This content originally appeared on DEV Community and was authored by meenachan
In the realm of development, every day can feel like an epic adventure. One fateful Tuesday, I found myself facing a formidable beast: a tidal wave of urgent user requests threatening to overwhelm my code. With deadlines looming and the clock ticking, I needed a hero’s strategy to conquer this chaos.
As the number of user requests surged, I realized that my initial approach—a simple filtering loop—was insufficient for managing the diverse types of requests pouring in. Urgent requests mixed with complex and incomplete ones created a tangled mess that slowed down processing and left users impatiently waiting. I needed a solution that could efficiently handle this variety while ensuring that urgent requests were prioritised.
What began as a simple filtering task quickly morphed into a high-stakes race against time, pushing my coding skills to their limits.
The Initial Struggle
I started simply, filtering requests with a basic loop:
List<Person> urgentRequests = new ArrayList<>();
for (Person person : people) {
if (person.isUrgent()) {
urgentRequests.add(person);
}
}
This worked well for a manageable list, but as the requests soared from hundreds to thousands, my code crawled, leaving users impatiently waiting.
The Parallel Stream Hail Mary
Desperate for speed, I turned to parallel streams, hoping to unleash some serious processing power:
List<Person> urgentRequests = people.parallelStream()
.filter(Person::isUrgent)
.collect(Collectors.toList());
While I felt like a coding hero, soon I realized that the varying request types—simple, complex, incomplete, and nested—overwhelmed my newfound speed. The chaos continued, and users were still in limbo.
The Spliterator Revelation đź’ˇ
In a moment of clarity, I discovered Spliterator.
What is Spliterator?
A Spliterator (short for "splittable iterator") is a Java interface introduced in Java 8 that allows you to traverse and partition elements of a data structure. Unlike a standard iterator, which processes elements sequentially, a Spliterator can divide a collection into smaller parts, enabling parallel processing. This is particularly useful for collections that have diverse or nested structures.
Key Characteristics of Spliterator
- Splitting: It can break down a data structure into smaller chunks, which can then be processed concurrently.
-
Characteristics: It provides information about the data, such as whether it is
SIZED
,SUBSIZED
,ORDERED
, orCONCURRENT
. - Efficiency: It allows for efficient traversal and can adapt to various data types and structures.
Key Methods of Spliterator
-
tryAdvance(Consumer<? super T> action)
: Performs the given action on the next element if one exists, returning true if an element was processed. -
trySplit()
: Attempts to split the spliterator into two parts, returning a new spliterator for the first part and leaving the second part for the original. -
estimateSize()
: Returns an estimate of the number of elements that remain to be processed. -
characteristics()
: Returns a set of characteristics for this spliterator.
When to Use Spliterator
- Complex Data Structures: When working with collections that have diverse or nested structures, Spliterator can handle each element more intelligently.
- Custom Processing Logic: If you need specific handling for different types of elements, Spliterator allows for that flexibility.
- Parallel Processing Needs: When you want fine control over how elements are processed in parallel, Spliterator shines.
The New Adventure: Spliterator Code 🛠️
I revamped my code to use Spliterator, ready to tackle each request type head-on:
List<Person> urgentRequests = new ArrayList<>();
List<Person> incompleteRequests = new ArrayList<>();
Spliterator<Person> spliterator = people.spliterator();
spliterator.forEachRemaining(person -> processRequest(person, urgentRequests, incompleteRequests));
In this code, I created two lists: one for urgent requests and another for incomplete ones. The spliterator.forEachRemaining()
method processes each Person
, applying tailored logic to each request.
Processing Different Requests
I defined the processRequest
method to handle the various types:
private static void processRequest(Person person, List<Person> urgentRequests, List<Person> incompleteRequests) {
if (person.isUrgent()) {
if (person.isSimpleRequest()) {
urgentRequests.add(person); // Quick and easy
} else if (person.isComplexRequest()) {
handleComplexRequest(person); // Handle complex scenarios
} else if (person.isIncompleteRequest()) {
incompleteRequests.add(person); // Log for later
} else if (person.isNestedRequest()) {
handleNestedRequest(person); // Address nested issues
}
}
}
- Simple Requests go straight to the urgent list.
- Complex Requests are handled in detail.
- Incomplete Requests are logged for follow-up.
- Nested Requests require specialized logic.
Victory!🚀
As I executed the updated code, it felt like crossing the finish line of a marathon. Spliterator handled the chaos beautifully, ensuring urgent requests were prioritized while incomplete ones were noted for future attention.
Final Thoughts: Conquer Your Coding Challenges! 🏆
To all you coding sorcerers out there, remember: with tools like Spliterator, you can transform chaos into clarity. Embrace the magic, and may your code thrive!
This content originally appeared on DEV Community and was authored by meenachan
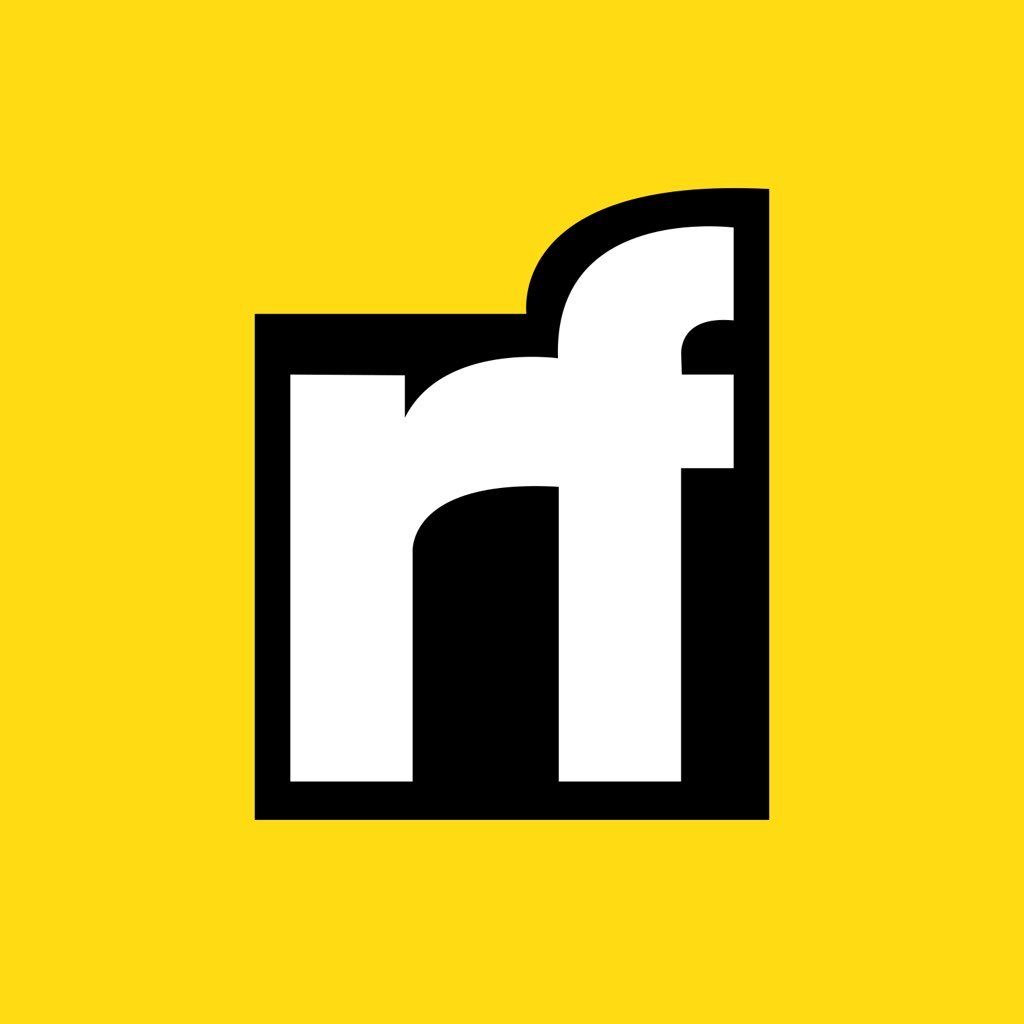
meenachan | Sciencx (2024-09-22T16:31:43+00:00) Code Quest: Taming the Request Beast with Spliterator Magic! 🧙‍♂️✨. Retrieved from https://www.scien.cx/2024/09/22/code-quest-taming-the-request-beast-with-spliterator-magic-%f0%9f%a7%99%e2%99%82%ef%b8%8f%e2%9c%a8/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.