This content originally appeared on Bits and Pieces - Medium and was authored by FardaKarimov
In this article, we will explore seven cool and modern JavaScript features that most developers may not be aware of.
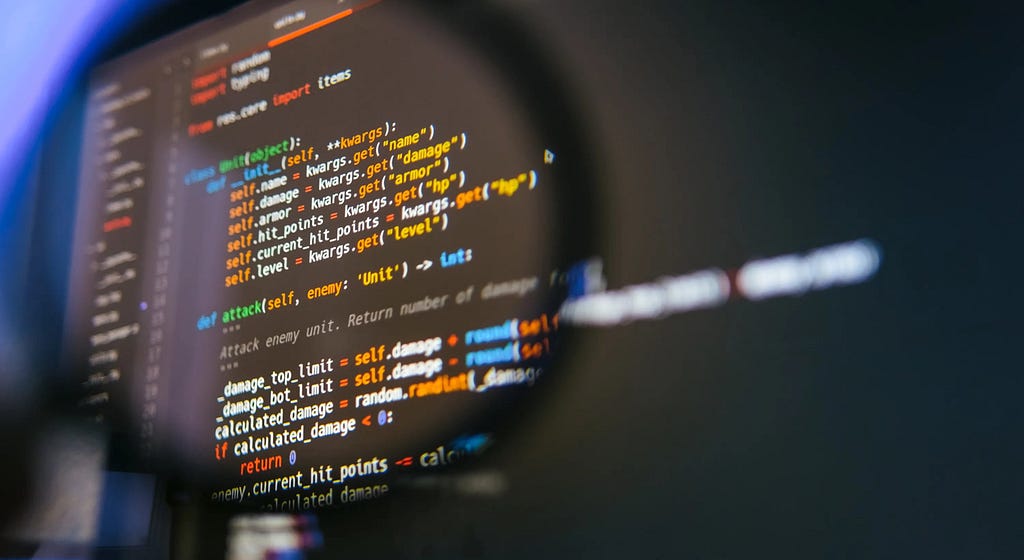
1. Optional Chaining
Optional chaining is a new feature in JavaScript that allows developers to write code that is more concise and easier to read. With optional chaining, you can access nested properties of an object without worrying about whether or not the properties exist.
const user = {
name: 'John',
address: {
city: 'New York',
state: 'NY'
}
};
console.log(user?.address?.city); // Output: New York
console.log(user?.address?.zipCode); // Output: undefined
In the above example, we are using the optional chaining operator (?.) to access the city property of the address object. If the address object did not exist, or if it did not have a city property, the code would simply return undefined.
2. Nullish Coalescing Operator
The nullish coalescing operator (??) is another new feature in JavaScript that can be used to provide a default value for variables that may be null or undefined.
const name = null ?? 'John';
console.log(name); // Output: John
In the above example, we are using the nullish coalescing operator to assign a default value of ‘John’ to the name variable, since its initial value is null.
3. Promise.allSettled()
The Promise.allSettled() method is a new addition to the Promise API in JavaScript. It allows developers to run multiple promises simultaneously and get the results of all of them, regardless of whether they resolve or reject.
const promises = [
Promise.resolve(1),
Promise.reject('Error'),
Promise.resolve(3)
];
Promise.allSettled(promises)
.then(results => console.log(results));
// Output:
// [
// { status: 'fulfilled', value: 1 },
// { status: 'rejected', reason: 'Error' },
// { status: 'fulfilled', value: 3 }
// ]
In the above example, we are using the Promise.allSettled() method to run three promises simultaneously, and then logging the results of all of them, including the ones that rejected.
4. Object.fromEntries()
The Object.fromEntries() method is a new addition to the Object API in JavaScript. It allows developers to create an object from an array of key-value pairs.
const entries = [
['name', 'John'],
['age', 30],
['city', 'New York']
];
const obj = Object.fromEntries(entries);
console.log(obj); // Output: { name: 'John', age: 30, city: 'New York' }
In the above example, we are using the Object.fromEntries() method to create an object from an array of key-value pairs.
5. BigInt
The BigInt data type is a new addition to JavaScript that allows developers to work with integers that are larger than the maximum value supported by the Number data type.
const a = BigInt(9007199254740991);
const b = BigInt(9007199254740991);
console.log(a + b); // Output: 18014398509481982n
In the above example, we are using BigInt to add two very large numbers.
6. Optional Catch Binding
Optional catch binding is a new feature in JavaScript that allows developers to catch errors without requiring a parameter. This can make code more concise and easier to read.
try {
// some code that may throw an error
} catch {
// handle the error without a parameter
}
In the above example, we are using the optional catch binding to catch errors without specifying a parameter. This can be useful if you do not need to use the error object in your catch block.
7. Array.prototype.flatMap()
The Array.prototype.flatMap() method is a new addition to the Array API in JavaScript. It allows developers to map and then flatten an array in a single step.
const arr = [1, 2, 3, 4];
const result = arr.flatMap(x => [x * 2]);
console.log(result); // Output: [2, 4, 6, 8]
In the above example, we are using the Array.prototype.flatMap() method to multiply each element of the array by 2, and then flatten the resulting array into a single array.
Conclusion
These are just a few of the many cool and modern JavaScript features that are available to developers. By keeping up with the latest updates to the language, you can write more efficient and concise code, and make use of new tools and techniques that can help you be a better developer.
Build Apps with reusable components, just like Lego
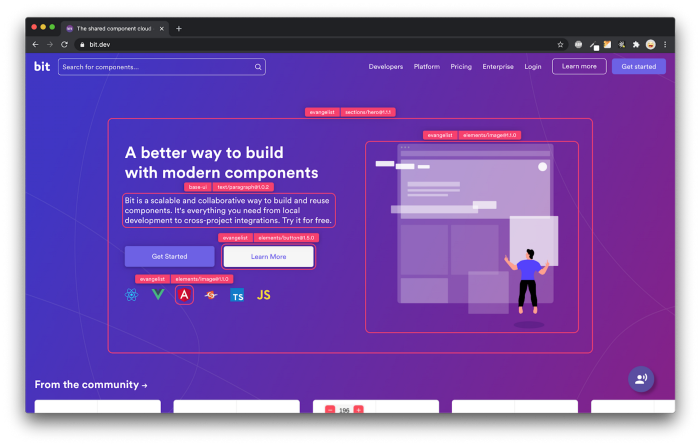
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
7 Modern and Powerful JavaScript Features You Didn’t Know About was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by FardaKarimov
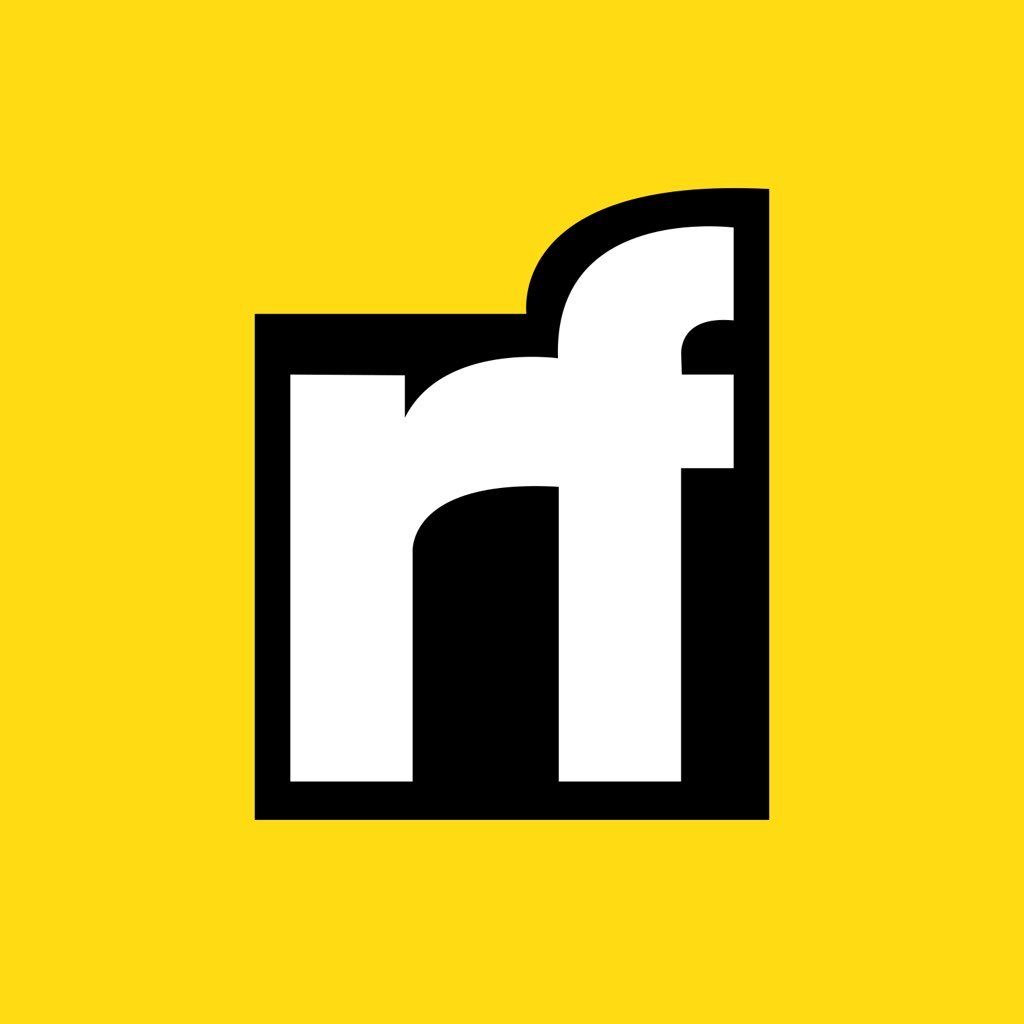
FardaKarimov | Sciencx (2023-04-18T08:09:12+00:00) 7 Modern and Powerful JavaScript Features You Didn’t Know About. Retrieved from https://www.scien.cx/2023/04/18/7-modern-and-powerful-javascript-features-you-didnt-know-about/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.