This content originally appeared on Level Up Coding - Medium and was authored by Arslan Ahmad
How to prepare smartly for coding interviews?
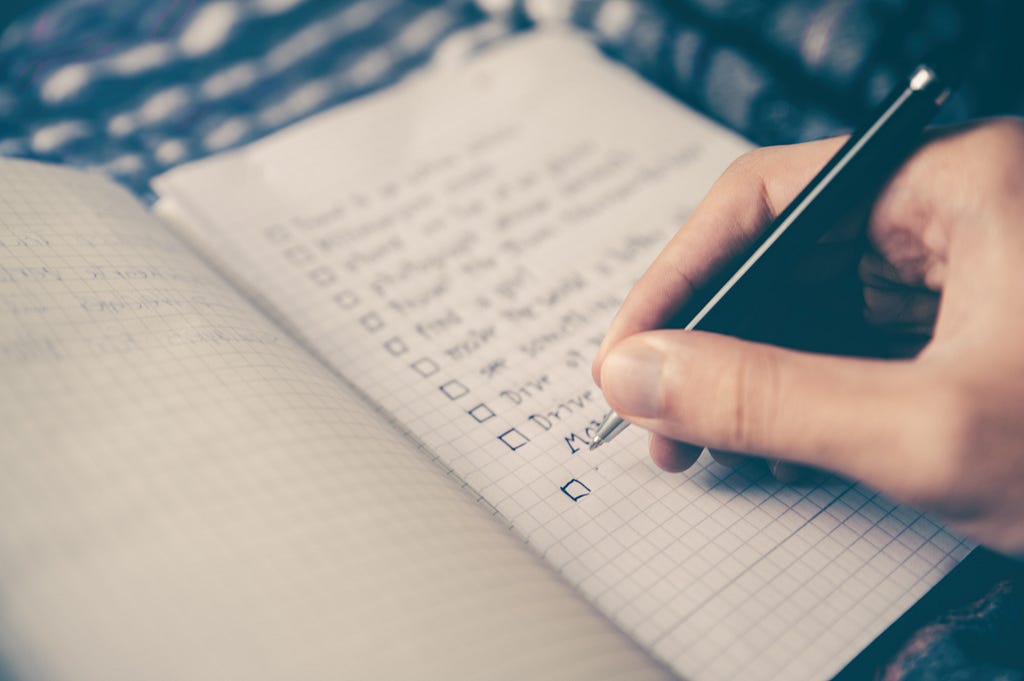
In recent years, the process of preparing for coding interviews has become more challenging. With access to huge sets of difficult coding problems, and an increasingly competitive interview process, it is no longer enough to simply brush up on key data structures and work through a few practice questions.
In this post, I would like to share the strategy that I use to prepare for coding interviews.
I have been working as a software engineer for around 15 years, during which time I have changed jobs five times. I have been through around 30 interview loops, comprising over 120 interviews, and have also had the opportunity to sit on the other side of the table and take over 200 coding interviews and 100 system design interviews.
Even though I consider myself to be a reasonably smart engineer, I have found it challenging to solve coding problems on a whiteboard, particularly in an interview setting where I am being evaluated. To overcome this, I have devoted a significant amount of time to preparation and practice. I follow a systematic approach, working through 12–15 questions for two hours each day. This allows me to solve over 350 problems within a month. Using this routine, I have been successful in my interviews with the FAANG companies (Facebook, Apple, Amazon, Netflix, Google).
How was I able to practice 12+ coding questions every day with a full-time job?
Rather than just solving coding problems, I practice mapping new problems onto ones I have already solved. I will read a problem and spend a few minutes trying to find a similar problem that I am already familiar with. If I can find a match, I will focus on the unique constraints of the new problem and how they differ from the original. If the problem is completely new to me, I will try to solve it and also do some research to see how other people have tackled it. Over time, I have built up a collection of problem patterns that help me quickly map a new problem onto one that I am already familiar with. Here are some examples of these patterns:
- If the given input is sorted (array, list, or matrix), we will use a variation of Binary Search or a Two Pointers strategy.
- If we’re dealing with top/maximum/minimum/closest k elements among n elements, we will use a Heap.
- If we need to try all combinations (or permutations) of the input, we can either use recursive Backtracking or iterative Breadth-First Search.
Following this pattern-based approach helped me save a lot of preparation time. Once you’re familiar with a pattern, you’ll be able to solve dozens of problems with it. Furthermore, this strategy made me confident to tackle unknown problems, as I’ve been practicing mapping unknown problems to known ones.
In the remaining post, I will share all the patterns I’ve collected and present sample problems for a few. For a detailed discussion of these and related problems with solutions, take a look at Grokking the Coding Interview.
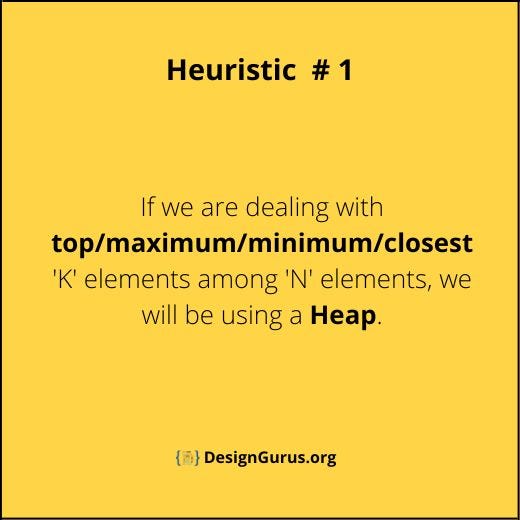
Sample Problem: K closest points to the origin
Problem Statement: Given an array of points in a 2D plane, find K closest points to the origin.
Example: Input: points = [[1,2],[1,3]], K = 1, Output: [[1,2]]
Solution
The Euclidean distance of a point P(x,y) from the origin can be calculated through the following formula:
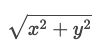
We can use a Max Heap to find K points closest to the origin. We can start by pushing K points in the heap. While iterating through the remaining points, if a point (say P) is closer to the origin than the top point of the max-heap, we will remove that top point from the heap and add P to always keep the closest points in the heap.
Code
Here is what our algorithm will look like:
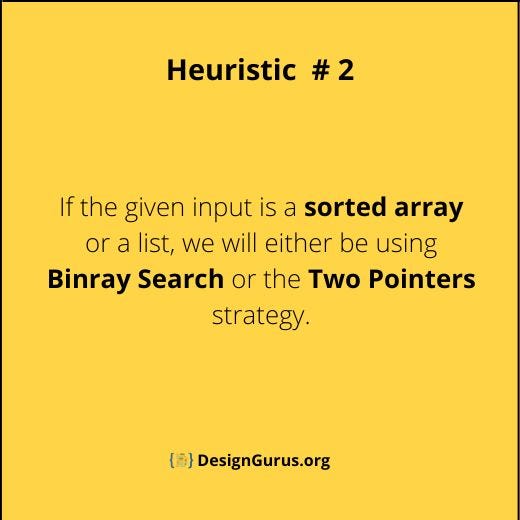
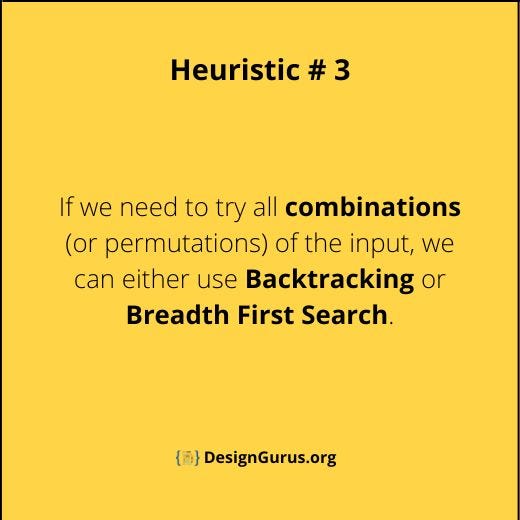
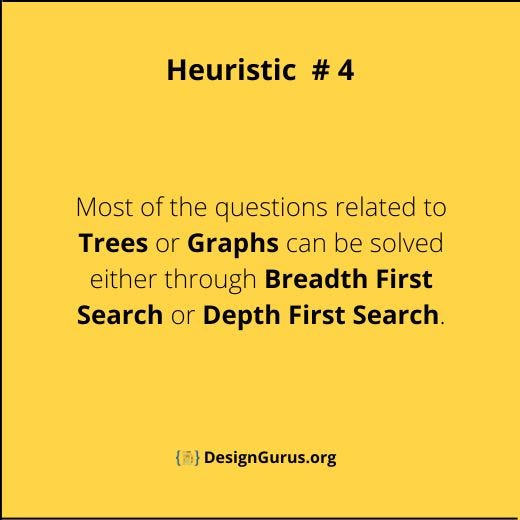
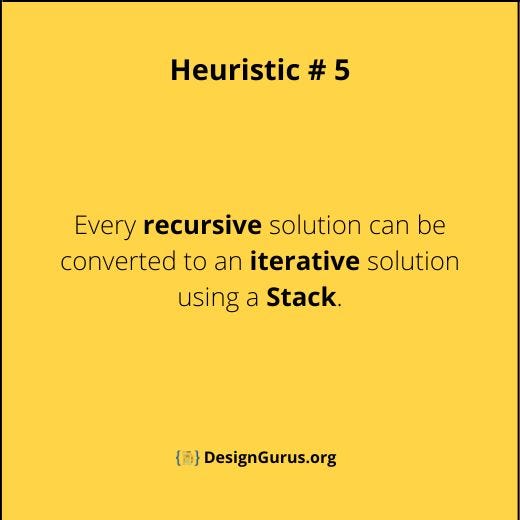
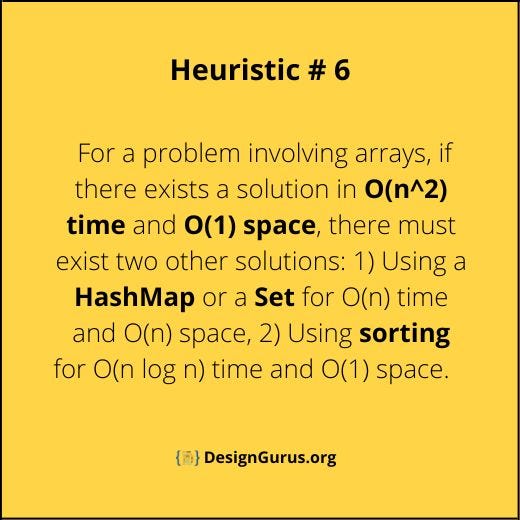
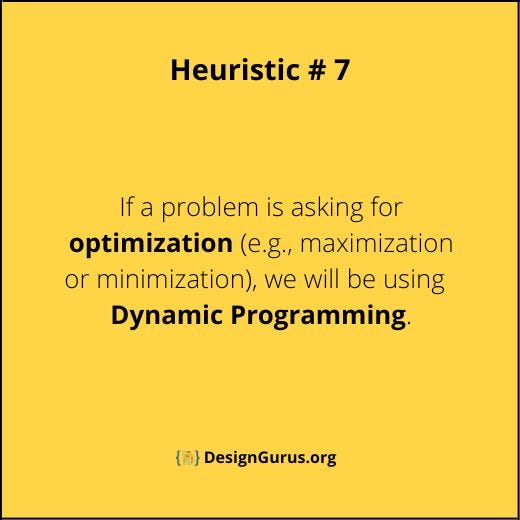
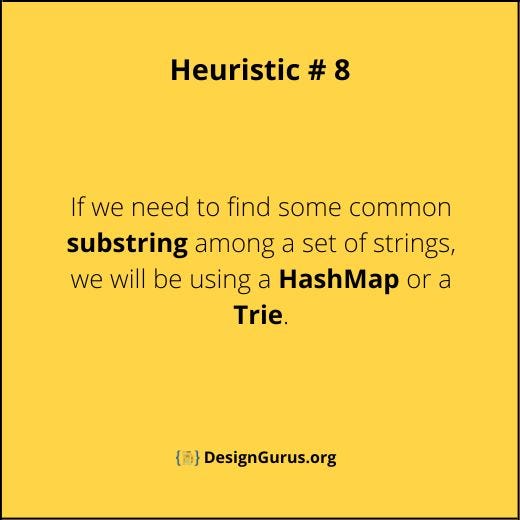
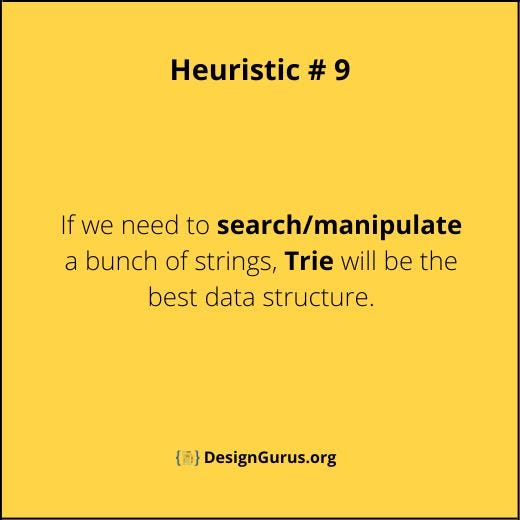
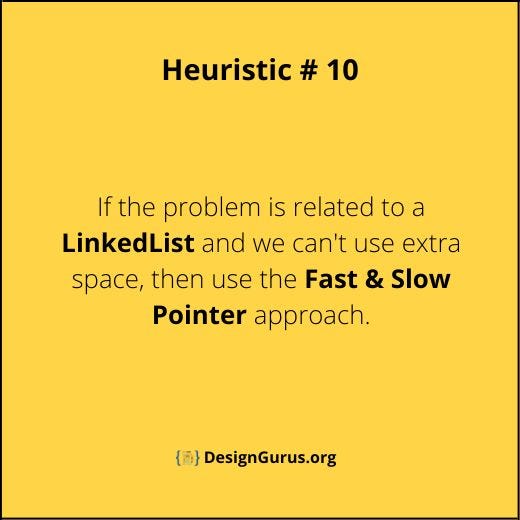
Sample Problem for Heuristic 3: Subsets
Problem Statement: Given a set with distinct elements, find all of its distinct subsets.
Example: Input: [1, 5, 3]
Output: [], [1], [5], [3], [1,5], [1,3], [5,3], [1,5,3]
Solution
We can use the Breadth-First Search (BFS) approach to generate all subsets of the given set. We can start with an empty set, iterate through all numbers one-by-one, and add them to existing sets to create new subsets.
Let’s take the aforementioned example to go through each step of our algorithm:
Given set: [1, 5, 3]
- Start with an empty set: [[]]
- Add the first number (1) to all the existing subsets to create new subsets: [[], [1]];
- Add the second number (5) to all the existing subsets: [[], [1], [5], [1,5]];
- Add the third number (3) to all the existing subsets: [[], [1], [5], [1,5], [3], [1,3], [5,3], [1,5,3]].
Here is the visual representation of the above steps:
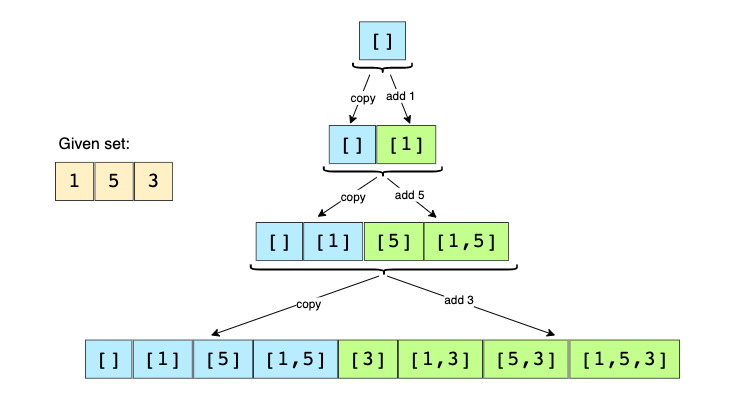
Code
Here is what our algorithm will look like:
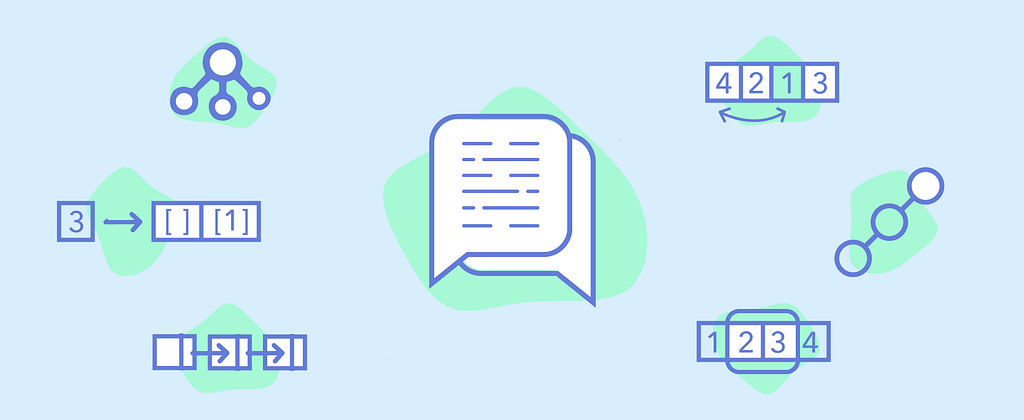
Conclusion
Following these patterns helped me save time for my coding interview prep. Please look at Grokking the Coding Interview to find more of such patterns and their sample problems.
Take a look at Grokking the System Design Interview and Grokking the Advanced System Design Interview for some good examples of system design question and their answers.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
10 Golden Heuristics for Solving a Coding Question in an Interview was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Arslan Ahmad
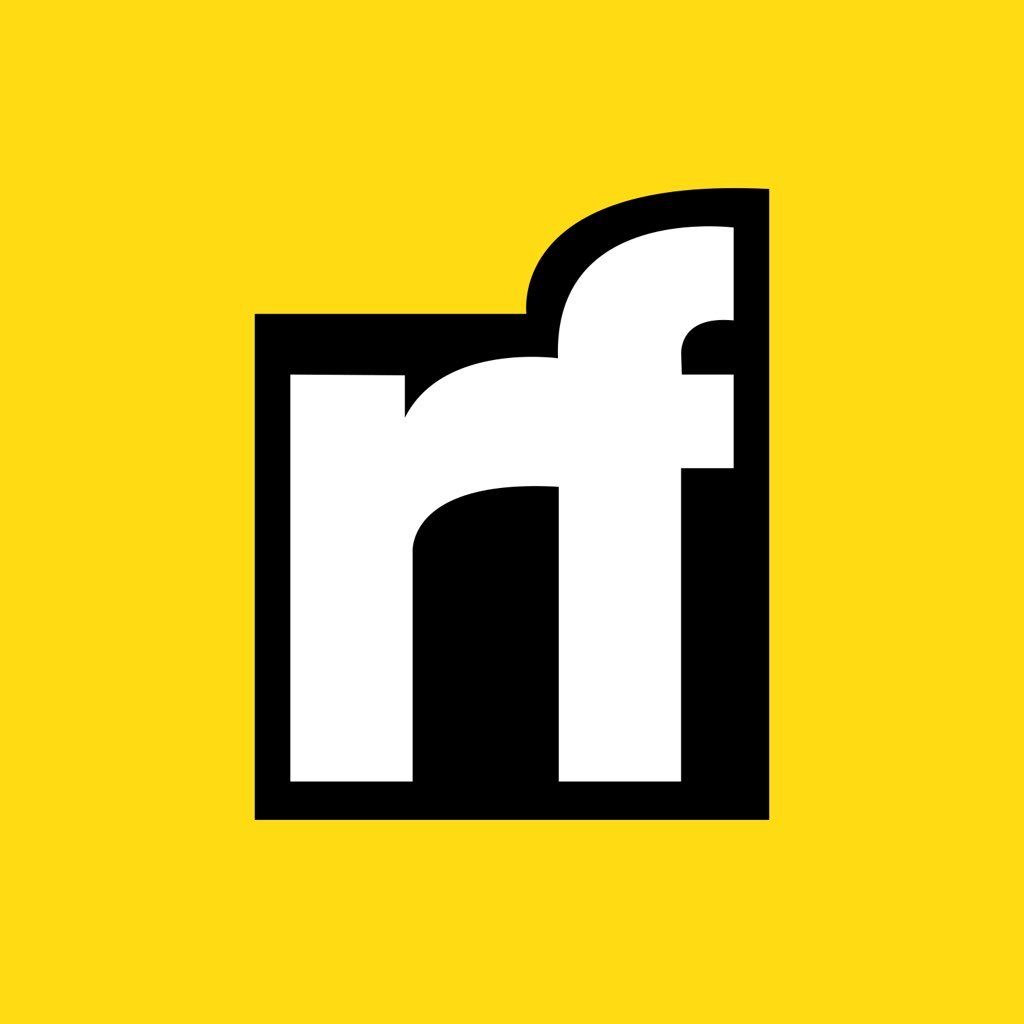
Arslan Ahmad | Sciencx (2022-12-06T12:38:20+00:00) 10 Golden Heuristics for Solving a Coding Question in an Interview. Retrieved from https://www.scien.cx/2022/12/06/10-golden-heuristics-for-solving-a-coding-question-in-an-interview/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.