This content originally appeared on Telerik Blogs and was authored by Nada Rifki
These 10 features of Nuxt 3 can enhance your Vue application development beyond Vue alone.
If you’ve read my previous articles, you know that I’m a Vue gal and that I love Nuxt! ♀️
In a nutshell, Nuxt is a powerful framework built on top of Vue that gives your Vue apps superpowers.
In this article, I discuss 10 important features of Nuxt 3 and why you should learn more about this framework if you are familiar with Vue. It’s another learning curve, but you will be able to enhance your Vue applications and take them to the next level.
Installing Nuxt
Creating a new Nuxt project is as easy as typing npx nuxi@latest init
in your terminal. Head over the official installation page for more details.
But First, Say Hello to TypeScript
JavaScript is great, but have you ever had to deal with an error in production that you didn’t catch in the dev or staging environment because of a type mismatch?!
Yes, yes, I have, and many web developers have had to deal with that too. Especially when you’re in a small team and/or when you don’t have code reviews. It even happens, in very rare occurrences, in big teams with a staging server, code reviews and pre-prod environments.
So do yourself, your team and your clients a favor and use TypeScript to avoid bugs and maintain consistency throughout your codebase. The good news is that Nuxt 3 was built to be a first-class TypeScript citizen. To be honest, using Nuxt 3 without TypeScript seems like a strange choice to me. One of the reasons almost everyone switched to the Composition API is because of its better integration with TypeScript.
To give you one great example, you will be able to see right from your editor when you are sending something wrong to one component prop. In our projects, we usually put all of our types in a folder called types at the root. I will create a file called bases.d.ts inside with the following content.
type BaseButtonColor = 'blue' | 'gray' | 'green'
Now, in my BaseButton
component, I will use something called PropTypes
(that is automatically imported in Nuxt) like this:
<script lang="ts" setup>
// Props
const props = defineProps({
color: {
default: 'gray',
type: String as PropType<BaseButtonColor>,
},
})
</script>
If I try to use this BaseButton
component somewhere and add the color red, your editor will warn you that this prop is not correct.
Of course, using TypeScript comes with many more benefits that you will discover along your journey, but believe me, once you make the switch, there is no going back.
Feature #1: Nuxt Makes Routing More Intuitive!
Nuxt 3 comes with an automatic file-based routing system, which simplifies the creation and management of your routes. As you remember, that’s different from Vue, where you have to set your routes manually. This means that now, you don’t have to get lost anymore in the maze that your router file becomes when your app scales up.
In Nuxt, your file structure dictates your routes. Each file is a route. As you can see in the documentation, if you structure your files like this, for example:
| pages/
---| about.vue
---| index.vue
---| posts/
-----| [id].vue
This will automatically generate this router structure:
{
"routes": [
{
"path": "/about",
"component": "pages/about.vue"
},
{
"path": "/",
"component": "pages/index.vue"
},
{
"path": "/posts/:id",
"component": "pages/posts/[id].vue"
}
]
}
Handy right!? ♀️
I’ve never had any issues with it, so I can’t recommend enough that you use the file system routing if you like it. It’s, in my opinion, the best way to quickly organize your application. As you can see, with Nuxt there is one less file to deal with.
Feature #2: Nuxt Uses Convention over Configuration
What does convention over configuration mean and why should we care?
Nuxt favors predefined conventions and opinionated defaults over requiring developers to explicitly configure every aspect of their app. This approach saves us a lot of time and allows us to focus on writing the unique parts of our application rather than setting up the environment. Of course, it means that you have to follow the conventions, but it’s a small price to pay for the benefits it brings.
This is something I enjoy about Nuxt. Everything is properly organized, and you don’t have to think a lot about the structure of your application. You just follow the framework’s best practices. For example, Nuxt includes powerful middleware and layout systems that you can use right from the start. And if you need to create your own library of components, just use the Nuxt layers as it provides a lot of flexibility (and believe me, it was harder in the past; I even wrote an article about it). ♀️
Note: You are working with a higher level of abstraction, and things can sometimes seem magical, but the good news is that with the Nuxt DevTools, you can see everything that is happening under the hood. ♀️ P.S. Here is my Get Started with Nuxt DevTools piece.
Another powerful aspect of Nuxt 3 is its runtime configuration. When you need to manage different environments (development, staging, production) without needing to rebuild your application, this feature allows you to maintain consistent behavior across various environments with minimal effort.
My point is that by choosing a framework that embraces this philosophy, you will enhance your development workflow, maintain cleaner code and build scalable applications with ease.
Feature #3: Nuxt Auto-Imports All Your Composables
One thing I enjoy about Nuxt 3 is that everything exported in your composable folder is available globally in your app. This is incredibly handy as it makes our developer experience more seamless (we don’t have to make sure the right thing is imported). You also have access to native composables or the ones coming from modules like useLocalePath
, useI18n
, useFetch
, useAsyncData
and many more. Additionally, managing cookies is made easy with the useCookies
composable. ♀️
Note: If you need to see all the composables that are auto-imported, use the DevTools by going to imports or composables.
One thing I like is to have a composable useIcons
where I put all my icons in one place. This way, I just have to call getIcon('myIcon')
. For example, you can use Iconify, which is free and comes with thousands of icons from multiple packs.
const icons = {
check: 'solar:check-circle-linear',
chevronLeft: 'solar:alt-arrow-left-linear',
chevronRight: 'solar:alt-arrow-right-linear',
} as const
export function getIcon(attribute: keyof typeof icons) {
return icons[attribute] || icons.default
}
export type UIcon = keyof typeof icons
Feature #4: Nuxt Comes with Different Rendering Modes for Better Performance
Like Next for React, one of the killer features of Nuxt has always been its server-side rendering (also known as SSR). In contrast, Vue 3 requires additional setup and configuration for SSR.
To summarize what SSR is about, it simply means that the server will fully render the HTML page before sending it to the client. This will improve SEO, the initial load performance (compared to client-side rendering) and the overall user experience. I have dedicated an entire section about why Nuxt is a better choice for SEO than using Vue, but as you can guess SSR is one of the main reasons people got attracted to Nuxt. By allowing webpages to be displayed faster, we get them indexed more effectively by search engines.
Also, over the years, Nuxt has evolved, and four additional rendering modes are now available:
- Static Site Generation (SSG): This means the page is pre-rendered at build time, resulting in static HTML files that enhance performance and security. You will have many HTML files ready to be served to your users that you can easily deploy on a CDN like Netlify or Vercel.
- Client-Side Rendering (CSR): Like a classic Vue app, the page is entirely rendered in the browser. It may benefit highly interactive applications but results in slower initial load times.
- Hybrid Rendering: It combines SSR, SSG and CSR, allowing different pages or routes to use different rendering modes as needed. This is a great feature that allows you to choose the best rendering mode for each page of your application.
- And the last one… Edge-Side Rendering (ESR): I’ve never used it, but it offloads the rendering process of your page to the CDN’s edge servers. As explained in the documentation: When a request for a page is made, instead of going all the way to the original server, it’s intercepted by the nearest edge server. This server generates the HTML for the page and sends it back to the user. This process minimizes the physical distance the data has to travel, reducing latency and loading the page faster.
If you want to know more about the different rendering modes, I recommend you read the Nuxt documentation.
Feature #5: Nuxt Will Make Your SEO Life Easier
We talked about SSR, but Nuxt 3 also comes with built-in composables that greatly enhance your SEO capabilities: useSeoMeta
. You can easily set your meta tags inside your <head>
section, titles and other SEO-related information with this composable. As Nuxt auto-imports all your composables, you can use it globally anywhere in your pages.
useSeoMeta({
description: 'My awesome description',
title: 'Welcome to my website',
titleTemplate: 'Project | %pageTitle',
})
There are over 100 parameters that you can use. You can see the full list here.
Additionally, there’s a fantastic package called Nuxt SEO that you will need. It is a collection of different modules that you can add to your app to easily deal with your sitemap (@nuxtjs/sitemap
), your robots.txt (nuxt-simple-robots
), your schema org or even to generate dynamic OG Images.
Note: If you use the Nuxt DevTools, take a look at the SEO section to see all your missing meta tags. It’s a great way to quickly check if everything is set correctly. ♀️
Feature #6: Nuxt Allows You to Write Your Own API
Nuxt 3 is a full-stack framework. This means you can write your own API directly in Nuxt. There’s no need to add another framework to your stack like Adonis or NestJS. Your backend logic, such as creating or managing user accounts, can be handled directly within Nuxt. ️♀️
For detailed information, check out the Nuxt API documentation. Take a moment to read through it because it’s really cool!
// server/api/hello.ts
export default defineEventHandler((event) => {
return {
hello: 'world'
}
})
This is a significant advantage over Vue 3, which focuses primarily on the frontend and requires additional backend configuration to achieve similar functionality.
Note: By utilizing
@sentry/vue
, you can catch errors in your API routes, monitor what’s going wrong and continuously improve your app’s performance and reliability.
Feature #7: Data Fetching with Nuxt Is Easier
Nuxt 3 simplifies data fetching with composables like useAsyncData
and useFetch
. These composables are designed to work seamlessly with SSR, making it easier to manage asynchronous data fetching without the hassle of additional boilerplate code. They also provide additional options for handling loading states, errors and caching, enhancing the overall user experience.
<script setup lang="ts">
const { data, status, error, refresh, clear } = await useFetch('/api/user', {
pick: ['username', 'email', 'avatar']
})
// data: the result of the asynchronous function that is passed in.
// refresh: a function that can be used to refresh the data returned by the handler function.
// error: an error object if the data fetching failed.
// status: a string indicating the status of the data request ("idle", "pending", "success", "error").
// clear: a function which will set data to undefined, set error to null, set status to 'idle', and mark any currently pending requests as cancelled.
</script>
In contrast, Vue 3 requires you to manage data fetching manually with a library like Axios.
Feature #8: Nuxt Has an I18N Module to Create Multilingual Applications
If you know that your application will be in multiple languages, I can’t recommend Nuxt I18N enough to start with from the beginning. This module makes it easy to create multilingual applications, saving you a lot of time and effort in the future.
It even allows you to use per-component translation (something I use intensively, as GitHub Copilot can easily translate for other languages).
<script setup lang="ts">
const { t } = useI18n({
useScope: 'local'
})
</script>
<template>
<p>{{ t('hello') }}</p>
</template>
<i18n lang="json">
{
"en": {
"hello": "hello world!"
},
"ja": {
"hello": "こんにちは、世界!"
}
}
</i18n>
Useful composables you will need all the time are: useLocalePath
, useI18n
and useSwitchLocalePath
.
Feature #9: Nuxt Has Nuxt Content, a Module to Manage Content
If you have a lot of content to write and want to use Markdown, use Nuxt Content. It will be automatically converted to HTML, making it ideal for blogs, documentation and static content.
You will have access to composables like queryContent
to query your content, and you can even use your own Vue component inside your Markdown files.
::alert{type="warning"}
The **alert** component.
::
I always use Nuxt Content for articles, privacy policies and other content needs. Additionally, consider using Nuxt Studio, a CMS for Nuxt Content, though it comes at a cost.
Feature #10: Nuxt Comes with a Lot of Modules
I could not end this article without mentioning one big advantage of Nuxt: its rich ecosystem of modules. There is a module for almost everything you need, from authentication to PWA, analytics and more. And what’s best is that it is easy to add powerful features to your application with minimal configuration. ♀️
For example, it’s easy to create a dark mode with the @nuxtjs/color-mode
module or optimize all your images with the @nuxt/image
module.
So don’t reinvent the wheel, and take a look at the modules that are available. You will be surprised by how much time you can save by using them.
Conclusion
I hope I could convince you to have a look at Nuxt. Next time you start a new project, consider using Nuxt instead of Vue if it makes more sense to you. I am sure it will make your life easier and your applications better.
You can reach me on Twitter @RifkiNada. And in case you are curious about my work or other articles, you can look at them here www.nadarifki.com.
Thank you for reading!
This content originally appeared on Telerik Blogs and was authored by Nada Rifki
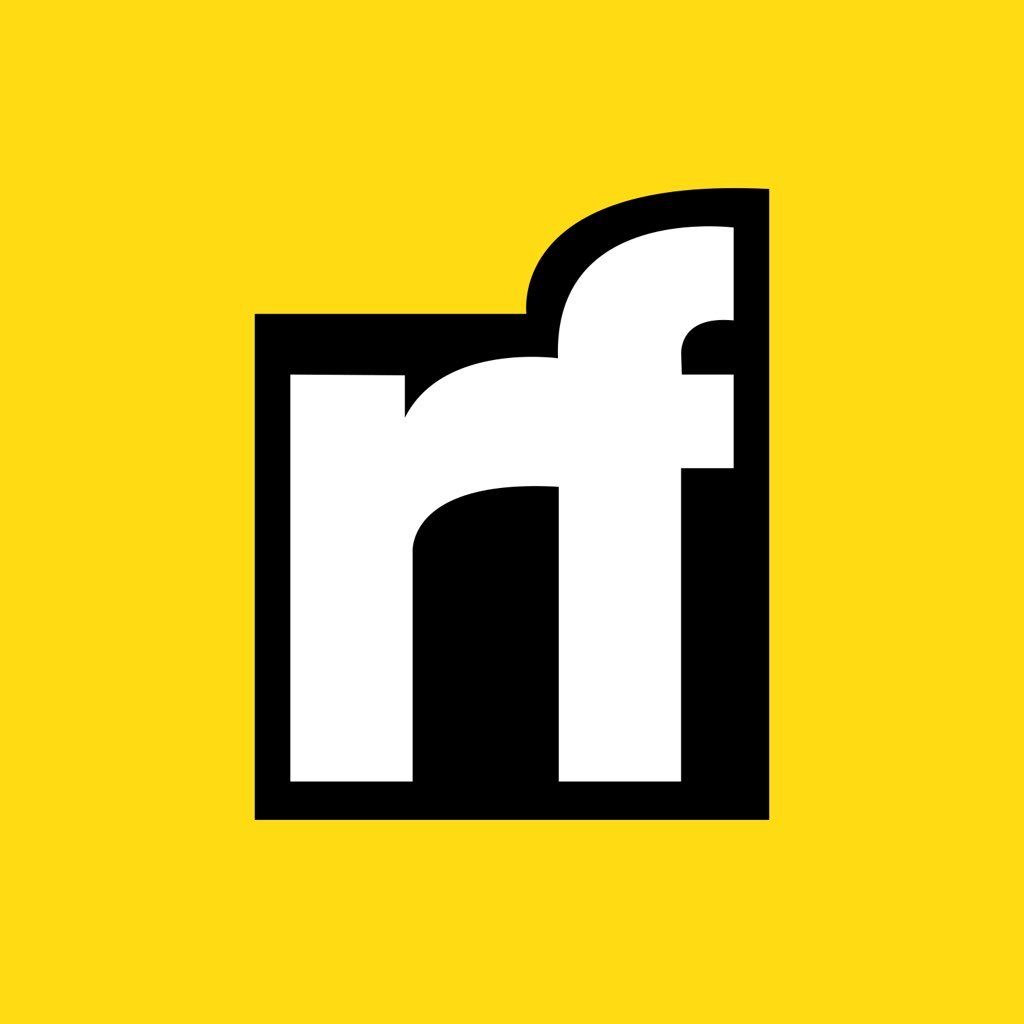
Nada Rifki | Sciencx (2024-10-01T09:16:11+00:00) Vue Basics: How to Develop Better Vue Applications with Nuxt. Retrieved from https://www.scien.cx/2024/10/01/vue-basics-how-to-develop-better-vue-applications-with-nuxt/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.