This content originally appeared on DEV Community and was authored by
Main Chain and DHT-Based Structure
Main Chain: A proof-of-work blockchain that only records group
lifecycle actions, specifically \"group ID - open\" and \"group ID -
close.\" This design keeps the main chain lightweight and focused on
essential operations.DHT for Groups: Each group stores its messages in a Distributed
Hash Table (DHT), allowing for decentralized storage and retrieval
of messages without requiring heavy consensus mechanisms like PBFT.Ban-List: Group admins manage a ban-list to permanently exclude
specific public keys from joining the group, enhancing security and
mitigating the risk of DHT poisoning.
Main Chain
The main chain is designed to be lightweight, focusing solely on
tracking the lifecycle of groups. The block structure includes:
Previous block hash
Timestamp
Data (group lifecycle action)
Nonce
This ensures the chain remains efficient and scalable, with a minimal
data footprint.
DHT for Groups
Each group uses a DHT for storing and retrieving messages. Key
characteristics include:
Messages are stored as key-value pairs in the DHT, where the key is
typically a hash of the message content or identifier.Message integrity is ensured via cryptographic hashing, and for
private groups, messages are encrypted using a Group Private Shared
Key (GPSK).The DHT is managed by group members, with access control enforced
through the ban-list.
Core Operations
Group Creation
Generate Group ID Set group type (public/private) Generate Group Private
Shared Key (GPSK) Add Group ID and type to main chain Initialize DHT for
group
Joining Groups
Public Groups
Receive public link with Group ID Verify Group ID on main chain Check if
public key is on the ban-list Join group by accessing its DHT Deny
access and notify user of ban
Private Groups
Receive encrypted link (Group ID, encrypted GPSK) Decrypt GPSK Verify
Group ID on main chain Check if public key is on the ban-list Join group
by accessing its DHT using GPSK Deny access and notify user of ban
Messaging
Encrypt message with GPSK Store message in the DHT with a key derived
from its content Broadcast the message key to the group members
Recipients decrypt the message after retrieval
Group Management
Admin-Controlled Ban-List
Group admins maintain a ban-list that prevents banned public keys from
accessing the group's DHT. This ban is permanent and ensures that once a
user is excluded, they cannot rejoin under the same identity. The
ban-list is stored in the group's DHT, signed by the admin's private key
for authenticity.
Security Enhancements
Reduced DHT Poisoning: The ban-list mitigates the risk of DHT
poisoning by limiting the participants to trusted members only.Encrypted Messaging for Private Groups: Messages in private
groups are encrypted with GPSK, ensuring confidentiality even if the
DHT is compromised.Efficient Key Management: The system simplifies key management
by integrating the GPSK into the group joining process, reducing
user friction.
Security and Performance
Main Chain Security
Security is ensured through the PoW mechanism, protecting the integrity
of group lifecycle actions. The simplicity of the main chain allows for
faster block times and reduced computational overhead, focusing
resources on message delivery rather than consensus.
DHT Security
Each group's messages are stored in a DHT, with message integrity
maintained through cryptographic hashes. The admin-controlled ban-list
further enhances security by preventing unauthorized access and reducing
the likelihood of DHT poisoning.
Message Privacy
Private Groups: End-to-end encryption using GPSK before storing
messages in the DHT.Public Groups: Messages are stored in plain text to prioritize
performance, with the option for encryption if needed.
Performance Metrics
Throughput: Estimated at 450,000+ msg/s, depending on the number
of active groups and their messaging rates.Latency: DHT-based message retrieval maintains a latency of
approximately 50ms, comparable to centralized systems.
System Comparison
Telelibre is compared against both centralized and decentralized
messaging systems:
Key Advantages:
Decentralization: Offers complete decentralization with robust
security and performance.Scalability: Efficient message storage and retrieval using DHTs.
Censorship Resistance: Decentralized architecture prevents
central authority control over user data.Security: Ban-list and encryption enhance group security and
privacy.
Challenges:
User Participation: Network relies on active user participation
for robustness.Complexity: Initial setup and user experience may be more
complex than centralized alternatives.Global State Updates: Slower than in centralized systems but
balanced by the lightweight nature of the main chain.
Telelibre aims to deliver a decentralized messaging solution that
balances performance, privacy, and security, with practical features
like a ban-list to ensure group integrity and reduce the risk of
attacks.
Appendix: Python Implementation
The following Python script provides a practical implementation of the
Telelibre system. It includes blockchain creation, group management,
message encryption, and performance testing.
import hashlib
import json
import time
# Block class for the Main Chain
class Block:
def __init__(self, index, previous_hash, timestamp, data, nonce=0):
self.index = index
self.previous_hash = previous_hash
self.timestamp = timestamp
self.data = data
self.nonce = nonce
self.hash = self.calculate_hash()
def calculate_hash(self):
block_string = f"{self.index}{self.previous_hash}{self.timestamp}{self.data}{self.nonce}"
return hashlib.sha256(block_string.encode()).hexdigest()
def mine_block(self, difficulty):
target = '0' * difficulty
while self.hash[:difficulty] != target:
self.nonce += 1
self.hash = self.calculate_hash()
# Blockchain class for managing the chain
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
self.difficulty = 4 # Set difficulty for PoW
def create_genesis_block(self):
return Block(0, "0", time.time(), "Genesis Block")
def get_latest_block(self):
return self.chain[-1]
def add_block(self, new_block):
new_block.previous_hash = self.get_latest_block().hash
new_block.mine_block(self.difficulty)
self.chain.append(new_block)
def is_chain_valid(self):
for i in range(1, len(self.chain)):
current_block = self.chain[i]
previous_block = self.chain[i - 1]
if current_block.hash != current_block.calculate_hash():
return False
if current_block.previous_hash != previous_block.hash:
return False
return True
# Group class for handling group creation
class Group:
def __init__(self, group_id, group_type, gpsk=None):
self.group_id = group_id
self.group_type = group_type
self.gpsk = gpsk # Group Private Shared Key for private groups
@staticmethod
def create_group(group_type):
group_id = hashlib.sha256(f"{time.time()}".encode()).hexdigest()
gpsk = None
if group_type == "private":
gpsk = hashlib.sha256(f"{group_id}{time.time()}".encode()).hexdigest()
return Group(group_id, group_type, gpsk)
# DHT class for storing and retrieving messages
class DHT:
def __init__(self):
self.storage = {}
def store_message(self, key, message):
self.storage[key] = message
def retrieve_message(self, key):
return self.storage.get(key, None)
# Message class for handling message creation and encryption
class Message:
def __init__(self, content, group):
self.content = content
self.group = group
self.key = self.calculate_key()
def calculate_key(self):
return hashlib.sha256(self.content.encode()).hexdigest()
def encrypt_message(self):
if self.group.group_type == "private":
return hashlib.sha256(f"{self.content}{self.group.gpsk}".encode()).hexdigest()
return self.content
def store(self, dht):
encrypted_message = self.encrypt_message()
dht.store_message(self.key, encrypted_message)
# Main execution script
def main():
# Initialize the main blockchain
blockchain = Blockchain()
# Create a public group
public_group = Group.create_group("public")
blockchain.add_block(Block(len(blockchain.chain), blockchain.get_latest_block().hash, time.time(), f"Group {public_group.group_id} - open"))
# Create a private group
private_group = Group.create_group("private")
blockchain.add_block(Block(len(blockchain.chain), blockchain.get_latest_block().hash, time.time(), f"Group {private_group.group_id} - open"))
# Initialize DHT for message storage
dht = DHT()
# Measure performance: messages per second
start_time = time.time()
num_messages = 1000 # Simulate storing and retrieving 1000 messages
for i in range(num_messages):
# Create and store public messages
message_public = Message(f"Public message {i}", public_group)
message_public.store(dht)
# Create and store private messages
message_private = Message(f"Private message {i}", private_group)
message_private.store(dht)
# Retrieve messages from DHT to simulate full cycle
dht.retrieve_message(message_public.key)
dht.retrieve_message(message_private.key)
end_time = time.time()
# Calculate performance metrics
total_time = end_time - start_time
messages_per_second = (num_messages * 2) / total_time # *2 because we store and retrieve each message
# Test blockchain integrity
blockchain_valid = blockchain.is_chain_valid()
# Retrieve and test messages
retrieved_message1 = dht.retrieve_message(message_public.key)
retrieved_message2 = dht.retrieve_message(message_private.key)
# Output results
print("==== Blockchain Analysis ====")
print(f"Blockchain valid: {blockchain_valid}")
print(f"Blockchain length: {len(blockchain.chain)}")
print(f"Latest Block Hash: {blockchain.get_latest_block().hash}")
print("\n==== Group and Messaging Analysis ====")
print(f"Public Group ID: {public_group.group_id}")
print(f"Private Group ID: {private_group.group_id}")
print(f"Private Group GPSK: {private_group.gpsk}")
print("\n==== Message Retrieval ====")
print(f"Retrieved Public Message: {retrieved_message1}")
print(f"Retrieved Private Message: {retrieved_message2}")
print(f"Original Private Message (unencrypted): {message_private.content}")
print("\n==== Performance Metrics ====")
print(f"Time to process {num_messages} public and private messages: {total_time:.4f} seconds")
print(f"Estimated Throughput: {messages_per_second:.2f} messages/second")
if __name__ == "__main__":
main()
This content originally appeared on DEV Community and was authored by
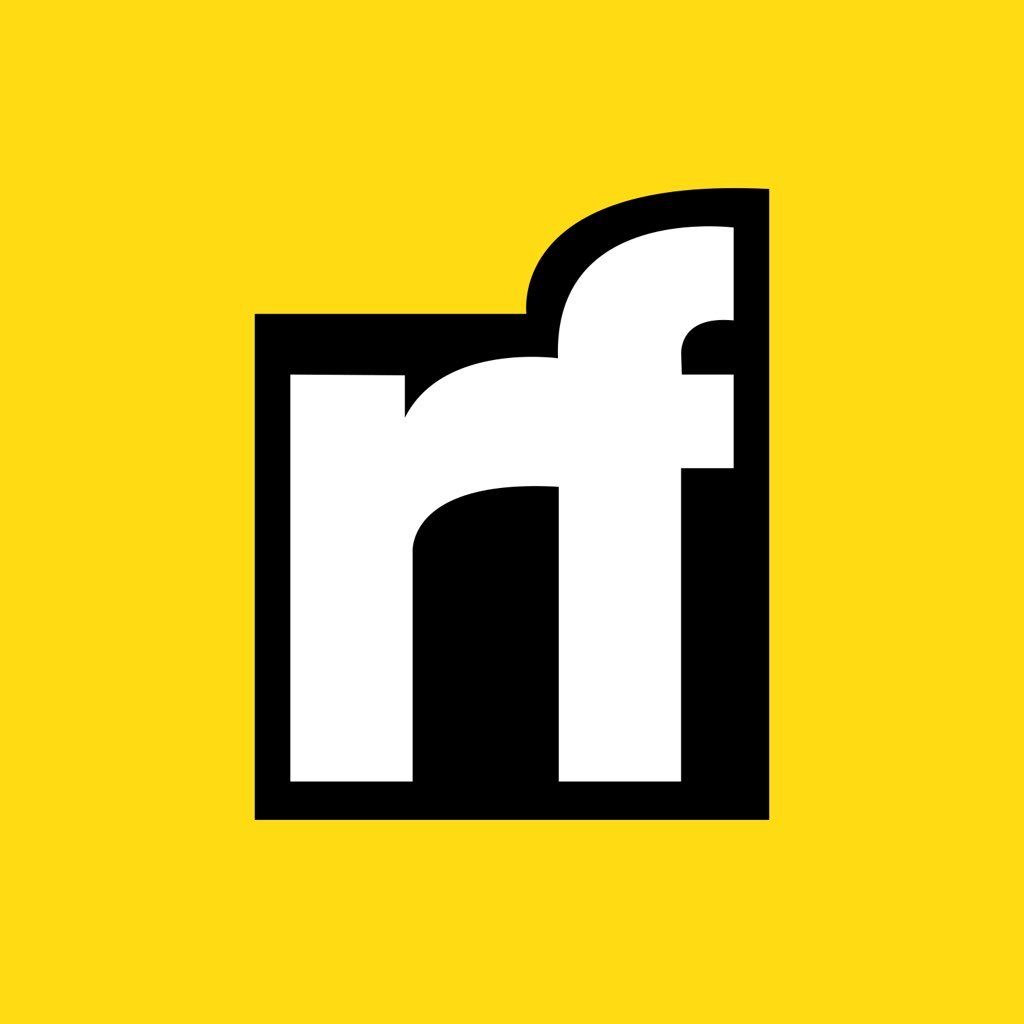
| Sciencx (2024-08-29T01:35:39+00:00) Telelibre: A Practical Decentralized Messaging System. Retrieved from https://www.scien.cx/2024/08/29/telelibre-a-practical-decentralized-messaging-system/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.