This content originally appeared on Level Up Coding - Medium and was authored by Davide Francesco Merico
Hello everyone, this time I want to share with you and get feedbacks about an approach I’ve used to handle API requests into a React project.
The context is a React project, written with Typescript, where we have a store handled using Redux Toolkit and API requests managed by Axios. Depending from the current component we have to do different actions based on the data retrieved as well as defining a common interface to access to the data exchanged.
If you want a more “bundled” solution, Redux Toolkit already provides the RTK Query utility.
Good, now we can start coding. We know that each request could be in four states:
- IDLE: The request was not sent so we have no data and no errors
- PENDING: The request was sent but we have no data and no errors yet.
- FULFILLED: The request was sent and we have received data.
- REJECTED: The request was sent but something goes wrong.
Next step is to know how to handle errors if the request was rejected. Maybe we can have a message and a code to identify the error type.
With these two steps we have a generic structure to handle all our requests:
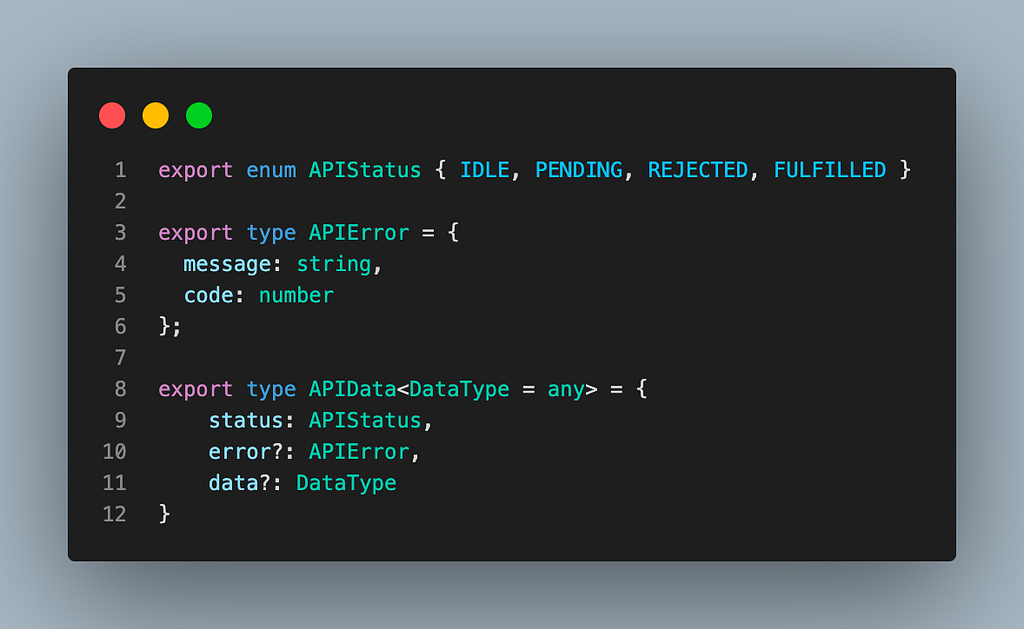
Now we have to setup at least an instance of axios, using interceptors, to handle the data properly. For this example we are going to create a generic instance without custom headers or weird logics. This is useful if there is a defined response ( like the APIError type ) returned from the server if a request fails.
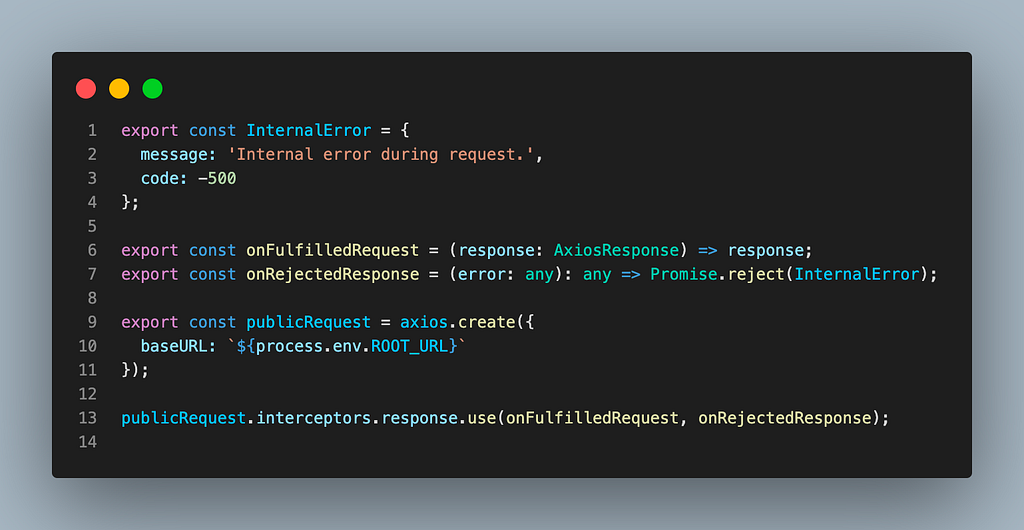
The InternalError was introduced if a request fails without any valid body.
At this point we can build the redux part to handle the request and its response. So we have to define the state, the reducer and the action:
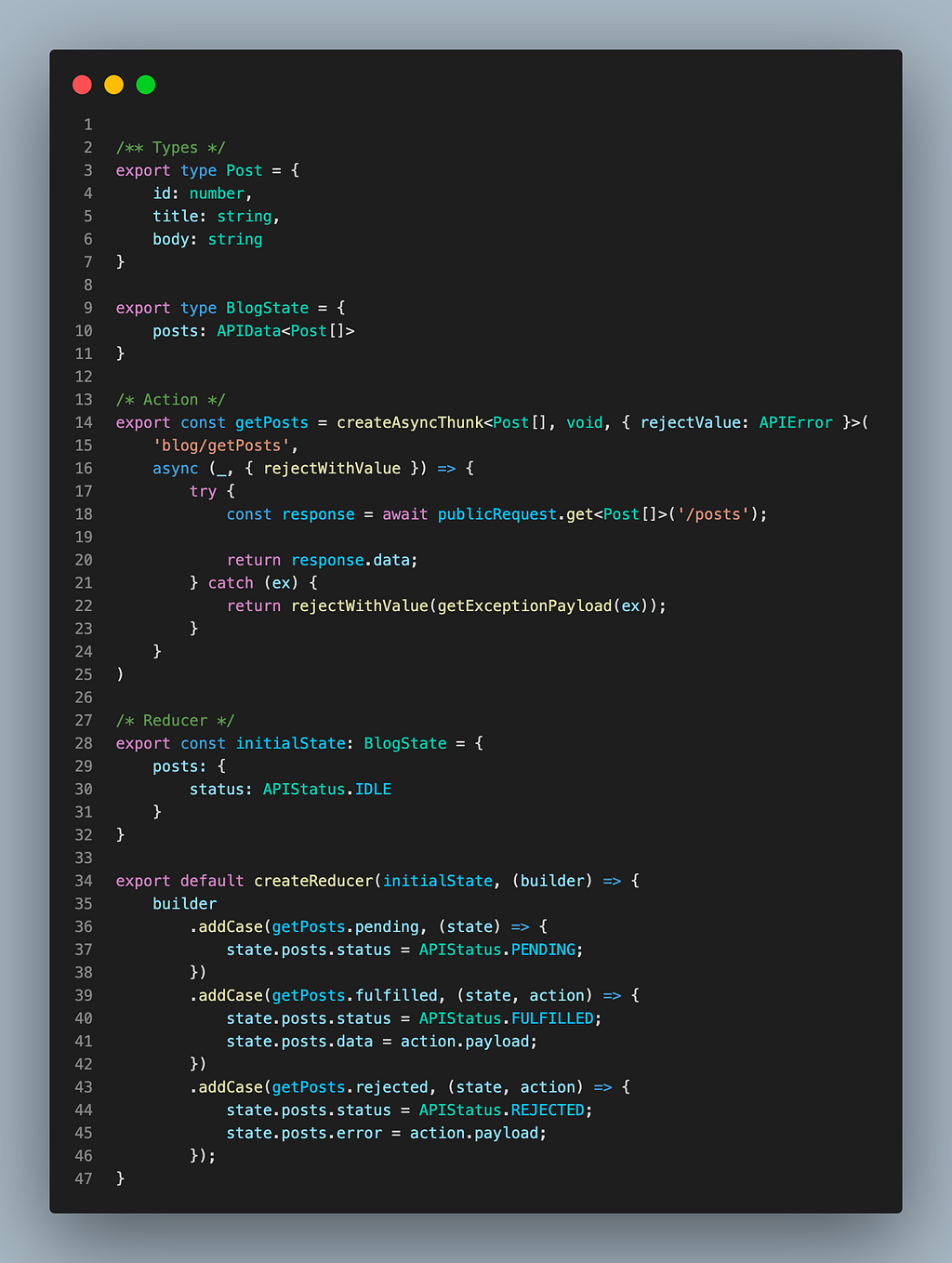
The function getExceptionPayload transforms the exception to an APIError type. If the exception was thrown by axios we can read the API response, otherwise, if the exception occurred due to our logic, we can set a custom error.
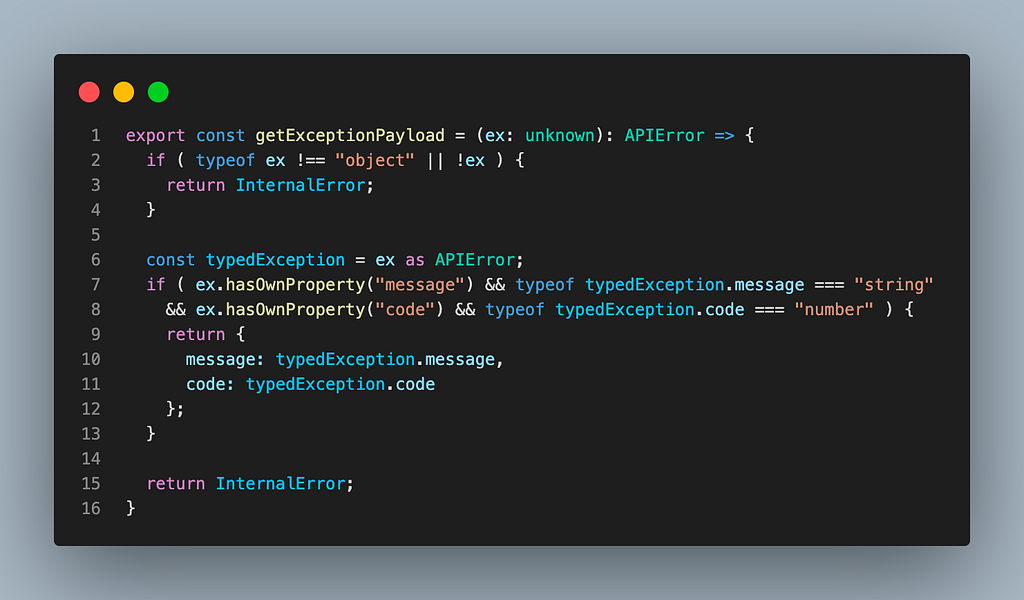
Now there are all the tools to dispatch an action, handle the result and spread the data over all components connected to the store. But.. how manage it inside a component?
The first method could be to handle the data inside the useEffect hook ( all at once or one hook for each state where there are logic to execute )
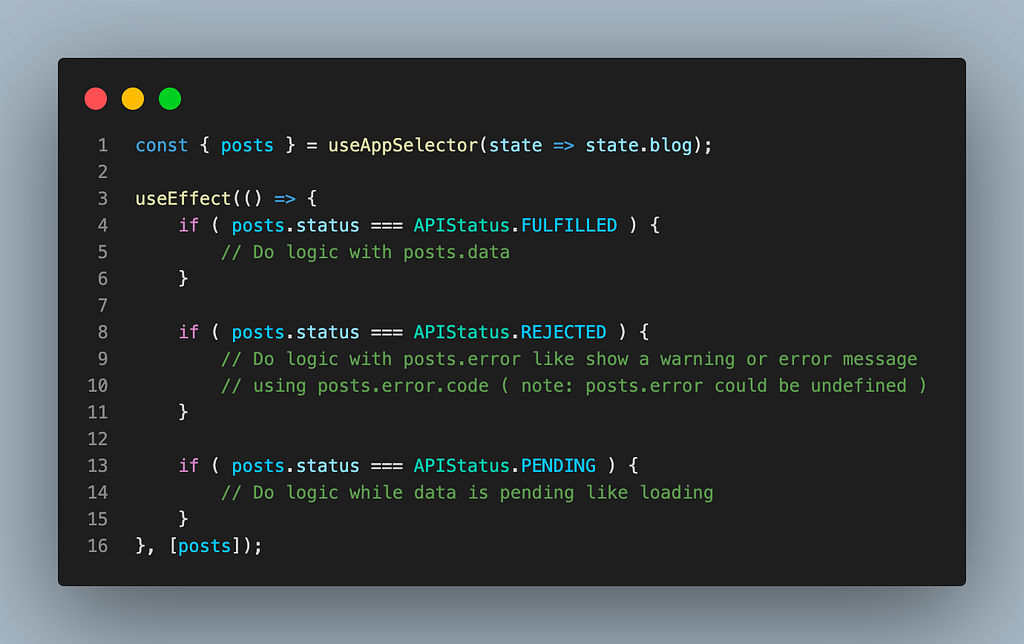
The second method is to create a custom hook to reduce the code complexity to check each state and handle all the types involved:
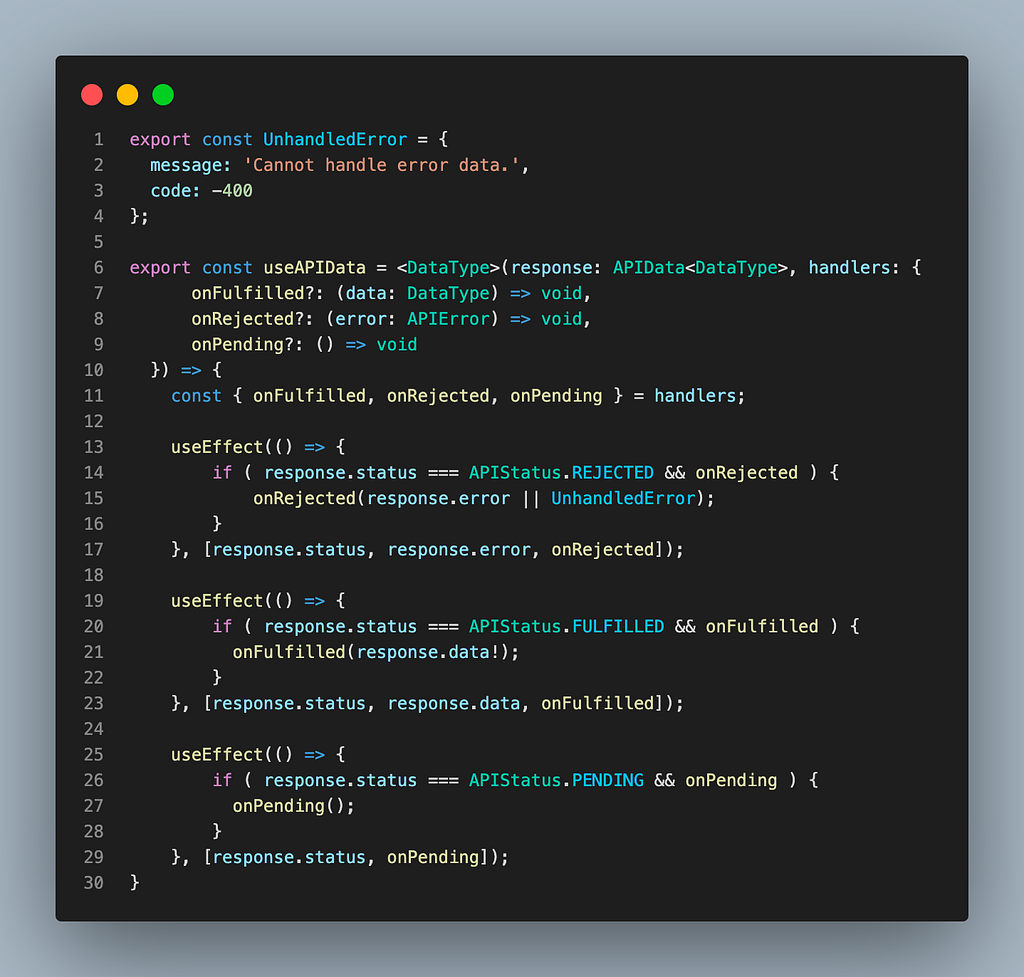
The hook above preserve the types defined into our API and provide, for each state, directly the usable data. The first argument is the APIData object used, the second argument is an object where we can define the callbacks for each state ( If there are commons logics now we can reuse the same callback straightway into multiple API ).
If the second argument object is defined inline in our component we need to wrap it inside the useMemo callback otherwise, for each rendering cycle the object will change firing again the events.
Below the code with the same logic used before but with useAPIData hook:
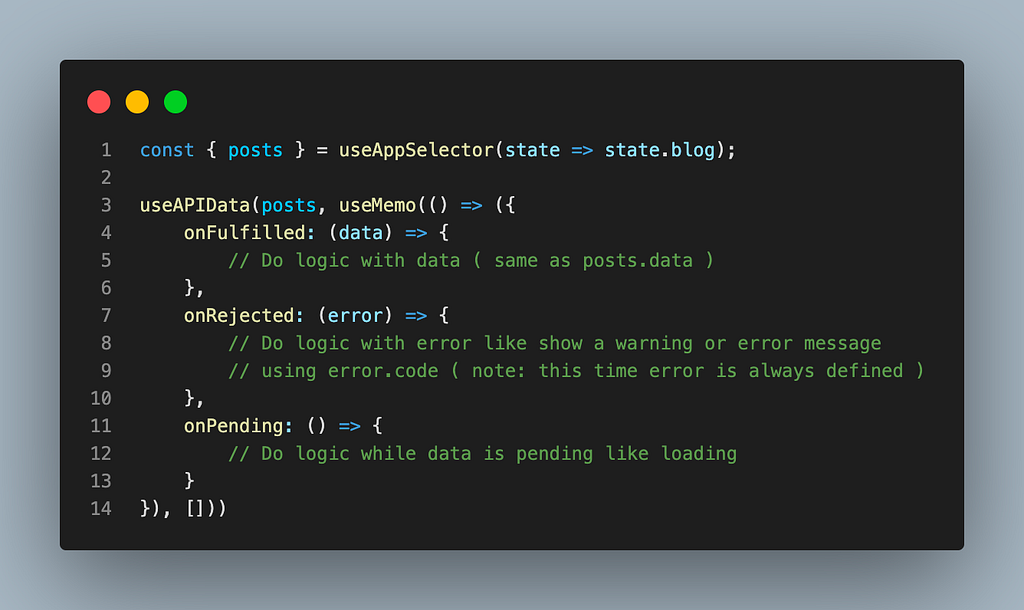
In this case I’ve put the object inline to show the use with useMemo hook but, if the there isn’t dependency from other component’s variables, is better to define it outside the component.
That’s all, I’ve skipped some concept about the use of hook, redux and axios but I’ve linked the website or the documentation for all them in this post :)
Thank you and wish you have an awesome coding journey!
Handle API lifecycle with React, Axios and Redux Toolkit was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Davide Francesco Merico
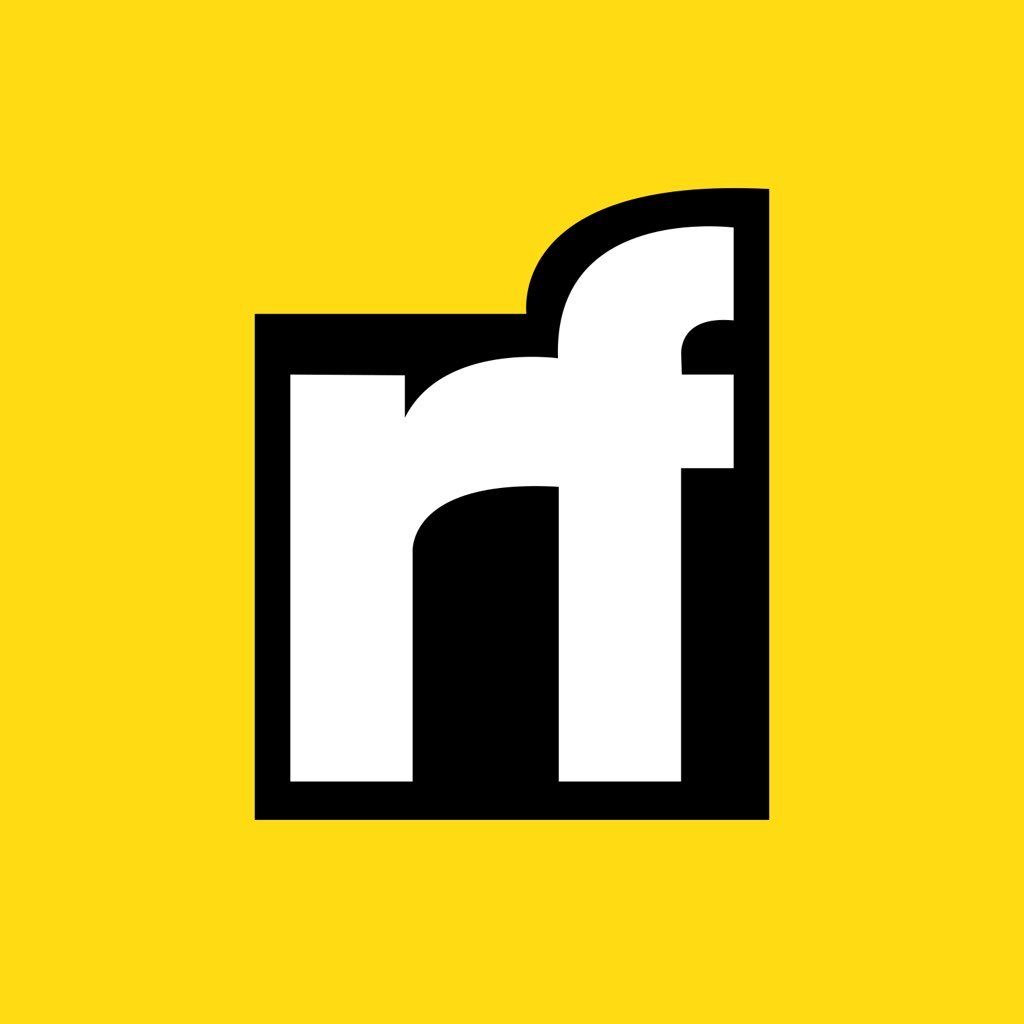
Davide Francesco Merico | Sciencx (2022-03-19T21:12:05+00:00) Handle API lifecycle with React, Axios and Redux Toolkit. Retrieved from https://www.scien.cx/2022/03/19/handle-api-lifecycle-with-react-axios-and-redux-toolkit/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.