This content originally appeared on DEV Community and was authored by Aleksei Berezkin
So you have some large amount of work to be done. Perhaps you prepare some heavy API answer, or parse a large document, or compute vertices for your 3d scene. Something like this:
function computeVertices() {
const vertices = []
for (let i = 0; i < 10_000_000; i++) {
vertices.push(computeVertex(i))
}
return vertices
}
This code works 200ms, the UI looks unresponsive, scrolls are jumping, and transitions are jarred — all the UX is terrible. Is there a nice way to make pauses during this work? Yes! Async generators to the rescue.
That's how it looks:
async function computeVertices() {
const workLimiter = createWorkLimiter()
const vertices = []
for (let i = 0; i < 10_000_000; i++) {
await workLimiter.next()
vertices.push(computeVertex(i))
}
return vertices
}
And here's implementation:
async function* createWorkLimiter(
work = 10,
pause = 6,
) {
let start = Date.now()
for ( ; ; ) {
yield
if (Date.now() >= start + work) {
await delay(pause)
startMs = Date.now()
}
}
}
function delay(ms) {
return new Promise(resolve =>
setTimeout(resolve, ms)
)
}
Cool, isn't it?
This content originally appeared on DEV Community and was authored by Aleksei Berezkin
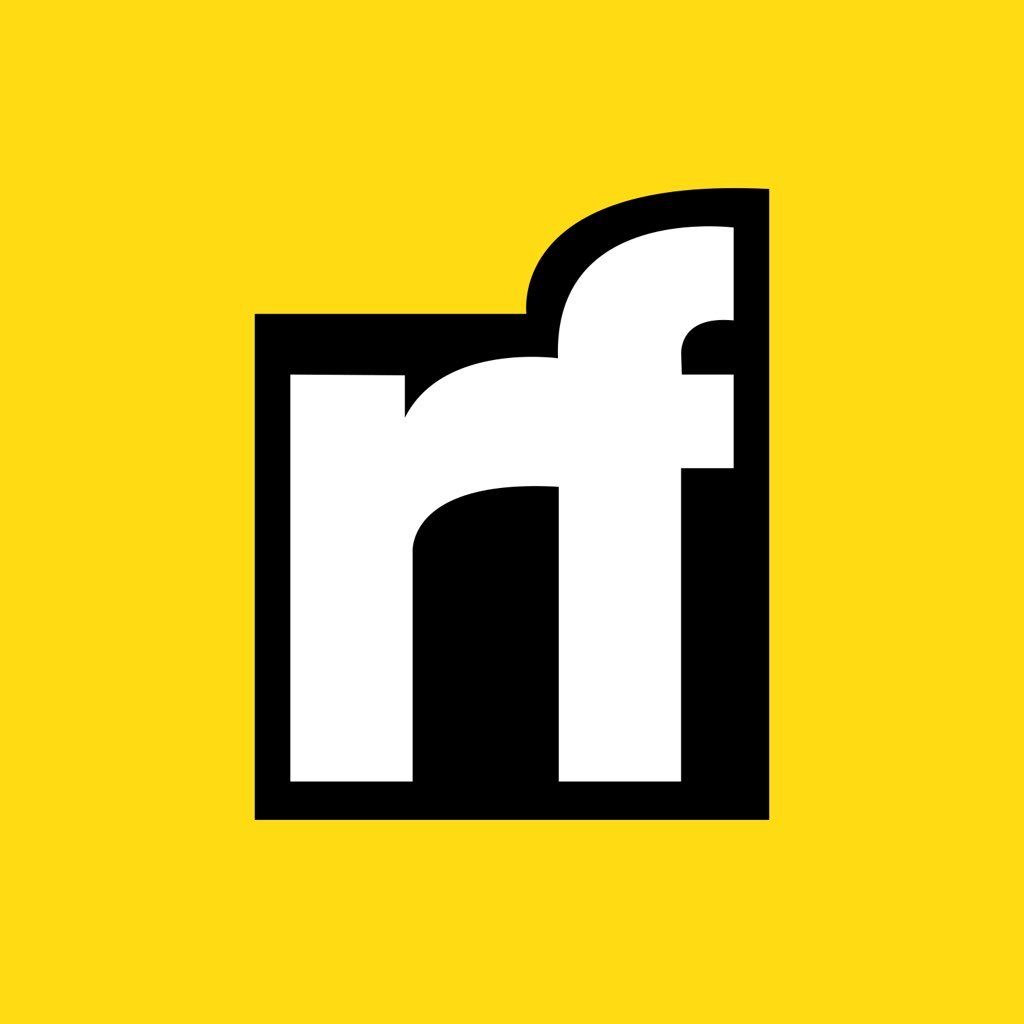
Aleksei Berezkin | Sciencx (2022-02-03T16:45:06+00:00) How to split up CPU-intensive work with async generators. Retrieved from https://www.scien.cx/2022/02/03/how-to-split-up-cpu-intensive-work-with-async-generators/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.