This content originally appeared on DEV Community and was authored by Nazmus Sayad
The Promise object represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
The Promise object supports two properties: state and result. While a Promise object is "pending" (working), the result is undefined. When a Promise object is "fulfilled", the result is a value. When a Promise object is "rejected", the result is an error object.
We use promise for ajax a lot. But we can also use it for different things. That can make our lives easier. And we can use this feature while working with HTML Form.
My simple HTML Form
<form hidden action="">
<input type="text" name="name" />
<br />
<input type="text" name="username" />
<br />
<input type="password" name="password" />
<br />
<button type="submit">Submit</button>
<button type="button">Close</button>
</form>
<button id="newUser">New User</button>
JavaScript Setup
const newUserBtn = document.getElementById("newUser");
const formElement = document.querySelector("form");
newUserBtn.addEventListener("click", createUser);
- We are working with Promises. The two main ways while working with promises:
// Async/Await version
const createUser = async () => {
try {
const data = await form.getData();
// Do something with your data
console.log(data);
} catch (error) {
// if user closes the form
console.log("User canceled the operation");
}
};
// Then/Catch version
const createUser = () => {
form
.getData()
.then((data) => {
// Do something with your data
console.log(data);
})
.catch(() => {
// if user closes the form
console.log("User canceled the operation");
});
};
- Now the main part:
const form = {
open() {
// Open your Form, In my case:
formElement.removeAttribute("hidden");
},
close() {
// Close your Form, In my case:
formElement.setAttribute("hidden", "");
},
getData() {
this.open(); // Opening Form
return new Promise((resolve, reject) => {
const submitHandler = (event) => {
// Prevent Default Reload
event.preventDefault();
// Getting Form input values
const name = formElement["name"].value;
const username = formElement["username"].value;
const password = formElement["password"].value;
// Sending fulfilled data
resolve({ name, username, password });
// Closing the Form
this.close();
};
const closeHandler = () => {
// Rejecting the promise
reject();
// Closing the form
this.close();
};
formElement.addEventListener("submit", submitHandler, { once: true });
formElement.addEventListener("reset", closeHandler, { once: true });
});
}
};
Demo (Codepen)
This is my first writing. So never mind for the mistakes.
This content originally appeared on DEV Community and was authored by Nazmus Sayad
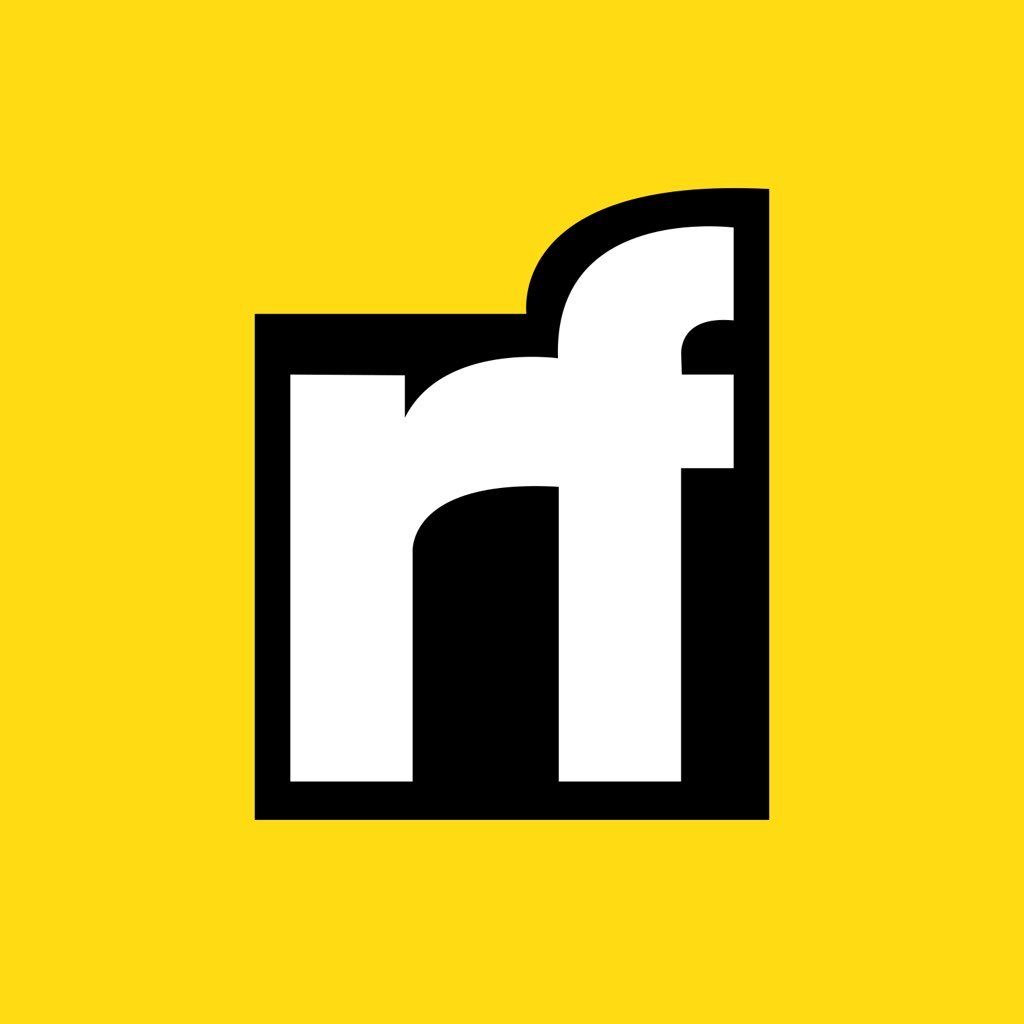
Nazmus Sayad | Sciencx (2022-06-29T18:48:07+00:00) Using promise & async/await with HTML Form. Retrieved from https://www.scien.cx/2022/06/29/using-promise-async-await-with-html-form/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.