This content originally appeared on Bits and Pieces - Medium and was authored by Mohammad Basit
Exploring the Limits of Web Workers: How Much Processing Power Can You Harness?
Have you ever used a computer program that seems to freeze or become unresponsive when it’s doing a lot of work? That’s because the program is busy with a lot of tasks, and it can’t respond to user input until it’s finished.
Web workers are a way for web developers to prevent this problem from happening in web applications. Essentially, a web worker is a separate “thread” of code that runs in the background, independently of the main thread that controls the user interface.
By running code in a web worker, web developers can prevent the main thread from becoming unresponsive, even if the web app is doing a lot of work. This means that the user can continue to interact with the app even while it’s doing complex operations in the background.
To put it simply, web workers are like helpers that work behind the scenes to keep your web app running smoothly and prevent it from freezing up.
Use case
Let’s say you have a web app that needs to perform a complex mathematical calculation that takes a long time to complete. If you run this calculation in the main thread of the web app, it could cause the user interface to freeze or become unresponsive.
You can use a web worker to perform the calculation in the background. Here’s how it works:
Steps to define a web worker
In your web app code, you create a new web worker by calling the Worker() constructor and passing in the name of a JavaScript file that contains the code to be run in the worker.
let myWorker = new Worker("web_worker.js");
In the web_worker.js file, you write the code that performs the calculation. For example, you could write a function that calculates the factorial of a large number:
function calculateFactorial(n) {
if (n === 0) {
return 1;
} else {
return n * calculateFactorial(n-1);
}
}
In the web app code, you set up an event listener to receive messages from the worker. This allows the worker to send messages back to the main thread with the results of the calculation.
myWorker.onmessage = function(event) {
console.log("Result: " + event.data);
}
Finally, in the web app code, you send a message to the worker with the data it needs to perform the calculation.
myWorker.postMessage({ number: 10 });
The worker receives the message and performs the calculation, then sends a message back to the main thread with the result.
onmessage = function(event) {
var result = calculateFactorial(event.data.number);
postMessage(result);
}
Once the calculation is complete, the worker sends the result back to the main thread, where it can be displayed to the user.
Where can it be used?
- Image and video processing: Web workers can help web apps handle big image and video jobs, like making pictures smaller or changing their colors, without slowing down the rest of the website. This way, users can still do things on the website while those jobs are happening in the background.
- Game development: Web workers can help games run smoothly by doing complicated math problems and physics calculations without making the game slow down or stop working.
- Data visualization: Web workers can help websites show big charts and graphs without making the website slow down or stop working. This way, users can still use the website while the charts and graphs are being made in the background.
- Machine learning: Web workers can be used to run machine learning algorithms in the browser, allowing web apps to perform tasks such as image recognition and natural language processing without relying on external APIs.
- Real-time communication: Web workers can help websites talk to other websites without making the website slow down or stop working. This way, users can still use the website while it’s talking to other websites in the background.
- Background tasks: Web workers can help websites do things in the background, like saving information, checking for new updates, and talking to other computers, without making the website slow down or stop working. This way, users can still use the website while these things are happening in the background.
Conclusion
By using web workers, web apps can continue to respond to user input and update the user interface while these tasks are being performed in the background, resulting in a smoother and more responsive user experience.
Build Apps with reusable components, just like Lego
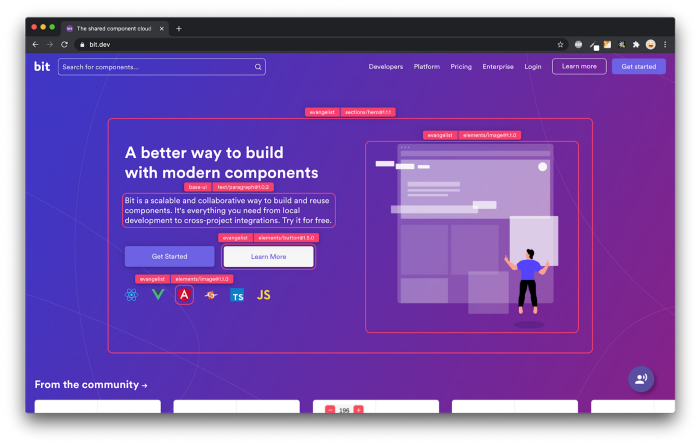
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Web Workers: How Much Processing Power Can You Harness? was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Mohammad Basit
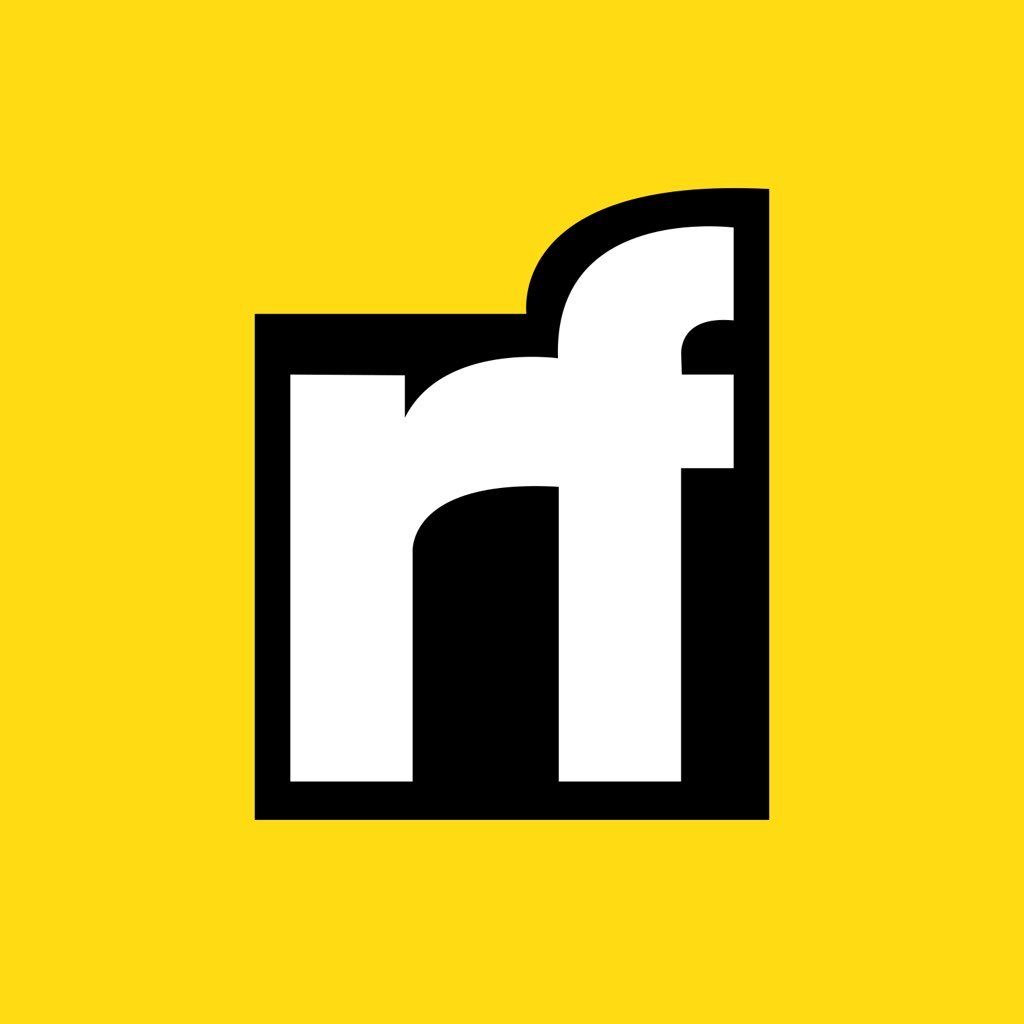
Mohammad Basit | Sciencx (2023-03-06T06:39:15+00:00) Web Workers: How Much Processing Power Can You Harness?. Retrieved from https://www.scien.cx/2023/03/06/web-workers-how-much-processing-power-can-you-harness/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.