Python Flask — A Powerful And Flexible Web App Framework
An introduction to the Python Flask Library
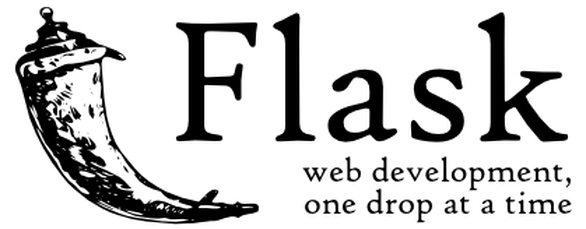
In some of my past projects, I was able to develop quick prototypes and even use python code for one of my chrome extension projects thanks to Flask!
Note* Chrome extensions are typically developed in Javascript only.
The simplicity and shallow learning curve make Flask the perfect web framework for beginners!
Wait! What is a web framework and how is Flask important?
In this article, I will be sharing everything about the Python Flask library.
What Exactly is Flask?
Accordingly to the official definition of Flask in PyPI
Flask is a lightweight WSGI web application framework. It is designed to make getting started quick and easy, with the ability to scale up to complex applications. It began as a simple wrapper around Werkzeug and Jinja and has become one of the most popular Python web application frameworks.
In simple terms, it is a web framework that is beginner-friendly and scalable.
For beginners, a web framework is essentially a template that serves as a foundation for you to build web applications (i.e. websites) and services.
All you’ll need to know is that with a web framework like Flask, you can get more done in less time by using a collection of packages and libraries, and it reduces a lot of friction in your workflow!
It’s almost like you have a prebuilt lego set that allows you to build on top of it for a seamless product creation process.

More specifically, Flask allows you to build…
- Blogging App
- Feedback Form
- Personal Website
- REST API solutions
- … and more!
You can even deploy machine learning models using Flask!
Deploying machine learning models on Chrome Extensions was actually one of my first uses of Flask in my project. You can check out my Machine Learning Chrome Extension video demo below.
I hope you enjoyed the demo video and are impressed by the capabilities of Flask! (and machine learning of course haha)
Note* This video was recorded in 2020 as part of Nanyang Polytechnic (School of Information Technology) Open House Demonstration
For those who are new to Flask, perhaps it may be better to stick with simpler use cases before moving to more advanced ones.
To help you with that, let me show you how you can get started with Flask.
Getting Started with Flask
In this article, I will presume you have some basic knowledge of Python programming.
Installation from PyPI
Firstly, you’d want to install the Flask library from PyPI.
pip install flask
To verify that you’ve installed it successfully, you can use
pip show flask
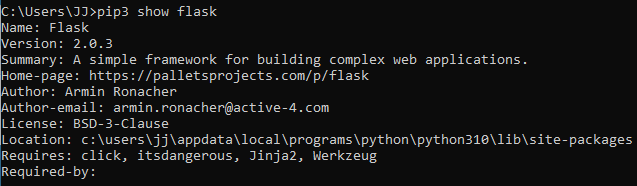
Time for the Code!
Next, create a python file flask_server.py and copy these python codes into the file.
# import the flask object
from flask import Flask
# define a flask application instance
app = Flask(__name__)
# specify a app route ('/') to handle incoming web requests
@app.route('/')
def hello():
# return 'Hello World' as the response for '/' route
return 'Hello World'
- As the comment explains, we first import that Flask class using from flask import Flask
- We then define our Flask application instance using app = Flask(__name__).
Why did we pass in __name__ into our Flask object? The short answer is that it helps Flask to better find resources (i.e. static and template files).
The long answer is that the Flask class takes in the “import_name” argument, which is the name of an imported package. Flask then proceeds to identify the root path of this imported package, and uses this root path as the base in which they begin to scan for static and template directory names. Confused? Please refer to this article if you wish to learn more!
- Next, we create an app route for the endpoint (‘/’) to return ‘Hello World’ when a user visits our web app.
Running your Flask Server
Great! Now we have our project fully set up and we are ready to run our flask server! The final thing we need to do to run our server is to set the Flask environment variable (a fancy term for variables that your computer can recognize)
Setting Environment Variable
We will need to set the FLASK_APP environment variable so that the Flask library knows where to find your application in order to use it. In the examples below, you can choose to either use PowerShell or Command Prompt on Windows.
PowerShell
# set the environment variable 'FLASK_APP' to our python filename
> $env:FLASK_APP = "flask_server"
CMD
# set the environment variable 'FLASK_APP' to our python filename
> set FLASK_APP=flask_server.py
Note* if your file is named app.py or wsgi.py, you don’t have to set the FLASK_APP environment variable as your Flask app would be automatically detected.
Running Flask
Finally, we can run the Flask server with a simple command
> flask run
That’s it! Your Flask server should now be up and running.

Visiting Your Flask Server Endpoint
By default, Flask runs on localhost port 5000(IP address = 127.0.0.1:5000). You can simply make an HTTP request by visiting HTTP://127.0.0.01:5000/ on your browser and you should see your returned message!
In browser

In Flask App

Congrats! You just created your FIRST Flask App!
You did it! You just created a functional flask app!
Now, I am positive that the current application is far from functional. But you now have a solid base to work on! Flask can be used to build beautiful websites and create valuable solutions.
Exploring Further
Here’s a (very) simple Flask website template you can explore more if you’re keen!
flask_server.py
# import the flask object and render_template
from flask import Flask, render_template
# define a flask application instance
app = Flask(__name__)
# specify a app route ('/') to handle incoming web requests
@app.route('/')
def home():
# return your html file
return render_template('index.html')
templates/index.html
<!DOCTYPE html>
<html>
<head></head>
<body>
<h1> Welcome to my Flask Website</h1>
</body>
</html>
Result

Closing Thoughts
That’s it! I hope you found this tutorial to be useful and perhaps even find yourself implementing this in your very next project!
I’ll likely be writing more technical articles like this so I am more than glad to hear any feedback and thoughts on how I could improve my writing.
As always, I am more than happy to connect on LinkedIn so feel free to hit me up there! I’ll catch you in the next article.
Cheers!
Python Flask — A Powerful And Flexible Web App Framework was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
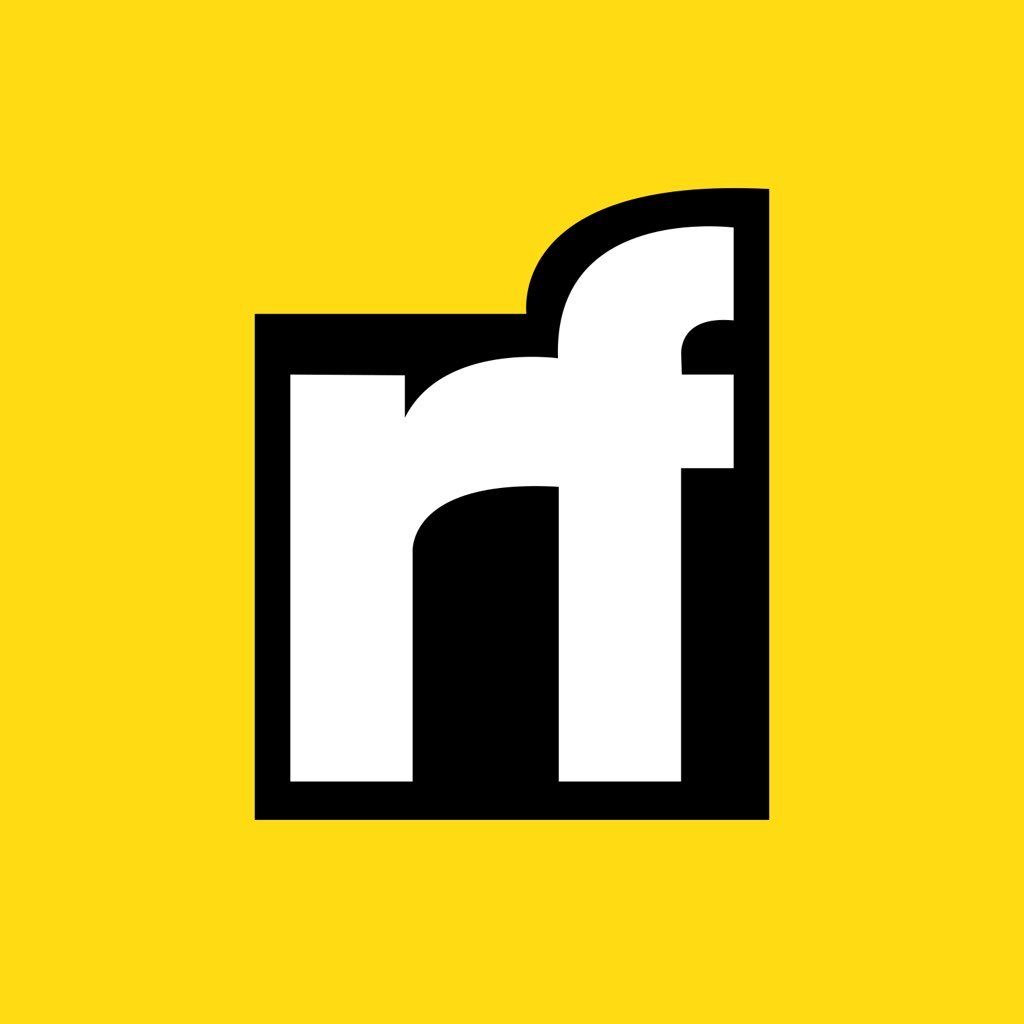
Lye Jia Jun | Sciencx (2022-05-01T15:57:30+00:00) Python Flask — A Powerful And Flexible Web App Framework. Retrieved from https://www.scien.cx/2022/05/01/python-flask-a-powerful-and-flexible-web-app-framework/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.