This content originally appeared on DEV Community and was authored by mohamed Tayel
In this guide, we will explore Entity Framework Core Migrations—a key tool for managing database schema changes over the lifetime of your application. From adding, applying, and rolling back migrations to handling advanced scenarios like table splits, data transformations, and managing migrations in production, this article will help you master EF Core migrations. By the end, you'll be able to apply migrations with confidence in both development and production environments.
1. Introduction to Migrations in EF Core
EF Core migrations provide a structured way to keep your database schema synchronized with your application’s data model as it evolves. This is essential for making sure your database remains up-to-date without losing data.
Why Use Migrations?
- Incrementally update your database schema without needing to rebuild the entire database.
- Keep track of schema changes in version control alongside your code.
- Generate and apply SQL scripts for changes in both development and production environments.
Key Commands Overview:
- Add Migration: Generates a migration file based on changes in your model classes.
- Update Database: Applies the migration to the database.
- Remove Migration: Deletes the most recent migration.
- Rollback Migration: Reverts the database to a previous migration.
2. Adding, Applying, and Rolling Back Migrations
Let’s walk through how to add, apply, and roll back migrations in EF Core.
Step-by-Step Example:
We’ll use an example of an Event Management system where you manage Events
, Categories
, and Attendees
. Suppose you add a new property Location
to your Event
class:
public class Event
{
public int EventId { get; set; }
public string Name { get; set; }
public DateTime Date { get; set; }
public string Location { get; set; } // New property
}
Adding a Migration
After making changes to your model, you need to add a migration to reflect those changes in the database schema.
Command:
dotnet ef migrations add AddLocationToEvent
EF Core will generate a migration file, capturing the schema change (adding the Location
column):
public partial class AddLocationToEvent : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.AddColumn<string>(
name: "Location",
table: "Events",
type: "nvarchar(max)",
nullable: true);
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropColumn(
name: "Location",
table: "Events");
}
}
Applying the Migration
To apply the migration and update the database, use the following command:
Command:
dotnet ef database update
This command applies the Up
method of the migration to your database, adding the Location
column to the Events
table.
Rolling Back a Migration
To roll back the most recent migration, use:
Command:
dotnet ef database update PreviousMigrationName
This applies the Down
method, reverting the changes made by the migration.
Alternatively, to remove the most recent migration entirely if it hasn't been applied:
Command:
dotnet ef migrations remove
3. Complex Model Changes
As your application evolves, you may need to handle more complex changes to your data model, such as renaming columns, splitting tables, or transforming data.
Scenario: Splitting a Table into Two
Let’s say you decide to normalize your Event
table by moving some columns to a new EventDetails
table.
-
Create the
EventDetails
Entity:
public class EventDetails
{
public int EventDetailsId { get; set; }
public string Description { get; set; }
public int EventId { get; set; }
public Event Event { get; set; }
}
-
Update your
Event
Entity:
public class Event
{
public int EventId { get; set; }
public string Name { get; set; }
public EventDetails EventDetails { get; set; }
}
- Create a migration:
dotnet ef migrations add SplitEventTable
-
Modify the migration to move data:
Edit the migration file to add SQL logic that moves data from the
Events
table toEventDetails
.
migrationBuilder.Sql(@"
INSERT INTO EventDetails (EventId, Description)
SELECT EventId, Description FROM Events
");
- Apply the migration:
dotnet ef database update
4. Renaming Tables or Columns
Renaming columns or tables in EF Core needs special care to prevent data loss, as EF Core will otherwise drop the old column and create a new one.
Scenario: Renaming a Column
Suppose you want to rename EventName
to Name
in your Event
entity.
- Update the model:
public class Event
{
public int EventId { get; set; }
public string Name { get; set; } // Renamed from EventName
}
- Create a migration:
dotnet ef migrations add RenameEventNameToName
-
Modify the migration:
In the generated migration, use
RenameColumn
instead of dropping and adding columns to avoid data loss:
migrationBuilder.RenameColumn(
name: "EventName",
table: "Events",
newName: "Name"
);
- Apply the migration:
dotnet ef database update
5. Managing Migrations Across Multiple Environments
When dealing with multiple environments like development, staging, and production, keeping your migrations synchronized can be a challenge.
Scenario: Handling Migrations in Multiple Environments
- Generate migration scripts: Always generate SQL scripts for migrations that will be applied to production. This allows for proper review and testing.
Command:
dotnet ef migrations script
Apply migrations manually in production: Execute the generated SQL script manually in production after testing it in a staging environment.
Version control migrations: Ensure that migration files are tracked in version control, so all team members and environments are aligned.
6. Managing Data Migrations
Sometimes you need to migrate data along with your schema changes. For example, transforming data from one format to another or copying data from one column to another.
Scenario: Migrating Data from One Column to Another
Let’s say you want to migrate the EventDate
column from DateTime
to a string
format.
-
Add the new column:
In the migration, add the new
EventDateString
column and migrate the data.
migrationBuilder.AddColumn<string>(
name: "EventDateString",
table: "Events",
nullable: true);
migrationBuilder.Sql(@"
UPDATE Events
SET EventDateString = CONVERT(VARCHAR, EventDate, 23)
");
migrationBuilder.DropColumn(name: "EventDate", table: "Events");
- Apply the migration:
dotnet ef database update
7. Using Migrations in Production
In production environments, applying migrations directly from the application can be risky. Instead, you should generate and review SQL scripts before applying them.
Best Practices for Production Migrations:
- Generate migration scripts:
dotnet ef migrations script
This generates a SQL script that can be reviewed and run manually in production.
Always backup your database: Before applying any migration to production, ensure that you have a recent backup.
Test in staging: Apply your migration scripts in a staging environment first to ensure they work as expected.
8. Seeding the Database with Initial Data
Seeding the database is useful for populating tables with initial data, such as lookup tables or default configurations.
Example: Seeding Categories
You can seed data directly in your DbContext
:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Category>().HasData(
new Category { CategoryId = 1, Name = "Technology" },
new Category { CategoryId = 2, Name = "Health" },
new Category { CategoryId = 3, Name = "Business" }
);
}
- Add the migration:
dotnet ef migrations add SeedCategories
- Apply the migration:
dotnet ef database update
This will insert the specified seed data into the database.
Conclusion
In this article, we explored both basic and advanced usage of **Entity Framework Core Migrations
**. From adding and applying migrations to handling complex model changes, data migrations, and managing multiple environments, you now have a complete toolkit for maintaining your database schema with EF Core.
By following these best practices and using the techniques outlined here, you can ensure your application’s database schema evolves smoothly without risking data loss or downtime, especially in production environments.
This content originally appeared on DEV Community and was authored by mohamed Tayel
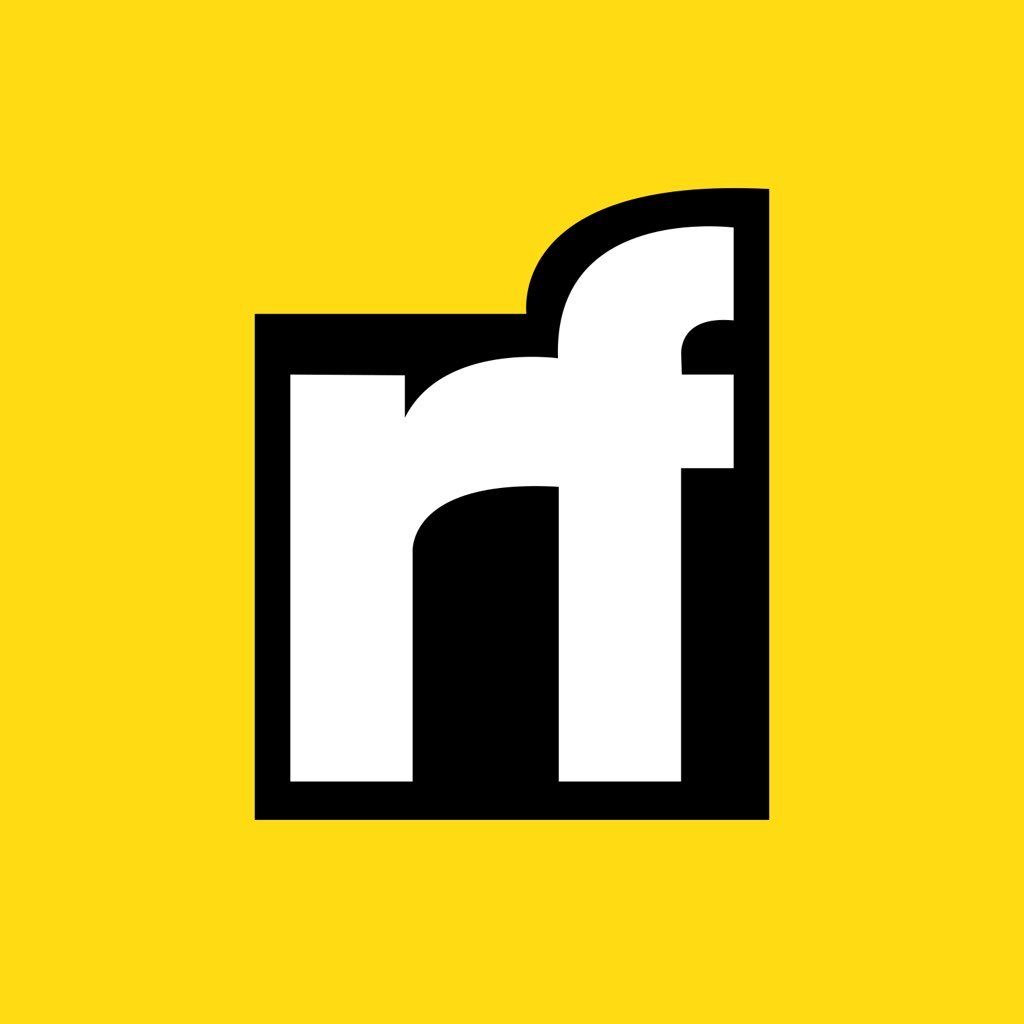
mohamed Tayel | Sciencx (2024-09-06T21:13:21+00:00) Entity Framework Core Tutorial:EF Core Migrations. Retrieved from https://www.scien.cx/2024/09/06/entity-framework-core-tutorialef-core-migrations/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.