This content originally appeared on Manuel Matuzović - Blog and was authored by Manuel Matuzović
You can use the ::part
CSS pseudo-element to style an element within a shadow tree.
Let's have a look at the following web component.
There's a heading and a paragraph in the shadow DOM and we can pass content via light DOM.
class NewsTeasers extends HTMLElement {
constructor() {
super();
this.attachShadow({mode: 'open'});
this.shadowRoot.innerHTML = `
<h3>Latest news</h3>
<p>Here's a selection of our latest blog posts.</p>
<slot></slot>
`
}
}
customElements.define('news-teasers', NewsTeasers);
<news-teasers>
<ol>
<li><a href="#1">Blog post 1</a></li>
<li><a href="#2">Blog post 2</a></li>
<li><a href="#3">Blog post 3</a></li>
<li><a href="#4">Blog post 4</a></li>
</ol>
</news-teasers>
Sometimes it's desirable to allow authors to style web components from the outside. I've written about our options on day 10, day 18, and day 28. In summary, we can style the element itself, we can change inheritable properties, and we can style elements in light DOM.
/* the element itself */
news-teasers {
border: 8px dashed;
}
/* inheritable properties */
news-teasers {
color: green;
}
/* light DOM */
news-teasers ol {
border: 4px dotted;
}
If we try to style elements in the shadow DOM, we're out of luck. We don't have access to them from the outside.
/* shadow DOM */
news-teasers h3 {
font-size: 2rem;
}
news-teasers p {
background-color: #000;
color: #fff;
}
This is where [part]
and ::part
come into play. The part
attribute exposes an element outside of the shadow tree and the ::part()
pseudo-element allows you to select the exposed element.
class NewsTeasers extends HTMLElement {
constructor() {
super();
this.attachShadow({mode: 'open'});
this.shadowRoot.innerHTML = `
<h3 part="heading">Latest news</h3>
<p part="intro">Here's a selection of our latest blog posts.</p>
<slot></slot>
`
}
}
customElements.define('news-teasers', NewsTeasers);
/* exposed parts from the shadow DOM */
news-teasers::part(heading) {
font-size: 2rem;
}
news-teasers::part(intro) {
background-color: #000;
color: #fff;
}
My blog doesn't support comments yet, but you can reply via blog@matuzo.at.
This content originally appeared on Manuel Matuzović - Blog and was authored by Manuel Matuzović
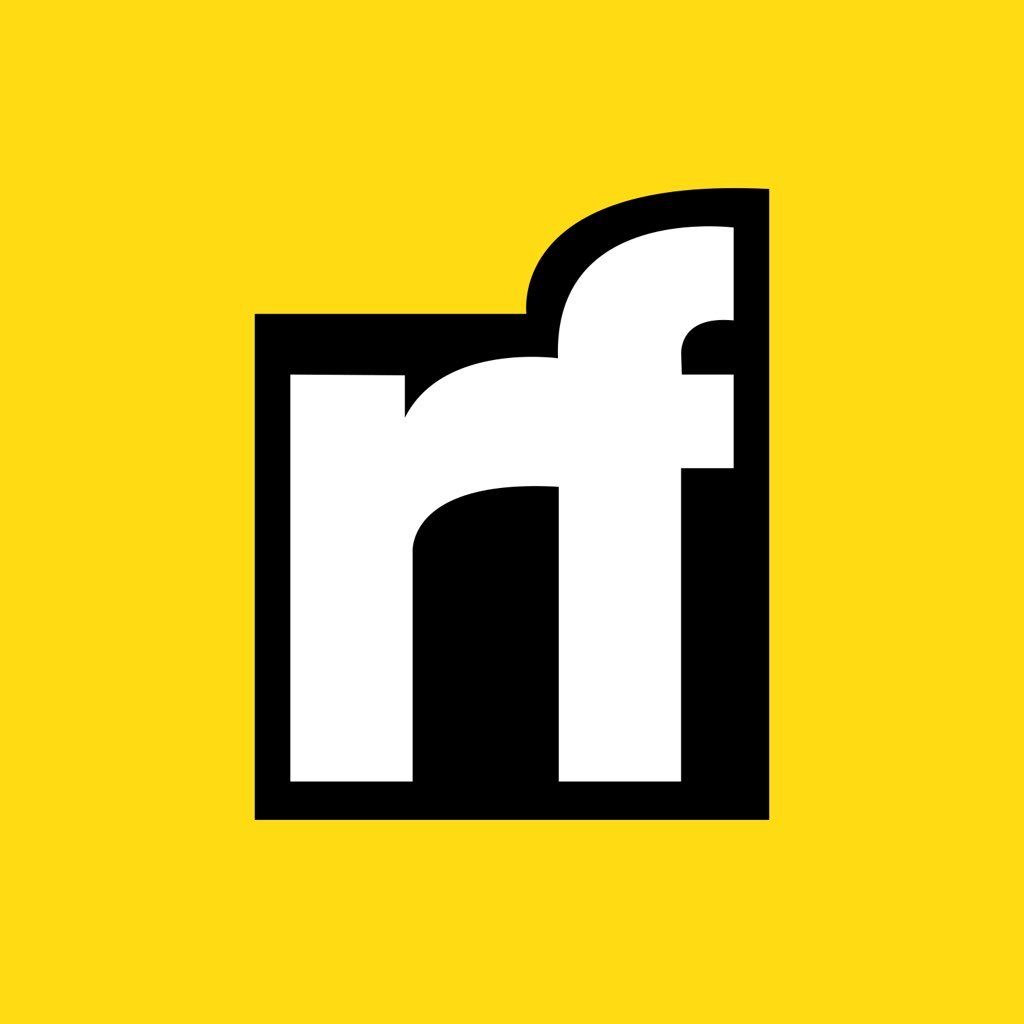
Manuel Matuzović | Sciencx (2022-12-16T00:00:00+00:00) Day 60: the ::part() pseudo-element. Retrieved from https://www.scien.cx/2022/12/16/day-60-the-part-pseudo-element-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.