This content originally appeared on Level Up Coding - Medium and was authored by CyCoderX
Boost SQL query speed with indexing, refactoring, and efficient joins!
In today’s data-driven world, databases are at the heart of many applications, supporting everything from real-time analytics to large-scale transaction processing. As your data grows, so does the complexity of your SQL queries, and without proper optimization, even simple queries can cause significant performance bottlenecks.
SQL (Structured Query Language) provides a powerful toolkit for retrieving and manipulating data, but writing efficient queries is essential to ensuring that your database operations run smoothly and quickly. Whether you’re managing a small project or working with large-scale data systems, optimizing your SQL queries can make a massive difference in performance.
Hi! My name is CyCoderX. In this article, we will explore various strategies for improving SQL query performance. From indexing to query refactoring, and common pitfalls to avoid, we’ll provide you with actionable tips to ensure your queries are as fast and efficient as possible.
Let’s dive in!
Did you know that you can clap up to 50 times ? Well now you do! Please consider helping me out by clapping and following me! 😊
Database SQL Sagas By CyCoderX
Why Query Performance Matters
Optimizing SQL queries is not just about making your application run faster; it’s about ensuring the long-term scalability, reliability, and efficiency of your database systems. As databases grow in size and complexity, poorly optimized queries can lead to a range of issues, including:
Slower Application Performance:
- Unoptimized queries take longer to execute, causing delays in loading times and response times. For applications with real-time data requirements, this can create a poor user experience.
Increased Resource Usage:
- Inefficient queries consume more CPU, memory, and disk I/O resources. As queries grow in complexity, they can monopolize system resources, impacting the performance of other operations and slowing down the entire database.
Bottlenecks in Large-Scale Systems:
- In systems that handle large datasets or high transaction volumes, slow queries can create bottlenecks, limiting the overall throughput of the system. Over time, these bottlenecks can lead to performance degradation, even for simple operations.
Cost Implications:
- For cloud-based databases or managed services, resource-intensive queries can result in higher costs. Database vendors typically charge based on resource usage, so optimizing your queries can directly translate to reduced operational costs.
Improved User Satisfaction:
- In a world where users expect fast, real-time responses, slow database performance can frustrate users and negatively impact your business. Optimized queries can help maintain a smooth, seamless experience for end users.
Ensuring Scalability:
- Optimizing queries early on ensures your database can scale as your application grows. As your data expands, having efficient queries will minimize the risk of performance degradation, allowing your system to handle increasing data loads without major issues.
In summary, optimizing SQL queries is essential for maintaining high performance, reducing resource consumption, and ensuring that your application can scale smoothly as it grows. In the following sections, we’ll explore specific techniques to achieve these goals.
Building a Port Scanner in Python
Use Indexes Effectively
Indexes are one of the most powerful tools for improving SQL query performance. They allow the database to find rows faster, much like how an index in a book helps you quickly locate a specific topic. However, creating and using indexes requires careful planning, as poorly implemented indexes can negatively affect performance.
Key Strategies for Using Indexes:
Create Indexes on Frequently Queried Columns:
- Index columns that are used frequently in WHERE, JOIN, and ORDER BY clauses. For example, if you often filter data by customer_id, create an index on this column:
CREATE INDEX idx_customer_id
ON customers(customer_id);
Use Composite Indexes for Multiple Columns:
- When filtering or sorting on multiple columns, a composite (multi-column) index can improve performance. However, the order of columns in the index matters. The most frequently queried column should come first.
CREATE INDEX idx_customer_order
ON orders(customer_id, order_date);
Avoid Over-Indexing:
- While indexes speed up read operations, they slow down write operations (INSERT, UPDATE, DELETE) since the index needs to be updated every time the data changes. Only index the columns you really need to, and periodically review unused indexes.
Monitor Index Usage:
- Use database tools like EXPLAIN (in MySQL) or EXPLAIN ANALYZE (in PostgreSQL) to analyze how your queries are utilizing indexes. If your indexes aren’t being used, the query may not be optimized to take advantage of them.
Clustered vs. Non-Clustered Indexes:
- Use clustered indexes for primary key columns where data retrieval is most common. Non-clustered indexes are useful for other frequently queried columns. Understanding the difference between these types can help in making efficient indexing decisions.
Indexes are a double-edged sword: they can dramatically improve query speed, but over-indexing can lead to performance penalties during data writes. Understanding when and where to use indexes is key to unlocking faster query performance.
Coffee Shop Implementation in Python
Optimize WHERE Clauses
The WHERE clause is critical to SQL query performance, as it defines which rows are returned. Poorly written WHERE clauses can lead to full table scans, which are slow and resource-intensive, especially with large datasets.
Techniques to Optimize WHERE Clauses:
Use Index-Friendly Conditions:
- Ensure that the conditions in your WHERE clause allow the database to utilize indexes. For example, avoid using functions on indexed columns, as this prevents the index from being used:
-- Avoid this:
SELECT *
FROM users
WHERE LOWER(username) = 'john';
-- Do this instead:
SELECT *
FROM users
WHERE username = 'JOHN';
-- Ensure data is normalized for consistent comparison
Leverage Selective Filtering:
- The more selective your WHERE clause, the faster your query will be. Selectivity refers to the proportion of rows returned compared to the total rows in the table. For example, filtering on a primary key is highly selective, whereas filtering on a common column like gender may not be.
SELECT *
FROM orders
WHERE order_id = 12345; -- Highly selective
Use EXISTS Instead of IN:
- When working with subqueries, EXISTS is generally more efficient than IN, especially for large datasets. The EXISTS clause returns true as soon as a match is found, while IN checks all values.
-- Prefer EXISTS over IN
SELECT *
FROM customers
WHERE EXISTS
(SELECT 1 FROM orders
WHERE customers.customer_id = orders.customer_id);
Avoid Wildcards at the Beginning of Strings:
- When using the LIKE operator, avoid starting the pattern with a wildcard, as this will result in a full table scan. Indexes can be used with LIKE only if the pattern begins with a constant.
-- This prevents index usage:
SELECT *
FROM products
WHERE name
LIKE '%phone';
-- This allows index usage:
SELECT *
FROM products
WHERE name LIKE 'iPhone%';
Use Logical Operators Efficiently:
- Avoid redundant conditions in your WHERE clause. Use AND and OR wisely to avoid unnecessary comparisons that slow down performance.
Optimizing your WHERE clauses ensures that your queries filter data more efficiently, reducing the amount of data processed and improving overall query performance.
Using the ChatGPT API in Your Projects
Automate Meeting Summaries Using the ChatGPT API with Python
Limit the Data You Retrieve
One of the easiest ways to optimize SQL queries is by limiting the amount of data you retrieve. Querying unnecessary data not only increases the load on the database but also makes your application slower and less responsive. By fetching only what you need, you can significantly improve performance.
Techniques to Limit Data Retrieval:
Use SELECT with Specific Columns:
- Avoid using SELECT * unless you truly need all columns. Fetching unnecessary columns consumes more memory and bandwidth, especially when dealing with large tables.
-- Avoid this:
SELECT *
FROM employees;
-- Instead, select only necessary columns:
SELECT first_name, last_name, email
FROM employees;
Implement LIMIT or TOP Clauses:
- If you only need a subset of rows, use LIMIT (in MySQL or PostgreSQL) or TOP (in SQL Server) to restrict the number of rows returned by the query.
-- MySQL/PostgreSQL:
SELECT *
FROM orders
WHERE status = 'Completed'
LIMIT 10;
-- SQL Server:
SELECT TOP 10 *
FROM orders
WHERE status = 'Completed';
Filter with Pagination:
- When dealing with large datasets, use pagination techniques to load data in chunks. This not only reduces the initial load time but also makes the application more user-friendly by loading data incrementally.
SELECT *
FROM products
WHERE category = 'Electronics'
ORDER BY product_id
LIMIT 20
OFFSET 40;
- This query fetches 20 rows, skipping the first 40, which is useful for loading pages of data.
Avoid Unnecessary Joins:
- Be mindful when joining tables. If you don’t need data from a joined table, avoid performing the join. Unnecessary joins can increase query complexity and slow down execution.
Use Aggregates and HAVING Wisely:
- When aggregating data (e.g., using COUNT, SUM, AVG), ensure that you filter unnecessary rows before aggregation. You can use the HAVING clause to filter aggregated results, but it's more efficient to filter rows beforehand using the WHERE clause.
SELECT department, COUNT(*) AS total_employees
FROM employees
WHERE hire_date > '2020-01-01'
GROUP BY department;
By retrieving only the necessary data, you can reduce the workload on your database, minimize resource consumption, and speed up query response times.
Refactor Complex Queries
Complex queries can often be optimized by refactoring them into simpler, more efficient versions. Breaking down complex operations into smaller, manageable steps can help improve readability, maintainability, and performance.
Techniques to Refactor Complex Queries:
Break Queries into Subqueries or Common Table Expressions (CTEs):
- If your query contains complex calculations or joins, consider breaking it into subqueries or using CTEs. This makes the query easier to read and allows the database to optimize execution.
-- Using a Common Table Expression (CTE):
WITH SalesData AS (
SELECT product_id, SUM(sales_amount) AS total_sales
FROM sales
GROUP BY product_id
)
SELECT product_id, total_sales
FROM SalesData
WHERE total_sales > 10000;
CTEs help organize complex logic and can improve performance, especially with large datasets.
Avoid Nested Subqueries When Possible:
- While subqueries can be helpful, deeply nested subqueries can become slow and difficult to maintain. If possible, rewrite them as joins or CTEs to improve performance.
-- Instead of this nested subquery:
SELECT *
FROM customers
WHERE customer_id IN
(SELECT customer_id
FROM orders
WHERE total > 500);
-- Consider using a JOIN:
SELECT DISTINCT customers.*
FROM customers
JOIN orders ON customers.customer_id = orders.customer_id
WHERE orders.total > 500;
Simplify Joins and Conditions:
- Complex joins and conditions can slow down query execution, especially with large tables. Review your query logic and simplify joins and WHERE conditions where possible. Use inner joins over outer joins if you don’t need data from non-matching rows.
-- Simplifying conditions:
SELECT *
FROM employees
WHERE (hire_date >= '2020-01-01' AND hire_date <= '2021-01-01');
-- Can be simplified to:
SELECT *
FROM employees
WHERE hire_date
BETWEEN '2020-01-01' AND '2021-01-01';
Decompose OR Clauses:
- OR clauses can prevent the database from utilizing indexes efficiently. When possible, refactor OR conditions to use UNIONs or break the query into separate conditions.
-- Instead of using OR:
SELECT *
FROM orders
WHERE status = 'Pending' OR status = 'Shipped';
-- Use UNION:
SELECT *
FROM orders
WHERE status = 'Pending'
UNION
SELECT *
FROM orders
WHERE status = 'Shipped';
Test and Compare Execution Plans:
- After refactoring a complex query, always compare the execution plan before and after the change. Use tools like EXPLAIN to see how the database processes the query and whether optimizations have improved performance.
Refactoring complex queries improves not only performance but also readability and maintainability. Breaking down complex logic into simpler, more efficient components ensures that your queries are both scalable and easier to debug.
What is PEP 8 and Why is it Important in Python?
Use Joins Efficiently
Joins are essential for combining data from multiple tables, but if not used efficiently, they can become a major source of performance issues, especially with large datasets. Properly optimizing how you use joins can significantly reduce query execution time.
Techniques for Efficient Use of Joins:
Choose the Right Type of Join:
Always use the most appropriate join type for your query. The most common join types are:
- INNER JOIN: Returns rows where there is a match in both tables.
- LEFT JOIN (or LEFT OUTER JOIN): Returns all rows from the left table, plus matched rows from the right table.
- RIGHT JOIN (or RIGHT OUTER JOIN): Returns all rows from the right table, plus matched rows from the left table.
- FULL OUTER JOIN: Returns all rows when there is a match in either table.
Use INNER JOIN when you only need matching rows. Outer joins should be used only when necessary, as they are generally slower.
-- Use INNER JOIN for matching rows:
SELECT employees.first_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
Index Join Columns:
- To improve join performance, ensure that the columns being joined are indexed. This allows the database to retrieve matching rows faster.
-- Create indexes on join columns:
CREATE INDEX idx_employee_department ON employees(department_id);
Avoid Joining Too Many Tables:
- Each join adds complexity to your query and can increase execution time. Avoid joining too many tables in a single query. If possible, break down complex joins into multiple steps or use Common Table Expressions (CTEs).
-- Consider simplifying this query:
SELECT *
FROM table1
JOIN table2 ON table1.id = table2.id
JOIN table3 ON table2.id = table3.id;
-- Break into multiple steps or use CTEs for clarity.
Use Joins Instead of Subqueries:
- In many cases, joins are more efficient than subqueries. Subqueries can often lead to slower query execution as they are evaluated for each row. Try rewriting subqueries using joins when possible.
-- Instead of a subquery:
SELECT *
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE department_name = 'HR');
-- Use a join:
SELECT employees.*
FROM employees
JOIN departments ON employees.department_id = departments.department_id
WHERE departments.department_name = 'HR';
Use Appropriate Join Conditions:
- Be specific with join conditions to avoid returning unnecessary rows. Make sure you are joining on columns that ensure the correct data relationship between tables, such as primary and foreign keys.
-- Use a precise join condition:
SELECT orders.order_id, customers.customer_name
FROM orders
JOIN customers ON orders.customer_id = customers.customer_id;
Efficiently handling joins is critical to reducing query execution time and improving performance, especially when working with large databases. By selecting the right join type, indexing join columns, and limiting complex joins, you can dramatically improve the speed of your queries.
Python Airflow Project For Your GitHub!
Monitor and Tune Your Queries
To optimize SQL query performance, it’s crucial to regularly monitor query execution and make necessary adjustments. Many database management systems (DBMS) offer tools that help identify slow-running queries and provide insights into how queries are processed.
Techniques for Monitoring and Tuning Queries:
Use EXPLAIN (or EXPLAIN ANALYZE):
- The EXPLAIN statement helps you analyze how the database executes a query by showing the query plan. It breaks down each step the database takes, including which indexes are used and whether a full table scan is happening. EXPLAIN ANALYZE (in PostgreSQL) not only shows the query plan but also the actual execution time.
-- Use EXPLAIN to analyze query performance:
EXPLAIN SELECT * FROM orders WHERE customer_id = 12345;
Identify Bottlenecks:
- Look for common bottlenecks in the query plan, such as full table scans, unoptimized joins, or missing indexes. Queries that scan the entire table instead of using an index can be significantly slower, especially with large datasets. Focus on optimizing these areas.
Monitor Query Performance Over Time:
- Use database monitoring tools such as MySQL’s Slow Query Log, PostgreSQL’s pg_stat_statements, or third-party tools like New Relic and Datadog to track long-running queries. Regularly review logs to identify problematic queries and tune them as your data grows.
-- Enable slow query log in MySQL:
SET GLOBAL slow_query_log = 'ON';
Optimize Query Cache Usage:
- Many databases have query caching mechanisms that store the results of frequently run queries. Ensure that your queries are optimized to take advantage of caching when possible. Cached queries can speed up repeated query execution by reusing the result instead of reprocessing the query.
-- Enable query cache (MySQL example):
SET GLOBAL query_cache_size = 16777216;
Tune Memory Settings:
- Memory allocation plays a big role in query performance. Depending on the size of your database, tuning memory settings like innodb_buffer_pool_size (for MySQL) or work_mem (for PostgreSQL) can improve how much data can be processed in memory, reducing reliance on slower disk I/O.
Parallelize Queries (PostgreSQL):
- In PostgreSQL, you can take advantage of parallel query execution, which splits the query into multiple parallel processes. This can speed up large queries, particularly those involving heavy aggregations or joins.
-- Enable parallel queries in PostgreSQL:
SET max_parallel_workers_per_gather = 4;
Test with Different Query Structures:
- After identifying slow queries, experiment with different query structures or approaches to see which performs best. Sometimes changing the order of joins, filtering conditions earlier, or even splitting a query into multiple parts can yield better results.
By regularly monitoring your queries and using tools like EXPLAIN and database logs, you can gain insight into how your database is processing queries. Tuning slow queries ensures that your database continues to run efficiently, even as data grows or query complexity increases.
Python Interpreter with 2 lines of code!
Common Pitfalls to Avoid
Even with the best intentions, it’s easy to make mistakes that can degrade SQL query performance. Here are some common pitfalls to avoid:
Using SELECT * Instead of Specifying Columns:
- Retrieving all columns with SELECT * can slow down queries, especially with large tables or when not all columns are needed. Always specify only the columns you need.
-- Avoid:
SELECT * FROM employees;
-- Better:
SELECT first_name, last_name, email FROM employees;
Not Using Indexes Properly:
- Failing to index the right columns or over-indexing can negatively impact performance. Indexes should be created on columns that are frequently used in WHERE, JOIN, and ORDER BY clauses, but avoid indexing every column, as this can slow down INSERT, UPDATE, and DELETE operations.
Ignoring Query Execution Plans:
- Failing to analyze the execution plan (EXPLAIN) means you miss out on crucial insights into how the query is processed. Not knowing if the query is performing a full table scan or skipping indexes can prevent you from optimizing efficiently.
Overusing DISTINCT:
- Using DISTINCT unnecessarily can slow down queries, as the database has to process and filter out duplicate rows. Only use it when absolutely necessary.
-- Avoid:
SELECT DISTINCT employee_id FROM employees WHERE department = 'Sales';
-- Better:
SELECT employee_id FROM employees WHERE department = 'Sales';
Inefficient Joins:
- Joining too many tables without considering the most efficient way to do so can slow down queries. Avoid joining unnecessary tables, and ensure that join conditions are based on indexed columns.
Not Filtering Early:
- Failing to use WHERE clauses early in the query can result in large amounts of unnecessary data being processed. Always filter the data as early as possible to minimize the rows the database needs to work with.
-- Inefficient:
SELECT department, COUNT(*)
FROM employees
GROUP BY department
HAVING department = 'HR';
-- Efficient:
SELECT department, COUNT(*)
FROM employees
WHERE department = 'HR'
GROUP BY department;
Over-Relying on Subqueries:
- Subqueries can sometimes slow down queries, particularly correlated subqueries that run for each row returned by the main query. Where possible, try using joins or Common Table Expressions (CTEs) instead.
Misusing OR Clauses:
- Using OR in WHERE clauses can prevent the database from using indexes efficiently. Refactor OR conditions into separate queries or use UNION if appropriate.
Avoiding these common pitfalls can prevent many performance issues and help ensure your queries remain efficient as your application and database grow.
Lambda Functions: A Powerful Tool for Simplifying Code
Conclusion
Optimizing SQL queries is a critical skill for anyone working with databases. Efficient queries not only improve application performance but also reduce resource consumption and ensure that your system can scale as data grows.
By using strategies like indexing, optimizing WHERE clauses, limiting data retrieval, refactoring complex queries, and monitoring query performance, you can significantly improve the speed and efficiency of your SQL operations. Additionally, being mindful of common pitfalls such as overusing SELECT *, ignoring execution plans, and inefficient joins can help you avoid many performance bottlenecks.
In a data-driven world, efficient query performance ensures faster, more responsive applications, lower costs, and happier users. Regularly review and optimize your queries to keep your database running at peak performance, and don’t hesitate to experiment with new techniques and tools to stay ahead of any potential slowdowns.
Thank you for reading, and happy optimizing!
Final Words:
Thank you for taking the time to read my article.
This article was first published on Medium by CyCoderX.
Hey There! I’m CyCoderX, a data engineer who loves crafting end-to-end solutions. I write articles about Python, SQL, AI, Data Engineering, lifestyle and more!
If you want to explore similar articles and updates, feel free to explore my Medium profile:
Join me as we explore the exciting world of tech, data and beyond!
What did you think about this article? Let me know in the comments below … or above, depending on your device! 🙃
Please consider supporting me by:
- Clapping 50 times for this story
- Leaving a comment telling me your thoughts
- Highlighting your favorite part of the story
Optimizing SQL Queries: Tips and Techniques for Faster Performance was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by CyCoderX
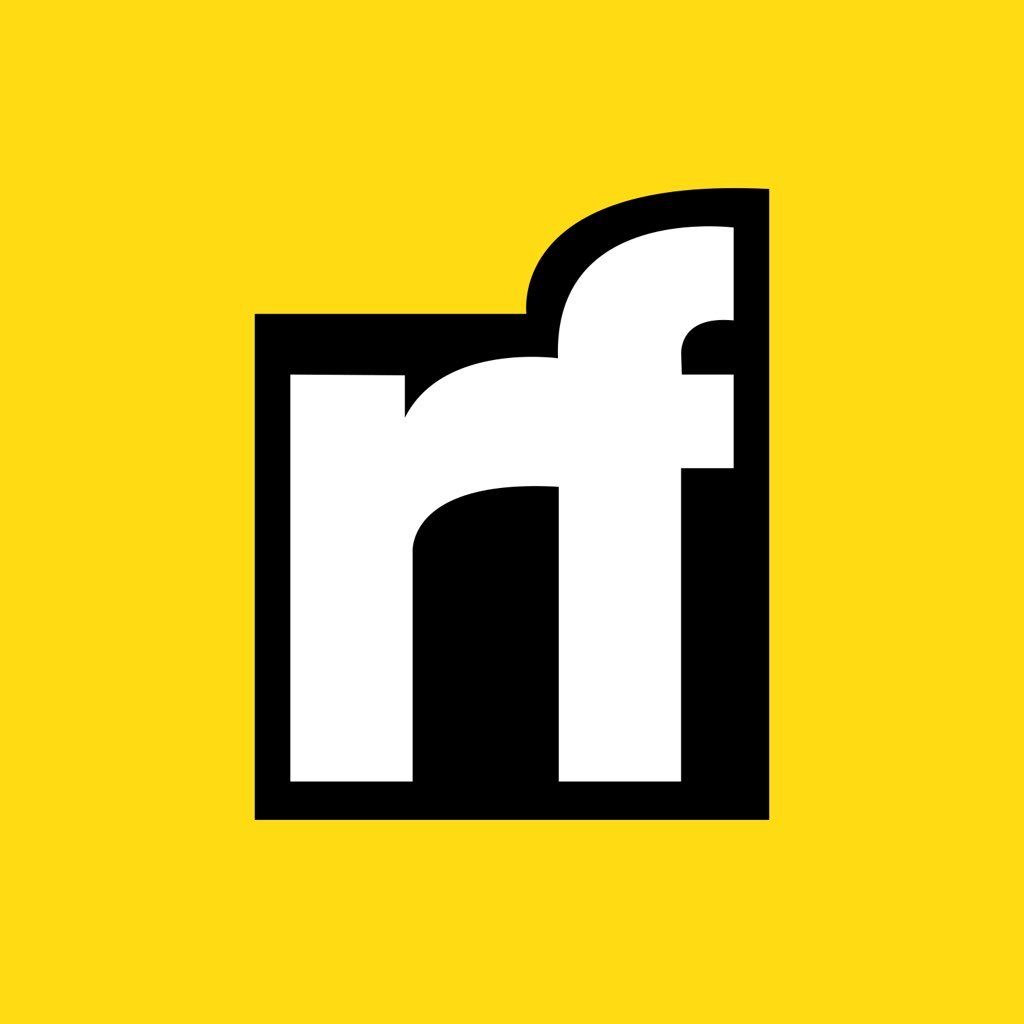
CyCoderX | Sciencx (2024-09-27T02:00:13+00:00) Optimizing SQL Queries: Tips and Techniques for Faster Performance. Retrieved from https://www.scien.cx/2024/09/27/optimizing-sql-queries-tips-and-techniques-for-faster-performance/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.