This content originally appeared on Bits and Pieces - Medium and was authored by Piumi Liyana Gunawardhana
How to disable SSR for Non-SSR Friendly Components in Next.js
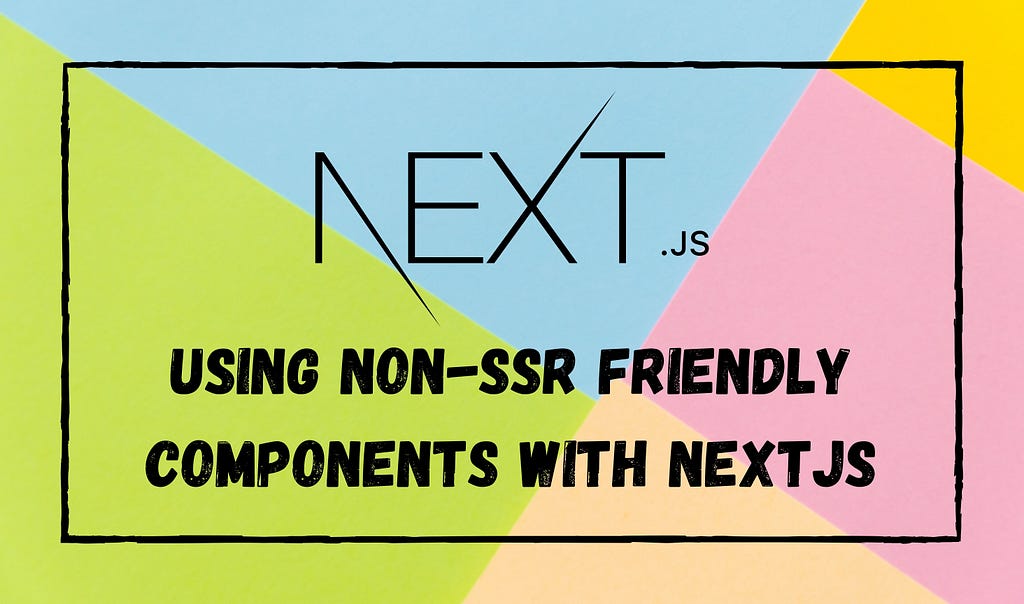
Next.js has become one of the most popular frameworks in the React ecosystem. It facilitates developing Server-side rendered (SSR) React apps without starting over with create-react-app. Next.js routing and other built-in configurations are designed primarily for SSR and crawling towards Static Site Generation (SSG).
But, there can be instances where you will not need SSR for one or more Next.js components in your application. So, in this article, let’s discuss how you can develop non-SSR-friendly Next.js components by disabling SSR.
What is Server-Side-Rendering?
Server-side rendering is an approach for rendering an app on the server in response to every request and then returning the full HTML and Javascript to the client. First, the server handles all necessary data and runs the JavaScript. Then, it generates the page and sends it to the client as a whole.
This makes it possible to serve dynamic components as static HTML markup. SSR can be beneficial for search engine optimization (SEO) whenever indexing fails to interpret JavaScript effectively. It may also be useful when slow internet connectivity makes downloading a large JavaScript bundle cumbersome. In addition, if you need your rendered page to be up-to-date, going for SSR would be ideal.
When Not to Use Server-Side-Rendering?
Sometimes, you might need to opt-out of SSR from Next.js pages or components. For example, integrating third-party libraries and modules that only operate in the browser, or if your component requires accessibility to browser-exclusive properties like the window object or localStorage does not require SSR.
Also, disabling SSR is also beneficial in reducing the size of huge or resource-intensive modules in your bundle that only a few users necessitate.
For non-trivial applications, the usage of SSR may differ because it demands a more extensive configuration and imposes a higher load on the server. Moreover, there is a possibility of the application being slow when there is a lot of processing because the client has to wait for every page to render after making a request. In such scenarios, disabling SSR would be a better option.
So, whether to use SSR or not in your project is a matter of personal preference, and you have to decide between trade-offs based on the situation.
Next, let’s look at a few methods you can use to disable SSR in Next.js.
How to Disable Server-Side-Rendering in Next.js?
1. Use next/dynamic imports
Next.js claims to support ES2020 dynamic import() for JavaScript. It allows you to import JavaScript modules dynamically and works collaboratively with SSR as well.
You can use the ssr: false object to disable server-side rendering of your dynamically imported component, as shown below.
import dynamic from 'next/dynamic'
const ComponentWithNoSSR = dynamic(
() => import('../components/helloWorld'),
{ ssr: false }
)
function Hello() {
return (
<div><Header /><ComponentWithNoSSR/><p>HELLO WORLD!</p></div>
)
}
export default Hello
Or else, you can create a wrapper component called NonSSRWrapper and then enclose any page in that component to disable SSR.
import dynamic from ‘next/dynamic’
import React from ‘react’
const NonSSRWrapper = props => (
<React.Fragment>{props.children}</React.Fragment>
)
export default dynamic(() => Promise.resolve(NonSSRWrapper), {
ssr: false
})
For example, assume that you want to disable SSR on a Home page in the pages folder. It can be done as follows:
// pages/home.js
import NonSSRWrapper from “../components/no-ssr-wrapper”;
const Feed = props => {
return <NonSSRWrapper>
//content
</NonSSRWrapper>
}
2. Check if the window object is defined
We have to check if the window object is defined to see whether we’re on the server-side or the client. To make this easier, a utility function can be defined.
Given below is an example to depict how to disable SSR for components by simply checking for window object is defined or not.
import { Home} from ‘./Home’;
const isSSR = () => typeof window === ‘undefined’;
const HomePage = () => {
return (
<Header />
{!isSSR() && <Home/>}
<Footer />
);
}
export default HomePage;
3. Using react-no-ssr package
no-ssr is a React component that wraps non-SSR components.
This wrapper makes it easy to deal with client-only components when working with applications that use Server-Side Rendering (SSR).
First, you need to install it as below.
npm i --save react-no-ssr
For example, let’s assume that Home component is needed to be rendered on the client-side. Here's how we do it.
import React from 'react';
import NoSSR from 'react-no-ssr';
import Home from './home.jsx';
const DisableSSR= () => (
<div><h2>My SSR Disabled App</h2><hr /><NoSSR><Home/></NoSSR></div>
);
This way <Home/> component will only be rendered on the client after it has been mounted. It will not render while server-side rendered HTML is booting up in the client. Therefore, no React warning messages will appear in the console.
Build composable web applications
Don’t build web monoliths. Use Bit to create and compose decoupled software components — in your favorite frameworks like React or Node. Build scalable and modular applications with a powerful and enjoyable dev experience.
Bring your team to Bit Cloud to host and collaborate on components together, and speed up, scale, and standardize development as a team. Try composable frontends with a Design System or Micro Frontends, or explore the composable backend with serverside components.
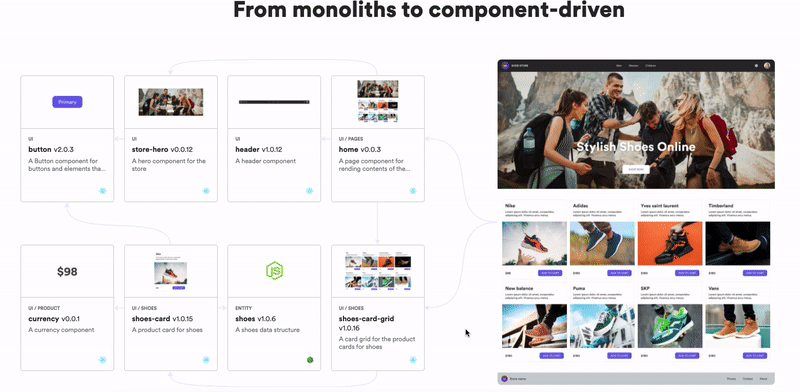
Final Thoughts
This article discussed a few techniques that you can use to disable SSR when it is not needed in your Next.js application. Try to use them where they are applicable to take the maximum benefits. Otherwise, you may face undesired outcomes.
Of course, it is totally up to you to decide whether to disable SSR or not and which of the above methods suits you based on your project requirements. If you have already used these approaches, share your experience in the comment section. Thank you for reading!
Learn More
- Building a Composable UI Component Library
- How We Build Micro Frontends
- How we Build a Component Design System
- How to build a composable blog
- The Composable Enterprise: A Guide
- Meet Component-Driven Content: Applicable, Composable
- Sharing Components at The Enterprise
Using Non-SSR Friendly Components with Next.js was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Piumi Liyana Gunawardhana
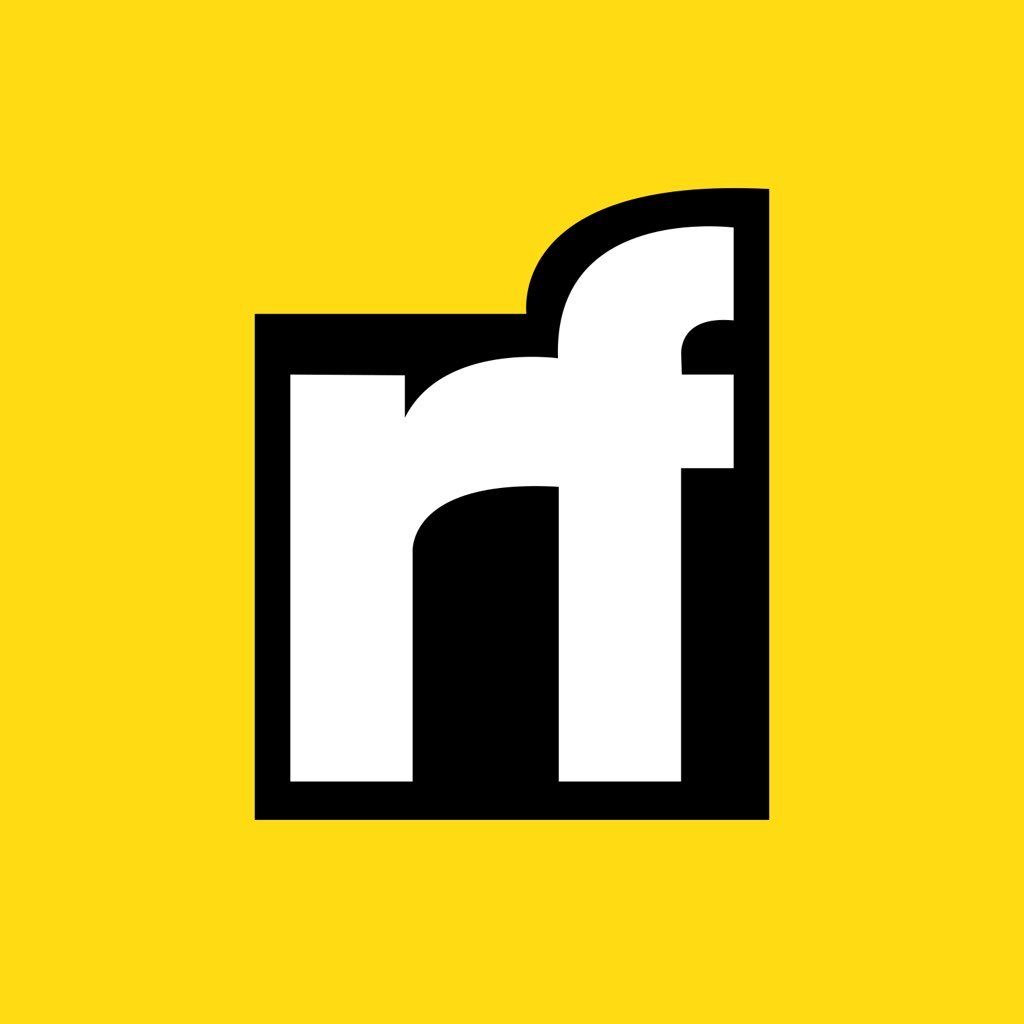
Piumi Liyana Gunawardhana | Sciencx (2022-06-01T06:02:31+00:00) Using Non-SSR Friendly Components with Next.js. Retrieved from https://www.scien.cx/2022/06/01/using-non-ssr-friendly-components-with-next-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.