This content originally appeared on DEV Community and was authored by DEV Community
What is Typescript?
Typescript is a superset of the JavaScript language. The purpose is to give JavaScript a flavor of strongly typed language so that our code errors are easily detected and we are protected from many unwanted behaviors in the application. Typescript cannot be written in a browser like JavaScript.
Globally typescript installation:
yarn global add typescript
or
npm install typescript -g
Installation typescript in local project:
yarn add typescript
or
npm install typescript
Initialization typescript in project
tsc --init
How to compile a specific file?
tsc app.ts
How to compile a specific file with watch mood?
tsc app.ts -w
Compilation of all files and also with watch mood:
tsc
tsc -w
Basic data types:
- Any
- number
- string
- boolean
- object
- Array
- Tuple
- Enum
- undefined
- null, void
- never
- unknown
_Let's go learn about function parameter and return type
_
The most interesting thing in typescript is that we can declare the type of our parameter that's why we can handle any error easily.
function add (n1: number, n2: number) => number;
function add (n1: number, n2: number): number;
When we fetch data from API we have to use an interface.
Example:
interface Person {
name: string;
age: number;
height: string;
}
const person1: Partial<Person> = {
name: 'Hridoy',
age: 28
}
That's the basic part of typescript.
This content originally appeared on DEV Community and was authored by DEV Community
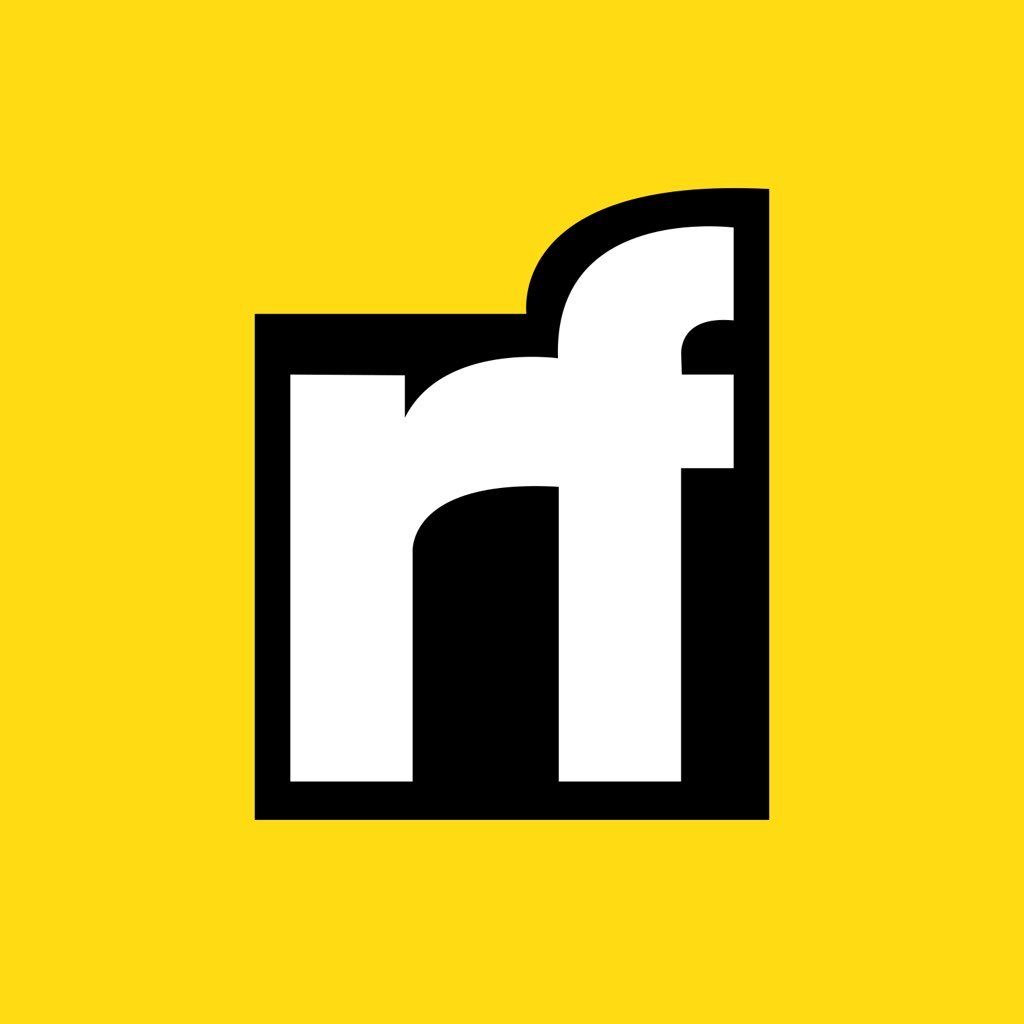
DEV Community | Sciencx (2022-02-25T06:57:43+00:00) Typescript with React. Retrieved from https://www.scien.cx/2022/02/25/typescript-with-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.