This content originally appeared on Level Up Coding - Medium and was authored by Corey Duffy
Beyond Basic Commands: Discovering the Hidden Gems of Git
Every tech company these days is using Git.
But are you using it to its full potential?
Here are three Git tricks that can help elevate your skills and make you an indispensable part of your development team.
Trick #1: Using git bisect to Identify Bugs
The git bisect command is a powerful tool in the Git toolkit, offering an efficient way to identify the exact commit that introduced a bug into your codebase. This command leverages a binary search algorithm, making it possible to isolate the problematic commit, even in a large project with an extensive commit history.
The operation of git bisect is based on the principle of binary search.
Understanding ‘git bisect’
Here's how it works:
Suppose your tests were passing at commit A, but failing at commit B. You can use git bisect to check out a commit midway between A and B. This is the "binary" part of the binary search—it effectively divides the range of commits into two halves.
At this point, you need to test the current commit, the one git bisect checked out.
This testing process isn't conducted by Git itself, but rather by you, the developer, using your existing test suite or manual testing methods. This is where git bisect interacts with your test suite—it doesn't run the tests, but it relies on you to run them and report back.
Once you’ve tested the commit, you’ll mark it as either good (git bisect good) if the tests pass, or bad (git bisect bad) if they fail. Based on your feedback, git bisect knows whether the bug was introduced in the first half or the second half of the commit range. If the tests pass, it understands the bug was introduced in the second half of the range, and vice versa.
Next, git bisect will check out a new commit midway between the current commit and either commit A or commit B, depending on whether the tests passed or failed.
You then repeat the testing and feedback process.
Git bisect in action
Here’s a simple example of how this all fits together:
git bisect start
git bisect bad # Current version is bad
git bisect good v2.6.13-rc2 # v2.6.13-rc2 is known to be good
Let’s walk through the provided example:
- git bisect start: This command starts the bisecting session.
- git bisect bad: This command marks the current commit (the commit you're on) as bad, meaning the bug is present.
- git bisect good v2.6.13-rc2: This command marks the v2.6.13-rc2 commit as good, meaning the bug is not present in this commit.
After these commands, Git will check out a commit halfway between the current bad commit and the good v2.6.13-rc2 commit. At this point, you need to run your tests. If the tests fail, you execute git bisect bad. If they pass, you run git bisect good.
Git will continue to narrow down the range of commits, checking out the midpoint commit each time for you to test. This process repeats until Git pinpoints the exact commit that introduced the bug. This algorithmically efficient approach can save you significant time and effort when debugging complex issues in large codebases.
Trick #2: Mastering the Interactive Rebase
Interactive rebase, or git rebase -i, is one of Git's most potent tools.
It can offer you a granular level of control over your commit history. With it, you can modify the sequence of commits, merge them, edit them, and even delete them, thereby presenting a cleaner, more understandable commit history. This feature is especially useful for intermediate-advanced-level developers who work on complex projects that require precise version control.
Let’s take a typical scenario: you have made a series of commits on your feature branch. However, upon reviewing the commits, you realise that they could be better organised. This is where interactive rebase comes into play.
Using git rebase -i
The command git rebase -i HEAD~5 will allow you to begin an interactive rebase for the most recent five commits.
This command opens a text editor that lists the last five commits in reverse order like this:
pick 1fc6c95 do something
pick 6b2481b do something else
pick dd1475d changed some things
pick c619268 updated
pick bbe5a2e final commit
Each line corresponds to a commit, and the word pick means that the commit will be included in the rebase. The commits can be rearranged simply by changing the order of the lines in the text editor. Git will apply the commits in the order they appear in the text file once it's saved and closed.
In addition to reordering, you can perform various operations on the commits:
- pick: Use the commit as-is.
- reword: Use the commit but edit the commit message.
- edit: Use the commit but stop for amending.
- squash: Use the commit, but amalgamate into the previous commit (the commit above it in the list).
- fixup: Similar to “squash”, but discard the commit log message.
- exec: Run some shell commands (useful for running tests).
- drop: Remove the commit.
When you’ve finished making changes in the text editor, save and close the file. Git will then start applying the commits based on the instructions you’ve given.
Be cautious when using git rebase -i as it alters the commit history. It's recommended to use it only for commits that have not been pushed to the shared repository. Misuse can lead to more complex issues, but with practice, you can use interactive rebasing to handle your commit history effectively and precisely.
Trick #3: Taking Advantage of git stash
In the world of software development, change is the only constant.
Deep stuff.
The ability to work on multiple features or bugs at the same time is a key part of a developer’s workflow. git stash is an essential tool that helps manage these changes and switch between different work contexts smoothly.
Imagine you’re in the middle of working on a new feature when a high-priority bug comes in. You need to switch branches, but you don’t want to lose your work. This is exactly what git stash was designed for.
Understanding ‘git stash’
At its core, git stash is a command that temporarily shelves (or stashes) changes you've made to your working directory that you want to save for later, so you can work on something else.
The stash is a stack, where your stashed changes are stored one after the other.
The git stash command will take your modified tracked files, staged changes, and some untracked files (specifically, files that are not ignored by gitignore), and save them on a new stash. Your working directory reverts back to the last commit.
Switching Contexts with ‘git stash’
The real power of git stash is in context switching. Let’s imagine that you're working on a new feature in a branch, and you need to switch to another branch to work on a high-priority bug. However, the feature you're working on is incomplete, and you're not ready to make a commit yet as the commit would not represent a logical unit of change. This is where git stash comes in handy.
After running git stash, you can switch branches and work on the bug without having to commit your previous changes or lose them.
Once you're done with the bug, you can switch back to your feature branch and simply run git stash apply to get your changes back and continue where you left off.
Potential Pitfalls and Mistakes
While git stash is incredibly helpful, there are a few common pitfalls that you need to watch out for:
- Stash is not a commit: While a stash is similar to a commit, they are not the same. Stashes are local to your git repository and are not transferred to the server when you push your changes.
- Stashes could be forgotten: It’s common to stash some changes and then forget about them. I’m very guilty of this. Over time, you might accumulate a number of stashes. It’s a good practice to regularly run git stash list to see all your stashes and apply or drop them as necessary.
- Conflicts while applying stash: When you apply a stash, you could run into conflicts, especially if the branch has changed significantly since you made the stash. In this case, you will have to resolve the conflicts manually.
And that’s it — these three Git tricks can significantly boost your productivity and streamline your workflow.
By mastering these, not only will you become more efficient in managing your code, but you’ll also become an indispensable part of your development team.
Remember, the key to mastering Git lies in understanding and effectively utilising its numerous features. Happy coding!
If you found this article helpful or informative, please consider clapping, sharing, checking out some of my other articles and following me on Medium and/or Twitter. Thanks a lot and happy reading!
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
3 Git Tricks That Will Make You an Indispensable Part of Your Development Team was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Corey Duffy
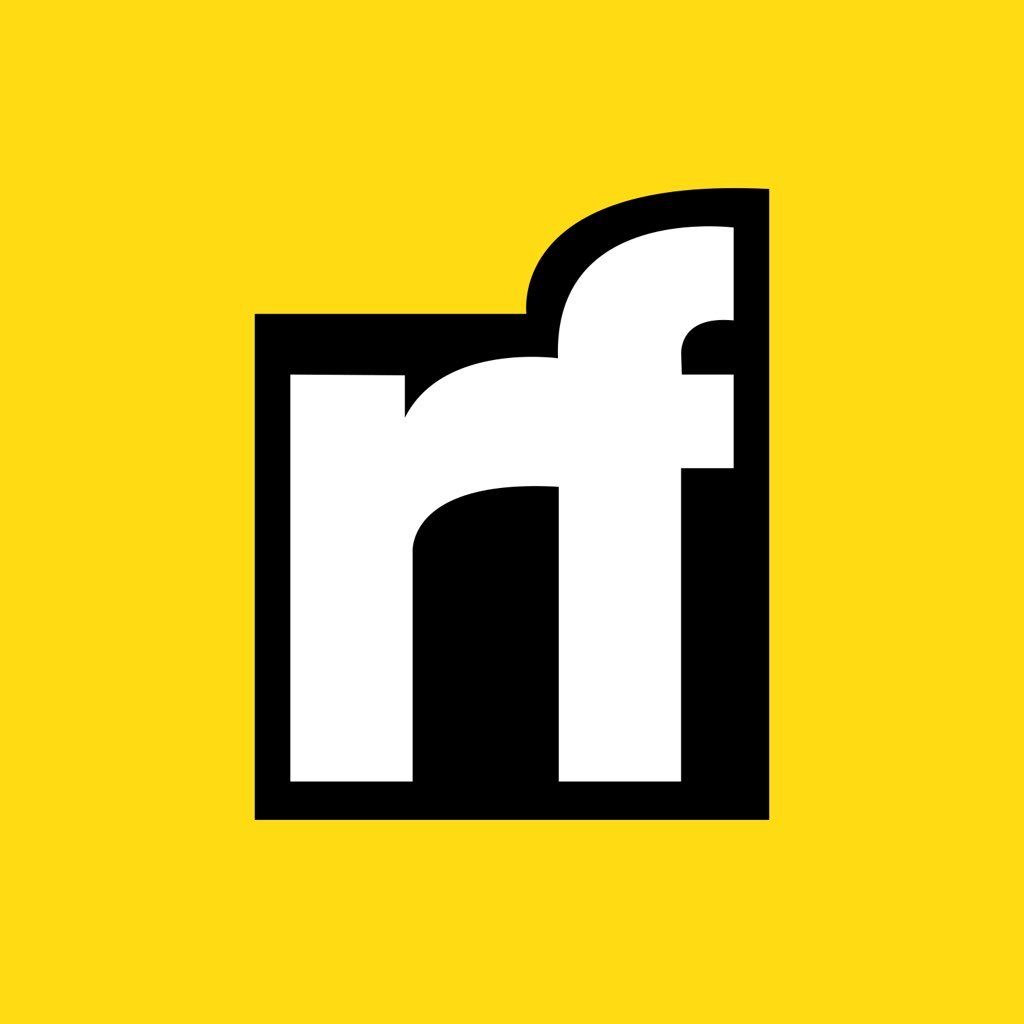
Corey Duffy | Sciencx (2023-05-14T15:24:16+00:00) 3 Git Tricks That Will Make You an Indispensable Part of Your Development Team. Retrieved from https://www.scien.cx/2023/05/14/3-git-tricks-that-will-make-you-an-indispensable-part-of-your-development-team/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.