This content originally appeared on DEV Community and was authored by Victor Melo
Starting today a diary on the development of an online game, as a hobbie.
My goal is to use Rust and Go, two languages I have interest on learning, but don't have any experience.
Client will be implemented with Rust, while the server will be done using Go.
On this first day, I started by the client. My goal was to show a window and render anything. I found a library ggez
and used it.
The result can be seen below:
On every update, it increments the counter.
The implemented code is below:
use ggez::{ContextBuilder, GameResult, event};
use ggez::graphics::{Canvas, Color, Text, DrawParam, PxScale};
struct Game {
counter: u32, // a simple counter to track the number of frames
}
impl Game {
fn new() -> Self {
Game {
counter: 0,
}
}
}
impl event::EventHandler for Game {
fn update(&mut self, _ctx: &mut ggez::Context) -> GameResult {
self.counter += 1; // Increment the counter on each update
Ok(())
}
fn draw(&mut self, ctx: &mut ggez::Context) -> GameResult {
// Clear the screen with a background color
let mut canvas = Canvas::from_frame(ctx, Color::BLACK);
let mut counter_text = Text::new(format!("Counter: {}", self.counter));
counter_text.set_scale(PxScale::from(48.0)); // Set the font size to 48 pixels
canvas.draw(&counter_text, DrawParam::default().dest([50.0, 50.0]));
canvas.finish(ctx)?;
Ok(())
}
}
fn main() -> GameResult {
let (ctx, event_loop) = ContextBuilder::new("rpg-client", "Victor Melo")
.window_setup(ggez::conf::WindowSetup::default().title("RPG Client"))
.window_mode(ggez::conf::WindowMode::default().dimensions(1280.0, 720.0)) // Set window size to 1280x720
.build()
.unwrap();
let game = Game::new();
event::run(ctx, event_loop, game)
}
This content originally appeared on DEV Community and was authored by Victor Melo
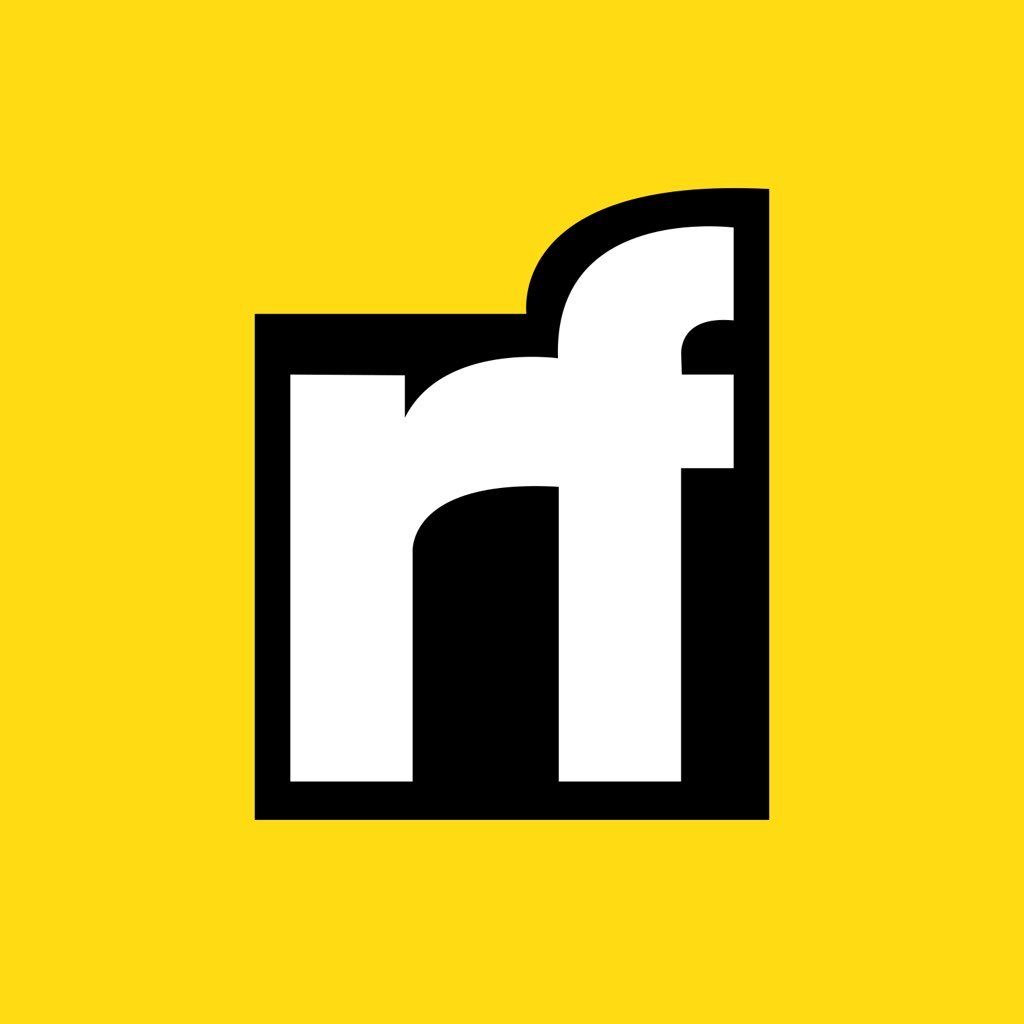
Victor Melo | Sciencx (2024-10-24T19:57:14+00:00) VRPG day 1. Retrieved from https://www.scien.cx/2024/10/24/vrpg-day-1/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.