This content originally appeared on Level Up Coding - Medium and was authored by Yurko
Practical GraphQL requests with Kotlin
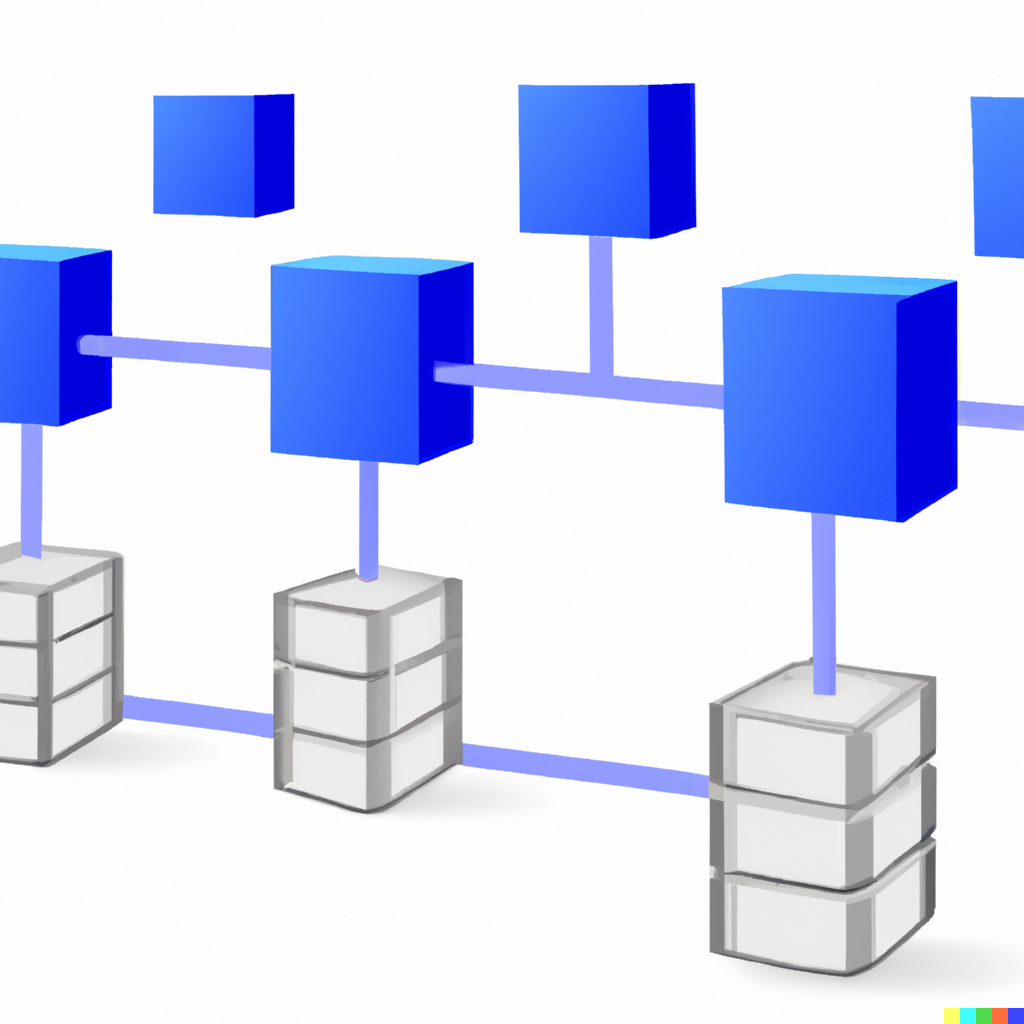
Introduction
Everyone knows how to use REST API but there are alternatives and I will try to briefly explain what we can do with them in the practical scenario. For this post, the example will walk through creating Queries/Mutations to obtain data from one of the NFT providers (Sorare) to check the scores, players' info, etc. The queries playground can be accessed at https://api.sorare.com/graphql/playground (and no, I am not paid to promote, it’s just a game I am playing sometimes and it’s always easier to do stuff you can use later on).
But first a bit of theory :
GraphQL is a query language for your API, and runtime for executing those queries against your data. Unlike REST, which exposes a fixed set of endpoints for each resource, GraphQL allows the client to request exactly the data they need and nothing more. This can result in fewer network requests and a more efficient API. Additionally, GraphQL includes built-in support for real-time updates, making it a popular choice for building real-time, collaborative applications. Overall, GraphQL can provide a more flexible and efficient alternative to REST for building APIs.
Tools:
There are a number of libraries that allow easier interaction with graphQL but for the post, I picked graphql-kotlin from expediagroup. It felt the most natural and easy to use for me. The biggest difference is that it does not require a full schema to generate supported classes but can do it query or mutation based. For our example we want the client to connect to sorare API and will place our query files in the resources folder. So the resulting build.gradle file will look something like this:
Here we tell the graphql-kotlin plugin to take all query files from main/resources/queries folder and put the resulting generated classes into the defined directory.
Implementation
Let’s start with a simple example of getting all players cards with simple conditions like position, rarity, and age. The query files should be placed in the directory specified in the build.gradle file in our case it’s ./src/main/resources/queies/ . The resulting query will look like
For the first example, we hard-coded all the values to make it a bit simple. We are, also, not interested in all fields in the result but only in name, to show the beauty of graphQL where we can specify what we need and not be spammed with whole objects where we don’t care about 90% of the content.
For simple queries, such a setup is enough but for a bit more complex the APIs will require some kind of authentication. In the case of sorare JWT tokens can be obtained from their signIn mutation. Let’s create a small service for that:
With this mutation, we will create a request with signInInput to obtain a token. Also, notice the token will be specific per “aud” (Audience). The corresponding service for this mutation can look like this:
We create a signIn object with the required data (the only mandatory fields are for email and hashed password in our case). Afterward, we just execute the call with the prepared request and return the token as a String. We do it in Kotlin coroutines block.
Let’s use this token to create a bit complex request to obtain all players with specific age and position and their scores for the last number of games. The query file can be written like this:
In this query, we connected the player node to our request and pass to it our parameter for the number of games. The graphql-kotlin library will generate everything for us and we only need to connect the dots in our service:
Here we create our request variables first in playersRequest, passing desired position, age, and the number of games. Then we get a token from signInService (the implementation is above) and pass it as a header to request, we also need to pass the same audience in the header as the one was used for generating the token.
Let’s put it all together for something that was my goal from the beginning :) — get a list of players for some position with certain age and the average score of the last five games more than the desired value. We will expose it via some REST API so we can call it from everywhere.
We can use the same query but we need to trick a service code a bit. We would like to filter the result to get only players' name and their average scores. The code is just a sample and will ignore the NPE checks etc:
And simple controller class to get the result from API:
We can simply start our application and check the result with the GET request (the screenshot is from a postman client). While there are not that many players with the age of 39 (within the pagination result of sorare cards) it gives us what we wanted.
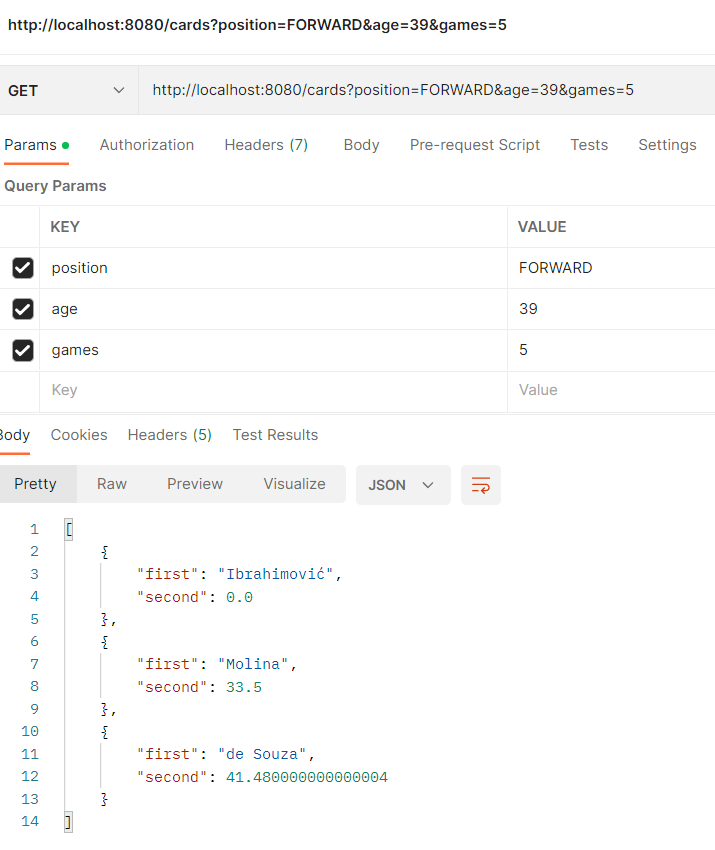
I hope it will help someone to write down graphql client implementation or, perhaps, somebody will create some sorare helper :)
In part 2 I will try to cover more complex cases and perhaps a small trading bot for the same game. And if anyone will use it — let the best bot win :)
Keep coding and have one.
— — — — — — —
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Real world example of GraphQL requests with Kotlin was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Yurko
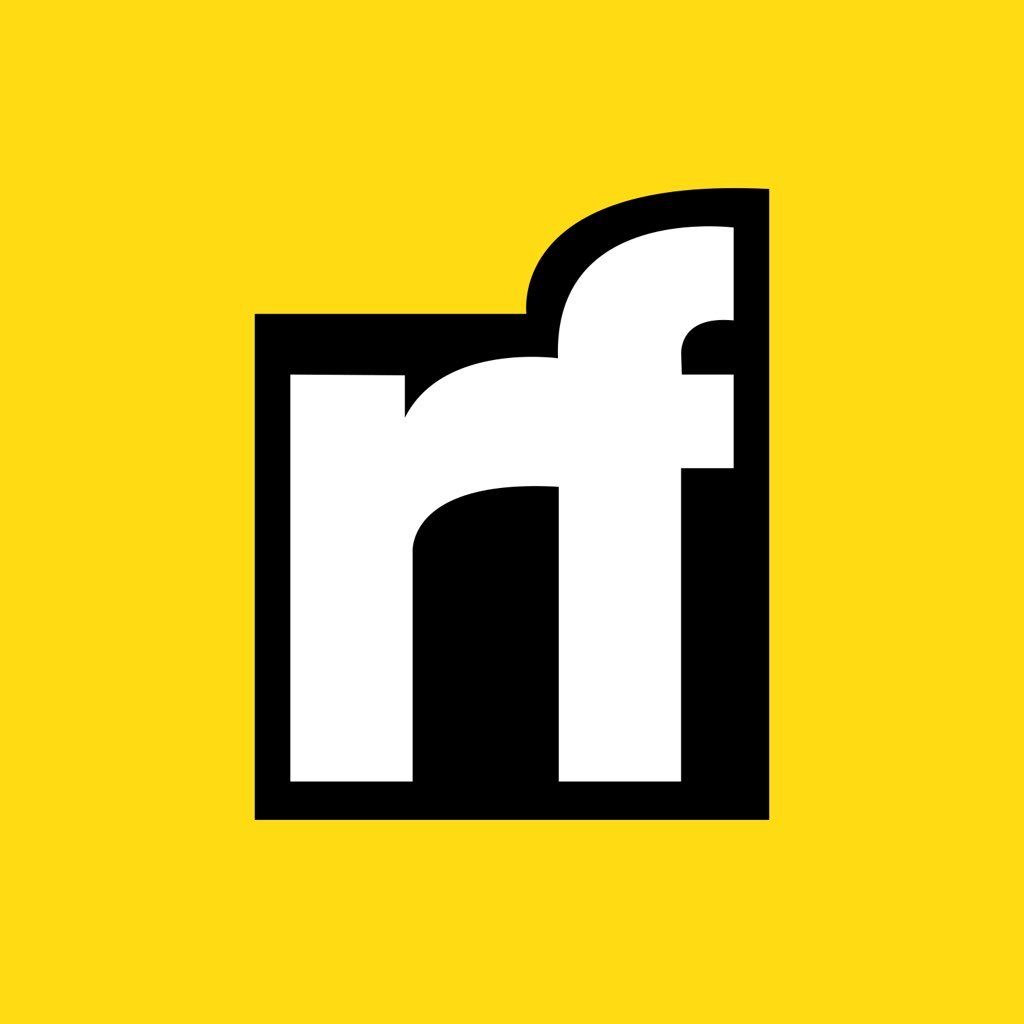
Yurko | Sciencx (2023-01-17T12:20:25+00:00) Real world example of GraphQL requests with Kotlin. Retrieved from https://www.scien.cx/2023/01/17/real-world-example-of-graphql-requests-with-kotlin/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.