This content originally appeared on DEV Community and was authored by John Kamau
Introduction
One of the most powerful features of the JavaScript language is the ability to handle asynchronous operations. Asynchronous programming allows developers to perform tasks like fetching data from a server or reading a file without blocking the main execution thread. This ensures that applications remain responsive and efficient, providing a smoother user experience and better performance.
Basics of Asynchronous Programming
In programming, operations can be either synchronous or asynchronous. Understanding the difference between these two types is crucial for effective programming.
Synchronous Programming
Synchronous programming blocks the execution of subsequent code until the current operation completes. This means that each task is performed one after the other, waiting for the previous one to finish.
Consider a scenario where you need to book a flight, reserve a hotel room, and rent a car for a trip. In a synchronous world, you would call each service one by one, waiting on hold until each booking is confirmed before moving to the next. This approach can be problematic in certain situations, particularly when dealing with tasks that take a significant amount of time to complete.
A good example is when a synchronous program performs a task that requires waiting for a response from a remote server. The program will be stuck waiting for the response and cannot do anything else until the response is returned. This is known as blocking, and it can lead to the application appearing unresponsive or “frozen” to the user.
Asynchronous Programming
In contrast, asynchronous programming allows the execution of subsequent code to proceed independently while waiting for the current operation to complete.
Using the same scenario where you need to book a flight, reserve a hotel room, and rent a car for a trip, in an asynchronous world, you could call all three services simultaneously. While waiting for each service to confirm your booking, you can continue with other trip preparations, making the overall process faster and more efficient.
Asynchronous programming allows a program to continue working on other tasks while waiting for external events, such as network requests, to occur. For example, while a program retrieves data from a remote server, it can continue to execute other tasks such as responding to user inputs. This greatly improves the performance and responsiveness of a program.
Handling Asynchronous Operations
In JavaScript, asynchronous programming can be achieved through a variety of techniques. In this article, we are going to cover ways in which you can do this.
Using Callbacks
Callbacks are one of the oldest and most widely used methods for handling asynchronous operations in JavaScript. A callback function is simply a function that is passed as an argument to another function and is executed after the completion of a task.
// Declare function
function fetchData(callback) {
setTimeout(() => {
const data = {name: "John", age: 24};
callback(data);
}, 3000);
}
// Execute function with a callback
fetchData(function(data) {
console.log(data);
});
console.log("Data is being fetched...");
In this example:
- We declare a function named fetchData that takes a callback function as an argument.
- Inside fetchData, the setTimeout function is used to simulate an asynchronous operation by delaying the execution of a callback function for 3 seconds.
- After the 3-second delay, the callback function passed to fetchData is invoked with a mock data object as its argument.
- The callback function passed to fetchData is an anonymous function that logs the received data object to the console.
- Output: Initially, a message “Data is being fetched…” is logged to the console. After 3 seconds, the mock data object is fetched, and the callback function is executed, logging the data object to the console.
Callbacks are powerful because they allow us to continue with other tasks while waiting for asynchronous operations to complete. However, they can lead to callback hell, a situation where multiple nested callbacks make the code difficult to read and maintain.
getData(function(data1) {
processData1(data1, function(data2) {
processData2(data2, function(data3) {
processData3(data3, function(data4) {
// and so on...
});
});
});
});
In this example, each asynchronous operation depends on the result of the previous one, leading to deeply nested callbacks. As more operations are added, the code becomes increasingly difficult to manage, often resulting in bugs and decreased readability.
Despite their drawbacks, callbacks are still widely used, especially in older codebases and libraries. Understanding how to use callbacks effectively is essential for any JavaScript developer.
Using Promises
Promises provide a more elegant way to handle asynchronous operations compared to callbacks. A Promise represents a value that may be available now, in the future, or never. Promises have three states: Pending, Fulfilled, and Rejected.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = {name: "John", age: 24};
resolve(data);
}, 3000);
});
}
fetchData().then((data) => {
console.log(data);
}).catch((error) => {
console.error(error);
});
In this example, the fetchData function returns a Promise. Inside the Promise constructor, an asynchronous operation is simulated using setTimeout. Once the operation is complete, the resolve function is called with the fetched data.
Promises allow for chaining using the then method, making it easy to perform sequential asynchronous operations. Additionally, error handling is simplified with the catch method, which handles any errors that occur during the execution of the Promise chain.
Async/Await: Simplifying Asynchronous Code
Async/Await is a powerful feature in JavaScript that simplifies asynchronous programming by providing a more synchronous-looking syntax. While it builds on top of Promises, it offers a more intuitive way to handle asynchronous operations without directly dealing with Promise chains.
async function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = {name: "John", age: 24};
resolve(data);
}, 3000);
});
}
async function getData() {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error(error);
}
}
getData();
Here, the fetchData function returns a Promise, as it did before. However, instead of chaining .then() and .catch() methods, the getData function is defined as an async function. Inside getData, the await keyword is used to pause the execution until the fetchData Promise resolves, making the asynchronous code flow appear synchronous.
Using Async/Await simplifies error handling with traditional try/catch blocks, providing a more natural way to handle errors in asynchronous code. Overall, Async/Await makes asynchronous programming in JavaScript more readable and maintainable by allowing developers to write asynchronous code that looks and behaves like synchronous code.
Practical Applications of Asynchronous JavaScript
Asynchronous JavaScript is essential for creating responsive and efficient applications. Here are some practical applications where asynchronous operations play a crucial role:
1.Fetching Data from APIs
Applications often need to retrieve data from external APIs. Asynchronous operations allow the application to continue running while waiting for the data to be fetched.
async function fetchUserData() {
try {
const response = await fetch('https://api.example.com/user');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching user data:', error);
}
}
fetchUserData();
In this example, the fetch API is used to retrieve user data. The await keyword ensures the code waits for the response before proceeding, without blocking the main thread.
2.File Operations
When working with files, such as reading or writing to the file system, asynchronous operations are essential to prevent the main thread from freezing.
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
console.log('This message will be printed first');
In this example, when fs.readFile() is called, Node.js initiates the file read operation but doesn't wait for it to complete. Instead, it registers the provided callback function and immediately continues executing the next line, which logs the message 'This message will be printed first'. When the file read operation completes, Node.js places the callback function in the event queue, and the event loop eventually dequeues and executes it on the main thread, logging the file contents or an error message.
By using asynchronous programming for file operations, the main execution thread remains responsive and can handle other tasks while waiting for the file operation to complete.
3.Timers
JavaScript provides the setTimeout and setInterval functions for scheduling code execution after a specified delay or at regular intervals, respectively. These functions are asynchronous, meaning they don't block the main execution thread while waiting for the specified time to elapse.
// Scheduling a recurring execution every second
const intervalId = setInterval(() => {
console.log('This message will be displayed every second');
}, 1000);
// After 5 seconds, stop the recurring execution
setTimeout(() => {
clearInterval(intervalId);
console.log('Interval cleared');
}, 5000);
In this example, we store the identifier returned by setInterval in the intervalId variable. After 5 seconds (using setTimeout), we call clearInterval(intervalId), which stops the recurring execution scheduled by setInterval.
The clearInterval function is useful when you want to stop a recurring task based on certain conditions, such as user interactions, state changes, or timers. Without clearInterval, the recurring execution would continue indefinitely unless the page is reloaded or the script is terminated.
4.Animations
Creating smooth animations is another important use case for asynchronous JavaScript. The requestAnimationFrame function is designed specifically for this purpose. It tells the browser to update an animation before the next repaint, ensuring optimal performance and efficient use of system resources.
const element = document.getElementById('myElement');
let position = 0;
function animateElement() {
position += 10; // Update the position
element.style.left = `${position}px`; // Move the element
// Request the next animation frame
if (position < 500) {
requestAnimationFrame(animateElement);
}
}
// Start the animation
requestAnimationFrame(animateElement);
In this example, the animateElement function is recursively called using requestAnimationFrame until the desired position is reached. The browser handles these function calls asynchronously, ensuring that the animation runs smoothly without blocking the main execution thread, ensuring a seamless user experience in web applications.
5.Event Handling
Event handling in the browser is inherently asynchronous, as events are triggered by user interactions or other external factors.
const button = document.getElementById('myButton');
button.addEventListener('click', () => {
console.log('Button clicked!');
});
console.log('This message will be displayed first');
In this example, when the script runs, it logs the message 'This message will be displayed first' to the console immediately. However, the event handler function (() => { console.log('Button clicked!'); }) is not executed until the user actually clicks the button.
When the user clicks the button, the browser generates a click event and adds it to the event queue. The event loop detects the event in the queue and executes the registered event handler function, which logs the message 'Button clicked!' to the console.
This asynchronous nature of event handling ensures that the JavaScript code doesn’t block the main execution thread while waiting for user interactions or other external events. The browser can continue to update the UI, parse HTML, execute other scripts, and perform other tasks while waiting for events to occur.
Conclusion
The practical applications discussed are indeed among the most important and widely-used cases where asynchronous JavaScript is employed. However, these examples do not represent an exhaustive list.
It is crucial to recognize that asynchronous programming is a widespread concept that finds applications in numerous other scenarios within the constantly evolving field of web development and beyond. As new technologies and use cases emerge, the significance of asynchronous programming in JavaScript is likely to continue increasing, ensuring efficient, responsive, and scalable applications across various domains.
This content originally appeared on DEV Community and was authored by John Kamau
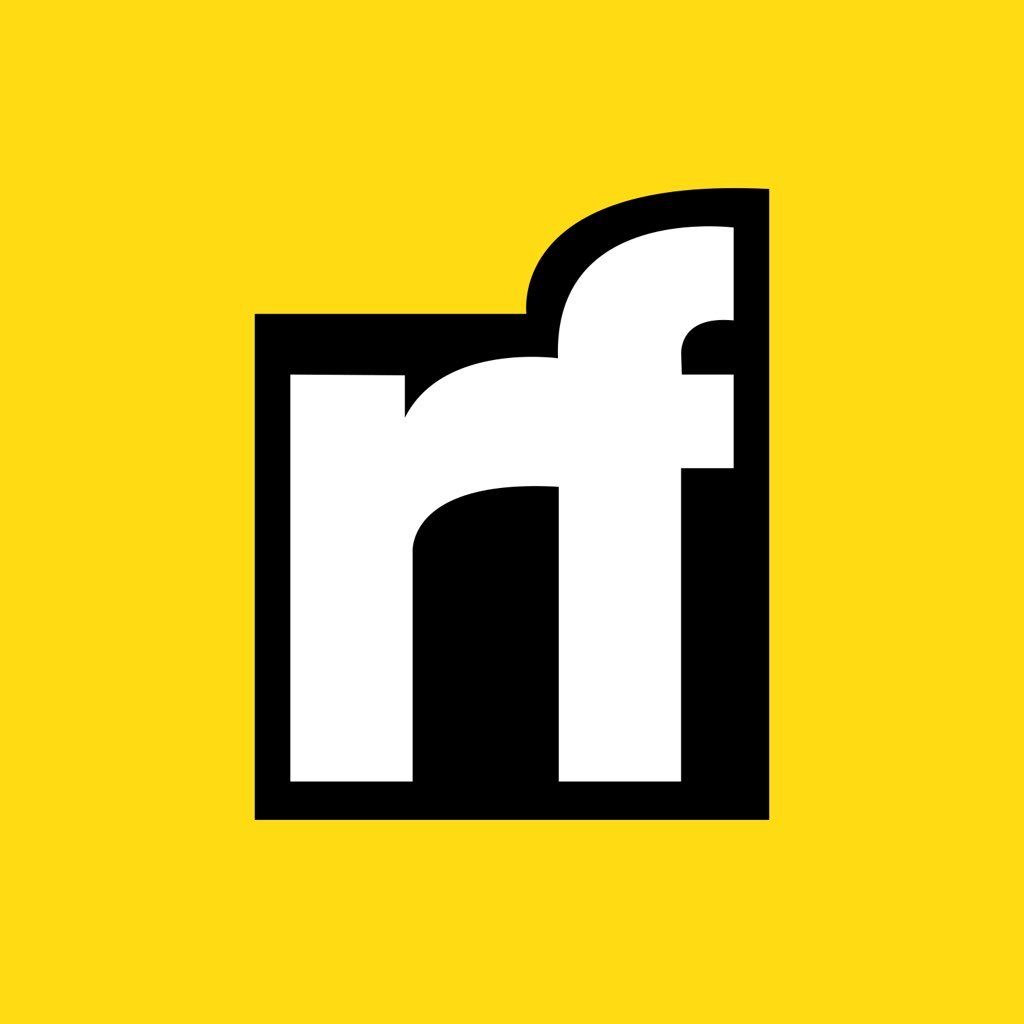
John Kamau | Sciencx (2024-06-21T11:29:57+00:00) Asynchronous JavaScript: Callbacks, Promises, and Async/Await. Retrieved from https://www.scien.cx/2024/06/21/asynchronous-javascript-callbacks-promises-and-async-await/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.