This content originally appeared on Go Make Things and was authored by Go Make Things
Yesterday, we learned how to get the value of an input as a number with JavaScript. Today, we’re going to learn how get a Date
object instead.
Let’s dig in!
An example
Let’s imagine you have an input
element with a [type="date"]
attribute.
This creates a browser-native date picker that works in all modern browsers. The value
property of the input
will return the selected date in YYYY-MM-DD
format.
<label for="date">Pick a date</label>
<input type="date" name="date" id="date">
Whenever the user updates the value of the field, you want to get the value of the field
as a Date
object.
let field = document.querySelector('#date');
// Handle date changes
date.addEventListener('input', function () {
// ...
});
Let’s look at two ways you can do that.
Using the new Date()
constructor
The most common way to do this is to pass the field.value
into a new Date()
constructor to create a new object.
There’s a bit of a trick with this approach though. Let’s say you selected April 7, 2022 from the date picker. If you pass the field.value
into new Date()
, depending on where in the world you live, the returned Date
object may actually be for April 6, 2022.
// Get the date
let date = new Date(field.value);
This happens because YYYY-MM-DD
format date strings use UTC/GMT as their timezone instead of your local timezone.
To work around this, we need to pass in a time string with the date string. We can use midnight, T00:00
, like this.
// Get the date
let date = new Date(`${field.value}T00:00`);
There’s another, simpler way though!
The valueAsDate
property
Just like the valueAsNumber
property we learned about yesterday, the valueAsDate
property gets the value of an input
as a Date
object.
let date = field.valueAsDate;
Unfortunately, it runs into the same issue that passing the field.value
without a time string does: the date is often a day earlier. Here’s another demo.
A more reliable use for the valueAsDate
property is setting the default value of a [type="date"]
field. For example, this will create a Date
object for the current moment in local time, and set the field
value to it.
// Set the field value to the current date
field.valueAsDate = new Date();
⏰ Last Chance! A new session of the Vanilla JS Academy started this week, but it's not too late to join. Sign up today and get 25% off registration.
This content originally appeared on Go Make Things and was authored by Go Make Things
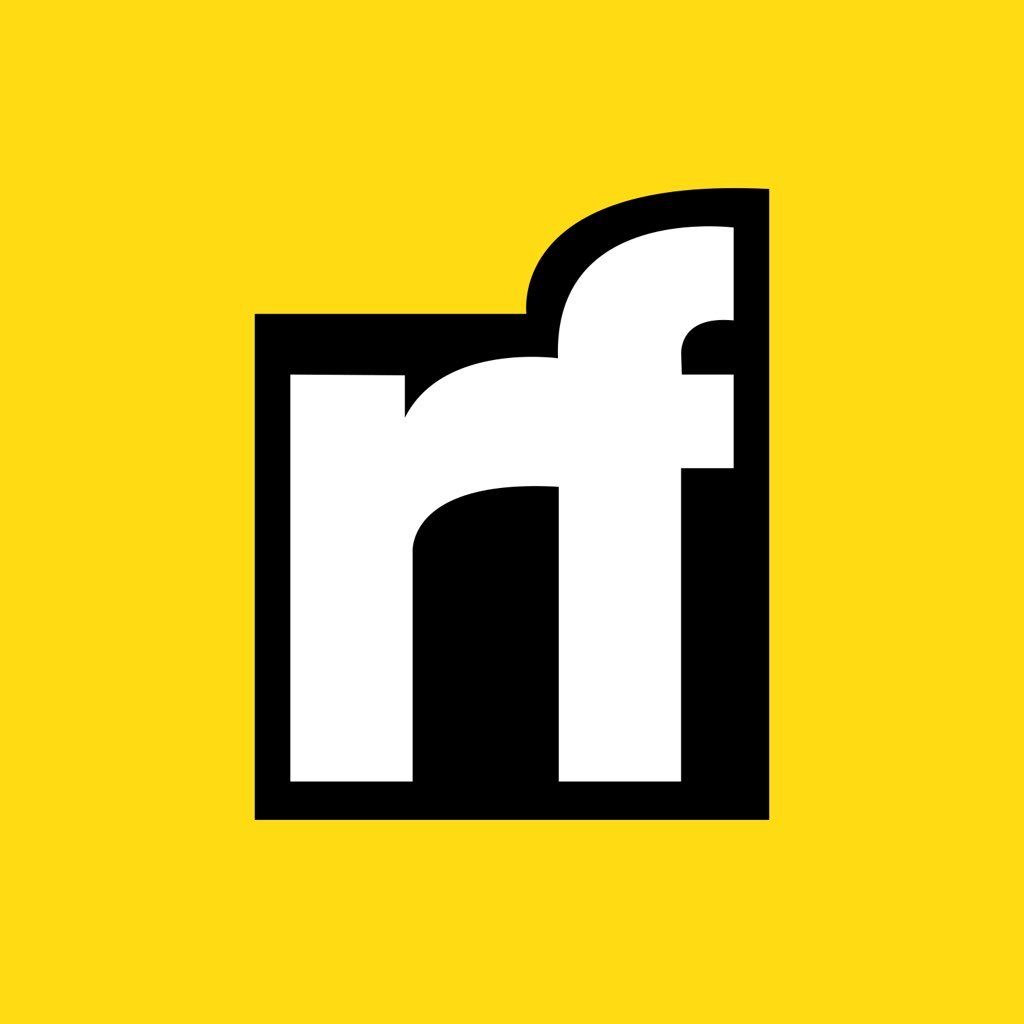
Go Make Things | Sciencx (2022-04-06T14:30:00+00:00) How to get and set a date object from an input with vanilla JavaScript. Retrieved from https://www.scien.cx/2022/04/06/how-to-get-and-set-a-date-object-from-an-input-with-vanilla-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.