This content originally appeared on DEV Community and was authored by Mr-peipei
Introduction
When you develop A React application, you have to manage the state.
And you have to think about the number of states.
If you use the state in the wrong way, the state size will be bigger and bigger.
And the program will become more complex.
So, you have to reduce the number of states.
In this article, I will show you how to reduce the number of states.
1. Read the official document !!!(Important)
In the React official document, you can find the best practices to manage the state.
https://react.dev/learn/choosing-the-state-structure
This document is very useful for manage the state, but most people doesn't realize they have a problem with the state.
So, I recommend you read this document first.
And I will introduce some example tips written in this document.
1.1 Try to use object state.
There are many ways to manage the state.
If you update the state in same time, you can use object state.
This is the bad example.
const [ name, setName ] = useState<string>('');
const [ age, setAge ] = useState<number>(0);
This is bad because if you read the state program, you might think that name and age are not related and not synchronized. And you would have to search the code to find the relationship between name and age.
In this case, you can use object state, just like this.
const [ user, setUser ] = useState<{ name: string, age: number }>({ name: '', age: 0 });
And you can use this state like this.
const onChangeName = (e: React.ChangeEvent<HTMLInputElement>) => {
setUser({ ...user, name: e.target.value });
};
But you have to be careful about updating the object state in one statement.
You have to use the spread operator to update the object state.
You cannot use this code.
// This is wrong.
const onChangeName = (e: React.ChangeEvent<HTMLInputElement>) => {
setUser({ name: e.target.value });
};
This tips also written in the official document.
So you have to read the official document first.
2. Create common hook.
To reduce the number of program steps, you need to eliminate unused state.
Most of the time, you will use the same state in multiple components.
// Page1.tsx
const [ notification, setNotification ] = useState<Notification | null>(null);
// Page2.tsx
const [ notification, setNotification ] = useState<Notification | null>(null);
// Page3.tsx
const [ notification, setNotification ] = useState<Notification | null>(null);
But this is not good because you have to write this code in every page component. And if you want to change the state name, you have to change the code in every page component. So, you have to create a common hook.
import { useState } from 'react';
export const useNotification = () => {
const [ notification, setNotification ] = useState<Notification | null>(null);
return { notification, setNotification };
};
And you can use this hook in every page component.
import { useNotification } from './hooks/useNotification';
const Page = () => {
const { notification, setNotification } = useNotification();
};
3. Use Global Context or Global state library
Global Context and Global state libraries are very useful for sharing state between components. This is an advanced version of the common hook.
If you retrieve user information on the login page, you need to share this information with other pages. In such cases, you can utilize global context or a global state library.
import { createContext, useContext, useState } from 'react';
const UserContext = createContext<{ user: User | null, setUser: (user: User) => void }>({
user: null,
setUser: () => {},
});
export const UserProvider: React.FC = ({ children }) => {
const [ user, setUser ] = useState<User | null>(null);
return (
<UserContext.Provider value={{ user, setUser }}>
{children}
</UserContext.Provider>
);
};
export const useUser = () => {
const { user, setUser } = useContext(UserContext);
return { user, setUser };
};
And you can use this hook in every page component.
import { useUser } from './hooks/useUser';
const Page = () => {
const { user, setUser } = useUser();
};
Don't call the API fetch in every page component. Instead, you can use global state to share the API fetch result.
However, when using global state, don't forget to reset the state when you no longer need it. Otherwise, the state will be kept in memory until the page is closed. Therefore, I also recommend creating a reset hook.
export const useResetUser = () => {
const { setUser } = useContext(UserContext);
return () => setUser(null);
};
There are many libraries for global state. For example, Redux, Recoil, Mobx, etc.
I recommend Recoil. Because it is very simple and easy to use.
4. Try to use openapi generator
If you use openapi, you can create react api fetch client code automatically.
This is very useful to reduce the number of program steps.
You can generate the api client code from openapi yaml file.
npx @openapitools/openapi-generator-cli generate -i openapi.yaml -g typescript-fetch -o api
And you can use this api client code like this.
import { api } from './api';
const Page = () => {
const fetchUser = async () => {
const user = await api.getUser();
};
};
And some type of openapi generator can generate the react hook code.
npx @openapitools/openapi-generator-cli generate -i openapi.yaml -g typescript-react-query -o api
And you can use this api client code like this.
import { useGetUserQuery } from './api';
const Page = () => {
const { data, error, isLoading } = useGetUserQuery();
};
So, you don't have to create loading state, error state, and data state.
Of course, you have to maintenance open api yaml file, and think about development process.
But this is very useful for reduce the number of program steps.
Conclusion
In this article, I showed you how to reduce the number of state.
But this is not the only way to reduce the number of states.
You have to think about the state structure, and the state relationship.
And you have to think about the state usage.
I hope this article will help you to reduce the number of state.
Thank you for reading.
This content originally appeared on DEV Community and was authored by Mr-peipei
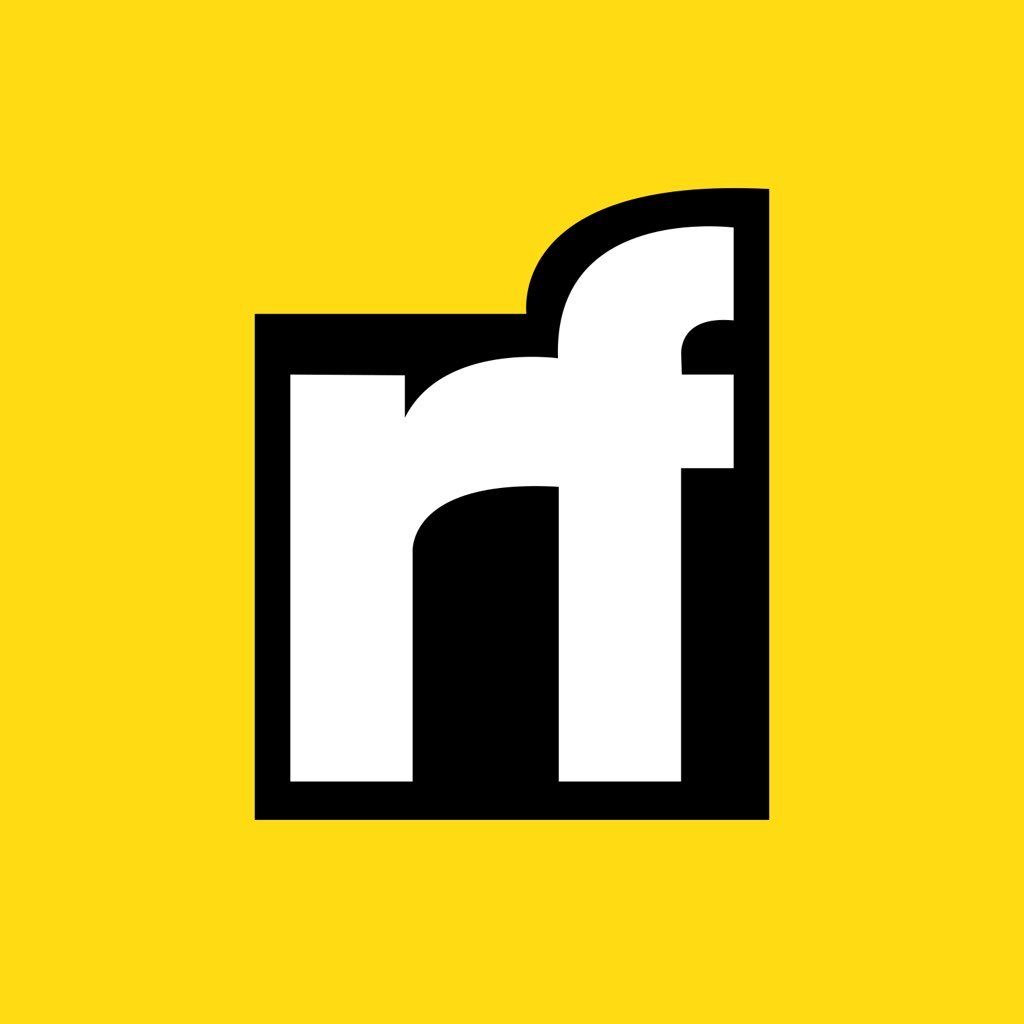
Mr-peipei | Sciencx (2024-09-07T03:16:14+00:00) Mastering State Management in React: Tips and Best Practices. Retrieved from https://www.scien.cx/2024/09/07/mastering-state-management-in-react-tips-and-best-practices-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.