This content originally appeared on Bits and Pieces - Medium and was authored by Mohammad Basit
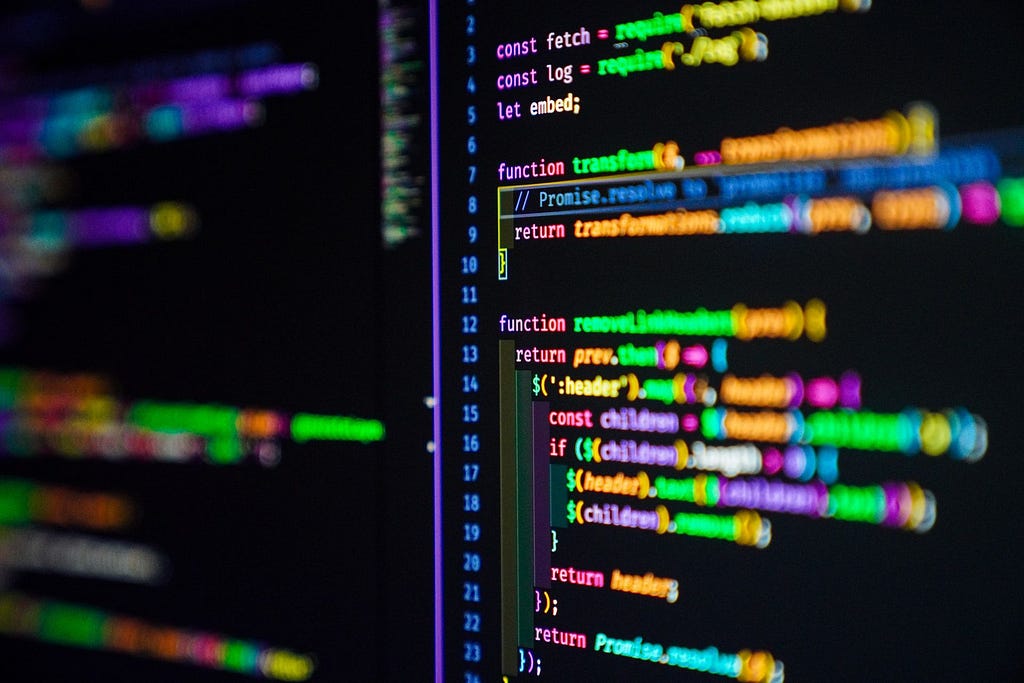
What’s a setTimeout()?
setTimeout() is a method in JavaScript that allows you to schedule a function to be executed after a specified amount of time has elapsed.
The setTimeout() method takes two parameters: a function to be executed and a delay time in milliseconds.
function sayHello() {
console.log("Hello Basit!");
}
setTimeout(sayHello, 2000); // the function will be executed after 2000 milliseconds (2 seconds)
Note
setTimeout() can be useful for executing code asynchronously, which means that it doesn't block the main thread of the browser.
Benefits
This can help prevent long-running scripts that may cause the page to become unresponsive. It can also be used for animations, timed events, and other types of tasks that require delayed execution.
How to use
To use setTimeout() to perform heavy tasks, we can break down the task into smaller chunks and execute each chunk with a delay.
Here's an example:
function heavyTask() {
console.log('heavy task started...');
let i = 0;
let chunkSize = 10;
let delay = 500; // 500 milliseconds delay
function processChunk() {
for (let j = 0; j < chunkSize; j++) {
i++;
console.log('processing item', i);
if (i === 100) {
console.log('heavy task complete.');
return;
}
}
setTimeout(processChunk, delay);
}
setTimeout(processChunk, 0);
}
heavyTask(); // CALL IT HERE
Explanation
In this example, the heavyTask() function sets up a loop that executes 100 times, with each iteration processing 10 items.
The loop is broken down into chunks of 10 items, and each chunk is executed with a delay of 500 milliseconds using setTimeout().
This allows the browser to process other pending tasks between each chunk, keeping the page responsive.
The processChunk() function is defined within the heavyTask() function, and it contains the logic for processing each chunk of items.
The function first checks if all items have been processed, and if so, it logs a message to the console indicating that the task is complete.
Otherwise, it processes the next chunk of items using a for loop and sets a setTimeout() function to execute the processChunk() function again after a delay of 500 milliseconds.
Finally, the heavyTask() function sets up the initial setTimeout() function to execute the processChunk() function immediately after the current script finishes executing (i.e., after all other pending tasks have been processed).
Points to note
- Improving page responsiveness: By breaking down the task into smaller chunks and executing each chunk with a delay, other tasks can be processed in between each chunk, keeping the page responsive.
- Preventing long-running scripts: By executing a heavy task in smaller chunks with a delay, the browser can process other tasks between each chunk, preventing the script from running for too long.
- Allowing interruption: If a user wants to interact with the page while a heavy task is running, the task can be interrupted or cancelled if it is executed in smaller chunks with a delay. This can prevent frustration and improve the overall user experience.
- Managing resources: By breaking down a heavy task into smaller chunks and executing each chunk with a delay, the browser can manage its resources more efficiently. The task can be executed in a way that maximizes the use of available resources while minimizing its impact on other tasks.
Conclusion
In conclusion, using setTimeout() to break down heavy tasks into smaller chunks can improve web performance by making pages more responsive, preventing long-running scripts, allowing interruption, and managing resources more efficiently.
By utilizing this method, developers can enhance the user experience and create a more efficient and effective website.
Build apps with reusable components like Lego
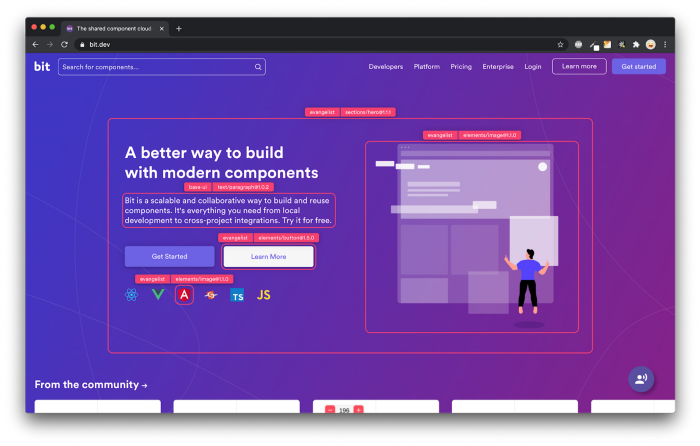
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- Bit - Component driven development
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Improving Web Performance: How to Break Down Heavy Tasks with setTimeout() was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Mohammad Basit
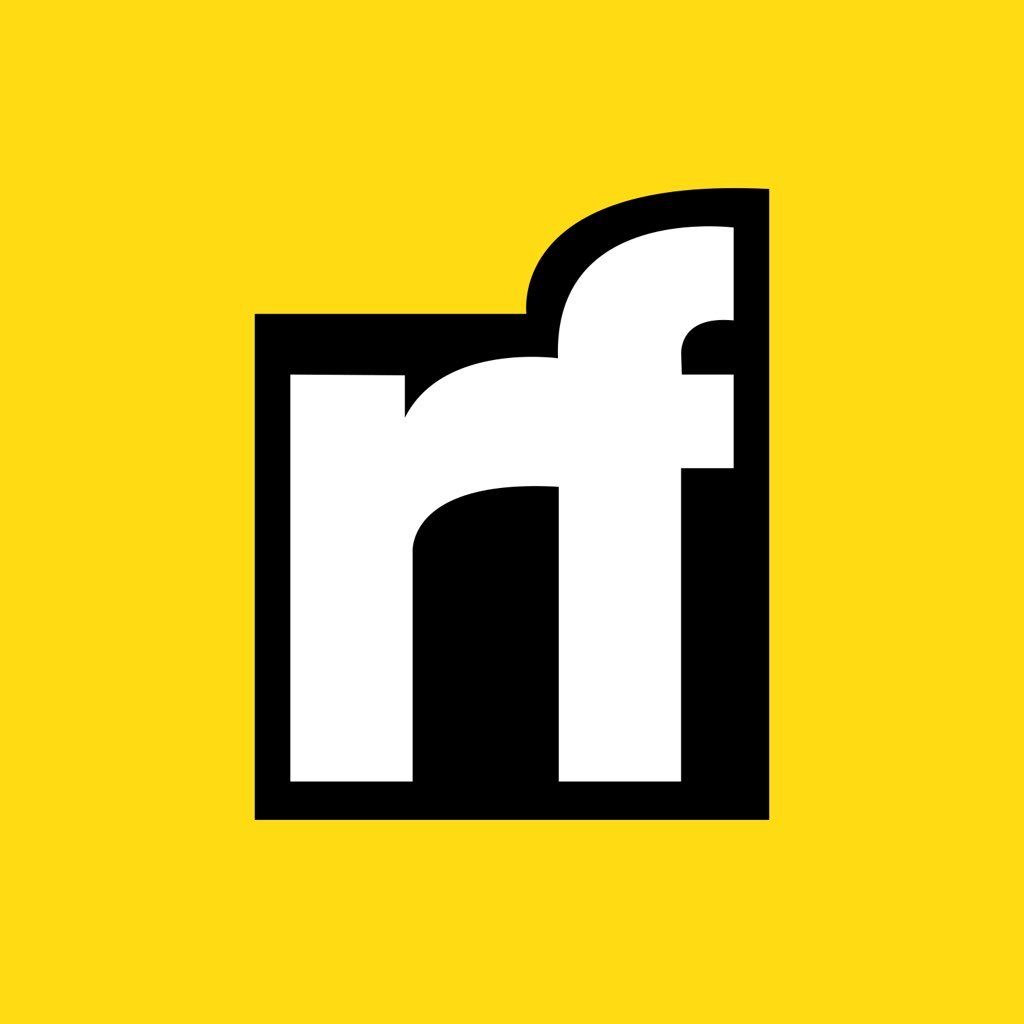
Mohammad Basit | Sciencx (2023-02-24T12:05:28+00:00) Improving Web Performance: How to Break Down Heavy Tasks with setTimeout(). Retrieved from https://www.scien.cx/2023/02/24/improving-web-performance-how-to-break-down-heavy-tasks-with-settimeout/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.