This content originally appeared on DEV Community and was authored by Muhib ur Rahman Bakar
I'd like to share some valuable tips on how to boost the performance of your ReactJS applications. Implementing these best practices can make a significant difference in your app's responsiveness and user experience. Let's dive in! ๐
1. PureComponent and React.memo ๐งฉ Utilize PureComponent for class components and React.memo for functional components to prevent unnecessary re-renders. These optimizations ensure that components only update when their props have changed.
import React, { PureComponent } from 'react';
class MyComponent extends PureComponent {
// Your component logic
}
// OR
import React, { memo } from 'react';
const MyComponent = memo(function MyComponent(props) {
// Your component logic
});
2. Debounce and Throttle User Input ๐๏ธ Debounce or throttle user input events like scrolling, typing, or resizing to reduce the number of updates and improve performance.
import { debounce } from 'lodash';
const handleInputChange = debounce((value) => {
// Your input change logic
}, 300);
3. Lazy Loading and Code Splitting ๐ฆ Leverage React.lazy and React.Suspense to split your code into smaller chunks and load components only when they're needed.
import React, { lazy, Suspense } from 'react';
const MyComponent = lazy(() => import('./MyComponent'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<MyComponent />
</Suspense>
</div>
);
}
4. Use the Profiler API and Chrome DevTools ๐ Identify performance bottlenecks using React DevTools and the Profiler API. This will help you pinpoint areas that need optimization.
import React, { Profiler } from 'react';
function onRenderCallback(
id,
phase,
actualDuration,
baseDuration,
startTime,
commitTime,
interactions
) {
// Log or analyze the profiling data
}
function App() {
return (
<Profiler id="MyComponent" onRender={onRenderCallback}>
<MyComponent />
</Profiler>
);
}
5. Optimize State and Props Management ๐ Use selectors with libraries like Reselect or Recoil to efficiently manage and derive state, minimizing unnecessary re-renders.
import { createSelector } from 'reselect';
const getItems = (state) => state.items;
const getFilter = (state) => state.filter;
const getFilteredItems = createSelector(
[getItems, getFilter],
(items, filter) => items.filter(item => item.includes(filter))
);
Implementing these tips can greatly enhance your ReactJS app's performance. Give them a try and let me know how they work for you! Happy coding! ๐
ReactJS #Performance #Optimization #WebDevelopment #BestPractices
This content originally appeared on DEV Community and was authored by Muhib ur Rahman Bakar
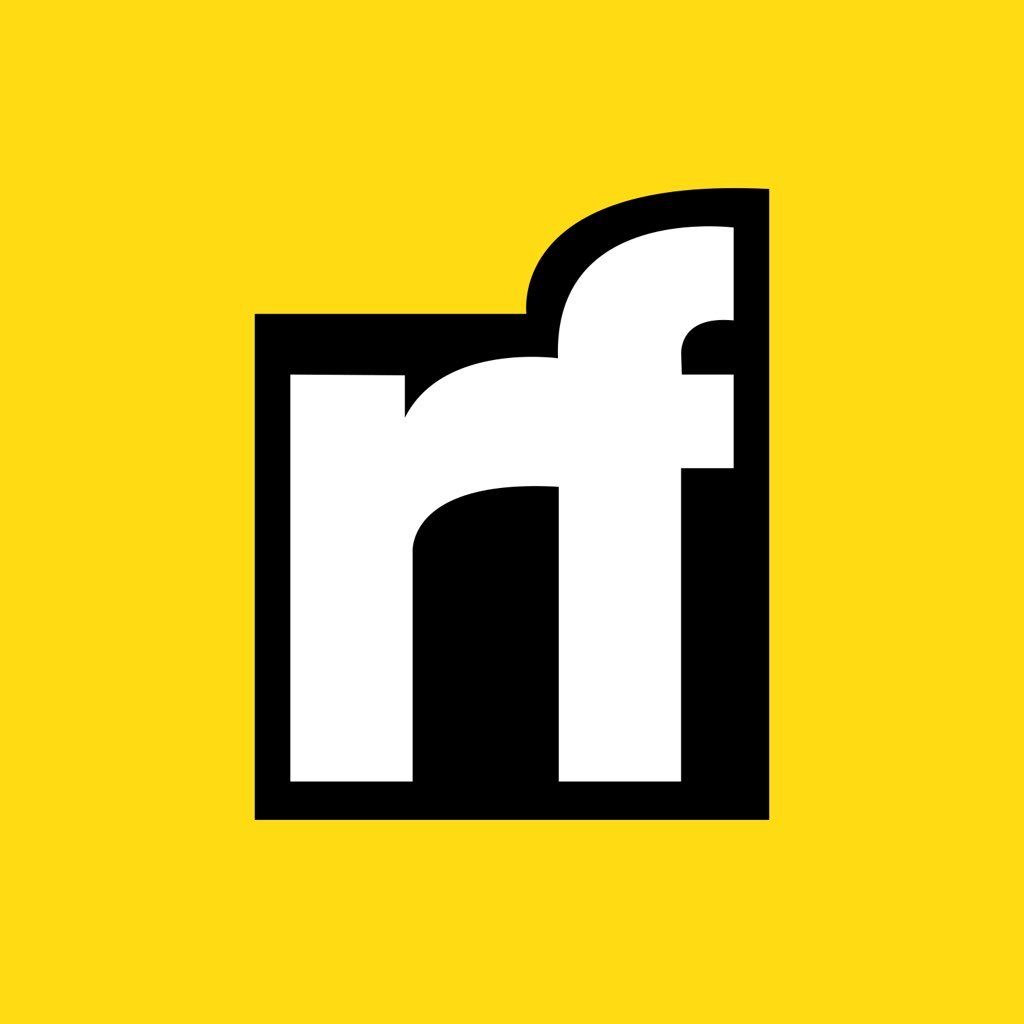
Muhib ur Rahman Bakar | Sciencx (2023-04-30T11:47:35+00:00) 5 Key Tips to Boost Your ReactJS Performance ๐. Retrieved from https://www.scien.cx/2023/04/30/5-key-tips-to-boost-your-reactjs-performance-%f0%9f%9a%80/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.