This content originally appeared on DEV Community and was authored by Alexandre Calaça
Introduction
Encountering errors while resetting your database or managing password fields can be a challenging part of Rails development.
In this simple article, I’ll walk through the process of resolving the BCrypt::Errors::InvalidHash
error, which occurred after recreating a user password. Here’s what happened, how I tested possible solutions, and the final approach that resolved the issue.
Context
After running rails db:drop to drop the database and recreate it, I had to re-enter user data, including setting a new password. However, when attempting to assign a password to the encrypted_password field directly in the User model, I encountered the following error:
BCrypt::Errors::InvalidHash (invalid hash)
This error typically occurs when the value stored in encrypted_password is not a valid BCrypt hash. In Rails, if you're using has_secure_password, BCrypt expects a hashed value in encrypted_password. Assigning a plain-text password directly to encrypted_password without hashing causes this mismatch.
Inital setup
After the database reset, I attempted to assign a password by manually setting encrypted_password:
user = User.new(email: "user@example.com")
user.encrypted_password = "newpassword123" # Incorrect approach
user.save
Solution
To resolve the error, I needed to ensure the password was properly hashed using BCrypt. Instead of manually assigning a value to encrypted_password, I updated the user’s password attribute directly and let BCrypt handle the hashing.
Here’s the code that worked:
User.where(email: "email@example.com").first
hashed_password = BCrypt::Password.create("newpassword#0")
user.update_column(:encrypted_password, hashed_password)
Explanation
In this solution, I used BCrypt::Password.create to hash the plain-text password "newpassword#0", then directly updated the password attribute.
This approach allowed BCrypt to generate a valid hash for encrypted_password, avoiding the BCrypt::Errors::InvalidHash error. By assigning the hashed password to password, Rails automatically handled storage in the correct format, fixing the issue.
This content originally appeared on DEV Community and was authored by Alexandre Calaça
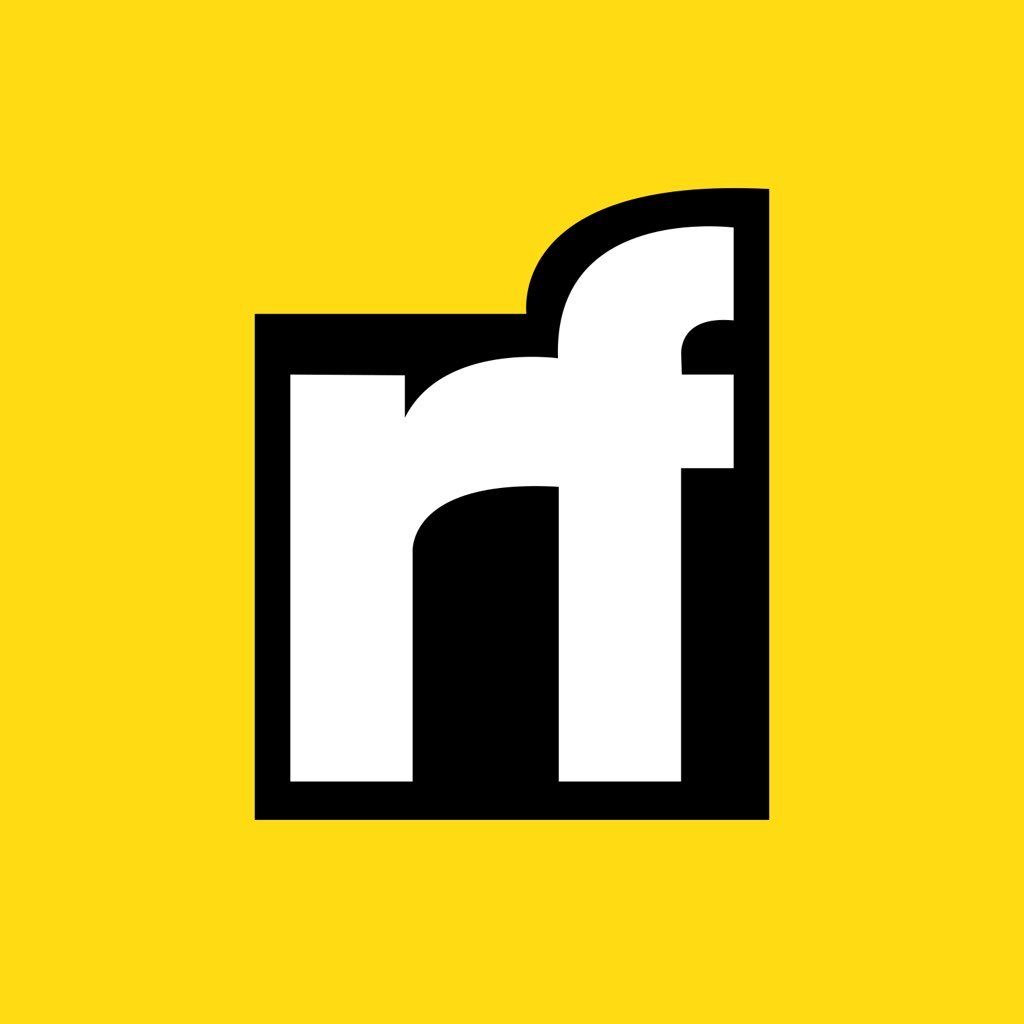
Alexandre Calaça | Sciencx (2024-10-28T17:35:12+00:00) BCrypt::Errors::InvalidHash (invalid hash). Retrieved from https://www.scien.cx/2024/10/28/bcrypterrorsinvalidhash-invalid-hash/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.