This content originally appeared on DEV Community and was authored by Neeraj Sharma
In this post, I'll explain how to develop an authentication API in Rust using the gRPC protocol. The authentication will be based on JWT tokens, and we'll also create a docker file to deploy the application in a docker container. Lastly, I'll provide instructions on how to deploy the app.
How to follow this guide
I will use the full pathname for each file I create, relative to the root of the project
/
, for example,/src/main.rs
.-
I will use
append path-to-symbol
to describe I am writing new content in a file/module/etc. for example:
append/proto/auth.proto/MyService
describe I am writing a new rpc method in theMyService
service.
rpc MyMethod(MyRequest) returns (MyResponse);
create /path/to/filename.ext
: describe I am creating a new file with namefilename
and extensionext
.
Features
- User Registration
- User login
- Password reset/FORGOT password
- OTP verification
- Password Hashing with salt
Crate a new project and open it in vs code editor
cargo new auth_api && cd auth_api && code .
Defining the GRPC scheme for the API
create /proto/auth.proto
append /proto/auth.proto
syntax = "proto3";
package tutorial.api.auth;
service AuthService {
}
Methods to create new account
I will break down the account creation into three methods
- User sends a request to initiate the new user account signup.
- User sends the OTP to verify the email.
- User creates the account with the username, password and other details such as full name gender, birth date, etc.
Let's start with the first method:
First, we need to define the RequestCreateAccount
method to initiate the new user account signup.
append /proto/auth.proto/AuthService
rpc RequestCreateAccount(RequestCreateAccountParams) returns (RequestCreateAccountResponse);
Let's define the request and response message body for this method
append /proto/auth.proto
message RequestCreateAccountParams {
string email_id = 1;
}
message RequestCreateAccountResponse {
bool success = 1;
string message = 2;
string token = 3;
}
Following describes the success response message for the RequestCreateAccount method
{
"success": true,
"message": "Otp was sent to your email",
"token": {
// issuer
"iss": "auth_api",
// subject
"sub": "<email_id>",
// this token should be short lived may be 2 minutes
"exp": <epoch-time>,
// issued at
"iat": <epoch-time>,
// requested permissions this would be granted by the VerifyOtp method
"req_perms": ["CreateAccount"]
}
}
This account creation flow make sure that the user is using his valid email id to create an account.
we can also use phone number for this, but for now, let's keep it simple.
Next define the VerifyOtp
method to verify the email and grant the requested permissions defined in the input token.
append /proto/auth.proto/AuthService
rpc VerifyOtp(VerifyOtpParams) returns (VerifyOtpResponse);
Let's define the request and response message body for this method
append /proto/auth.proto
message VerifyOtpParams {
string token = 1;
string otp = 2;
}
message VerifyOtpResponse {
bool success = 1;
string message = 2;
string token = 3;
}
Following describes the success response message for the VerifyOtp method
{
"success": true,
"message": "Your email is verified",
"token": {
// issuer
"iss": "auth_api",
// subject
"sub": "<email_id>",
// this token should be short lived may be 2 minutes
"exp": <epoch-time>,
// issued at
"iat": <epoch-time>,
// permissions granted to the user
"perms": ["CreateAccount"]
}
}
This method will be used to grant the permissions asked in req_perms
claim of the input token.
Actully this mutate the token with perms
claim. And return it to the client user.
Next create a CreateAccount
method to create the user account with the username, password and other details.
append /proto/auth.proto/AuthService
rpc CreateAccount(CreateAccountParams) returns (CreateAccountResponse);
let's define the request and response message body for this method
append /proto/auth.proto
message CreateAccountParams {
string username = 1;
string password = 2;
// other details
string full_name = 3;
Gender gender = 4;
string birth_date = 5;
}
message CreateAccountResponse {
bool success = 1;
string message = 2;
string session_token = 3;
User user = 4;
}
message User {
string username = 1;
string email = 2;
string full_name = 3;
Gender gender = 4;
string birth_date = 5;
}
enum Gender {
MALE = 0;
FEMALE = 1;
OTHER = 2;
}
Following describes the success response message for the CreateAccount method
{
"success": true,
"message": "Account created successfully",
"session_token": {
"iss": "auth_api",
"sub": "<username>",
"exp": <epoch-time>,
"iat": <epoch-time>,
"perms": ["ApiAccess"]
},
"user": {
"username": "<username>",
"email": "<email>",
"full_name": "<full_name>",
"gender": "<gender>",
"birth_date": "<birth_date>"
}
}
Now we have created all the methods for creating a new account.
Methods to login to the account
Next, we need to define the Login method to login to the account.
User can use either their email or username to login to their account.
First, we need to define the Login
method to login to the account.
append /proto/auth.proto/AuthService
rpc Login(LoginParams) returns (LoginResponse);
Let's define the request and response message body for this method
append /proto/auth.proto
message LoginParams {
string email_or_username = 1;
string password = 2;
}
message LoginResponse {
bool success = 1;
string message = 2;
string session_token = 3;
User user = 4;
}
Success response would have a session token as follows
{
"success": true,
"message": "Login successful",
"session_token": {
"iss": "auth_api",
"sub": "<username>",
"exp": <epoch-time>,
"iat": <epoch-time>,
"perms": ["Session"]
},
"user": {
"username": "<username>",
"email": "<email>",
"full_name": "<full_name>",
"gender": "<gender>",
"birth_date": "<birth_date>"
}
}
Methods to reset the user password
First I will define the InitiateResetPassword
method to initiate the password reset process.
This method will accept the email id and send a password reset token to the user's email id.
Also, server will send a otp code to the user's email id.
append /proto/auth.proto/AuthService
rpc InitiateResetPassword(InitiateResetPasswordParams) returns (InitiateResetPasswordResponse);
Let's define the parameters and response message body for this method
append /proto/auth.proto
message InitiateResetPasswordParams {
string email_id = 1;
}
message InitiateResetPasswordResponse {
bool success = 1;
string message = 2;
string token = 3;
}
A success response for the InitiateResetPassword
method will have a password reset token as follows
{
"success": true,
"message": "Password reset token sent to your email",
"token": {
"iss": "auth_api",
"sub": "<email_id>",
"exp": <epoch-time>,
"iat": <epoch-time>,
"req_perms": ["ResetPassword"]
}
}
the req_perms claim in the token describes that the user will be granted the permission to reset the password after giving right otp code to the VerifyOtp
method. We already have VerifyOtp
method in the previous section.
Let's define the ResetPassword
method to reset the user password.
ResetPassword method will accept the password reset token and the new password.
append /proto/auth.proto/AuthService
rpc ResetPassword(ResetPasswordParams) returns (ResetPasswordResponse);
Let's define the parameters and response message body for this method
append /proto/auth.proto
message ResetPasswordParams {
string token = 1;
string new_password = 2;
}
message ResetPasswordResponse {
bool success = 1;
string message = 2;
}
A success response for the ResetPassword
method will have a message as follows
{
"success": true,
"message": "Password reset successful"
}
Conclusion
We have defined the GRPC scheme for the authentication API. For now it supports create account, login, reset password in case if the user forgot their password. This also includes the OTP verification to make sure the user is using the valid email id.
In the next part, we will start coding the API in Rust.
See you in the next part.
Bye.
This content originally appeared on DEV Community and was authored by Neeraj Sharma
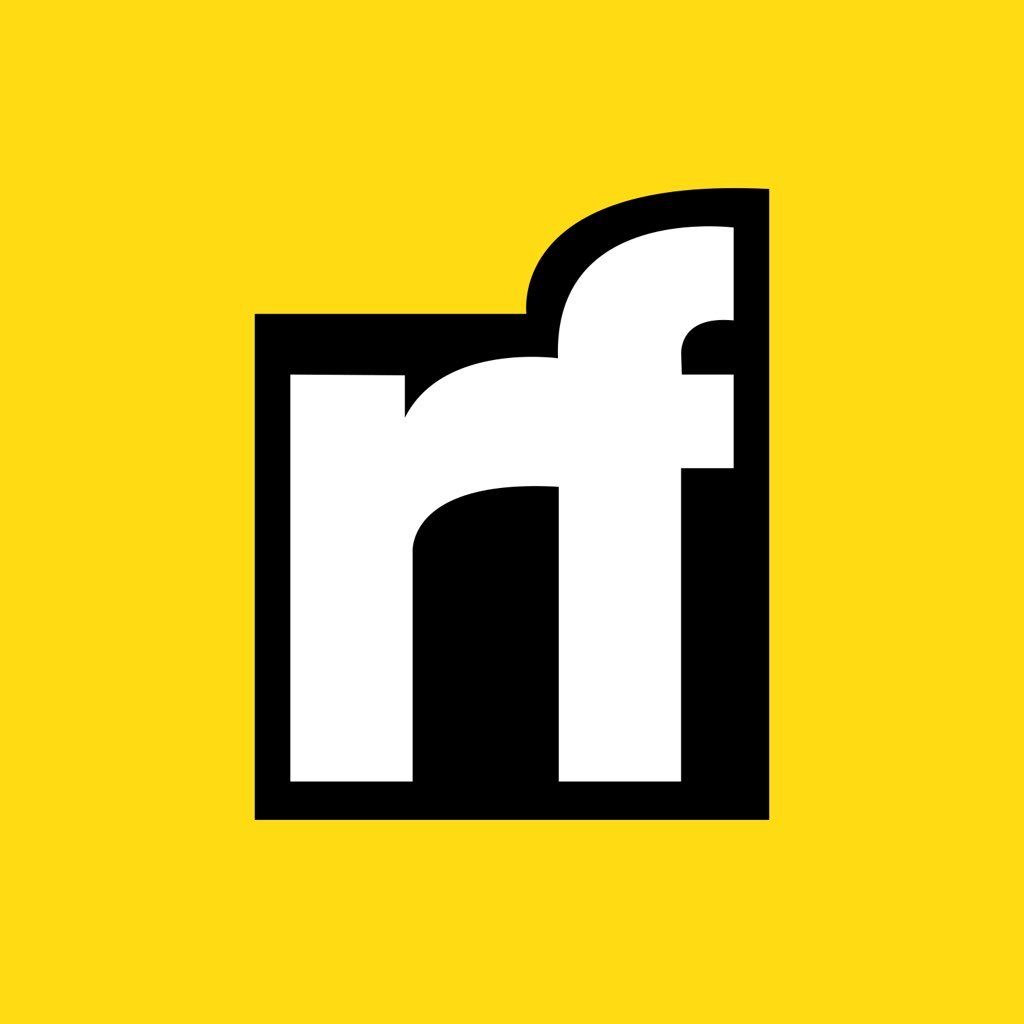
Neeraj Sharma | Sciencx (2024-09-03T00:00:00+00:00) Part 1: Defining the GRPC scheme. Retrieved from https://www.scien.cx/2024/09/03/part-1-defining-the-grpc-scheme/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.