This content originally appeared on DEV Community and was authored by Kauna Hassan
For good reason, serverless architecture has become a popular approach for developing web applications in recent years. It has numerous advantages, including scalability, cost-effectiveness, and flexibility. In this article, we'll look at how to build a serverless application using React and serverless technologies like AWS Lambda, API Gateway, and DynamoDB.
Starting Over
Before we get into the specifics of creating a serverless application with React, let's first define serverless architecture and how it works.
A server is in charge of processing client requests and generating responses in a traditional web application. This responsibility is transferred to cloud services managed by third-party providers, such as AWS or Microsoft Azure, with serverless architecture.
Functions in a serverless application are deployed to the cloud and are triggered by specific events, such as an HTTP request or a database change. These functions are carried out in stateless containers that are automatically provisioned and scaled in response to demand. This makes it simple to create highly available, scalable, and cost-effective applications.
Using React to Create a Serverless Application
Now that we have a basic understanding of serverless architecture, let's look at how to build a serverless application with React, AWS Lambda, API Gateway, and DynamoDB.
Step 1: Create an AWS account and assign IAM roles.
To begin, you must first create an AWS account and assign IAM roles to your application. IAM is an acronym that stands for Identity and Access Management. It is used to manage access to AWS services.
Navigate to the IAM console in your AWS account and create a new role. Give it a name and choose AWS Lambda
as the trusted entity type. Then, associate the role with the AWSLambdaFullAccess
policy. This grants your Lambda function complete access to AWS.
Step 2 : Creating a Lambda Function
Next, let's write a Lambda function to handle client HTTP requests. Create a new function in the AWS Lambda console and select Author from scratch
. Give it a name, then choose "
Node.js 14.x
as the runtime and the role you created in Step 1
.
You can write your React application code in the function code. For example, you could handle HTTP requests and return responses using the Express.js
framework. Here's an illustration:
const express = require('express');
const app = express();
app.get('/hello', (req, res) => {
res.send('Hello, world!');
});
module.exports = app;
Step 3: Establishing an API Gateway
We need to create an API Gateway to serve as the entry point for our serverless application now that we have a Lambda function that can handle HTTP requests.
Create a new API in the AWS API Gateway console and select REST API
. Give it a name and make a new resource out of it. Then, for the resource, create a new method and select Lambda Function
as the integration type. Save the integration after selecting the Lambda function you created in Step 2
.
Step 4: Make a DynamoDB Table
Finally, in order to store data for our serverless application, we must create a DynamoDB table. Create a new table and name it in the AWS DynamoDB console. If necessary, select a partition key and a sort key. The table should then be saved.
Step 5 : Connecting the Components
Now that we have all of the components in place, we must connect them. Navigate to the Resources
tab in the AWS API Gateway console and select the resource you created in Step 3. Then, in the Integration Request
section, add a mapping template that will convert the incoming request to a format that the Lambda function can process. Here's an illustration:
{
"body": $input.json('$')
}
Next, go to the Integration Response
section and add a mapping template that will convert the Lambda function's response to a format that the client can understand. Here's an illustration:
$util.toJson($input.body)
Finally, select Deploy API
from the Actions
dropdown. This will launch the API and generate a URL from which you can access your serverless application.
Conclusion
In this article, we looked at how to build a serverless application using React and serverless technologies like AWS Lambda, API Gateway, and DynamoDB. While this may appear to be a daunting task at first, the benefits of serverless architecture make it worthwhile. You can use serverless to create highly available, scalable, and cost-effective applications without having to manage servers. So, if you're building a web application with React, think about using serverless architecture to take your app to the next level.
This content originally appeared on DEV Community and was authored by Kauna Hassan
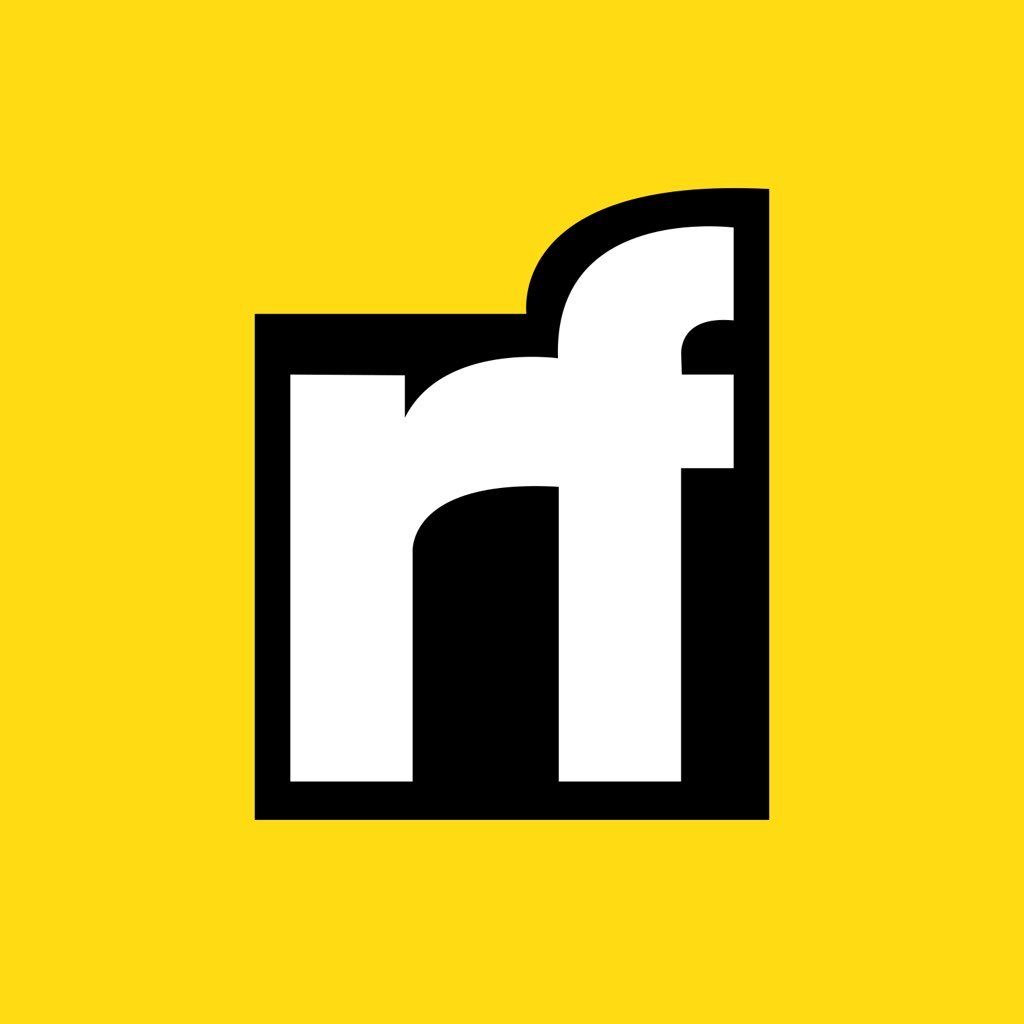
Kauna Hassan | Sciencx (2023-02-25T14:55:19+00:00) Building Serverless Applications with React. Retrieved from https://www.scien.cx/2023/02/25/building-serverless-applications-with-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.