This content originally appeared on DEV Community and was authored by Roberto Umbelino
Hey there! Let's dive into how to test an API built with Fastify using Vitest and TypeScript. We'll focus on a simple health check route and make sure everything's working as it should.
Why Test an API?
Testing an API is super important to make sure everything is running smoothly. Automated tests help us catch bugs quickly and keep our code solid and reliable.
Setting Up the Environment
Let's start from scratch and set up our project. First, create a directory for the project and initialize a new Node.js project:
mkdir my-fastify-api
cd my-fastify-api
npm init -y
Next, let's install Fastify and TypeScript:
npm install fastify
npm install typescript ts-node @types/node --save-dev
Create a tsconfig.json
file to configure TypeScript:
{
"compilerOptions": {
"target": "ESNext",
"module": "CommonJS",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"outDir": "./dist",
"rootDir": "./src"
},
"include": ["src/**/*.ts"]
}
Creating the Fastify Server
Now, let's set up a Fastify server with a simple health check route. Create a src
directory and inside it, make an app.ts
file:
// src/app.ts
import fastify from 'fastify';
const app = fastify();
app.get('/health-check', async (request, reply) => {
return { status: 'ok' };
});
export default app;
Next, let's create the server.ts
file to start the server:
// src/server.ts
import app from './app';
const start = async () => {
try {
await app.listen({ port: 3000 });
console.log('Server is running on http://localhost:3000');
} catch (err) {
app.log.error(err);
process.exit(1);
}
};
start();
Installing Vitest
Now, let's install Vitest so we can write our tests:
npm install --save-dev vitest
Add the following scripts to your package.json
:
"scripts": {
"dev": "ts-node src/server.ts",
"test": "vitest",
"test:watch": "vitest --watch"
}
Writing Tests
Let's write a test for our health-check
route. Create a tests
directory and inside it, make a health-check.test.ts
file:
// tests/health-check.test.ts
import app from '../src/app';
import { test, expect } from 'vitest';
test('GET /health-check should return status OK', async () => {
const response = await app.inject({
method: 'GET',
url: '/health-check'
});
expect(response.statusCode).toBe(200);
expect(response.json()).toEqual({ status: 'ok' });
});
Running the Tests
To run the tests, use this command:
npm test
If you want the tests to run continuously whenever you make changes, use:
npm run test:watch
Conclusion
And that's it! In this article, we saw how to set up a Fastify project with TypeScript from scratch and how to write tests for a simple route using Vitest. Testing your routes makes sure your API is always working correctly and helps avoid any nasty surprises later on. Happy coding!
This content originally appeared on DEV Community and was authored by Roberto Umbelino
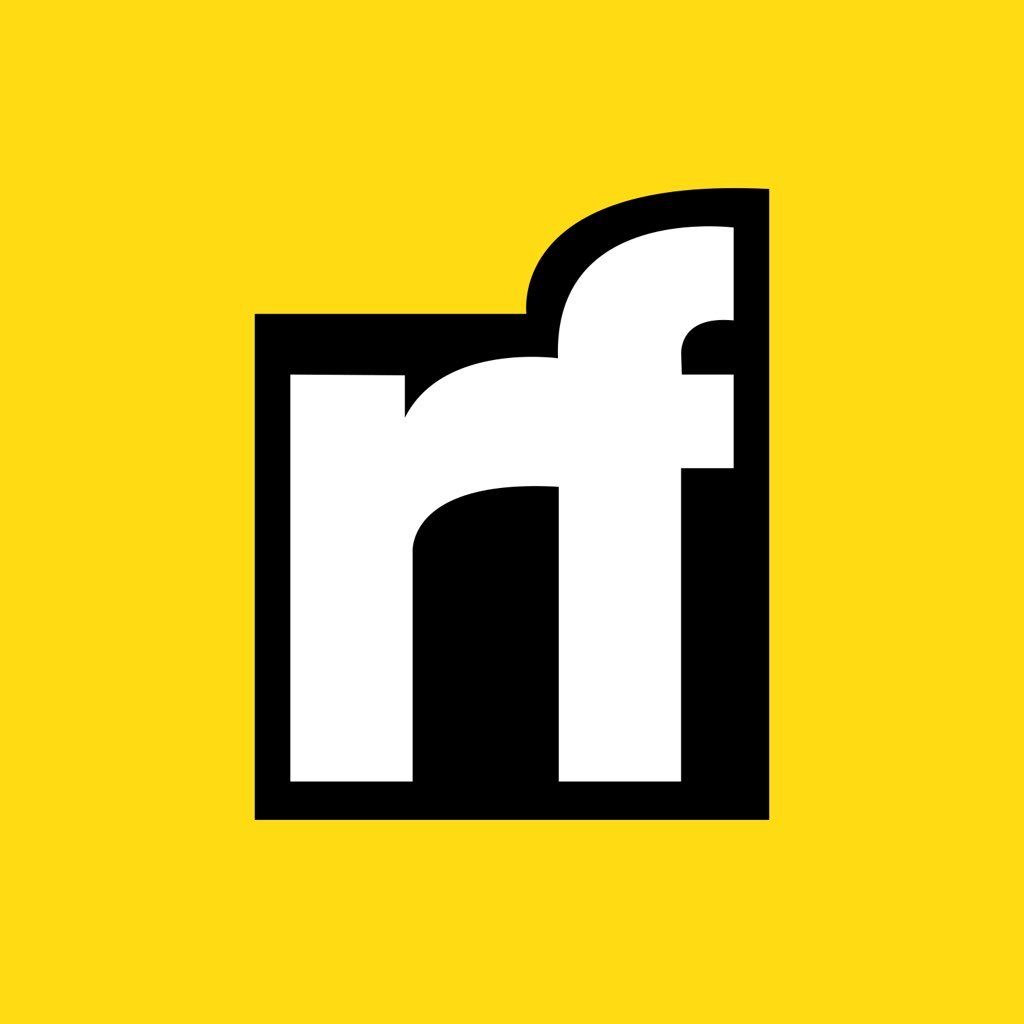
Roberto Umbelino | Sciencx (2024-06-20T16:38:29+00:00) Testing Your API with Fastify and Vitest: A Step-by-Step Guide. Retrieved from https://www.scien.cx/2024/06/20/testing-your-api-with-fastify-and-vitest-a-step-by-step-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.