This content originally appeared on DEV Community and was authored by Gülsen 🦋
copyWith
copyWith methodu farklı değerlere sahip nesneleri klonlamayı sağlar.
Not: Birçok alternatifin aksine, Freezed kullanılırken copyWith yöntemi bir değere null değer atamayı doğru şekilde destekler.
Örnek olarak bir Person sınıfını ele alacak olursak:
@freezed
class Person with _$Person {
factory Person(String name, int age) = _Person;
}
O zaman şunu yazabiliriz:
var person = Person('Remi', 24);
// `age` değiştirilmedi, değerini korur
print(person.copyWith(name: 'Dash')); // Person(name: Dash, age: 24)
// `age` `null` olarak ayarlandı
print(person.copyWith(age: null)); // Person(name: Remi, age: null)
CopyWith'in null parametreleri nasıl doğru bir şekilde anlayabildiğine dikkat edin.
Deep copy
Aşağıdaki sınıfları göz önünde bulunduracak olursak:
@freezed
class Company with _$Company {
factory Company({String? name, Director? director}) = _Company;
}
@freezed
class Director with _$Director {
factory Director({String? name, Assistant? assistant}) = _Director;
}
@freezed
class Assistant with _$Assistant {
factory Assistant({String? name, int? age}) = _Assistant;
}
Ardından, Company ile ilgili bir referanstan Assistant üzerinde değişiklikler yapmak isteyebiliriz.
Örneğin, bir asistanın name alanını değiştirmek için copyWith kullanarak şunu yazmamız gerekir:
Company company;
Company newCompany = company.copyWith(
director: company.director.copyWith(
assistant: company.director.assistant.copyWith(
name: 'John Smith',
),
),
);
Bu işe yarar, ancak birçok kopya ile nispeten ayrıntılıdır. Burada Freezed'in "deep copy"sini kullanabiliriz.
Company company;
Company newCompany = company.copyWith.director.assistant(name: 'John Smith');
Bu snippet, önceki snippet'le (güncellenmiş bir assistant name ile yeni bir company oluşturma) kesinlikle aynı sonucu elde edecek, ancak artık kopyaları yok.
Company company;
Company newCompany = company.copyWith.director(name: 'John Doe');
Genel olarak, yukarıda bahsedilen Company/Director/Assistant tanımlarına göre, aşağıdaki tüm "copy" sözdizimleri çalışacaktır:
Company company;
company = company.copyWith(name: 'Google', director: Director(...));
company = company.copyWith.director(name: 'Larry', assistant: Assistant(...));
company = company.copyWith.director.assistant(name: 'John', age: 42);
Boş değerlendirme-Null consideration
Bazı nesneler de boş olabilir. Örneğin, Company sınıfımızı kullanarak, Director null olabilir.
Buna göre aşağıdakini yazmak mantıklı değil:
Company company = Company(name: 'Google', director: null);
Company newCompany = company.copyWith.director.assistant(name: 'John');
Başta director yoksa director'ün asistanını değiştiremeyiz.\
Bu durumda, company.copyWith.director null değerini döndürür ve önceki örneğimiz null istisnasıyla sonuçlanır.
Düzeltmek için ?. operatör'ünü kullanabiliriz.
Company? newCompany = company.copyWith.director?.assistant(name: 'John');
Ayrıca copyWith'i tüm constructor'larda tanımlanan propertilerle kullanabilirsiniz:
var example = Example.person('Remi', 24);
print(example.copyWith(name: 'Dash')); // Example.person(name: Dash, age: 24)
example = Example.city('London', 8900000);
print(example.copyWith(name: 'Paris')); // Example.city(name: Paris, population: 8900000)
This content originally appeared on DEV Community and was authored by Gülsen 🦋
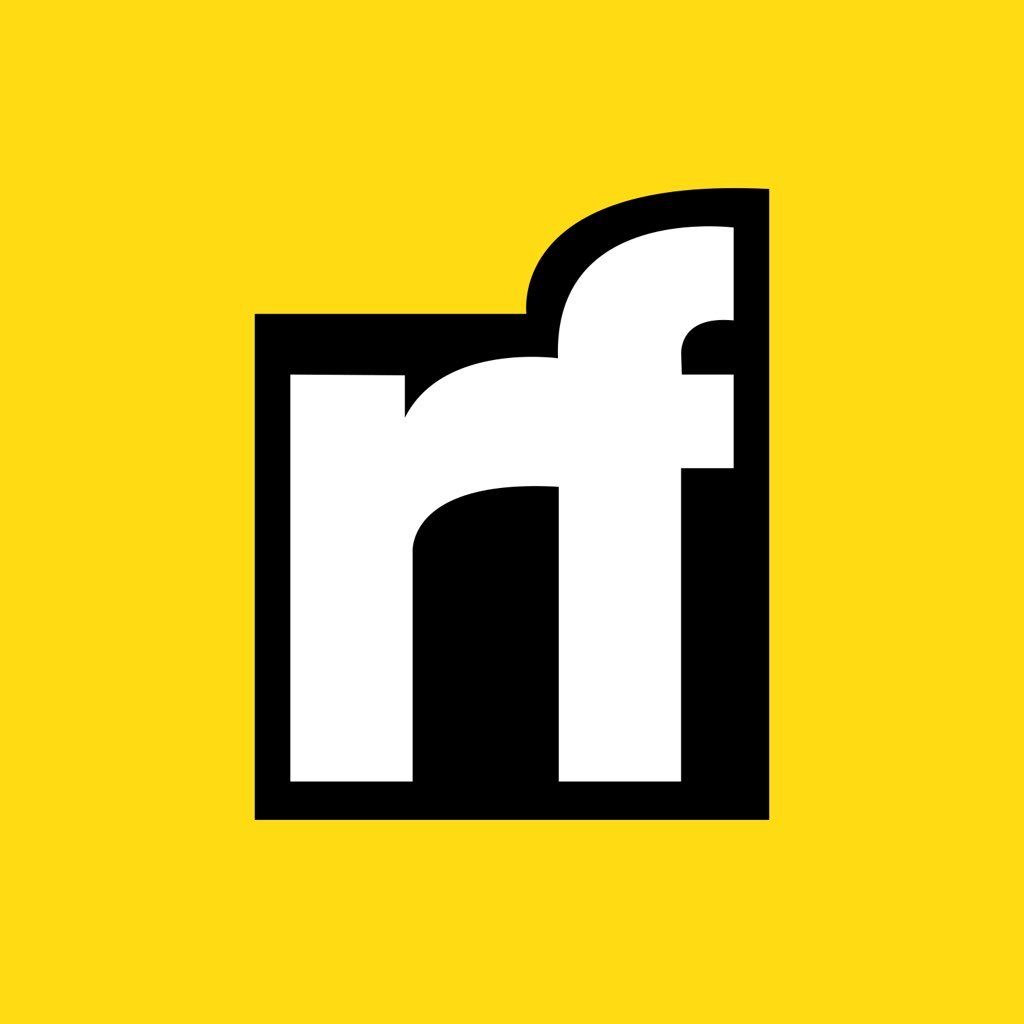
Gülsen 🦋 | Sciencx (2022-03-20T21:43:23+00:00) Flutter copyWith Method 💫 🌌 ✨. Retrieved from https://www.scien.cx/2022/03/20/flutter-copywith-method-%f0%9f%92%ab-%f0%9f%8c%8c-%e2%9c%a8/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.