This content originally appeared on DEV Community and was authored by Joanna
In this post we're going to talk about four more methods that will help you manipulate the DOM
.
There's a huge number of methods/properties that helps you manipulate the DOM
. Not all of them are mentioned, but only the important or commonly used ones.
prepend
The prepend()
method places a group of Node objects or DOMString objects before a parent node's first child.
<ul id="list">
<li>first item</li>
<li>second item</li>
<li>third item</li>
</ul>
const list = document.getElementById("list");
const item = document.createElement("li");
item.textContent = "fourth item";
list.prepend(item);
As you can see, the element we created using JavaScript is placed before the first child of that list.
append
The append()
method inserts a number of Node objects or DOMString objects after a parent node's final child,
<ul id="list">
<li>first item</li>
<li>second item</li>
<li>third item</li>
</ul>
const list = document.getElementById("list");
const item = document.createElement("li");
item.textContent = "fourth item";
list.append(item);
replaceChild
The replaceChild()
method is used to replace a parent node's child.
Syntax
parentNode.replaceChild(newChild, oldChild);
Using the example above we're going to replace the first item of the list.
<ul id="list">
<li>first item</li>
<li>second item</li>
<li>third item</li>
</ul>
const list = document.getElementById("list");
const item = document.createElement("li");
item.textContent = "new child item";
list.replaceChild(item, list.firstElementChild);
removeChild
The removeChild()
method is used to remove a child element from a node.
Syntax
parentNode.removeChild(childNode);
Using the same example, we're going to remove the first element in the unordered list.
<ul id="list">
<li>first item</li>
<li>second item</li>
<li>third item</li>
</ul>
const list = document.getElementById("list");
list.removeChild(list.firstElementChild);
This content originally appeared on DEV Community and was authored by Joanna
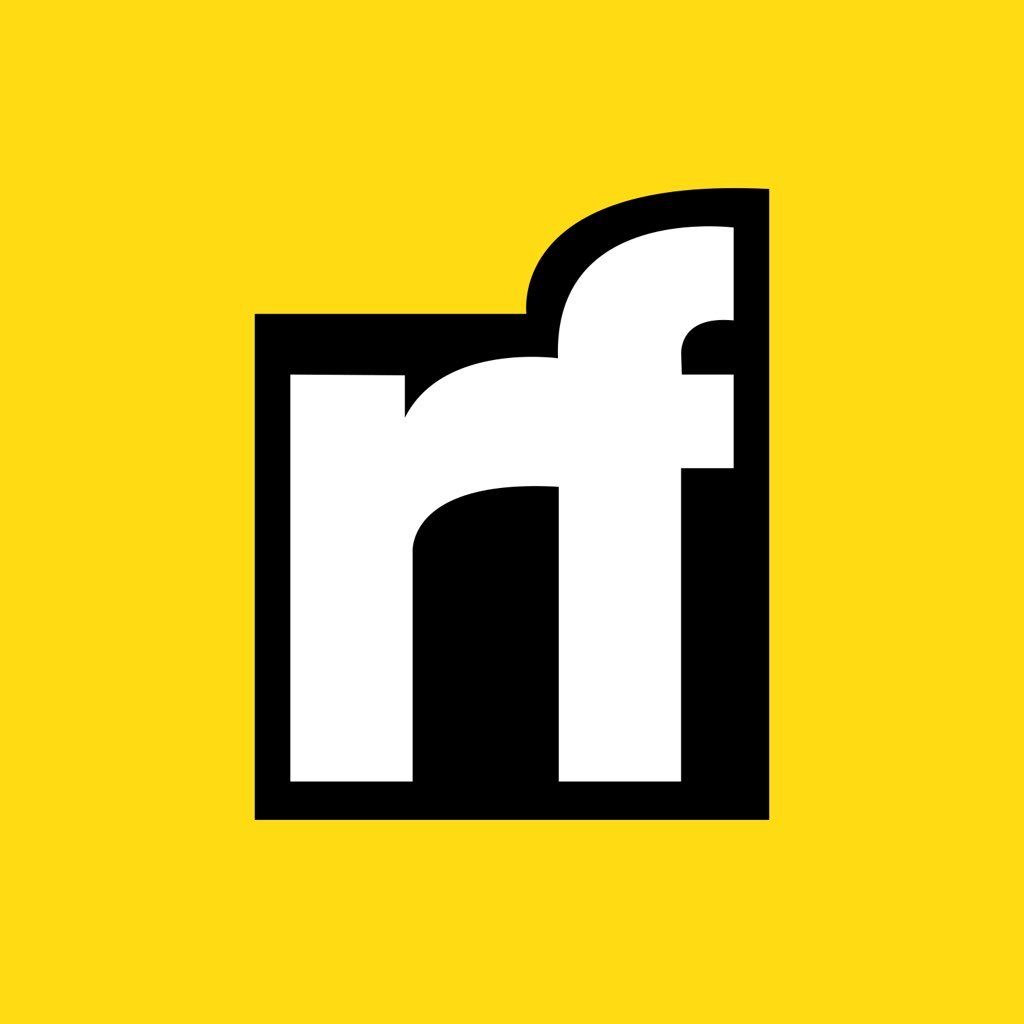
Joanna | Sciencx (2023-03-05T21:20:09+00:00) JavaScript Tutorial Series: Manipulating elements part 2.. Retrieved from https://www.scien.cx/2023/03/05/javascript-tutorial-series-manipulating-elements-part-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.