This content originally appeared on Bits and Pieces - Medium and was authored by Kabilan Mahathevan
Build a RESTful API with Node.js — A Step-by-Step Guide
Node.js is a popular runtime environment widely used for building server-side applications. It offers an effective way to create applications that are data-intensive, high-performance, and scalable.
Node.js is lightweight and fast, which means that it can be used to build high-performance web applications with minimal overhead. This makes it a great choice for building microservices and other types of lightweight web applications.
Note: Learn more about building Microservices with Node.js in the link below:
Component-Driven Microservices with NodeJS and Bit
Because of easiness to set up and deploy a Node.js application, developers can quickly get started with building and testing their APIs, which also makes it easier for beginners to start learning and developing APIs.
In this article, we will learn how to build an API using Node.js, Express and MongoDB.
What is an API?
API stands for Application Programming Interface. An API is a set of rules and protocols allowing different software applications to communicate with each other. They allow developers to link various systems together and build new applications that make use of already-existing data and functionality, which accelerates development times and boosts innovation in the software sector.
APIs have become increasingly popular in recent years for several reasons;
- Modularity and Reusability: APIs allow developers to divide large systems into smaller, easier-to-manage components that can be used in other applications. Large systems may be built and maintained more easily because of its modularity because developers can work on individual components without affecting the entire system.
- Scalability: Systems can handle massive volumes of traffic and data without becoming overburdened because to the highly scalable nature of APIs. This is significant for web applications that need to manage a lot of user requests.
- Flexibility: It is simpler to create apps that integrate with a variety of other systems by using APIs to connect various systems and technologies together. Because to this flexibility, developers are not constrained to a single technology stack and can select the optimal tools for each component of their program.
- Openness: Publicly accessible APIs enable developers to build on top of already-existing systems and produce fresh software that makes use of current functionality and data. Without APIs, innovation and the development of new services and apps would not have been conceivable.
To build an API with Node.js, we will be using the following framework tools and technologies:
- Express.js: Express is a popular web application framework for Node.js. It offers a comprehensive range of functionality, including as routing, middleware, and HTTP request/response handling, for developing APIs and web applications.
- MongoDB: MongoDB is a popular NoSQL database that is widely used for building web applications. We will be using MongoDB to store and retrieve data for our API.
- Mongoose: Mongoose is an object data modelling (ODM) library for MongoDB. It provides a simple and easy-to-use interface for interacting with MongoDB from Node.js.
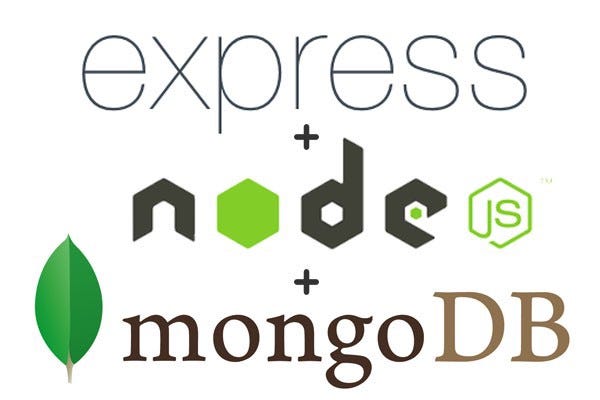
Before we begin, make sure you have the following prerequisites installed on your system:
- Node.js: You can download and install Node.js from the official website.
- MongoDB: You can download and install MongoDB from the official website.
Once you have installed these prerequisites, you are ready to start building your API.
Step 1: Set up the project
The first step is to set up the project and install the required dependencies. To do this, create a new folder for your project and navigate to it in your terminal. Then, run the following command:
npm init
This will ask for a few project initialization values and will create a new package.json file for your project, which will contain the project metadata and the list of dependencies.
Next, install the required dependencies by running the following commands:
npm install express
npm install mongoose
Step 2: Create the server
The next step is to create the server and set up the HTTP request/response handling. To do this, create a new file called server.js and add the following code:
const express = require('express');
const mongoose = require('mongoose');
const app = express();
// built-in middleware for json
app.use(express.json());
// connect to the database
mongoose.connect('mongodb://localhost/mydatabase', {
useUnifiedTopology: true,
useNewUrlParser: true
});
// set up the HTTP request/reponse handling
app.get('/', (req, res) => {
res.send('Hello World!');
});
// start the server
app.listen(3000, ()=> {
console.log('Server started in port 3000')
});
In this code, we are importing the required modules (Express and Mongoose), creating a new instance of the Express app, and connecting to the MongoDB database. We are also setting up a route for handling the HTTP GET request and starting the server on port 3000. To confirm that our server is configured correctly run the server.js file in the terminal using Node.js:
node server.js
You should be able to see the output Server started in the port 3000in the terminal and listening for HTTP requests. To check whether the server is listening on the specified port in the localhost, open your browser and go to localhost:3000. You should be able to the Hello World! text output in the browser as a response to your HTTP request. Since we are building APIs, it’s better to use API clients like Postman and Thunder Client (Lightweight VS Code extension) to create HTTP request to the API endpoints.
Step 3: Create API endpoints
The next step is to create the API endpoints for handling the HTTP requests. To do this, we will create a new file called api.js and add the following code:
const express = require('express');
const router = express.Router();
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
// create a new schema for the data
const DataSchema = new Schema({
name: String,
age: Number
});
const DataModel = mongoose.model("Data", DataSchema);
// get all the data
router.get("/data", (req, res) => {
DataModel.find({}, (err, data) => {
if (err) {
res.status(500).send(err);
}else{
res.json(data);
}
});
});
// get a single data
router.get('/data/:id', (req, res) => {
DataModel.findById(req.params.id, (err, data) => {
if(err) {
res.status(500).send(err);
}else {
res.json(data);
}
});
});
// create new data
router.post('/data', (req, res) => {
const newData = new DataModel(req.body);
newData.save((err, data) => {
if (err) {
res.status(500).send(err);
} else {
res.json(data);
}
});
});
// update existing data
router.put('/data/:id', (req, res) => {
DataModel.findByIdAndUpdate(req.params.id, req.body, { new: true }, (err, data) => {
if (err) {
res.status(500).send(err);
} else {
res.json(data);
}
});
});
// delete existing data
router.delete('/data/:id', (req, res) => {
DataModel.findByIdAndDelete(req.params.id, (err, data) => {
if (err) {
res.status(500).send(err);
} else {
res.json(data);
}
});
});
// export the router
module.exports = router;
In this code, we are creating a new instance of the Express Router and defining the API endpoints for handling the HTTP request. We are also defining a new schema and model for the data and using the Mongoose library to interact with the MongoDB database.
Step 4: Use API in the server
The final step is to use the API in the server by adding the following code to the server.js file:
const api = require('./api');
// use the API endpoints
app.use('/api', api);
In this code, we are importing the API file and using the API endpoints in the Express app by prefixing them with /api. In the MongoDBCompass, you should be able to see your data in the newly created database mydatabase , and the collection datas in the database.
Conclusion
This gives a comprehensive idea of creating an API using Node.j, Express, and MongoDB and how to set up the API endpoints for handling the HTTP requests but this is just a basic setup and there are lot more to it when it comes to creating a large-scale API with different functionalities.
Building APIs with Node.js is a great way to create scalable and high-performance web application, specially good starting point for beginners, and it is a useful skill for any web developer to have.
Build Node.js Apps with reusable components
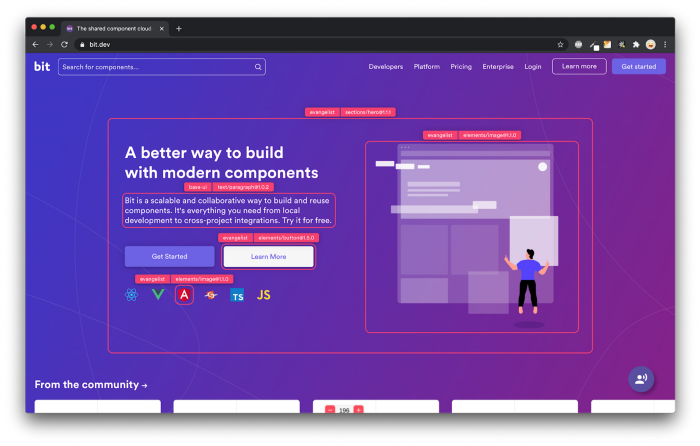
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- Bit - Component driven development
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Build a RESTful API with Node.js — A Step-by-Step Guide was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Kabilan Mahathevan
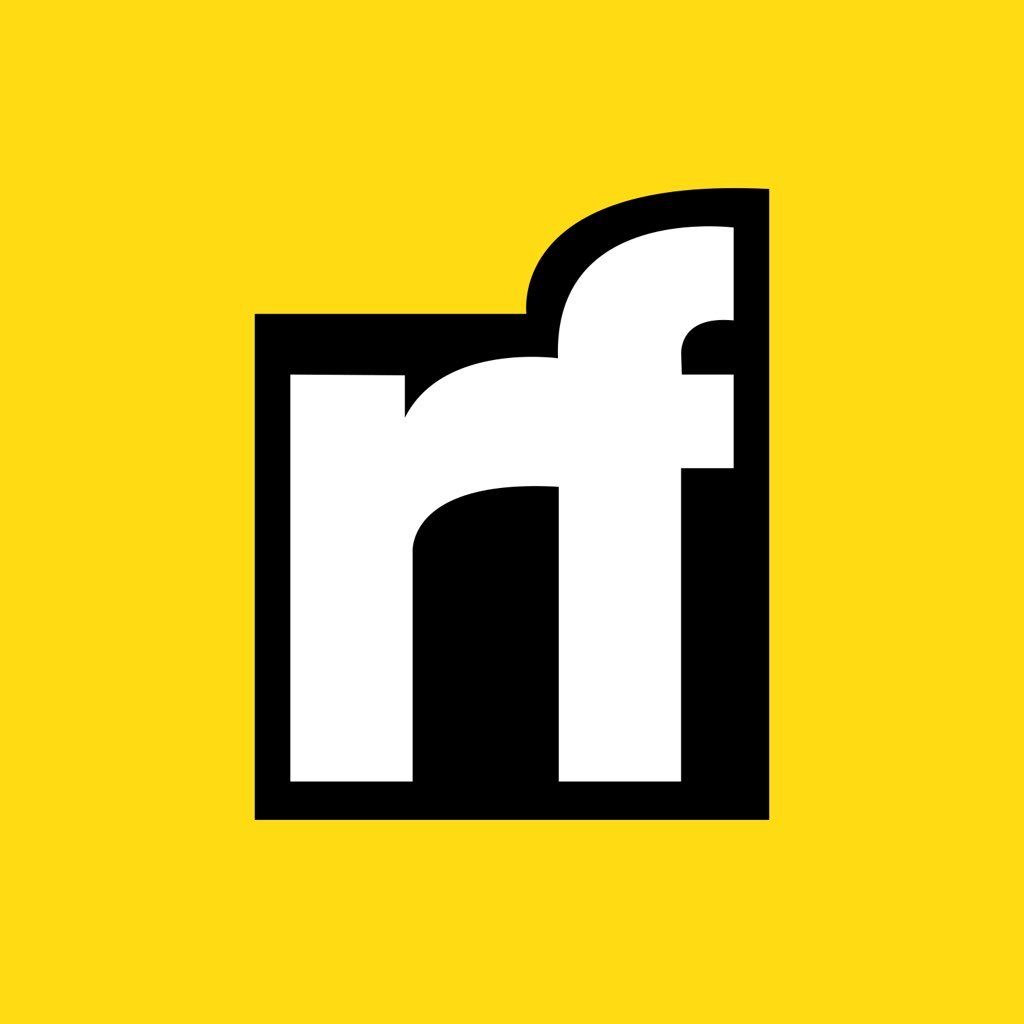
Kabilan Mahathevan | Sciencx (2023-02-21T07:01:25+00:00) Build a RESTful API with Node.js — A Step-by-Step Guide. Retrieved from https://www.scien.cx/2023/02/21/build-a-restful-api-with-node-js-a-step-by-step-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.