This content originally appeared on Level Up Coding - Medium and was authored by Open Data Analytics
Abstract Syntax Tree (AST) is a common term in software development that refers to a data structure used to represent the structure of source code in a programming language. ASTs are important because they provide a way for developers to analyze, transform, and optimize code. In this blog post, we will explore what an AST is, why it’s important, and how it can be used in software development.
What is an AST?
An AST is a tree-like data structure that represents the structure of source code in a programming language. It is generated by a parser and contains all of the information necessary to describe the structure of the code. The nodes in the tree represent the different parts of the code, such as expressions, statements, and declarations. The edges between the nodes represent the relationships between the different parts of the code.
For example, consider the following code snippet in Python:
def foo(x, y):
z = x + y
return z
The AST for this code would look like the following tree:
FunctionDef(name='foo',
args=arguments(args=[arg(arg='x', annotation=None), arg(arg='y', annotation=None)],
vararg=None, kwonlyargs=[], kw_defaults=[], kwarg=None, defaults=[]),
body=[Assign(targets=[Name(id='z', ctx=Store())],
value=BinOp(left=Name(id='x', ctx=Load()),
op=Add(),
right=Name(id='y', ctx=Load())))],
decorator_list=[],
returns=Name(id='z', ctx=Load()))
As you can see, the tree consists of nested nodes and edges that represent the structure of the code.
Why is AST important in software development?
ASTs are important because they provide a way for developers to analyze and manipulate code. They allow developers to build tools that can automatically refactor, optimize, or analyze code. For example, an AST can be used to detect unused variables, identify performance bottlenecks, and even generate documentation. ASTs can also be used to build code editors and other tools that provide syntax highlighting, code completion, and other features.
How can AST be used in software development?
ASTs can be used in many ways in software development. Some common use cases include:
1. Code optimization
ASTs can be used to identify inefficient code and suggest optimizations. For example, consider the following code snippet:
x = 1
y = 2
z = x + y
The AST for this code would look like the following tree:
Module(body=[Assign(targets=[Name(id='x', ctx=Store())], value=Constant(value=1, kind=None)),
Assign(targets=[Name(id='y', ctx=Store())], value=Constant(value=2, kind=None)),
Assign(targets=[Name(id='z', ctx=Store())],
value=BinOp(left=Name(id='x', ctx=Load()),
op=Add(),
right=Name(id='y', ctx=Load())))])
From this AST, we can see that the variables x and y are only used once, so we can optimize the code by removing them and replacing the expression with the constant value 3.
2. Code analysis
ASTs can be used to analyze code and identify potential bugs or security issues. For example, consider the following code snippet in Python:
password = input("Enter your password: ")
if password == "secret":
print("Access granted")
The AST for this code would look like the following tree:
Module(body=[Assign(targets=[Name(id='password', ctx=Store())],
value=Call(func=Name(id='input', ctx=Load()),
args=[Constant(value='Enter your password: ', kind=None)],
keywords=[])),
If(test=Compare(left=Name(id='password', ctx=Load()),
ops=[Eq()],
comparators=[Constant(value='secret', kind=None)]),
body=[Expr(value=Call(func=Name(id='print', ctx=Load()),
args=[Constant(value='Access granted', kind=None)],
keywords=[]))],
orelse=[])])
From this AST, we can see that the password is being compared directly to a string literal “secret”, which is not a secure way to handle passwords. We can use the AST to identify this issue and suggest better security practices, such as using a secure hashing algorithm to store the password.
3. Code transformation
ASTs can be used to transform code from one form to another. For example, we can use an AST to transform Python code into a different language such as JavaScript. This is useful for developers who need to write code in multiple languages.
3.1. Transpiling code from one language to another:
ASTs can be used to transform code from one language to another. For example, let’s say we have a Python code snippet that we want to transpile to JavaScript. We can use the AST to convert the Python AST to a JavaScript AST, and then generate JavaScript code from the resulting AST. Here’s an example:
import ast
import astor
code = """
x = 1
y = 2
print(x + y)
"""
tree = ast.parse(code)
# Transform the tree to a JavaScript AST
js_tree = astor.to_source(tree, 'js')
# Print the resulting JavaScript code
print(js_tree)
The output of this code would be (in Javascript):
var x = 1;
var y = 2;
console.log(x + y);
3.2. Removing unnecessary code
ASTs can also be used to remove unnecessary code from a program. For example, let’s say we have a Python function that contains a block of code that is never executed. We can use the AST to remove this block of code from the function. Here’s an example:
import ast
code = """
def foo(x):
if x > 0:
print("Positive")
else:
print("Negative")
print("Done")
"""
tree = ast.parse(code)
# Remove the second print statement
for node in ast.walk(tree):
if isinstance(node, ast.If):
node.body.pop()
# Print the resulting code
print(astor.to_source(tree))
The output of this code would be:
def foo(x):
if x > 0:
print("Positive")
else:
print("Negative")
As you can see, the second print statement has been removed from the function.
3.3. Optimizing code
ASTs can also be used to optimize code by performing various optimizations on the AST. For example, let’s say we have a Python function that contains a loop that can be optimized. We can use the AST to perform this optimization. Here’s an example:
import ast
code = """
def foo(n):
total = 0
for i in range(n):
total += i
return total
"""
tree = ast.parse(code)
# Optimize the loop
for node in ast.walk(tree):
if isinstance(node, ast.For):
node.iter = ast.Call(
ast.Name("range", ast.Load()),
[node.iter.args[0]],
[]
)
# Print the resulting code
print(astor.to_source(tree))
The output of this code would be:
def foo(n):
total = 0
total += sum(range(n))
return total
The loop has been optimized to use the sum() function instead of manually adding up the values. This can lead to faster and more efficient code.
Conclusion
In this blog post, we explored what an Abstract Syntax Tree (AST) is, why it’s important, and how it can be used in software development. ASTs provide a way for developers to analyze, transform, and optimize code, and they are used in many tools and applications. By understanding how ASTs work, developers can improve their coding skills and build better software. We hope this blog post has provided you with a good introduction to ASTs and their importance in software development.
If you have any questions or comments, please feel free to leave them below.
And one more thing, feel free to check out the GitHub Project that I am contributing to. It is an AI-empowered, Open Source Data Analysis & Visualization tool named RATH. Thank you for reading!
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Understanding Abstract Syntax Trees (AST) in Software Development was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Open Data Analytics
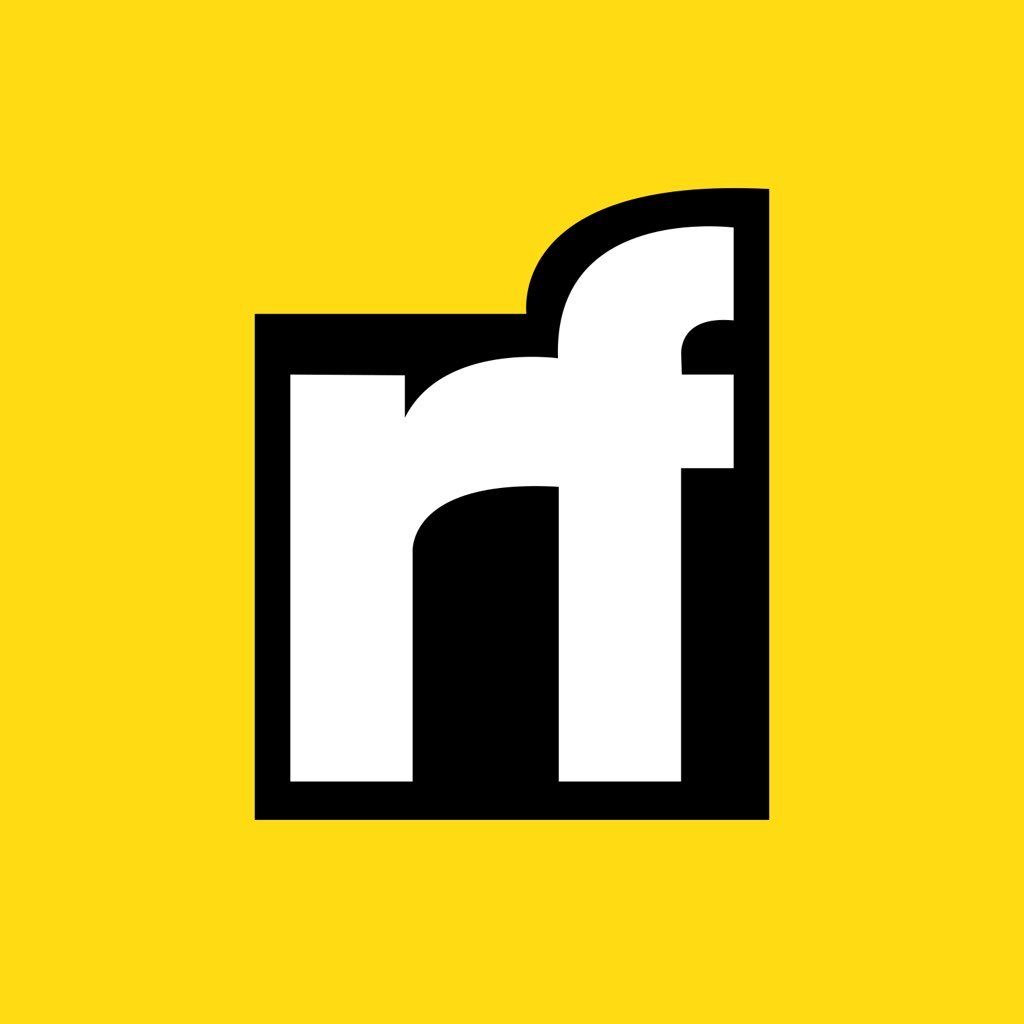
Open Data Analytics | Sciencx (2023-02-21T17:46:36+00:00) Understanding Abstract Syntax Trees (AST) in Software Development. Retrieved from https://www.scien.cx/2023/02/21/understanding-abstract-syntax-trees-ast-in-software-development/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.