This content originally appeared on CodeSource.io and was authored by Mellaoui Mohamed
Imagine that you have a subscription-based application built with Laravel, and you want to send daily emails to all users, I don’t think you really like doing it manually. Checking for new users to copy the email addresses and send emails to them it’s boring and not productive, right? sure,
And here comes the power of Cron jobs.
Cron jobs are powerful tools in any developer’s toolkit, they allow you to automate repetitive tasks and schedule them to run at specific intervals, freeing up your time to focus on other productive things, in Laravel 9, you can easily set up cron jobs using the artisan command-line,
so In this tutorial, we will go through all the steps you should take to make sending emails automated with Laravel Cron Jobs in Laravel 9, we will see how to set up a cron job and schedule Tasks.
Prerequisites
To make sure you get the best out of this tutorial you must have these prerequisites:
- Basic understanding of PHP and Laravel,
- Familiar with MVC architecture and routing in Laravel
- Familiar with Laravel Artisan command
- Understanding of basic database operations in Laravel.
- Access to a server with Laravel 9 Installed.
- Text Editor and a web browser.
Having these prerequisites will help you understand the concept and the steps covered in the tutorial more effectively.
Steps to create Cron Jobs Scheduling in Laravel:
- Install Laravel Application
- Setup Database
- Create a Command Line
- Register Task Scheduler.
- Run a Scheduler.
- Scheduled Jobs on Live server.
1. Install Laravel Application:
First of all, we need to download and set up a Laravel application. With composer installed on your computer, you can download and install Laravel via composer like so:
composer create-project laravel/laravel Newapp
Or you can install Laravel installer globally with the following command:
composer global require laravel/installer
And install Laravel on your local machine using the following command:
laravel new Newapp
2. Setup Database
Now our Laravel Application is installed, we need to setup our database to store the data, head to the .env
file in the Laravel application, and modify the following:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_database_username
DB_PASSWORD=your_database_password
3. Create Command Line
In order to create a command line, you must go inside the Laravel application we created
cd /Newapp
Now you are in the app we can run the Artisan command like so:
php artisan make:command DailyEmails --daily:mails
This will create a new command class in the app/Console/Commands
directory, let’s go to this file.
Here is how it looks:
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\Http;
use Mail;
Use App\Models\User;
class DailyEmails extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'daily:mails';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
info(" daily mailing running". now());
$response = Http::get("https://jsonplaceholder.typicode.com/users");
$users = $response->json();
if(!empty($users)){
foreach($users as $key => $user)
{
if(! User::where('email',$user['email'])->exists()){
User::create([
'name'=> $user['name'],
'email' => $user['email'],
'Password' =>bcrypt('12345678')
]);
}
}
$recivers = User::all();
foreach($recievers as $reciever){
Mail::to($reciever->email)->send('Your email');
}
}
In case you’re wondering why We are using jsonplaceholder
, it’s a free API tool we are using to generate users and save them on our database.
4. Register Task Scheduler
In this step we need to register our new command in the kernel.php file and define the time when we need the command to run:
namespace App\Console;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
use Illuminate\Support\Facades\DB;
class Kernel extends ConsoleKernel
{
/**
* Define the application's command schedule.
*
* @param \Illuminate\Console\Scheduling\Schedule $schedule
* @return void
*/
protected function schedule(Schedule $schedule)
{
$schedule->command('daily:mails')->daily();
}
protected function commands()
{
$this->load(__DIR__.'Commands');
Return base_path('routes/console.php');
}
}
5. Run a Scheduler:
Now we can test our cron, we can manually check using the following command of our cron, so let’s run it:
php artisan schedule:run
check our storage/logs/laravel.php
file you will notice some logs like so:
[2022-03-01 03:51:02] local.INFO: daily mailing running at 2022-03-01 03:51:02
In my case, I Scheduled the command using the daily( )
option which Run the task every day at midnight here the command will run in 7 hours from now as shown below, when to see the scheduled tasks, you need to run the following command:
php artisan schedule:list

6. Scheduled Jobs on the Live server
To schedule Jobs on a live server we need to install crontab, assuming you are using an ubuntu server so it’s already installed, we can run the following command and add a new entry for our cron job:
crontab -e
Now, let’s add the following line to the crontab file, you need to set the Laravel app path correctly on it.
cd /path/to/your/laravel/project && php artisan schedule:run >> /dev/null 2>&1
this line will add a single cron configuration entry to our server that runs the schedule:run
command every time based on the interval we choose here it runs every day at midnight.
Conclusion:
Cron jobs in Laravel are a very powerful tool and flexible, they allow you to automate tasks and perform them at regular time intervals (daily – hourly … etc), freeing up your time to focus on other tasks, some of the key benefits of using cron jobs in Laravel:
- 1 Task scheduling: you can schedule tasks to run automatically at a specific time, without manual intervention.
- 2 Time-saving: Automating tasks using cron jobs saves time and reduces manual efforts.
- 3 Increase efficiency: By automating tasks, you can increase the efficiency of your application and ensure that tasks are completed on time.
In short, cron jobs in Laravel offer a powerful and flexible way to automate tasks and make the most of your time and resources. whether you need to send emails, generate reports, or perform other routine tasks, cron jobs in Laravel are a powerful tool for achieving your goals.
This content originally appeared on CodeSource.io and was authored by Mellaoui Mohamed
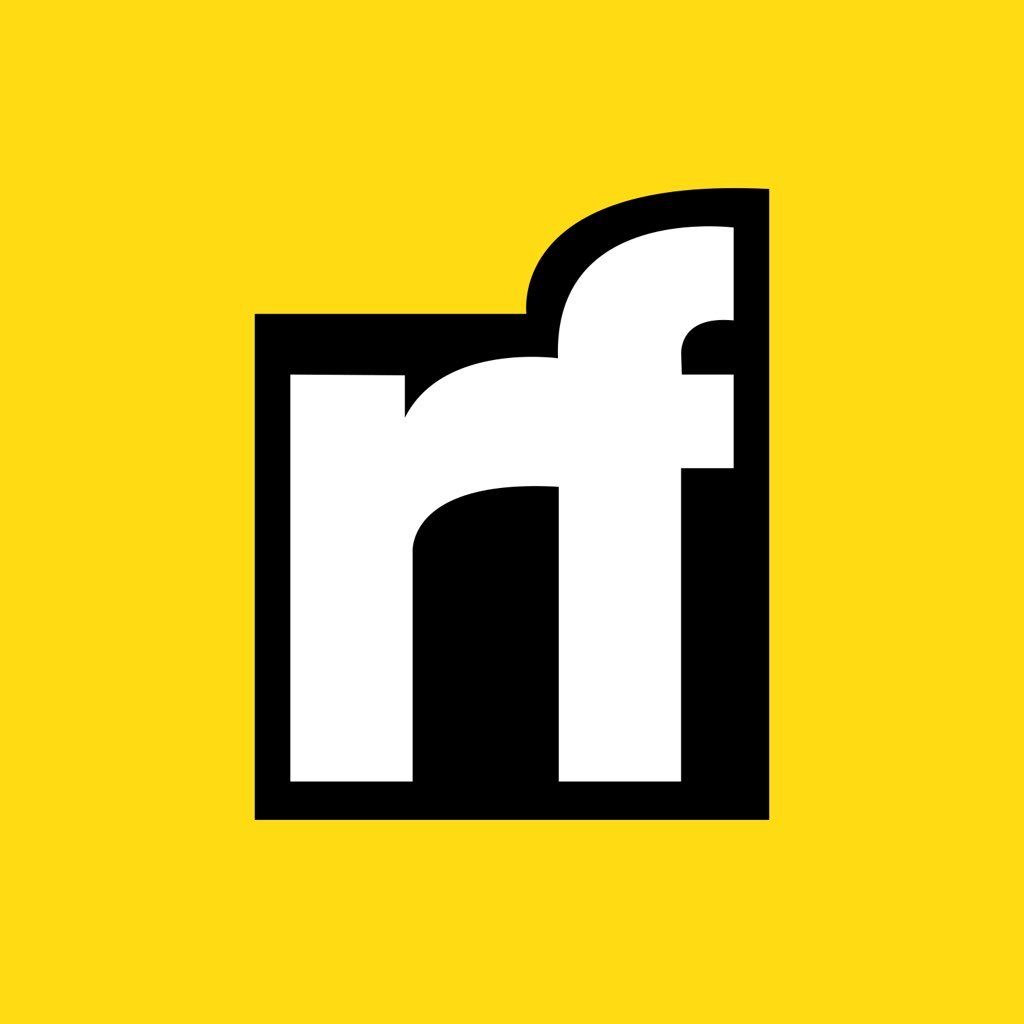
Mellaoui Mohamed | Sciencx (2023-02-08T12:48:27+00:00) A comprehensive Laravel 9 Cron Jobs Scheduling with example. Retrieved from https://www.scien.cx/2023/02/08/a-comprehensive-laravel-9-cron-jobs-scheduling-with-example/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.