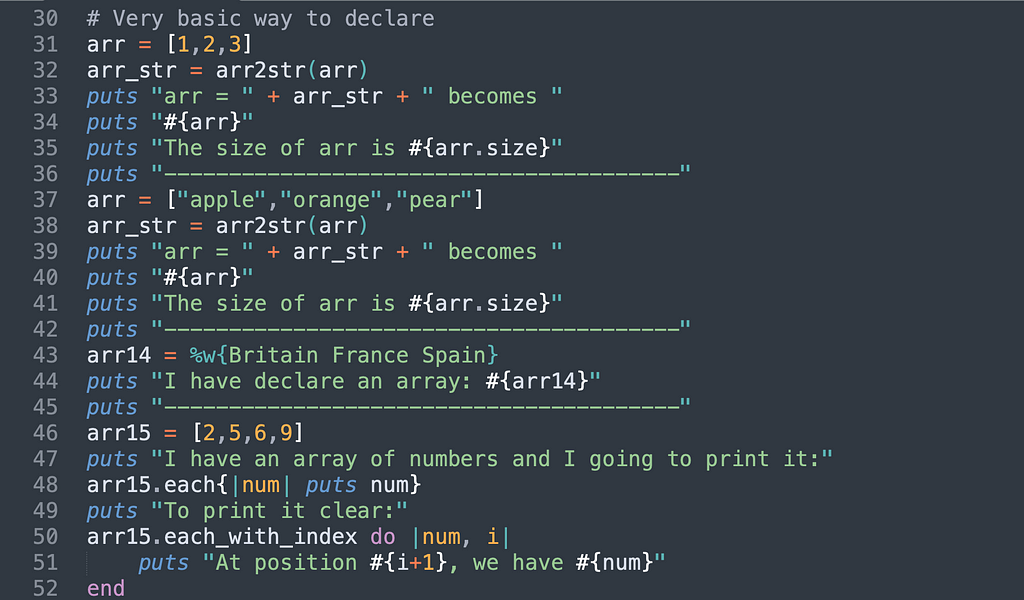
After I started working in my current company, I realized I need to learn to program in Ruby. Since I have been using Python since college, learning a new programming language was not too hard for me. It is not surprised the Ruby syntax and style are different from Python, but I would like to give you some tips if you want to learn Ruby and have a Python background to speed up your learning curve!
Understand Double Quotation for Strings
Pasting a variable in a string in Ruby is extremely easy as you would paste “#{variable}” in the string, similar to f string in Python. For example, if you have a variable age = 10, I can put the below code to print a person’s age in a string.
However, I was first confused why it works sometimes but sometimes I get an error. Then, I found out you “#{variable}” only works on a string with double quotations. As a new Ruby programmer, I did not know what are the differences between a string using single and double quotations. Apparently, you can only paste “#{}” and escape sequence in the double quotation and either syntax will throw an error if it is pasted in a single-quoted string.
# To print a person's age
age = 10
puts “The person’s age is #{age}”
The best practice is always to define a string with single quotations unless you need to paste either “#{}” or escape sequence.
Getting Used to Ruby Syntax — Especially Nil
Try to forget the Python style syntax for a moment when you coding in Ruby! In the first script, my head is always thinking if variable is None when coding in Ruby. Null has its own keyword in many programming languages, its syntax in Ruby is nil, and use .nil? to testify whether a variable is null/
Indentation is replaced with “end”
Indentation is a Python thing! There is no such rule in Ruby, you use the keyword end to close a function. It is similar to the brackets used in Java. Besides being absent-minded after the weekend, a common reason I forget to put the keyword end after a function is that there are too many functions that required me to put place an end to close a function. A good habit I got from coding Java is to put a comment after a closed bracket to indicate what function am I closing, and I am carrying this good habit to Ruby to put a comment of end func or end if.
def some_func(num1, num2)
if num1 > num2
then puts "#{num1} is greater than #{num2}"
else
puts 'num1 is a small number!'
end # end if
end # end func
Putting a comment to close a function helps you a lot with debugging! Although indentation is not a rule in Ruby, you should still try your best to intend your code to be more readable. This Python thing is not required but it is definitely a good habit and gives you an easier time while debugging your script.
Boolean is not a Data Type
A surprising fact is True and False are two different data types in Ruby! What it means is that Boolean does not exist in Ruby as a data type. True itself is one data type; false itself is another data type. Negating true or false in Ruby is the same as converting a variable from one type to another type.
Another unique syntax related to true or false in Ruby is the unless statement, which is a negation of an if statement. This is very helpful if you want to testify whether some condition is true or false.
# Let's pretend we want to print if num is not 0
unless num == 0
then puts num
end # end unless
Using the unless statement is not as straightforward as a new Rubyist because it involves a lot of “negation thinking” in your head, don’t feel bad if you are confused in the first place!
Speaking of the unless statement, if you want to testify whether a variable is not null, you should have a habit to use the unless statement to testify, like below:
# Python way
if var is not None:
print('Variable is null')
# Ruby way
unless var.nil?
then puts 'Variable is null'
end # end unless
Ruby style instead of Python habit
Although Ruby has adopted the Python style syntax to iterate elements in an array (for i in some_array style), it is best to get used to the Ruby style of the each() function.
# some_array is an array with numbers
# This is the Python style
for i in some_array do
puts i
end # end for
# It works but not very Rubyist
# Try to write this instead
some_array.each{|i| puts i}
One of the reasons I try to avoid using the each function in the beginning because I thought each function can only do one operation for each iteration as there is no room in the bracket to put more than one operation. Not until a couple of months later, I realized you can do multiple operations with a slight syntax modification! Simply replace the bracket with the “do” keyword and end the each function with “end”. It is especially usefully when you need to put an if statement:
# If you have multiple operations within each function
some_array.each do |i|
if i>=0
then puts i
else
puts "#{i}" is a negative number
end # end if
end # end each
If you adopt the Ruby style syntax, not only your code is more authentic as a Rubyist, but also it helps you understand and get used to using the pipe for arguments in other functionalities. Another common task you may do is to save data in a CSV file with the CSV gem, which uses the pipe to accept arguments to open a file and write data into it.
CSV.open(filename,"w") do |csv_file|
some_array.each{|i| csv_file << i}
end # Close csv
Know what is Symbol
Symbol major confusion to many new Rubyists like me, especially when they were learning how to write a class in Ruby. Writing a class in Ruby is very convenient because you can an attribute assessor to function as a combination of “getter” and “setter” functions to obtain or set an attribute with a class object:
class person
def initialize(age) # Constructor
@age = age
end
# Setter + Getter function
attr_accessor :age
end
However, I was extremely confused when the syntax of colon and attribute and mistakenly thought it is a special type of variable. It turns out a symbol in Ruby is an immutable string to allow multiple references without taking extra memory to improve efficiency. It is not a variable nor a string, but a special Ruby instance to keep in mind to use in writing class for Ruby beginners.
My other use case of symbols is using Sequel, a Ruby gem to query from a relational database. When querying with Sequel, it requires column names in symbols to execute. An example is when we have a table of stock prices and we want to have Apple stock price in chronological order:
# This is same as select * from fund_price where ticker='AAPL' order by pricedate
result = ds.select(:ticker, :pricedate, :price).order(:pricedate).where(ticker: 'AAPL').all
In case you have a list of column names in string, use .to_sym to convert the strings to symbols.
Conclusion
Learning Ruby is fun for a Pythonist and it doesn’t take too much time to learn if you have knew Python. But try not to code Ruby in a Python mindset like coding for i in some_array, try to practice coding Ruby with its native syntax like using the each function!
Jacques Sham - Data Engineer - GoodData | LinkedIn
Tips to Learn Ruby with a Python Background was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Jacques Sham
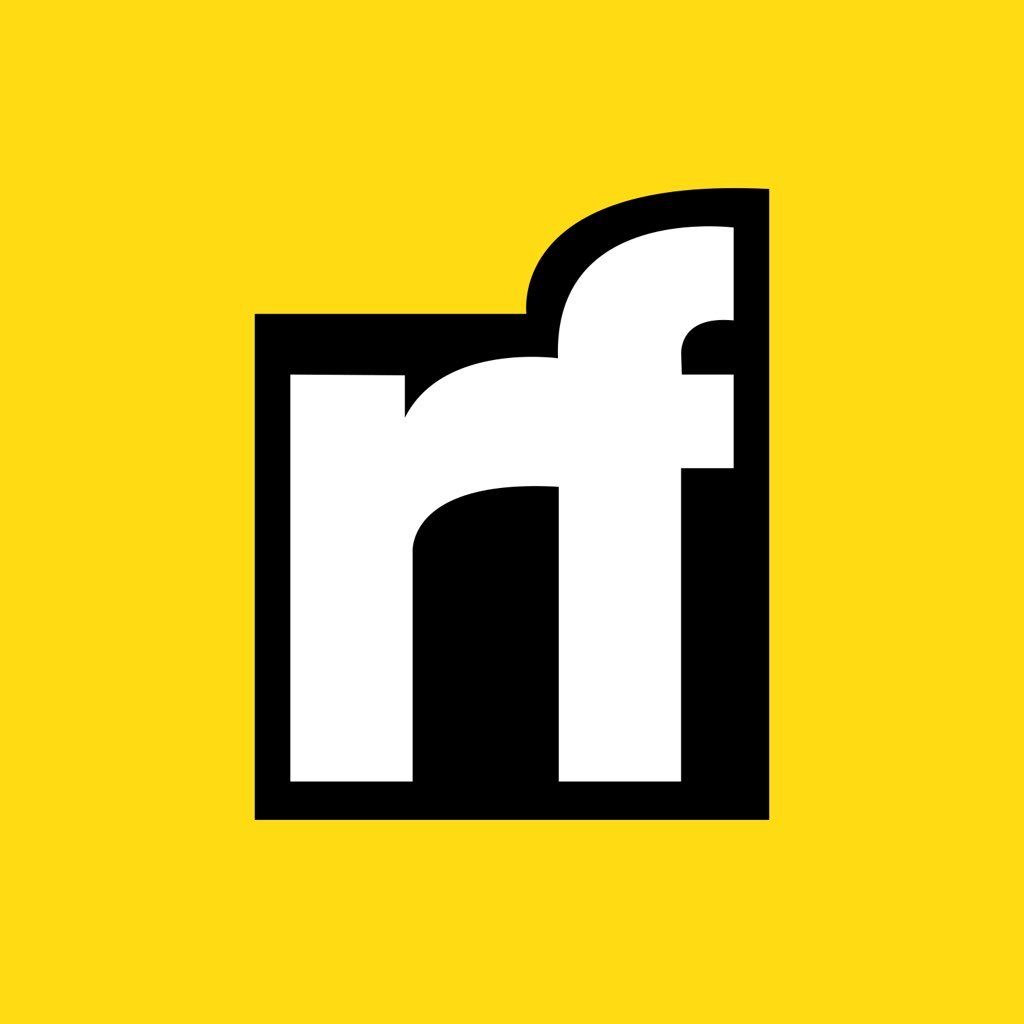
Jacques Sham | Sciencx (2022-01-10T12:52:01+00:00) Tips to Learn Ruby with a Python Background. Retrieved from https://www.scien.cx/2022/01/10/tips-to-learn-ruby-with-a-python-background/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.