This content originally appeared on Bits and Pieces - Medium and was authored by Brian Ridolce
I have worked on numerous Next.js applications and have come across various libraries that aid in building robust and scalable applications. One such library that has recently gained a lot of popularity is Zod. Zod is a TypeScript-first schema validation library that provides a concise and type-safe way to validate inputs, outputs, and API responses. On the other hand, TypeScript’s generics provide a powerful way to create reusable code that can work with different data types. In this article, we will explore the benefits of combining Zod and generics in a Next.js application.
Overview of Zod
Before we dive into the benefits of combining Zod and generics, let’s first take a quick look at what Zod is and how it works. Zod is a TypeScript-first schema validation library that provides a concise and type-safe way to validate inputs, outputs, and API responses. Zod is designed to be easy to use and understand while providing powerful validation capabilities.
With Zod, you define your validation schema using TypeScript’s type system. For example, let’s say you have an API that returns an object with a specific set of properties. You can define a schema for this object using Zod’s object() method like this:
import { object, string, number } from 'zod';
const mySchema = object({
name: string(),
age: number(),
});
In this example, we defined a schema for an object with two properties: name, which is a string, and age, which is a number. Now, when we receive an object from our API, we can validate it using the mySchema.validate() method. If the object doesn't match the schema, Zod will throw an error.
const data = { name: 'John', age: '30' };
mySchema.validate(data); // Throws an error because age is a string instead of a number
This is just a simple example of what you can do with Zod. Zod supports many other types of validation, including arrays, enums, unions, and more. You can also create custom validators and use Zod’s chaining syntax to build complex validation schemas.
The Benefits of Combining Zod and Generics
Now that we have an understanding of what Zod is and how it works, let’s explore the benefits of combining Zod and generics in a Next.js application.
1. Improved Type Safety
Type safety is a crucial aspect of building scalable and maintainable applications. By using TypeScript and Zod, we can ensure that our data is always of the correct type and shape. However, as our application grows, we may find ourselves repeating the same validation code across multiple components and pages.
This is where TypeScript’s generics come in. Generics provide a way to create reusable code that can work with different data types. By combining Zod and generics, we can create reusable validation functions that can be used across our entire application.
For example, let’s say we have an API endpoint that returns a list of users. We can create a generic validation function that takes in a schema and a list of data to validate.
import { TypeOf, ZodType } from 'zod';
function validateList<T extends ZodType<any>>(schema: T, data: unknown[]): TypeOf<T>[] {
const parsedData = data.map((item) => schema.parse(item));
return parsedData;
}
In this example, we defined a validateList function that takes in a schema and a list of data to validate. The function uses TypeScript's TypeOf utility type to infer the type of the data based on the schema. This allows us to ensure that the return type of the function matches the expected type based on the schema.
Now, we can use this generic validation function across our entire application to validate lists of different data types.
import { object, string, number } from 'zod';
interface User {
name: string;
age: number;
}
const userSchema = object({
name: string(),
age: number(),
});
const users: unknown[] = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
];
const validatedUsers = validateList(userSchema, users); // Returns an array of User objects with correct types and shape
In this example, we defined a schema for a user object and used the validateList function to validate a list of users. The validatedUsers variable will now contain an array of user objects with the correct types and shape.
By using generics and Zod together, we can create reusable and type-safe validation functions that can be used across our entire application, improving the overall type safety of our codebase.
2. Simplified Validation Logic
Another benefit of combining Zod and generics is simplified validation logic. As our application grows, we may find ourselves with complex validation logic that is difficult to understand and maintain. By using Zod’s schema validation and TypeScript’s generics, we can simplify our validation logic and make it easier to understand.
For example, let’s say we have a form that requires multiple fields to be validated. We can create a generic validation function that takes in a schema and a data object to validate.
import { TypeOf, ZodType } from 'zod';
function validateObject<T extends ZodType<any>>(schema: T, data: unknown): TypeOf<T> {
const parsedData = schema.parse(data);
return parsedData;
}
In this example, we defined a validateObject function that takes in a schema and a data object to validate. The function uses TypeScript's TypeOf utility type to infer the type of the data based on the schema. This allows us to ensure that the return type of the function matches the expected type based on the schema.
Now, we can use this generic validation function to simplify the validation logic of our form.
import { object, string, number } from 'zod';
interface FormValues {
firstName: string;
lastName: string;
age: number;
}
const formSchema = object({
firstName: string(),
lastName: string(),
age: number(),
});
function onSubmit(formData: unknown) {
const validatedData = validateObject(formSchema, formData);
// Do something with validatedData
}
In this example, we defined a schema for our form and used the validateObject function to validate the form data. The validatedData variable will now contain the form data with the correct types and shape.
By using generics and Zod together, we can simplify our validation logic and make it easier to understand and maintain.
3. Increased Code Reusability
Finally, combining Zod and generics can lead to increased code reusability. As we mentioned earlier, generics provide a powerful way to create reusable code that can work with different data types. By using Zod’s schema validation and TypeScript’s generics, we can create reusable validation functions that can be used across our entire application.
For example, let’s say we have a Next.js application that has multiple pages with forms that require validation. We can create a reusable validation function that takes in a schema and form data to validate.
import { TypeOf, ZodType } from 'zod';
export function validateFormData<T extends ZodType<any>>(schema: T,
formData: unknown
): TypeOf<T> {
const parsedData = schema.parse(formData);
return parsedData;
}
In this example, we created a `validateFormData` function that takes in a schema and form data to validate. The function uses TypeScript’s `TypeOf` utility type to infer the type of the data based on the schema. This allows us to ensure that the return type of the function matches the expected type based on the schema. Now, we can use this reusable validation function across our entire application to validate different forms.
import { object, string, number } from 'zod';
import { validateFormData } from '../utils/validation';
interface LoginFormValues {
username: string;
password: string;
}
const loginFormSchema = object({
username: string(),
password: string(),
});
function onLogin(formData: unknown) {
const validatedData = validateFormData(loginFormSchema, formData);
// Do something with validatedData
}
interface SignUpFormValues {
email: string;
password: string;
confirmPassword: string;
}
const signUpFormSchema = object({
email: string(),
password: string(),
confirmPassword: string(),
});
function onSignUp(formData: unknown) {
const validatedData = validateFormData(signUpFormSchema, formData);
// Do something with validatedData
}
In this example, we defined two different schemas for a login form and a sign-up form, and used the validateFormData function to validate the form data. We can reuse the validateFormData function across our entire application to validate different forms, reducing code duplication and improving maintainability.
By combining Zod and generics, we can create reusable and type-safe validation functions that can be used across our entire application, improving the overall code reusability and maintainability.
💡 Note: If you find yourself building similar forms over and over, you should create a general-purpose Zod validation function that matches most use cases, and share it to an open-source platform like Bit so you can reuse it across projects with a simple npm install. Learn more about this here.
Conclusion
In this article, we explored the benefits of combining Zod and generics in a Next.js application. We showed how Zod’s schema validation and TypeScript’s generics can work together to create reusable and type-safe validation functions that can be used across the entire application.
By using generics and Zod together, we can create more type-safe, simplified, and reusable code. This can lead to a more maintainable and scalable application, with fewer bugs and less code duplication.
As a software engineer, it’s important to stay up-to-date with the latest tools and techniques to improve your code quality and productivity. By using Zod and generics in your Next.js application, you can take advantage of the benefits of type safety, simplified validation logic, and increased code reusability.
Build Apps with reusable components, just like Lego
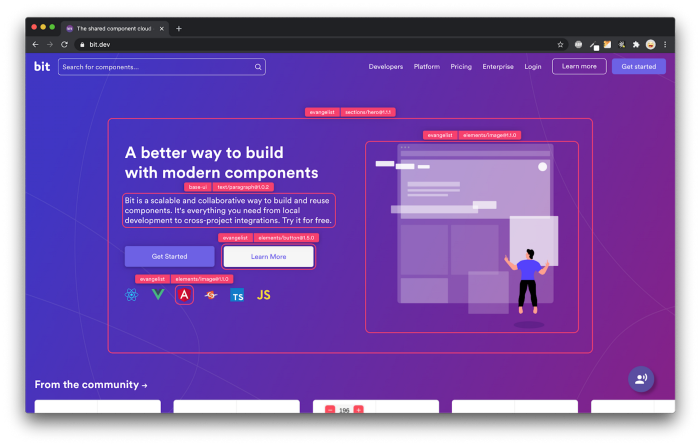
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
The Benefits of Combining Zod and Generics Type in Next.js Applications was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Brian Ridolce
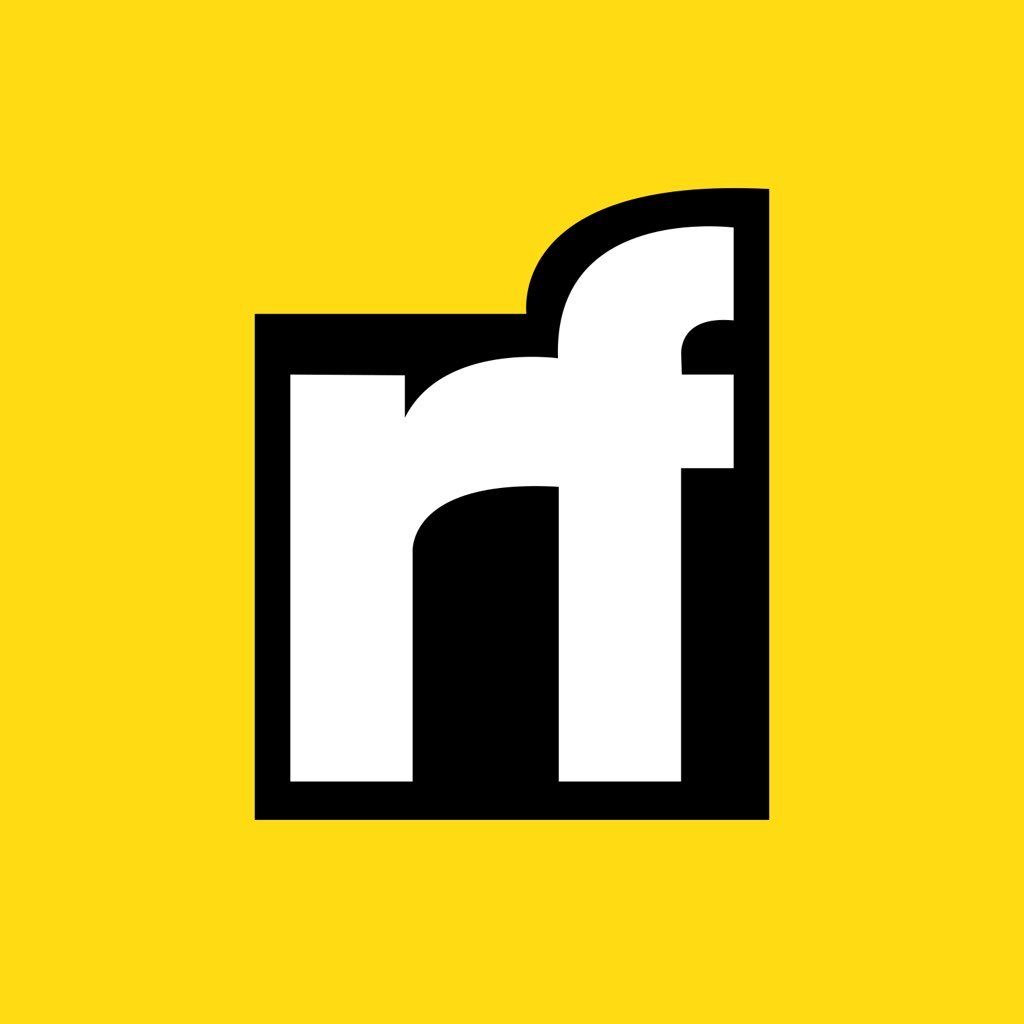
Brian Ridolce | Sciencx (2023-03-06T09:02:14+00:00) The Benefits of Combining Zod and Generics Type in Next.js Applications. Retrieved from https://www.scien.cx/2023/03/06/the-benefits-of-combining-zod-and-generics-type-in-next-js-applications/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.