This content originally appeared on Bits and Pieces - Medium and was authored by Ben Mishali
Explore the importance of scheduling tasks in Node.js, its various use cases, code examples using third-party packages
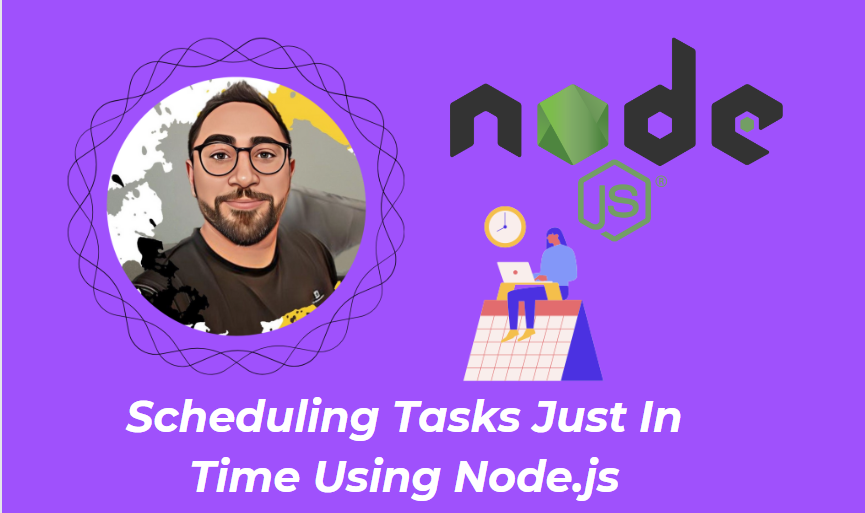
Introduction
The rapidly evolving world of web development has given rise to a plethora of powerful and versatile tools. Among these is Node.js, a popular JavaScript runtime environment that has helped developers streamline their workflows and create efficient applications. One of its many useful features is task scheduling. This article will explore the importance of scheduling tasks in Node.js, its various use cases, code examples using third-party packages like Agenda, and some helpful do’s and don’ts to follow.
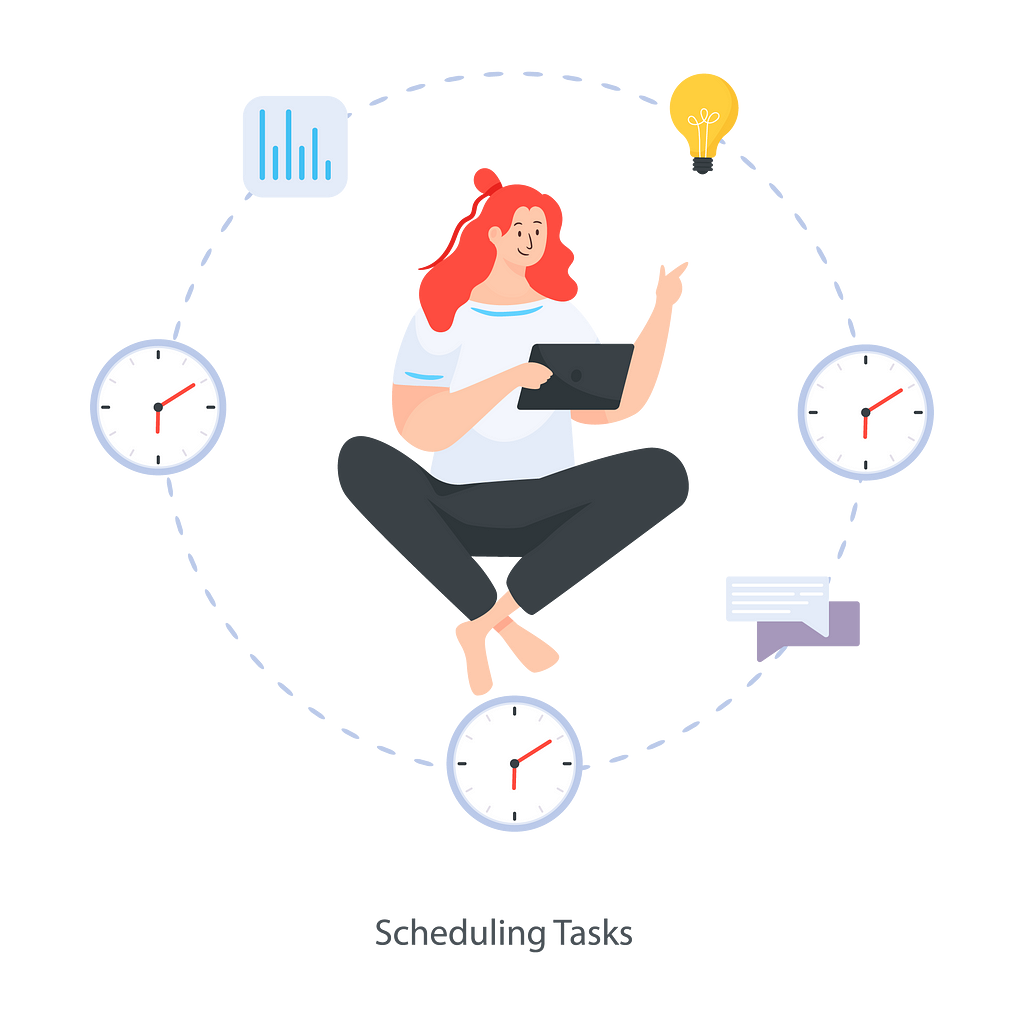
Why We Need Scheduling Tasks
Scheduling tasks is essential for ensuring that certain events occur at specific times or intervals, automating repetitive processes, and managing resources effectively.
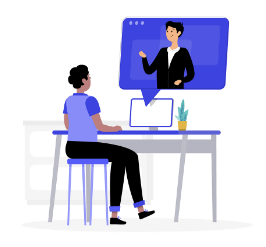
In the context of Node.js, scheduling tasks allows developers to:
- Perform time-sensitive operations, such as sending reminders or notifications.
- Automatically update data or generate reports at regular intervals.
- Manage long-running processes without affecting the overall performance of the application.
- Balance workloads and optimize resource usage.
Use Cases
Some common use cases of scheduling tasks in Node.js include:
- Email and SMS notifications: Sending reminders or alerts to users at predetermined times.
- Data backup and synchronization: Performing regular backups or synchronizing data across multiple databases.
- Report generation: Generating reports or analytics data at specific intervals for business intelligence or monitoring purposes.
- System maintenance: Running routine maintenance tasks like database cleanup, cache invalidation, or log rotation.
- Miscellaneous: Run any task you want whenever you want in a dynamic and independent way
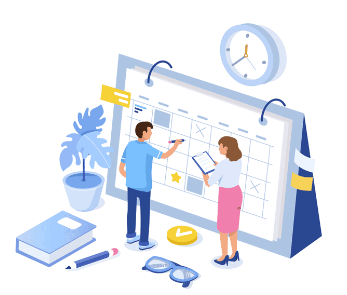
Code Examples Using 3rd-Party packages
Agenda
Agenda is a popular, lightweight job scheduling library for Node.js that uses MongoDB as a backend for persistence.
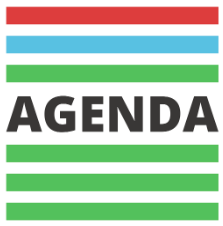
Let’s explore how to use Agenda to schedule tasks in a Node.js application.
Agenda is a flexible and powerful job scheduling library for Node.js that simplifies the process of creating, managing, and executing scheduled tasks. Some of its key features include:
- Persistence: Agenda uses MongoDB as a backend for storing job data, ensuring that scheduled tasks are not lost during server restarts or crashes. This provides a level of fault tolerance and consistency that is often lacking in memory-based schedulers.
- Event-driven architecture: Agenda provides an event-based API for handling job events, such as job completion, failure, or success. This makes it easy for developers to react to various job states and implement custom logic accordingly.
- Job concurrency and locking: Agenda allows you to control the concurrency of job execution, ensuring that multiple instances of the same job don’t run simultaneously. This is particularly useful when dealing with tasks that require exclusive access to shared resources.
- Flexible scheduling: Agenda supports various scheduling options, including one-time tasks, recurring tasks with fixed intervals, and cron-style expressions for more complex scheduling requirements.
- Job prioritization: Agenda allows you to set job priorities, ensuring that more critical tasks are executed before less critical ones. This can help improve the overall performance of your application by prioritizing important tasks.
- Job retries and backoff: Agenda supports configurable retry strategies, including exponential backoff, for failed jobs. This allows you to gracefully handle job failures and improve the reliability of your application.
- Time zone support: Agenda can be combined with other libraries, such as Moment.js, to handle time zones effectively. This ensures that your scheduled tasks are executed accurately, regardless of server location or daylight saving time changes.
- Middleware integration: Agenda can be easily integrated into existing Node.js applications, such as Express.js or Koa.js, allowing you to manage scheduled tasks alongside other application components seamlessly.
First, install Agenda and its MongoDB dependency:
npm install agenda mongodb
Next, create a new file called agendaJobs.js and configure Agenda:
const Agenda = require('agenda');
const MongoClient = require('mongodb').MongoClient;
const connectionString = 'mongodb://localhost/agenda';
(async function() {
const client = await MongoClient.connect(connectionString, { useNewUrlParser: true, useUnifiedTopology: true });
const agenda = new Agenda({ mongo: client.db('agenda') });
// Define tasks
agenda.define('send email', async (job, done) => {
console.log('Sending email...');
done();
});
// Schedule tasks
await agenda.start();
agenda.every('1 hour', 'send email');
})();
In the example above, we define a task called ‘send email’ and schedule it to run every hour. You can replace the email sending logic with your implementation.
node-cron
Another popular library for scheduling tasks in Node.js is node-cron. It allows you to create cron jobs with a familiar cron syntax, making it easy to schedule tasks at specific intervals or times.
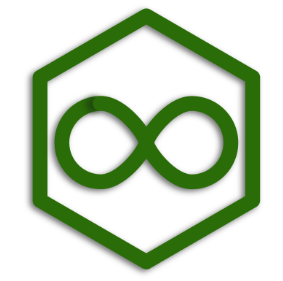
Here's an example of how to use node-cron to schedule a task that sends an email every day at 8 AM.
First, install node-cron:
npm install node-cron
Next, create a new file called cronJobs.js and configure node-cron:
const cron = require('node-cron');
// Define your task
const sendEmail = () => {
console.log('Sending email...');
};
// Schedule the task
const job = cron.schedule('0 8 * * *', sendEmail, {
scheduled: false,
timezone: 'Asia/Jerusalem',
});
// Start the job
job.start();
In this example, we define a function called sendEmail that contains our email sending logic. We then use node-cron to schedule this function to run every day at 8 AM, according to the Asia/Jerusalem timezone.
node-cron is an excellent library for developers familiar with cron syntax, as it provides a simple and intuitive API for scheduling tasks in Node.js. Its support for custom time zones and various scheduling options makes it a versatile choice for a wide range of use cases.
Bull
Another useful library for scheduling tasks in Node.js is Bull. Bull is a powerful and fast job queue library that uses Redis as a backend for persistence and job management. It allows you to create, schedule, and process jobs in a distributed and scalable manner.
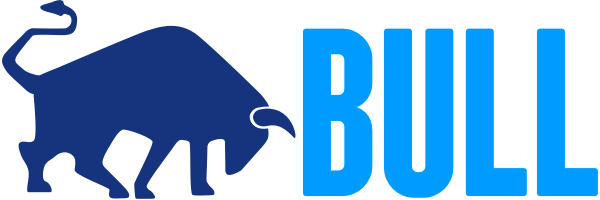
Here's an example of how to use Bull to schedule a task that sends an email every day at 8 AM.
First, install Bull and its Redis dependency:
npm install bull ioredis
Next, create a new file called bullJobs.js and configure Bull:
const Bull = require('bull');
const { setQueues, UI } = require('bull-board');
const Redis = require('ioredis');
const express = require('express');
const emailQueue = new Bull('emailQueue', {
createClient: () => new Redis(),
});
// Define your task
emailQueue.process(async (job) => {
console.log('Sending email...');
});
// Schedule the task
const scheduleEmail = async () => {
await emailQueue.add(
{},
{ repeat: { cron: '0 8 * * *', tz: 'Asia/Jerusalem' } }
);
};
scheduleEmail();
// Set up Bull Board (optional)
const app = express();
setQueues([emailQueue]);
app.use('/admin/queue', UI);
app.listen(3000, () => console.log('Bull board listening on port 3000'));
In this example, we first create an instance of the Bull queue called emailQueue. Then, we define a function called process that contains our email sending logic. We use the add method to schedule the task, providing an empty object as the job data and a repeat object with the cron expression and tz (timezone) properties.
The cron expression '0 8 * * *' translates to "every day at 8:00 AM". The timezone used in this example is 'Asia/Jerusalem'. The scheduleEmail function is called to schedule the task.
Additionally, we set up Bull Board, a simple dashboard to monitor and manage Bull queues. We create an Express application, set up the Bull Board middleware, and start listening on port 3000.
Bull is a powerful and flexible library that provides advanced features, such as job prioritization, concurrency control, rate limiting, and more. Its support for Redis as a backend ensures high performance and persistence, making it suitable for use in distributed and scalable systems.
💡 Note: If you find yourself using these schedulers often, you should consider using an opensource toolchain such as Bit to extract the code for any of these schedulers into a reusable code/module that you could then reuse across projects with a simple npm i @bit/your-username/schedule-task.
Learn more here:
Extracting and Reusing Pre-existing Components using bit add
Do’s and Don’ts Tips
- Do use a persistent storage backend, like MongoDB, to ensure that scheduled tasks are not lost during server restarts or crashes. (Agenda & Bull done it for us)
- Don’t schedule tasks with extremely high frequencies, as this can lead to performance issues and consume resources unnecessarily.
- Do ensure that the scheduling logic can handle edge cases, such as daylight saving time changes or leap years.
- Don’t rely solely on the server’s local time for scheduling tasks.
- Do monitor and log task execution to identify potential issues and optimize performance.
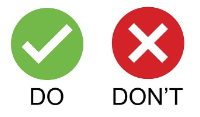
Conclusion
Scheduling tasks in Node.js is crucial for automating processes, optimizing resource usage, and improving the overall efficiency of your application. By leveraging powerful third-party packages like Agenda, developers can quickly implement robust task-scheduling mechanisms that can handle various use cases. Keep in mind the do’s and don’ts to ensure that your task scheduling implementation is both reliable and efficient.
Build Apps with reusable components, just like Lego
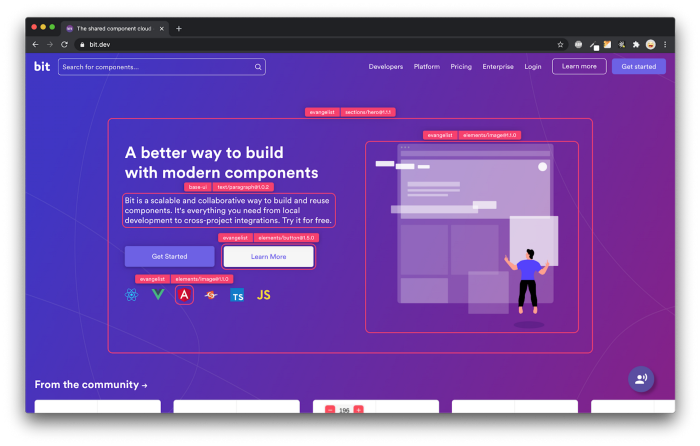
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Creating a Developer Website with Bit components
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
- How to Reuse and Share React Components in 2023: A Step-by-Step Guide
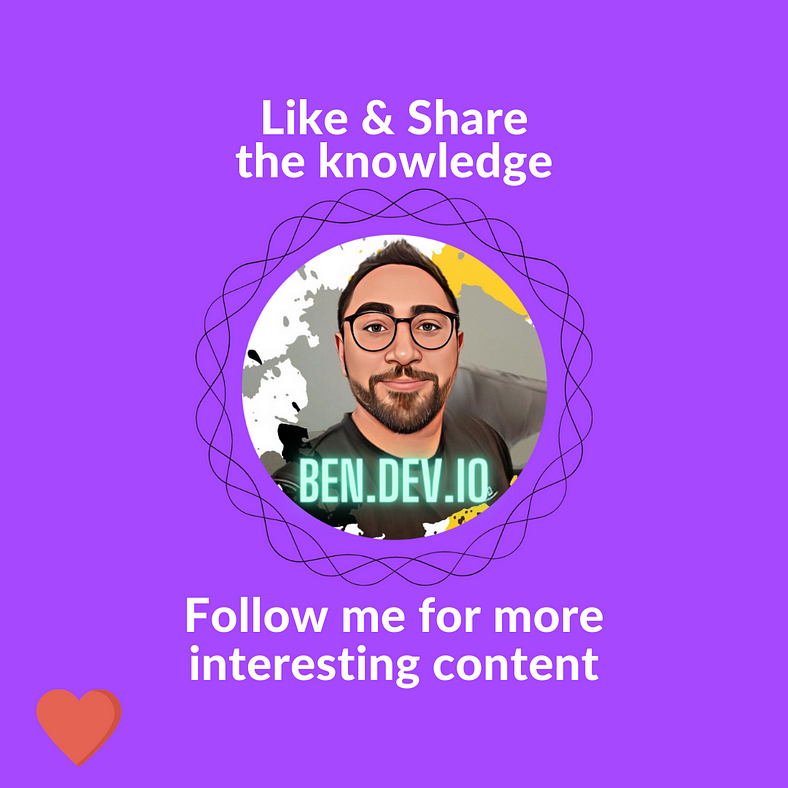
Visit me at ben.dev.io
Scheduling Tasks Just in Time Using Node.js was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Ben Mishali
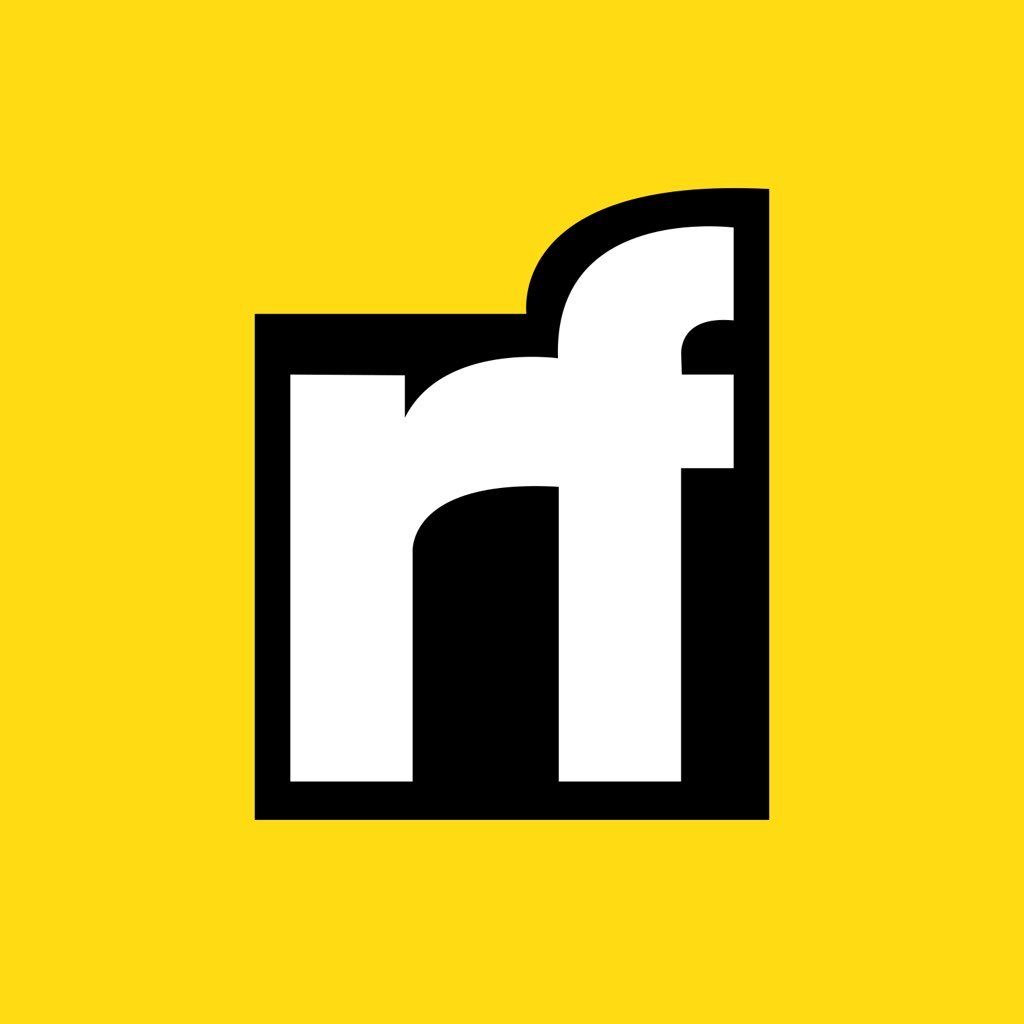
Ben Mishali | Sciencx (2023-04-25T01:45:46+00:00) Scheduling Tasks Just in Time Using Node.js. Retrieved from https://www.scien.cx/2023/04/25/scheduling-tasks-just-in-time-using-node-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.