This content originally appeared on DEV Community and was authored by Megan Paffrath
Objects are collections of key-value pairs (properties).
To create an object we can do as follows:
let person = {
firstName: 'Megan',
isOnVacation: true,
favFoods: ['plum', 'salad', 'fritos']
}
To access values within the object we can:
console.log(person.isOnVacation); // true
console.log(person['isOnVacation']); // true
We can also make an object that contains objects:
let student = {
name: 'Jimmy',
exams: {
artFinal: 'A',
mathFinal: 88
}
}
To access a value in the object's object, we can:
console.log(student.exams.artFinal); // 'A'
Also, we can make arrays containing objects:
let friends = [
{
name: 'Sorour',
favColor: 'blue',
metAt: 'college'
},
{
name: 'Timothy',
favColor: 'red',
metAt: 'Starbucks'
}
];
To access a value in the second object, we can call:
console.log(friends[1].favColor); // 'red'
To update a value in the second object, we can:
friends[1].name = 'Tim';
console.log(friends[1].name); // Tim
This content originally appeared on DEV Community and was authored by Megan Paffrath
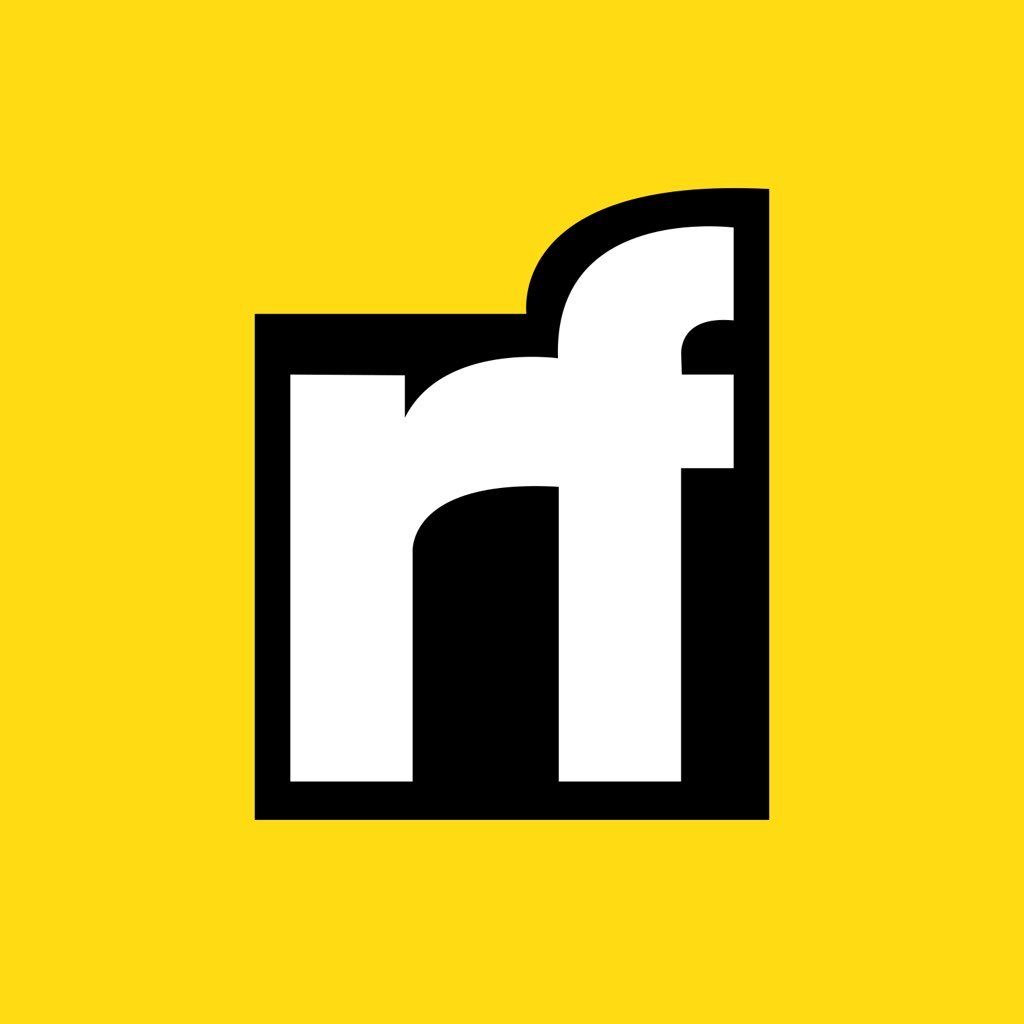
Megan Paffrath | Sciencx (2024-08-06T16:56:20+00:00) Object Literals. Retrieved from https://www.scien.cx/2024/08/06/object-literals/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.