This content originally appeared on Level Up Coding - Medium and was authored by Ulrik Thyge Pedersen
Risk of Rust — Part 2: Syntax, Data Types and Control Flow
An Introduction to the Core Building Blocks of Rust Programming
Explore “Risk of Rust,” a series on the modern programming language Rust that combines speed and safety for writing efficient and resilient code. From syntax basics to advanced topics like concurrency and memory management, we’ll guide you through practical examples and real-world applications in game development, web development, and operating systems. Whether you’re new to programming or experienced, learn how Rust can help you write safe and fast code for complex systems.
Introduction
Welcome to the second article in our “Risk of Rust” series. In our previous article, “Introduction to Rust and Installing Rust,” we covered the basics of Rust programming language and how to set up Rust on various operating systems. We learned about Rust’s performance benefits, memory safety guarantees, and its robust package manager, Cargo. In this article, we’ll take a closer look at Rust’s syntax, data types, and control flow constructs. We’ll explore the building blocks of Rust programs, including variables, functions, loops, and conditionals.
Understanding Rust’s syntax and data types is crucial for any Rust programmer. Rust is designed to be a fast and safe systems programming language, and its syntax reflects that goal.
Rust’s syntax is concise and expressive, with a focus on clarity and readability.
Rust’s data types are powerful and flexible, allowing for efficient memory usage and high performance.
In the following sections, we’ll explore Rust’s syntax and data types in more detail. We’ll start with variables and data types, then move on to control flow constructs like loops and conditionals. By the end of this article, you’ll have a solid understanding of the basic building blocks of Rust programs.
Let’s get started!
Rust Syntax
Rust has a concise and expressive syntax that combines ideas from functional programming with low-level systems programming. In this section, we’ll explore Rust’s syntax and cover some of the basic constructs that you’ll need to know to start writing Rust code.
Variables and Mutability
In Rust, variables are immutable by default, meaning that once you assign a value to a variable, you cannot change that value. To create a mutable variable, you need to use the mut keyword:
In this example, we start by declaring an immutable variable x with the value 5. We then declare a mutable variable y with the value 10. We print the values of x and y, and then change the value of y to 15. We print the new value of y.
Next, we declare a new immutable variable x with the value "Hello, world!". This demonstrates variable shadowing, where we can declare a new variable with the same name as an existing variable. The new variable shadows the old one, meaning that we can't access the old variable anymore.
This example shows how we can declare both immutable and mutable variables in Rust, and how we can use variable shadowing to declare new variables with the same name.
Data Types
Rust has a strong, static type system that ensures memory safety and thread safety, and prevents a wide range of programming errors at compile-time. Rust’s basic data types include:
- Booleans: The bool type represents a boolean value, either true or false
- Characters: The char type represents a single Unicode character, and is denoted by single quotes
- Numeric types: Rust provides a variety of numeric types, including signed and unsigned integers of various sizes, as well as floating-point numbers
- Arrays: An array is a fixed-size collection of elements of the same type
- Tuples: A tuple is an ordered collection of elements of different types
- Option and Result types: Rust provides two special types, Option<T> and Result<T, E>, to handle cases where a value may be absent or an operation may fail
In addition to these basic data types, Rust also provides a variety of advanced data structures, such as Vec, String, HashMap, HashSet, enum, struct, and trait.
Control Flow
Control flow constructs in Rust are similar to other programming languages. Rust supports if/else, loop, while, for, and match expressions.
if/else
The if statement in Rust works the same way as in other languages. Here's an example:
The else clause is optional.
loop
The loop statement is used to create an infinite loop. Here's an example:
This will print the numbers from 1 to 5.
while
The while loop is used to execute a block of code as long as a certain condition is true. Here's an example:
This will print the numbers from 1 to 5.
for
The for loop is used to iterate over a collection of items. Here's an example:
This will print the numbers from 1 to 5.
match
The match expression is used to match a value against a pattern and execute the corresponding code. Here's an example:
This will print “the answer to life, the universe, and everything”.
The _ pattern is used as a catch-all for patterns that are not explicitly matched.
Putting the pieces together
Let’s apply our knowledge by creating a simple Rust script that checks if numbers are even or odd, utilizing the concepts we’ve learned so far!
In this example, we define a function is_even that takes an integer as input and returns a boolean indicating whether the number is even or odd. We then define several variables of different types, including an integer num, a mutable integer count, a string name, and a boolean is_rust_cool.
We use println! to print the values of these variables to the console. We also show how to change the value of a mutable variable using the assignment operator.
Next, we use an if-else statement to check if a number is even or odd using the is_even function we defined earlier. Finally, we use a match statement to determine the message to print based on the value of the count variable. This demonstrates Rust's powerful pattern matching capabilities.
Conclusion
In conclusion, we’ve covered the basics of Rust syntax, data types, and control flow constructs. We learned about variables and mutability, data types like integers, floats, booleans, and strings, as well as control flow constructs like if/else statements and loops.
By practicing with the code example, we gained a better understanding of how to use these concepts together in a real-world scenario. We also explored Rust’s powerful match control flow construct and how it can be used to handle complex logic.
In the next article in this series, we will dive into Rust’s ownership system and explore advanced data structures like structs. We will also learn about borrowing and lifetimes, which are crucial concepts for understanding Rust’s approach to memory safety. Stay tuned!
Thank you for reading my story!
Subscribe for free to get notified when I published a new story!
Find me on LinkedIn and Kaggle!
…and I would love your feedback!
Risk of Rust — Part 2: Syntax, Data Types and Control Flow was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Ulrik Thyge Pedersen
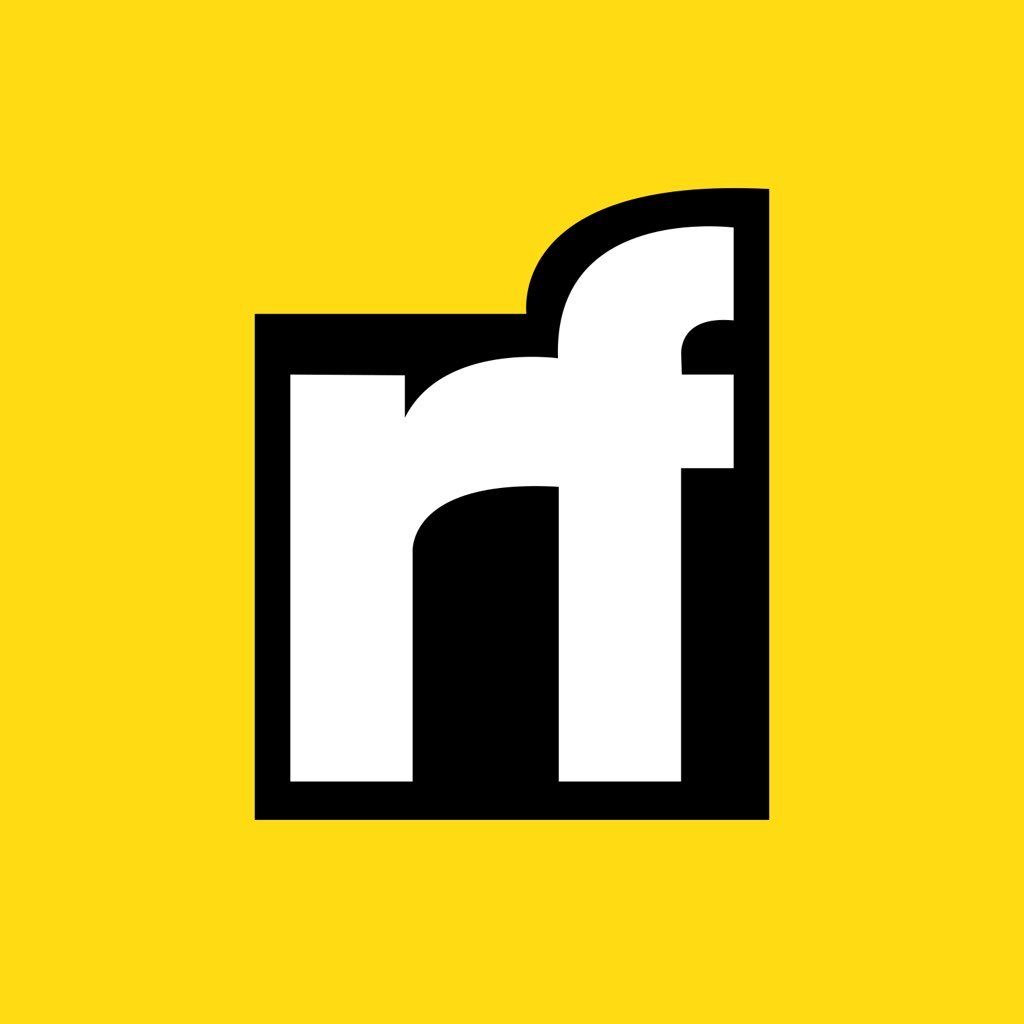
Ulrik Thyge Pedersen | Sciencx (2023-04-06T02:46:12+00:00) Risk of Rust — Part 2: Syntax, Data Types and Control Flow. Retrieved from https://www.scien.cx/2023/04/06/risk-of-rust-part-2-syntax-data-types-and-control-flow/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.