This content originally appeared on DEV Community and was authored by _Khojiakbar_
DOM Traversal refers to navigating the hierarchy of the DOM to access and manipulate elements relative to other elements. This includes accessing parent nodes, child nodes, and sibling nodes. Here's a detailed look at various DOM traversal methods and properties, with examples to illustrate their use.
1. Parent Nodes
- parentNode: Accesses the parent node of the current element.
- parentElement: Accesses the parent element (returns null if the parent is not an element).
Example:
<!DOCTYPE html>
<html>
<head>
<title>DOM Traversal - Parent Nodes</title>
</head>
<body>
<div id="parent">
<div id="child">Child Element</div>
</div>
<script>
const child = document.getElementById('child');
console.log(child.parentNode); // Output: <div id="parent">...</div>
console.log(child.parentElement); // Output: <div id="parent">...</div>
</script>
</body>
</html>
2. Child Nodes
childNodes: Returns a NodeList of all child nodes (including text nodes).
children: Returns an HTMLCollection of all child elements.
firstChild: Returns the first child node (including text nodes).
lastChild: Returns the last child node (including text nodes).
firstElementChild: Returns the first child element.
lastElementChild: Returns the last child element.
childElementCount: Returns the number of child elements.
Example:
<!DOCTYPE html>
<html>
<head>
<title>DOM Traversal - Child Nodes</title>
</head>
<body>
<div id="parent">
<p>First Child</p>
<p>Second Child</p>
<p>Third Child</p>
</div>
<script>
const parent = document.getElementById('parent');
console.log(parent.childNodes); // Output: NodeList(7) [#text, <p>, #text, <p>, #text, <p>, #text]
console.log(parent.children); // Output: HTMLCollection(3) [<p>, <p>, <p>]
console.log(parent.firstChild); // Output: #text (whitespace)
console.log(parent.lastChild); // Output: #text (whitespace)
console.log(parent.firstElementChild); // Output: <p>First Child</p>
console.log(parent.lastElementChild); // Output: <p>Third Child</p>
console.log(parent.childElementCount); // Output: 3
</script>
</body>
</html>
3. Sibling Nodes
- nextSibling: Accesses the next sibling node (including text nodes).
- previousSibling: Accesses the previous sibling node (including text nodes).
- nextElementSibling: Accesses the next sibling element. previousElementSibling: Accesses the previous sibling element.
Example:
<!DOCTYPE html>
<html>
<head>
<title>DOM Traversal - Sibling Nodes</title>
</head>
<body>
<div id="sibling1">Sibling 1</div>
<div id="sibling2">Sibling 2</div>
<div id="sibling3">Sibling 3</div>
<script>
const sibling2 = document.getElementById('sibling2');
console.log(sibling2.nextSibling); // Output: #text (whitespace)
console.log(sibling2.previousSibling); // Output: #text (whitespace)
console.log(sibling2.nextElementSibling); // Output: <div id="sibling3">Sibling 3</div>
console.log(sibling2.previousElementSibling); // Output: <div id="sibling1">Sibling 1</div>
</script>
</body>
</html>
Summary
Parent Nodes
- parentNode: Accesses the parent node of the current element.
- parentElement: Accesses the parent element (returns null if the parent is not an element).
Child Nodes
- childNodes: Returns a NodeList of all child nodes (including text nodes).
- children: Returns an HTMLCollection of all child elements.
- firstChild: Returns the first child node (including text nodes).
- lastChild: Returns the last child node (including text nodes).
- firstElementChild: Returns the first child element.
- lastElementChild: Returns the last child element.
- childElementCount: Returns the number of child elements.
Sibling Nodes
- nextSibling: Accesses the next sibling node (including text nodes).
- previousSibling: Accesses the previous sibling node (including text nodes).
- nextElementSibling: Accesses the next sibling element.
- previousElementSibling: Accesses the previous sibling element.
This content originally appeared on DEV Community and was authored by _Khojiakbar_
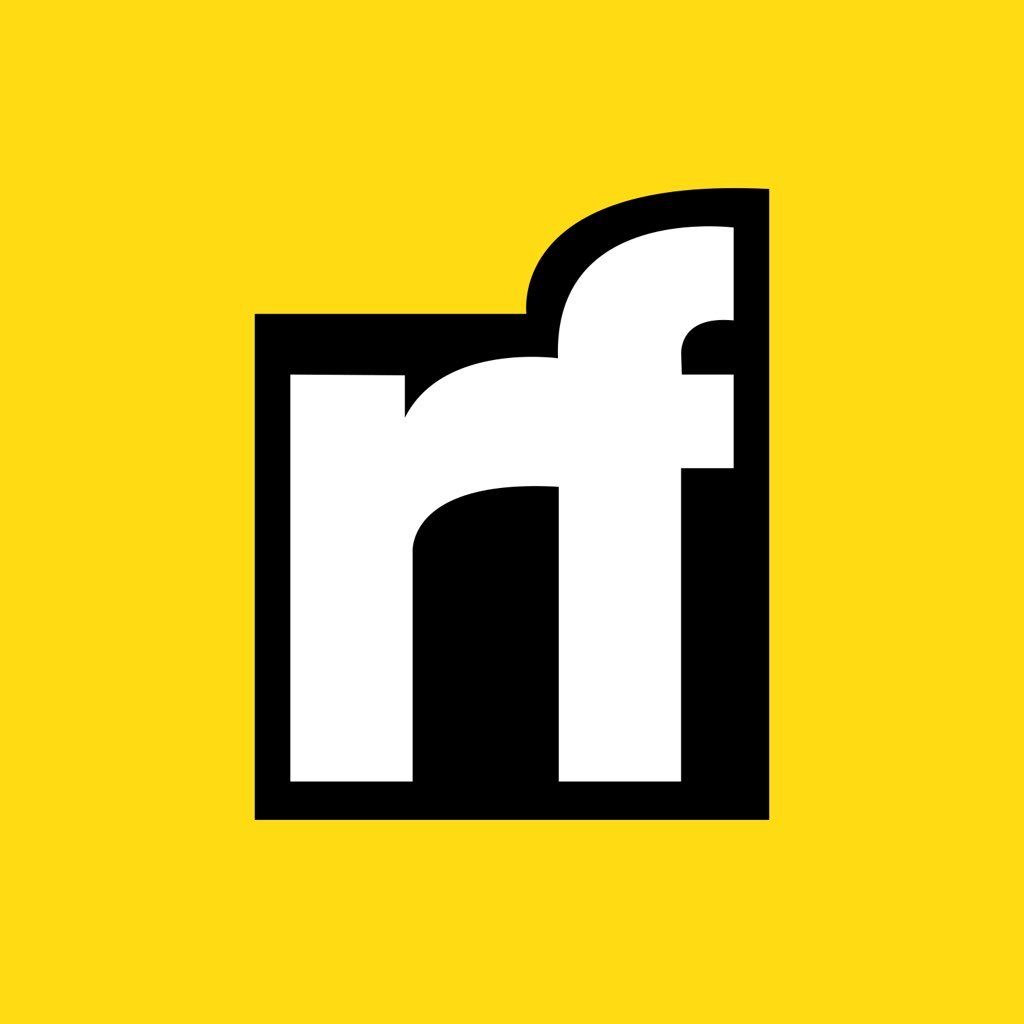
_Khojiakbar_ | Sciencx (2024-06-24T05:13:09+00:00) DOM Traversal👨👩👧. Retrieved from https://www.scien.cx/2024/06/24/dom-traversal%f0%9f%91%a8%f0%9f%91%a9%f0%9f%91%a7/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.