This content originally appeared on Level Up Coding - Medium and was authored by Mohamed Ayman
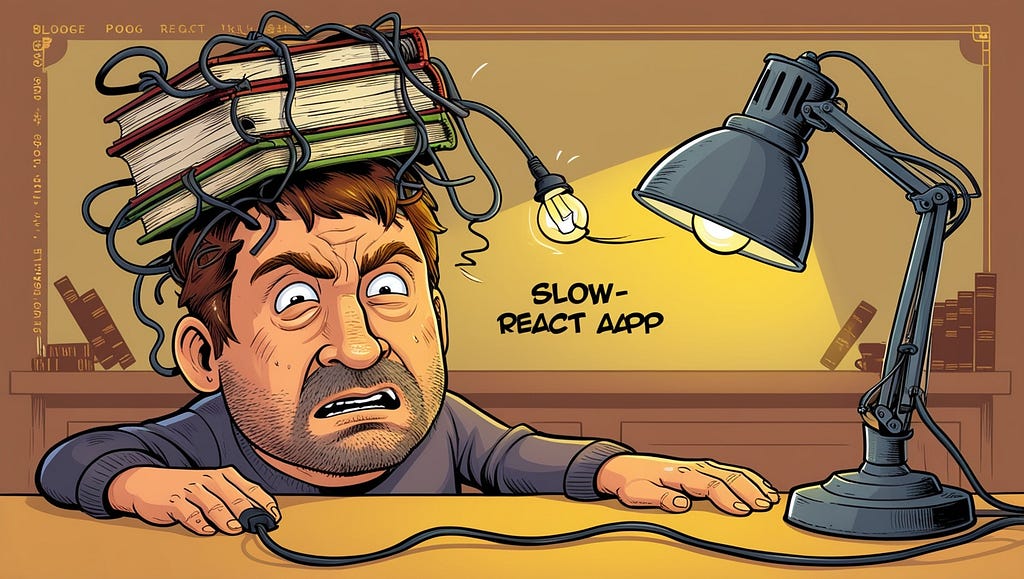
In modern web development, performance is key to delivering a smooth user experience. React and Next JS are popular choices for building dynamic, fast applications, but poorly optimized code can slow things down. In this post, I will share five proven strategies to boost performance in your React and Next JS apps.
Table of contents:
· Why You Should Optimize Your React App:
· 1. Minimize Re-renders with React.memo:
· 2. Use useMemo and useCallback Hooks for Optimization
· 3. Use Code Splitting with React.lazy and Suspense
· 4. Optimize Images with Next.js Image Component
· 5. Implement Server-Side Rendering (SSR) and Static Generation (SSG)
· Conclusion
Why You Should Optimize Your React App:
- Improved User Experience: Fast apps enhance user satisfaction. When your app loads quickly, users stay longer, engage more, and are less likely to leave, improving overall retention.
- Better SEO: Search engines like Google favor fast-loading sites. Optimizing performance boosts your ranking, increasing visibility and driving more organic traffic to your app.
- Reduced Costs: Optimized apps use fewer server resources and bandwidth, which can lower infrastructure and hosting costs, especially for apps with high traffic.
- Scalability: An optimized app is better equipped to handle growth. It can manage higher traffic, more users, and larger datasets without compromising performance.
- Mobile Performance: Mobile users often have slower connections, so optimizing ensures your app loads quickly on mobile, providing a seamless experience regardless of device or network speed.
1. Minimize Re-renders with React.memo:
Unnecessary re-renders can slow down your app. React.memo helps by preventing re-renders when the props haven’t changed.
Example:
const MyButton = React.memo(({ label }) => {
console.log('Rendering button…');
return <button>{label}</button>;
});
function App() {
return (
<div>
<MyButton label="Click Me" />
<MyButton label="Click Me" /> {/* Will not re-render */}
</div>
);
}
What Happens:
In the console, you’ll see “Rendering button…” only once, even though MyButton is rendered twice. This shows that React.memo successfully prevented the second button from re-rendering since it had the same props. Without React.memo, both buttons would have re-rendered.
2. Use useMemo and useCallback Hooks for Optimization
The useMemo and useCallback hooks help optimize performance by memoizing expensive calculations and preventing unnecessary re-renders of child components.
Difference Between useMemo and useCallback:
- useMemo: Returns a memoized value from a computation, useful for optimizing expensive calculations. It recalculates the value only when specified dependencies change.
- useCallback: Returns a memoized function, ideal for preventing unnecessary re-renders of child components that rely on reference equality. It creates a new function only when its dependencies change.
When to Use Each One:
- Use useMemo when:
- You have an expensive calculation that should not run on every render.
- You want to derive a value based on props or state. - Use useCallback when:
- Passing a function as a prop to a child component to avoid unnecessary re-renders.
- The function needs to remain stable and updated only when necessary.
Example (useMemo)
import React, { useState, useMemo } from 'react';
function ExpensiveComponent({ value }) {
const computedValue = useMemo(() => {
console.log('Computing expensive value...');
return value * 2;
}, [value]);
return <div>Computed Value: {computedValue}</div>;
}
function App() {
const [count, setCount] = useState(0);
return (
<div>
<ExpensiveComponent value={count} />
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
What You’ll See:
The console logs “Computing expensive value…” only when count changes, demonstrating that the calculation is optimized.
Example (useCallback)
import React, { useState, useCallback } from 'react';
const Button = React.memo(({ onClick }) => {
console.log('Button rendered');
return <button onClick={onClick}>Click Me</button>;
});
function App() {
const [count, setCount] = useState(0);
const increment = useCallback(() => {
setCount((prevCount) => prevCount + 1);
}, []);
return (
<div>
<p>Count: {count}</p>
<Button onClick={increment} />
</div>
);
}
Result:
“Button rendered” appears only once, as useCallback prevents unnecessary re-renders.
3. Use Code Splitting with React.lazy and Suspense
Loading large JavaScript bundles can slow down your app. Code splitting allows you to load only the code required for a specific part of your app.
Example:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<h1>Welcome to my app!</h1>
<React.Suspense fallback={<div>Loading…</div>}>
<LazyComponent />
</React.Suspense>
</div>
);
}
Outcome:
While the LazyComponent is being fetched, the user will see “Loading...” displayed on the screen. Once the component is loaded, it appears in place of the loading text. This setup allows the main content to load quickly while deferring parts of the app until they are actually needed.
4. Optimize Images with Next.js Image Component
Heavy images slow down load times. Next.js’s Image component optimizes images automatically for faster loading and better performance.
Example:
import Image from 'next/image';
function App() {
return (
<div>
<h1>Optimized Image Example</h1>
<Image
src="/image.jpg"
alt="Beautiful Landscape"
width={800}
height={500}
/>
</div>
);
}
What to Expect:
The image is displayed in an optimized format, with the appropriate size based on the user’s device. This ensures fast loading times, even for users with slower internet connections or on mobile devices. The Image component also supports lazy loading, which loads the image only when it’s visible on the screen, further improving performance.
5. Implement Server-Side Rendering (SSR) and Static Generation (SSG)
SSR and SSG allow you to serve pages faster by generating HTML on the server or at build time.
Example (SSG with Next.js):
export async function getStaticProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: {
data,
},
};
}
function Home({ data }) {
return (
<div>
<h1>Pre-rendered Data</h1>
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
}
What You’ll See:
Once the page loads, the list of data will be instantly visible to the user because it was pre-rendered at build time. This means users receive the HTML quickly, without waiting for the data to be fetched on the client side, improving both load times and SEO.
Conclusion
Optimizing your React and Next JS apps for performance is essential to ensure a smooth user experience and better engagement. By following best practices such as preventing unnecessary re-renders, using code splitting, optimizing images, choosing the right state management, and leveraging SSR or SSG, you can significantly improve your app’s performance.
What challenges have you faced while optimizing your React apps? Share your experiences with me!
If you want your React app to still be slow, don’t read this article. was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Mohamed Ayman
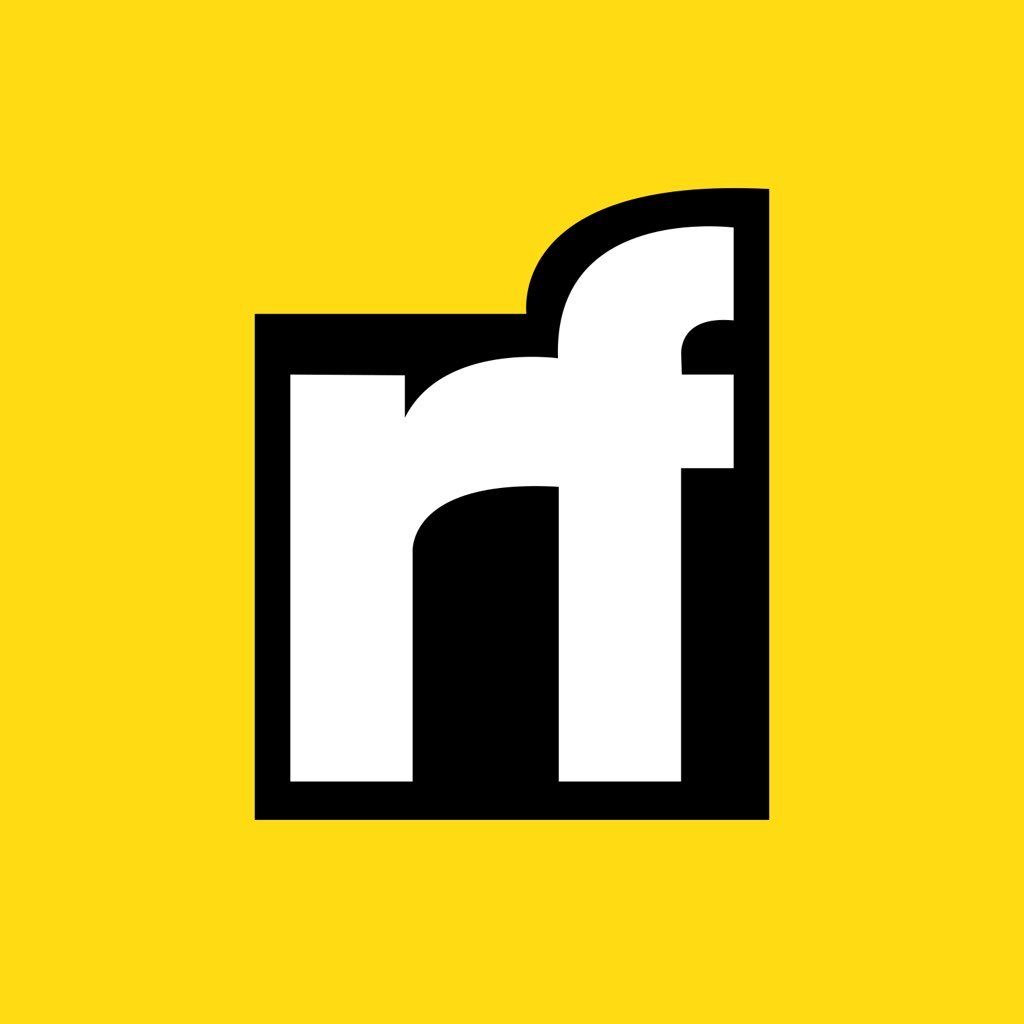
Mohamed Ayman | Sciencx (2024-10-01T17:49:14+00:00) If you want your React app to still be slow, don’t read this article.. Retrieved from https://www.scien.cx/2024/10/01/if-you-want-your-react-app-to-still-be-slow-dont-read-this-article/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.