This content originally appeared on DEV Community and was authored by Johns Code
This one is pretty common. Sounds difficult, but not really bad once you think it through.
Write a golang function to check if a string is a palindrome.
A palindrome is a sequence of characters that is the same even when reversed, for example:
"aba" is a palindrome
"abb is not
"ab a" is considered a palindrome by most, so we ignore whitespace.
func PalindromeCheck(str string) bool {
trimmedStr := strings.ReplaceAll(str, " ", "")
len := len(trimmedStr)
chars := []rune(trimmedStr)
for i := 0; i < len/2; i++ {
if chars[i] != chars[len-i-1] {
return false
}
}
return true
}
This solution is functionally the same as you will find for C or Java when searching online. We are essentially using dual pointers to traverse from the beginning and the end looking for a mismatched character. When a mismatch is found, we can declare the string is not a palindrome.
Can we make it better?
Is there a better way to trim whitespace rather than using strings.ReplaceAll
? (there is but it can get ugly)
What about the efficiency of converting to an []rune
, is there a better way?
Post your thoughts in the comments.
Thanks!
This content originally appeared on DEV Community and was authored by Johns Code
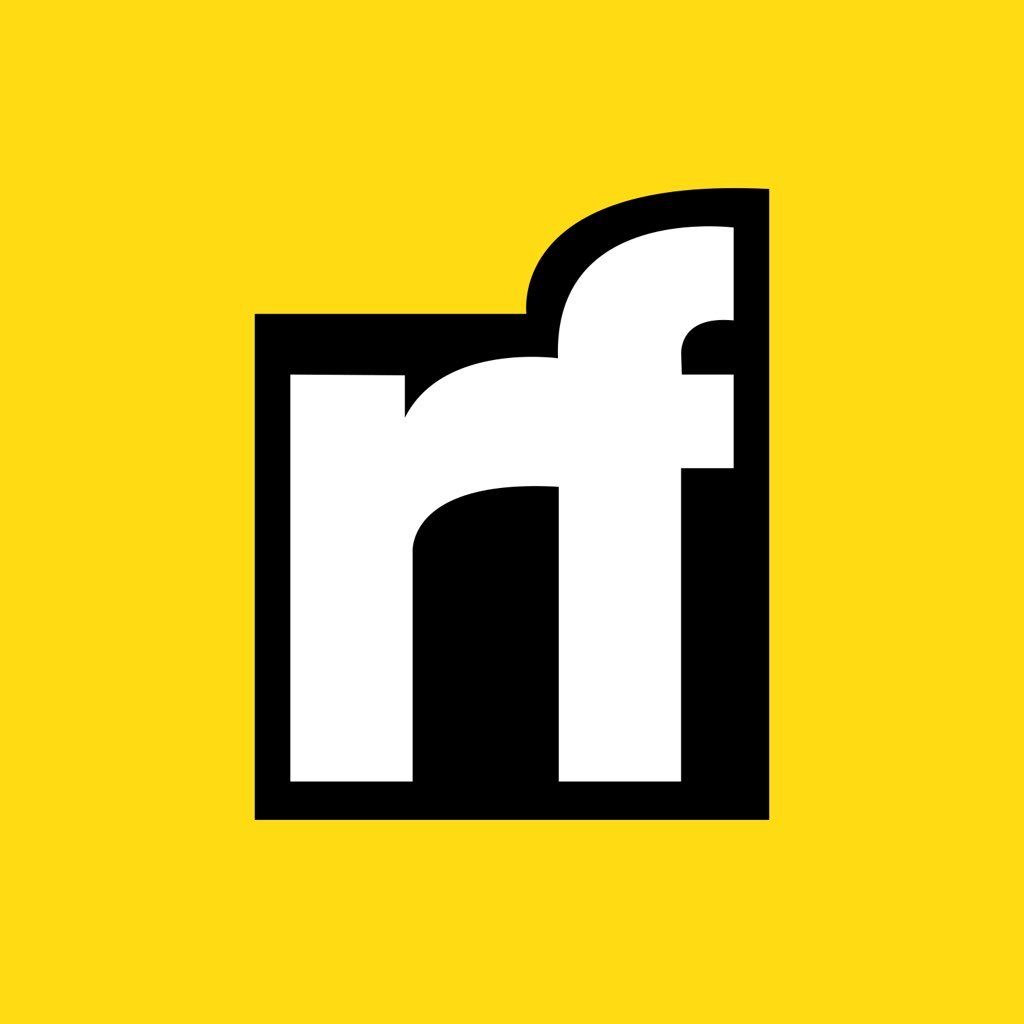
Johns Code | Sciencx (2024-06-18T15:53:10+00:00) Palindrome check a string. Retrieved from https://www.scien.cx/2024/06/18/palindrome-check-a-string/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.